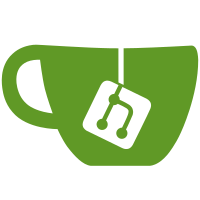
11 changed files with 278 additions and 47 deletions
@ -0,0 +1,18 @@
@@ -0,0 +1,18 @@
|
||||
package com.github.apognu.otter.repositories |
||||
|
||||
import android.content.Context |
||||
import com.github.apognu.otter.utils.FunkwhaleResponse |
||||
import com.github.apognu.otter.utils.Track |
||||
import com.github.apognu.otter.utils.TracksCache |
||||
import com.github.apognu.otter.utils.TracksResponse |
||||
import com.github.kittinunf.fuel.gson.gsonDeserializerOf |
||||
import com.google.gson.reflect.TypeToken |
||||
import java.io.BufferedReader |
||||
|
||||
class ArtistTracksRepository(override val context: Context?, private val artistId: Int) : Repository<Track, TracksCache>() { |
||||
override val cacheId = "tracks-artist-${artistId}" |
||||
override val upstream = HttpUpstream<Track, FunkwhaleResponse<Track>>(HttpUpstream.Behavior.AtOnce, "/api/v1/tracks/?playable=true&artist=${artistId}", object : TypeToken<TracksResponse>() {}.type) |
||||
|
||||
override fun cache(data: List<Track>) = TracksCache(data) |
||||
override fun uncache(reader: BufferedReader) = gsonDeserializerOf(TracksCache::class.java).deserialize(reader) |
||||
} |
@ -0,0 +1,59 @@
@@ -0,0 +1,59 @@
|
||||
package com.github.apognu.otter.views |
||||
|
||||
import android.animation.ObjectAnimator |
||||
import android.content.Context |
||||
import android.graphics.drawable.Drawable |
||||
import android.view.View |
||||
import android.widget.ImageView |
||||
import com.github.apognu.otter.R |
||||
import com.google.android.material.floatingactionbutton.ExtendedFloatingActionButton |
||||
|
||||
object LoadingFlotingActionButton { |
||||
fun start(button: ExtendedFloatingActionButton): ObjectAnimator { |
||||
button.isEnabled = false |
||||
button.setIconResource(R.drawable.fab_spinner) |
||||
button.shrink() |
||||
|
||||
return ObjectAnimator.ofFloat(button, View.ROTATION, 0f, 360f).apply { |
||||
duration = 500 |
||||
repeatCount = ObjectAnimator.INFINITE |
||||
start() |
||||
} |
||||
} |
||||
|
||||
fun stop(button: ExtendedFloatingActionButton, animator: ObjectAnimator) { |
||||
animator.cancel() |
||||
|
||||
button.isEnabled = true |
||||
button.setIconResource(R.drawable.play) |
||||
button.rotation = 0.0f |
||||
button.extend() |
||||
} |
||||
} |
||||
|
||||
object LoadingImageView { |
||||
fun start(context: Context?, image: ImageView): ObjectAnimator? { |
||||
context?.let { |
||||
image.isEnabled = false |
||||
image.setImageDrawable(context.getDrawable(R.drawable.fab_spinner)) |
||||
|
||||
return ObjectAnimator.ofFloat(image, View.ROTATION, 0f, 360f).apply { |
||||
duration = 500 |
||||
repeatCount = ObjectAnimator.INFINITE |
||||
start() |
||||
} |
||||
} |
||||
|
||||
return null |
||||
} |
||||
|
||||
fun stop(context: Context?, original: Drawable, image: ImageView, animator: ObjectAnimator?) { |
||||
context?.let { |
||||
animator?.cancel() |
||||
|
||||
image.isEnabled = true |
||||
image.setImageDrawable(original) |
||||
image.rotation = 0.0f |
||||
} |
||||
} |
||||
} |
@ -0,0 +1,30 @@
@@ -0,0 +1,30 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?> |
||||
<layer-list xmlns:android="http://schemas.android.com/apk/res/android"> |
||||
|
||||
<item android:id="@android:id/background"> |
||||
<rotate |
||||
android:fromDegrees="0" |
||||
android:pivotX="50%" |
||||
android:pivotY="50%" |
||||
android:toDegrees="360"> |
||||
|
||||
<shape |
||||
android:shape="ring" |
||||
android:thickness="3dp" |
||||
android:type="sweep" |
||||
android:useLevel="false"> |
||||
|
||||
<gradient |
||||
android:angle="0" |
||||
android:centerColor="#00ffffff" |
||||
android:endColor="#00ffffff" |
||||
android:startColor="#ffffffff" |
||||
android:type="sweep" |
||||
android:useLevel="false" /> |
||||
|
||||
</shape> |
||||
|
||||
</rotate> |
||||
</item> |
||||
|
||||
</layer-list> |
Loading…
Reference in new issue