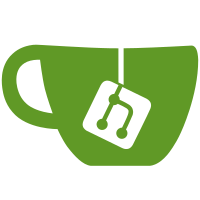
3 changed files with 200 additions and 1 deletions
@ -0,0 +1,35 @@
@@ -0,0 +1,35 @@
|
||||
/* |
||||
* Copyright (c) 2024 New Vector Ltd |
||||
* |
||||
* Licensed under the Apache License, Version 2.0 (the "License"); |
||||
* you may not use this file except in compliance with the License. |
||||
* You may obtain a copy of the License at |
||||
* |
||||
* http://www.apache.org/licenses/LICENSE-2.0 |
||||
* |
||||
* Unless required by applicable law or agreed to in writing, software |
||||
* distributed under the License is distributed on an "AS IS" BASIS, |
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
||||
* See the License for the specific language governing permissions and |
||||
* limitations under the License. |
||||
*/ |
||||
|
||||
package io.element.android.features.share.impl |
||||
|
||||
import android.content.Intent |
||||
|
||||
class FakeShareIntentHandler( |
||||
private val onIncomingShareIntent: suspend ( |
||||
Intent, |
||||
suspend (List<DefaultShareIntentHandler.FileToShare>) -> Boolean, |
||||
suspend (String) -> Boolean, |
||||
) -> Boolean = { _, _, _ -> false }, |
||||
) : ShareIntentHandler { |
||||
override suspend fun handleIncomingShareIntent( |
||||
intent: Intent, |
||||
onFile: suspend (List<DefaultShareIntentHandler.FileToShare>) -> Boolean, |
||||
onPlainText: suspend (String) -> Boolean, |
||||
): Boolean { |
||||
return onIncomingShareIntent(intent, onFile, onPlainText) |
||||
} |
||||
} |
@ -0,0 +1,164 @@
@@ -0,0 +1,164 @@
|
||||
/* |
||||
* Copyright (c) 2024 New Vector Ltd |
||||
* |
||||
* Licensed under the Apache License, Version 2.0 (the "License"); |
||||
* you may not use this file except in compliance with the License. |
||||
* You may obtain a copy of the License at |
||||
* |
||||
* http://www.apache.org/licenses/LICENSE-2.0 |
||||
* |
||||
* Unless required by applicable law or agreed to in writing, software |
||||
* distributed under the License is distributed on an "AS IS" BASIS, |
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
||||
* See the License for the specific language governing permissions and |
||||
* limitations under the License. |
||||
*/ |
||||
|
||||
package io.element.android.features.share.impl |
||||
|
||||
import android.content.Intent |
||||
import android.net.Uri |
||||
import app.cash.molecule.RecompositionMode |
||||
import app.cash.molecule.moleculeFlow |
||||
import app.cash.turbine.test |
||||
import com.google.common.truth.Truth.assertThat |
||||
import io.element.android.libraries.architecture.AsyncAction |
||||
import io.element.android.libraries.core.mimetype.MimeTypes |
||||
import io.element.android.libraries.matrix.api.MatrixClient |
||||
import io.element.android.libraries.matrix.test.A_MESSAGE |
||||
import io.element.android.libraries.matrix.test.A_ROOM_ID |
||||
import io.element.android.libraries.matrix.test.FakeMatrixClient |
||||
import io.element.android.libraries.matrix.test.room.FakeMatrixRoom |
||||
import io.element.android.libraries.mediaupload.api.MediaPreProcessor |
||||
import io.element.android.libraries.mediaupload.test.FakeMediaPreProcessor |
||||
import io.element.android.tests.testutils.WarmUpRule |
||||
import kotlinx.coroutines.test.TestScope |
||||
import kotlinx.coroutines.test.runTest |
||||
import org.junit.Rule |
||||
import org.junit.Test |
||||
import org.junit.runner.RunWith |
||||
import org.robolectric.RobolectricTestRunner |
||||
|
||||
@RunWith(RobolectricTestRunner::class) |
||||
class SharePresenterTest { |
||||
@get:Rule |
||||
val warmUpRule = WarmUpRule() |
||||
|
||||
@Test |
||||
fun `present - initial state`() = runTest { |
||||
val presenter = createSharePresenter() |
||||
moleculeFlow(RecompositionMode.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.shareAction.isUninitialized()).isTrue() |
||||
} |
||||
} |
||||
|
||||
@Test |
||||
fun `present - on room selected error then clear error`() = runTest { |
||||
val presenter = createSharePresenter() |
||||
moleculeFlow(RecompositionMode.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.shareAction.isUninitialized()).isTrue() |
||||
presenter.onRoomSelected(listOf(A_ROOM_ID)) |
||||
assertThat(awaitItem().shareAction.isLoading()).isTrue() |
||||
val failure = awaitItem() |
||||
assertThat(failure.shareAction.isFailure()).isTrue() |
||||
failure.eventSink.invoke(ShareEvents.ClearError) |
||||
assertThat(awaitItem().shareAction.isUninitialized()).isTrue() |
||||
} |
||||
} |
||||
|
||||
@Test |
||||
fun `present - on room selected ok`() = runTest { |
||||
val presenter = createSharePresenter( |
||||
shareIntentHandler = FakeShareIntentHandler { _, _, _ -> true } |
||||
) |
||||
moleculeFlow(RecompositionMode.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.shareAction.isUninitialized()).isTrue() |
||||
presenter.onRoomSelected(listOf(A_ROOM_ID)) |
||||
assertThat(awaitItem().shareAction.isLoading()).isTrue() |
||||
val success = awaitItem() |
||||
assertThat(success.shareAction.isSuccess()).isTrue() |
||||
assertThat(success.shareAction).isEqualTo(AsyncAction.Success(listOf(A_ROOM_ID))) |
||||
} |
||||
} |
||||
|
||||
@Test |
||||
fun `present - send text ok`() = runTest { |
||||
val matrixRoom = FakeMatrixRoom() |
||||
val matrixClient = FakeMatrixClient().apply { |
||||
givenGetRoomResult(A_ROOM_ID, matrixRoom) |
||||
} |
||||
val presenter = createSharePresenter( |
||||
matrixClient = matrixClient, |
||||
shareIntentHandler = FakeShareIntentHandler { _, _, onText -> |
||||
onText(A_MESSAGE) |
||||
} |
||||
) |
||||
moleculeFlow(RecompositionMode.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.shareAction.isUninitialized()).isTrue() |
||||
presenter.onRoomSelected(listOf(A_ROOM_ID)) |
||||
assertThat(awaitItem().shareAction.isLoading()).isTrue() |
||||
val success = awaitItem() |
||||
assertThat(success.shareAction.isSuccess()).isTrue() |
||||
assertThat(success.shareAction).isEqualTo(AsyncAction.Success(listOf(A_ROOM_ID))) |
||||
} |
||||
} |
||||
|
||||
@Test |
||||
fun `present - send media ok`() = runTest { |
||||
val matrixRoom = FakeMatrixRoom() |
||||
val matrixClient = FakeMatrixClient().apply { |
||||
givenGetRoomResult(A_ROOM_ID, matrixRoom) |
||||
} |
||||
val presenter = createSharePresenter( |
||||
matrixClient = matrixClient, |
||||
shareIntentHandler = FakeShareIntentHandler { _, onFile, _ -> |
||||
onFile( |
||||
listOf( |
||||
DefaultShareIntentHandler.FileToShare( |
||||
uri = Uri.parse("content://image.jpg"), |
||||
mimeType = MimeTypes.Jpeg, |
||||
) |
||||
) |
||||
) |
||||
} |
||||
) |
||||
moleculeFlow(RecompositionMode.Immediate) { |
||||
presenter.present() |
||||
}.test { |
||||
val initialState = awaitItem() |
||||
assertThat(initialState.shareAction.isUninitialized()).isTrue() |
||||
presenter.onRoomSelected(listOf(A_ROOM_ID)) |
||||
assertThat(awaitItem().shareAction.isLoading()).isTrue() |
||||
val success = awaitItem() |
||||
assertThat(success.shareAction.isSuccess()).isTrue() |
||||
assertThat(success.shareAction).isEqualTo(AsyncAction.Success(listOf(A_ROOM_ID))) |
||||
} |
||||
} |
||||
|
||||
private fun TestScope.createSharePresenter( |
||||
intent: Intent = Intent(), |
||||
shareIntentHandler: ShareIntentHandler = FakeShareIntentHandler(), |
||||
matrixClient: MatrixClient = FakeMatrixClient(), |
||||
mediaPreProcessor: MediaPreProcessor = FakeMediaPreProcessor() |
||||
): SharePresenter { |
||||
return SharePresenter( |
||||
intent = intent, |
||||
appCoroutineScope = this, |
||||
shareIntentHandler = shareIntentHandler, |
||||
matrixClient = matrixClient, |
||||
mediaPreProcessor = mediaPreProcessor |
||||
) |
||||
} |
||||
} |
Loading…
Reference in new issue