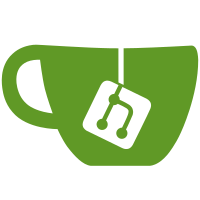
25 changed files with 1116 additions and 2 deletions
@ -1,2 +1,68 @@ |
|||||||
# core-php |
# Open Legends Core |
||||||
Open Legends Core Library in PHP 8 |
|
||||||
|
Project under development! |
||||||
|
|
||||||
|
## Install |
||||||
|
|
||||||
|
`composer require openlegends/core:dev-main` |
||||||
|
|
||||||
|
## Test features |
||||||
|
|
||||||
|
### Cards |
||||||
|
|
||||||
|
`composer require openlegends/asset:dev-main` |
||||||
|
|
||||||
|
``` |
||||||
|
<?php |
||||||
|
|
||||||
|
require_once __DIR__ . '/../vendor/autoload.php'; |
||||||
|
|
||||||
|
$mouse = new \OpenLegends\Asset\Test\Card\Mouse(); |
||||||
|
$rat = new \OpenLegends\Asset\Test\Card\Rat(); |
||||||
|
|
||||||
|
$damage = new \OpenLegends\Asset\Test\Card\Action\Damage( |
||||||
|
$mouse |
||||||
|
); |
||||||
|
|
||||||
|
$damage->card( |
||||||
|
$rat |
||||||
|
); |
||||||
|
|
||||||
|
var_dump( |
||||||
|
$mouse->getHealth() |
||||||
|
); |
||||||
|
|
||||||
|
var_dump( |
||||||
|
$rat->getHealth() |
||||||
|
); |
||||||
|
``` |
||||||
|
|
||||||
|
### Pool |
||||||
|
|
||||||
|
Useful for decks, hand, discard pile, etc |
||||||
|
|
||||||
|
`composer require openlegends/asset:dev-main` |
||||||
|
|
||||||
|
``` |
||||||
|
<?php |
||||||
|
|
||||||
|
require_once __DIR__ . '/../vendor/autoload.php'; |
||||||
|
|
||||||
|
$deck = new \OpenLegends\Asset\Test\Pool\Card(); |
||||||
|
|
||||||
|
$deck->addCard( |
||||||
|
new \OpenLegends\Asset\Test\Card\Goblin() |
||||||
|
); |
||||||
|
|
||||||
|
$deck->addCard( |
||||||
|
new \OpenLegends\Asset\Test\Card\Mouse() |
||||||
|
); |
||||||
|
|
||||||
|
$deck->addCard( |
||||||
|
new \OpenLegends\Asset\Test\Card\Rat() |
||||||
|
); |
||||||
|
|
||||||
|
var_dump( |
||||||
|
$deck->getRandomCard()->getName() |
||||||
|
); |
||||||
|
``` |
@ -0,0 +1,16 @@ |
|||||||
|
{ |
||||||
|
"name": "openlegends/core", |
||||||
|
"description": "Core Library for OpenLegends Game", |
||||||
|
"type": "library", |
||||||
|
"license": "MIT", |
||||||
|
"autoload": { |
||||||
|
"psr-4": { |
||||||
|
"OpenLegends\\Core\\": "src/" |
||||||
|
} |
||||||
|
}, |
||||||
|
"authors": [ |
||||||
|
{ |
||||||
|
"name": "OpenLegends" |
||||||
|
} |
||||||
|
] |
||||||
|
} |
@ -0,0 +1,235 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract; |
||||||
|
|
||||||
|
abstract class Card implements \OpenLegends\Core\Interface\Card |
||||||
|
{ |
||||||
|
private \OpenLegends\Core\Abstract\Card\Ability $_ability; |
||||||
|
private \OpenLegends\Core\Abstract\Card\Attribute $_attribute; |
||||||
|
private \OpenLegends\Core\Abstract\Card\Rarity $_rarity; |
||||||
|
private \OpenLegends\Core\Abstract\Card\Type $_type; |
||||||
|
|
||||||
|
private string $_name; |
||||||
|
private string $_description; |
||||||
|
|
||||||
|
private int $_cost; |
||||||
|
private int $_power; |
||||||
|
private int $_health; |
||||||
|
private int $_craft; |
||||||
|
private int $_extract; |
||||||
|
|
||||||
|
private bool $_breakthrough; |
||||||
|
private bool $_cover; |
||||||
|
private bool $_drain; |
||||||
|
private bool $_guard; |
||||||
|
private bool $_lethal; |
||||||
|
private bool $_pilfer; |
||||||
|
private bool $_prophecy; |
||||||
|
private bool $_unique; |
||||||
|
|
||||||
|
abstract public function __construct(); |
||||||
|
|
||||||
|
abstract public function act( |
||||||
|
\OpenLegends\Core\Abstract\Game &$game |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getBreakthrough(): bool |
||||||
|
{ |
||||||
|
return $this->_breakthrough; |
||||||
|
} |
||||||
|
|
||||||
|
public function setBreakthrough(bool $value): void |
||||||
|
{ |
||||||
|
$this->_breakthrough = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getCover(): bool |
||||||
|
{ |
||||||
|
return $this->_cover; |
||||||
|
} |
||||||
|
|
||||||
|
public function setCover(bool $value): void |
||||||
|
{ |
||||||
|
$this->_cover = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDrain(): bool |
||||||
|
{ |
||||||
|
return $this->_drain; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDrain(bool $value): void |
||||||
|
{ |
||||||
|
$this->_drain = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getGuard(): bool |
||||||
|
{ |
||||||
|
return $this->_guard; |
||||||
|
} |
||||||
|
|
||||||
|
public function setGuard(bool $value): void |
||||||
|
{ |
||||||
|
$this->_guard = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getLethal(): bool |
||||||
|
{ |
||||||
|
return $this->_lethal; |
||||||
|
} |
||||||
|
|
||||||
|
public function setLethal(bool $value): void |
||||||
|
{ |
||||||
|
$this->_lethal = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getPilfer(): bool |
||||||
|
{ |
||||||
|
return $this->_pilfer; |
||||||
|
} |
||||||
|
|
||||||
|
public function setPilfer(bool $value): void |
||||||
|
{ |
||||||
|
$this->_pilfer = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getProphecy(): bool |
||||||
|
{ |
||||||
|
return $this->_prophecy; |
||||||
|
} |
||||||
|
|
||||||
|
public function setProphecy(bool $value): void |
||||||
|
{ |
||||||
|
$this->_prophecy = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getUnique(): bool |
||||||
|
{ |
||||||
|
return $this->_unique; |
||||||
|
} |
||||||
|
|
||||||
|
public function setUnique(bool $value): void |
||||||
|
{ |
||||||
|
$this->_unique= $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getName(): string |
||||||
|
{ |
||||||
|
return $this->_name; |
||||||
|
} |
||||||
|
|
||||||
|
public function setName(string $value): void |
||||||
|
{ |
||||||
|
$this->_name = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDescription(): string |
||||||
|
{ |
||||||
|
return $this->_description; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDescription(string $value): void |
||||||
|
{ |
||||||
|
$this->_description = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getCost(): string |
||||||
|
{ |
||||||
|
return $this->_cost; |
||||||
|
} |
||||||
|
|
||||||
|
public function setCost(int $value): void |
||||||
|
{ |
||||||
|
$this->_cost = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getPower(): int |
||||||
|
{ |
||||||
|
return $this->_power; |
||||||
|
} |
||||||
|
|
||||||
|
public function setPower(int $value): void |
||||||
|
{ |
||||||
|
$this->_power = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getHealth(): int |
||||||
|
{ |
||||||
|
return $this->_health; |
||||||
|
} |
||||||
|
|
||||||
|
public function setHealth(int $value): void |
||||||
|
{ |
||||||
|
$this->_health = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getCraft(): int |
||||||
|
{ |
||||||
|
return $this->_craft; |
||||||
|
} |
||||||
|
|
||||||
|
public function setCraft(int $value): void |
||||||
|
{ |
||||||
|
$this->_craft = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getExtract(): int |
||||||
|
{ |
||||||
|
return $this->_extract; |
||||||
|
} |
||||||
|
|
||||||
|
public function setExtract(int $value): void |
||||||
|
{ |
||||||
|
$this->_extract = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function setAbility( |
||||||
|
\OpenLegends\Core\Abstract\Card\Ability $ability |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_ability = $ability; |
||||||
|
} |
||||||
|
|
||||||
|
public function getAbility(): \OpenLegends\Core\Abstract\Card\Ability |
||||||
|
{ |
||||||
|
return $this->_ability; |
||||||
|
} |
||||||
|
|
||||||
|
public function setAttribute( |
||||||
|
\OpenLegends\Core\Abstract\Card\Attribute $attribute |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_attribute = $attribute; |
||||||
|
} |
||||||
|
|
||||||
|
public function getAttribute(): \OpenLegends\Core\Abstract\Card\Attribute |
||||||
|
{ |
||||||
|
return $this->_attribute; |
||||||
|
} |
||||||
|
|
||||||
|
public function setType( |
||||||
|
\OpenLegends\Core\Abstract\Card\Type $type |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_type = $type; |
||||||
|
} |
||||||
|
|
||||||
|
public function getType(): \OpenLegends\Core\Abstract\Card\Type |
||||||
|
{ |
||||||
|
return $this->_type; |
||||||
|
} |
||||||
|
|
||||||
|
public function setRarity( |
||||||
|
\OpenLegends\Core\Abstract\Card\Rarity $rarity |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_rarity = $rarity; |
||||||
|
} |
||||||
|
|
||||||
|
public function getRarity(): \OpenLegends\Core\Abstract\Card\Rarity |
||||||
|
{ |
||||||
|
return $this->_rarity; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,33 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
abstract class Ability implements \OpenLegends\Core\Interface\Card\Ability |
||||||
|
{ |
||||||
|
private string $_name; |
||||||
|
private string $_description; |
||||||
|
|
||||||
|
abstract public function __construct(); |
||||||
|
|
||||||
|
public function setName(string $value): void |
||||||
|
{ |
||||||
|
$this->_name = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getName(): string |
||||||
|
{ |
||||||
|
return $this->_name; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDescription(string $value): void |
||||||
|
{ |
||||||
|
$this->_description = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDescription(): string |
||||||
|
{ |
||||||
|
return $this->_description; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,20 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
abstract class Action implements \OpenLegends\Core\Interface\Card\Action |
||||||
|
{ |
||||||
|
abstract public function __construct( |
||||||
|
\OpenLegends\Core\Abstract\Card $card |
||||||
|
); |
||||||
|
|
||||||
|
abstract public function card( |
||||||
|
\OpenLegends\Core\Abstract\Card &$card |
||||||
|
): void; |
||||||
|
|
||||||
|
abstract public function player( |
||||||
|
\OpenLegends\Core\Abstract\Player &$player |
||||||
|
): void; |
||||||
|
} |
@ -0,0 +1,31 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
abstract class Attribute implements \OpenLegends\Core\Interface\Card\Attribute |
||||||
|
{ |
||||||
|
private string $_name; |
||||||
|
private string $_description; |
||||||
|
|
||||||
|
public function setName(string $value): void |
||||||
|
{ |
||||||
|
$this->_name = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getName(): string |
||||||
|
{ |
||||||
|
return $this->_name; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDescription(string $value): void |
||||||
|
{ |
||||||
|
$this->_description = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDescription(): string |
||||||
|
{ |
||||||
|
return $this->_description; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,31 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
abstract class Rarity implements \OpenLegends\Core\Interface\Card\Rarity |
||||||
|
{ |
||||||
|
private string $_name; |
||||||
|
private string $_description; |
||||||
|
|
||||||
|
public function setName(string $value): void |
||||||
|
{ |
||||||
|
$this->_name = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getName(): string |
||||||
|
{ |
||||||
|
return $this->_name; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDescription(string $value): void |
||||||
|
{ |
||||||
|
$this->_description = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDescription(): string |
||||||
|
{ |
||||||
|
return $this->_description; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,31 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
abstract class Type implements \OpenLegends\Core\Interface\Card\Type |
||||||
|
{ |
||||||
|
private string $_name; |
||||||
|
private string $_description; |
||||||
|
|
||||||
|
public function setName(string $value): void |
||||||
|
{ |
||||||
|
$this->_name = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getName(): string |
||||||
|
{ |
||||||
|
return $this->_name; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDescription(string $value): void |
||||||
|
{ |
||||||
|
$this->_description = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDescription(): string |
||||||
|
{ |
||||||
|
return $this->_description; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,48 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract; |
||||||
|
|
||||||
|
abstract class Game implements \OpenLegends\Core\Interface\Game |
||||||
|
{ |
||||||
|
private array $_players = []; |
||||||
|
|
||||||
|
private int $_act = 0; |
||||||
|
|
||||||
|
abstract public function __construct(); |
||||||
|
|
||||||
|
abstract public function start(): void; |
||||||
|
|
||||||
|
abstract public function act(): void; |
||||||
|
|
||||||
|
abstract public function end(): void; |
||||||
|
|
||||||
|
public function addPlayer( |
||||||
|
\OpenLegends\Core\Abstract\Game\Player $player |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_players[] = $player; |
||||||
|
} |
||||||
|
|
||||||
|
public function getPlayer( |
||||||
|
int $slot |
||||||
|
): \OpenLegends\Core\Abstract\Game\Player |
||||||
|
{ |
||||||
|
return $this->_players[ |
||||||
|
$slot |
||||||
|
]; |
||||||
|
} |
||||||
|
|
||||||
|
public function setAct( |
||||||
|
int $value |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_act = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getAct(): int |
||||||
|
{ |
||||||
|
return $this->_act; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,105 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\Game; |
||||||
|
|
||||||
|
abstract class Player implements \OpenLegends\Core\Interface\Game\Player |
||||||
|
{ |
||||||
|
private \OpenLegends\Core\Abstract\User $_user; |
||||||
|
|
||||||
|
private \OpenLegends\Core\Abstract\Pool\Card $_deck; |
||||||
|
private \OpenLegends\Core\Abstract\Pool\Card $_hand; |
||||||
|
private \OpenLegends\Core\Abstract\Pool\Card $_pile; |
||||||
|
|
||||||
|
private \OpenLegends\Core\Abstract\Pool\Card $_left; |
||||||
|
private \OpenLegends\Core\Abstract\Pool\Card $_right; |
||||||
|
|
||||||
|
private int $_health; |
||||||
|
|
||||||
|
abstract public function __construct(); |
||||||
|
|
||||||
|
public function setHealth( |
||||||
|
int $value |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_health = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getHealth(): int |
||||||
|
{ |
||||||
|
return $this->_health; |
||||||
|
} |
||||||
|
|
||||||
|
public function getUser(): \OpenLegends\Core\Abstract\User |
||||||
|
{ |
||||||
|
return $this->_user; |
||||||
|
} |
||||||
|
|
||||||
|
public function setUser( |
||||||
|
\OpenLegends\Core\Abstract\User $user |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_user = $user; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDeck(): \OpenLegends\Core\Abstract\Pool\Card |
||||||
|
{ |
||||||
|
return $this->_deck; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDeck( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $deck |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_deck = $deck; |
||||||
|
} |
||||||
|
|
||||||
|
public function getHand(): \OpenLegends\Core\Abstract\Pool\Card |
||||||
|
{ |
||||||
|
return $this->_hand; |
||||||
|
} |
||||||
|
|
||||||
|
public function setHand( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $hand |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_hand = $hand; |
||||||
|
} |
||||||
|
|
||||||
|
public function getPile(): \OpenLegends\Core\Abstract\Pool\Card |
||||||
|
{ |
||||||
|
return $this->_pile; |
||||||
|
} |
||||||
|
|
||||||
|
public function setPile( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $pile |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_pile = $pile; |
||||||
|
} |
||||||
|
|
||||||
|
public function getLeft(): \OpenLegends\Core\Abstract\Pool\Card |
||||||
|
{ |
||||||
|
return $this->_left; |
||||||
|
} |
||||||
|
|
||||||
|
public function setLeft( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $left |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_left = $left; |
||||||
|
} |
||||||
|
|
||||||
|
public function getRight(): \OpenLegends\Core\Abstract\Pool\Card |
||||||
|
{ |
||||||
|
return $this->_right; |
||||||
|
} |
||||||
|
|
||||||
|
public function setRight( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $right |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_right = $right; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,83 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\Pool; |
||||||
|
|
||||||
|
abstract class Card implements \OpenLegends\Core\Interface\Pool\Card |
||||||
|
{ |
||||||
|
private array $_cards = []; |
||||||
|
|
||||||
|
public function getCard( |
||||||
|
int $slot |
||||||
|
): \OpenLegends\Core\Abstract\Card |
||||||
|
{ |
||||||
|
return $this->_cards[ |
||||||
|
$slot |
||||||
|
]; |
||||||
|
} |
||||||
|
|
||||||
|
public function addCard( |
||||||
|
\OpenLegends\Core\Abstract\Card $card |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_cards[] = $card; |
||||||
|
} |
||||||
|
|
||||||
|
public function deleteCard( |
||||||
|
int $slot |
||||||
|
): void |
||||||
|
{ |
||||||
|
unset( |
||||||
|
$this->_cards[ |
||||||
|
$slot |
||||||
|
] |
||||||
|
); |
||||||
|
} |
||||||
|
|
||||||
|
public function getTopCard( |
||||||
|
?int &$slot = null |
||||||
|
): \OpenLegends\Core\Abstract\Card |
||||||
|
{ |
||||||
|
$slot = array_key_first( |
||||||
|
$this->_cards |
||||||
|
); |
||||||
|
|
||||||
|
return $this->_cards[ |
||||||
|
$slot |
||||||
|
]; |
||||||
|
} |
||||||
|
|
||||||
|
public function getBottomCard( |
||||||
|
?int &$slot = null |
||||||
|
): \OpenLegends\Core\Abstract\Card |
||||||
|
{ |
||||||
|
$slot = array_key_last( |
||||||
|
$this->_cards |
||||||
|
); |
||||||
|
|
||||||
|
return $this->_cards[ |
||||||
|
$slot |
||||||
|
]; |
||||||
|
} |
||||||
|
|
||||||
|
public function getRandomCard( |
||||||
|
?int &$slot = null |
||||||
|
): \OpenLegends\Core\Abstract\Card |
||||||
|
{ |
||||||
|
$slot = array_rand( |
||||||
|
$this->_cards |
||||||
|
); |
||||||
|
|
||||||
|
return $this->_cards[ |
||||||
|
$slot |
||||||
|
]; |
||||||
|
} |
||||||
|
|
||||||
|
public function shuffleCards(): void |
||||||
|
{ |
||||||
|
shuffle( |
||||||
|
$this->_cards |
||||||
|
); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,38 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract; |
||||||
|
|
||||||
|
abstract class User implements \OpenLegends\Core\Interface\User |
||||||
|
{ |
||||||
|
abstract public function __construct(); |
||||||
|
|
||||||
|
private \OpenLegends\Core\Abstract\User\Type $_type; |
||||||
|
|
||||||
|
private string $_name; |
||||||
|
|
||||||
|
public function setType( |
||||||
|
\OpenLegends\Core\Abstract\User\Type $type |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_type = $type; |
||||||
|
} |
||||||
|
|
||||||
|
public function getType(): \OpenLegends\Core\Abstract\User\Type |
||||||
|
{ |
||||||
|
return $this->_type; |
||||||
|
} |
||||||
|
|
||||||
|
public function setName( |
||||||
|
string $value |
||||||
|
): void |
||||||
|
{ |
||||||
|
$this->_name = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getName(): string |
||||||
|
{ |
||||||
|
return $this->_name; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,33 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Abstract\User; |
||||||
|
|
||||||
|
abstract class Type implements \OpenLegends\Core\Interface\User\Type |
||||||
|
{ |
||||||
|
private string $_name; |
||||||
|
private string $_description; |
||||||
|
|
||||||
|
abstract public function __construct(); |
||||||
|
|
||||||
|
public function setName(string $value): void |
||||||
|
{ |
||||||
|
$this->_name = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getName(): string |
||||||
|
{ |
||||||
|
return $this->_name; |
||||||
|
} |
||||||
|
|
||||||
|
public function setDescription(string $value): void |
||||||
|
{ |
||||||
|
$this->_description = $value; |
||||||
|
} |
||||||
|
|
||||||
|
public function getDescription(): string |
||||||
|
{ |
||||||
|
return $this->_description; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,98 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface; |
||||||
|
|
||||||
|
interface Card |
||||||
|
{ |
||||||
|
public function __construct(); |
||||||
|
|
||||||
|
public function act( |
||||||
|
\OpenLegends\Core\Abstract\Game &$game |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getBreakthrough(): bool; |
||||||
|
|
||||||
|
public function setBreakthrough(bool $value): void; |
||||||
|
|
||||||
|
public function getCover(): bool; |
||||||
|
|
||||||
|
public function setCover(bool $value): void; |
||||||
|
|
||||||
|
public function getDrain(): bool; |
||||||
|
|
||||||
|
public function setDrain(bool $value): void; |
||||||
|
|
||||||
|
public function getGuard(): bool; |
||||||
|
|
||||||
|
public function setGuard(bool $value): void; |
||||||
|
|
||||||
|
public function getLethal(): bool; |
||||||
|
|
||||||
|
public function setLethal(bool $value): void; |
||||||
|
|
||||||
|
public function getPilfer(): bool; |
||||||
|
|
||||||
|
public function setPilfer(bool $value): void; |
||||||
|
|
||||||
|
public function getProphecy(): bool; |
||||||
|
|
||||||
|
public function setProphecy(bool $value): void; |
||||||
|
|
||||||
|
public function getUnique(): bool; |
||||||
|
|
||||||
|
public function setUnique(bool $value): void; |
||||||
|
|
||||||
|
public function getName(): string; |
||||||
|
|
||||||
|
public function setName(string $value): void; |
||||||
|
|
||||||
|
public function getDescription(): string; |
||||||
|
|
||||||
|
public function setDescription(string $value): void; |
||||||
|
|
||||||
|
public function getCost(): string; |
||||||
|
|
||||||
|
public function setCost(int $value): void; |
||||||
|
|
||||||
|
public function getPower(): int; |
||||||
|
|
||||||
|
public function setPower(int $value): void; |
||||||
|
|
||||||
|
public function getHealth(): int; |
||||||
|
|
||||||
|
public function setHealth(int $value): void; |
||||||
|
|
||||||
|
public function getCraft(): int; |
||||||
|
|
||||||
|
public function setCraft(int $value): void; |
||||||
|
|
||||||
|
public function getExtract(): int; |
||||||
|
|
||||||
|
public function setExtract(int $value): void; |
||||||
|
|
||||||
|
public function setAbility( |
||||||
|
\OpenLegends\Core\Abstract\Card\Ability $ability |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getAbility(): \OpenLegends\Core\Abstract\Card\Ability; |
||||||
|
|
||||||
|
public function setAttribute( |
||||||
|
\OpenLegends\Core\Abstract\Card\Attribute $attribute |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getAttribute(): \OpenLegends\Core\Abstract\Card\Attribute; |
||||||
|
|
||||||
|
public function setType( |
||||||
|
\OpenLegends\Core\Abstract\Card\Type $type |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getType(): \OpenLegends\Core\Abstract\Card\Type; |
||||||
|
|
||||||
|
public function setRarity( |
||||||
|
\OpenLegends\Core\Abstract\Card\Rarity $rarity |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getRarity(): \OpenLegends\Core\Abstract\Card\Rarity; |
||||||
|
} |
@ -0,0 +1,22 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\Card; |
||||||
|
|
||||||
|
interface Ability |
||||||
|
{ |
||||||
|
public function __construct(); |
||||||
|
|
||||||
|
public function setName( |
||||||
|
string $value |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getName(): string; |
||||||
|
|
||||||
|
public function setDescription( |
||||||
|
string $value |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getDescription(): string; |
||||||
|
} |
@ -0,0 +1,20 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\Card; |
||||||
|
|
||||||
|
interface Action |
||||||
|
{ |
||||||
|
public function __construct( |
||||||
|
\OpenLegends\Core\Abstract\Card $card |
||||||
|
); |
||||||
|
|
||||||
|
public function card( |
||||||
|
\OpenLegends\Core\Abstract\Card &$card |
||||||
|
): void; |
||||||
|
|
||||||
|
public function player( |
||||||
|
\OpenLegends\Core\Abstract\Player &$player |
||||||
|
): void; |
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\Card; |
||||||
|
|
||||||
|
interface Attribute |
||||||
|
{ |
||||||
|
public function setName(string $value): void; |
||||||
|
|
||||||
|
public function getName(): string; |
||||||
|
|
||||||
|
public function setDescription(string $value): void; |
||||||
|
|
||||||
|
public function getDescription(): string; |
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\Card; |
||||||
|
|
||||||
|
interface Rarity |
||||||
|
{ |
||||||
|
public function setName(string $value): void; |
||||||
|
|
||||||
|
public function getName(): string; |
||||||
|
|
||||||
|
public function setDescription(string $value): void; |
||||||
|
|
||||||
|
public function getDescription(): string; |
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\Card; |
||||||
|
|
||||||
|
interface Type |
||||||
|
{ |
||||||
|
public function setName(string $value): void; |
||||||
|
|
||||||
|
public function getName(): string; |
||||||
|
|
||||||
|
public function setDescription(string $value): void; |
||||||
|
|
||||||
|
public function getDescription(): string; |
||||||
|
} |
@ -0,0 +1,30 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface; |
||||||
|
|
||||||
|
interface Game |
||||||
|
{ |
||||||
|
public function __construct(); |
||||||
|
|
||||||
|
public function start(): void; |
||||||
|
|
||||||
|
public function act(): void; |
||||||
|
|
||||||
|
public function end(): void; |
||||||
|
|
||||||
|
public function addPlayer( |
||||||
|
\OpenLegends\Core\Abstract\Game\Player $player |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getPlayer( |
||||||
|
int $slot |
||||||
|
): \OpenLegends\Core\Abstract\Game\Player; |
||||||
|
|
||||||
|
public function setAct( |
||||||
|
int $value |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getAct(): int; |
||||||
|
} |
@ -0,0 +1,52 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\Game; |
||||||
|
|
||||||
|
interface Player |
||||||
|
{ |
||||||
|
public function __construct(); |
||||||
|
|
||||||
|
public function setHealth( |
||||||
|
int $value |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getHealth(): int; |
||||||
|
|
||||||
|
public function getUser(): \OpenLegends\Core\Abstract\User; |
||||||
|
|
||||||
|
public function setUser( |
||||||
|
\OpenLegends\Core\Abstract\User $user |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getDeck(): \OpenLegends\Core\Abstract\Pool\Card; |
||||||
|
|
||||||
|
public function setDeck( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $deck |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getHand(): \OpenLegends\Core\Abstract\Pool\Card; |
||||||
|
|
||||||
|
public function setHand( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $hand |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getPile(): \OpenLegends\Core\Abstract\Pool\Card; |
||||||
|
|
||||||
|
public function setPile( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $pile |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getLeft(): \OpenLegends\Core\Abstract\Pool\Card; |
||||||
|
|
||||||
|
public function setLeft( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $left |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getRight(): \OpenLegends\Core\Abstract\Pool\Card; |
||||||
|
|
||||||
|
public function setRight( |
||||||
|
\OpenLegends\Core\Abstract\Pool\Card $right |
||||||
|
): void; |
||||||
|
} |
@ -0,0 +1,34 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\Pool; |
||||||
|
|
||||||
|
interface Card |
||||||
|
{ |
||||||
|
public function getCard( |
||||||
|
int $slot |
||||||
|
): \OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
public function addCard( |
||||||
|
\OpenLegends\Core\Abstract\Card $card |
||||||
|
): void; |
||||||
|
|
||||||
|
public function deleteCard( |
||||||
|
int $slot |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getTopCard( |
||||||
|
?int &$slot = null |
||||||
|
): \OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
public function getBottomCard( |
||||||
|
?int &$slot = null |
||||||
|
): \OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
public function getRandomCard( |
||||||
|
?int &$slot = null |
||||||
|
): \OpenLegends\Core\Abstract\Card; |
||||||
|
|
||||||
|
public function shuffleCards(): void; |
||||||
|
} |
@ -0,0 +1,22 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface; |
||||||
|
|
||||||
|
interface User |
||||||
|
{ |
||||||
|
public function __construct(); |
||||||
|
|
||||||
|
public function setType( |
||||||
|
\OpenLegends\Core\Abstract\User\Type $type |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getType(): \OpenLegends\Core\Abstract\User\Type; |
||||||
|
|
||||||
|
public function setName( |
||||||
|
string $value |
||||||
|
): void; |
||||||
|
|
||||||
|
public function getName(): string; |
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
declare(strict_types=1); |
||||||
|
|
||||||
|
namespace OpenLegends\Core\Interface\User; |
||||||
|
|
||||||
|
interface Type |
||||||
|
{ |
||||||
|
public function setName(string $value): void; |
||||||
|
|
||||||
|
public function getName(): string; |
||||||
|
|
||||||
|
public function setDescription(string $value): void; |
||||||
|
|
||||||
|
public function getDescription(): string; |
||||||
|
} |
Loading…
Reference in new issue