Browse Source
Allows [VoiceMessagePresenter] instances to keep their progress and download states while going in and out of the timeline viewport. This is implemented by caching each instance of a TimelineItem presenter inside the RoomScope. TimelineItem presenters can move some of their state outside of the `present()` function so that such state will survive scrollings of the timeline.pull/1803/head
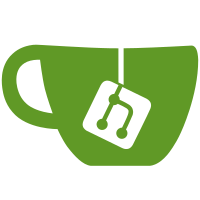
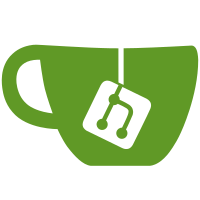
4 changed files with 87 additions and 31 deletions
@ -0,0 +1,26 @@
@@ -0,0 +1,26 @@
|
||||
/* |
||||
* Copyright (c) 2023 New Vector Ltd |
||||
* |
||||
* Licensed under the Apache License, Version 2.0 (the "License"); |
||||
* you may not use this file except in compliance with the License. |
||||
* You may obtain a copy of the License at |
||||
* |
||||
* http://www.apache.org/licenses/LICENSE-2.0 |
||||
* |
||||
* Unless required by applicable law or agreed to in writing, software |
||||
* distributed under the License is distributed on an "AS IS" BASIS, |
||||
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. |
||||
* See the License for the specific language governing permissions and |
||||
* limitations under the License. |
||||
*/ |
||||
|
||||
package io.element.android.features.messages.impl.timeline.di |
||||
|
||||
import androidx.compose.runtime.staticCompositionLocalOf |
||||
|
||||
/** |
||||
* Provides a [TimelineItemPresenterFactories] to the composition. |
||||
*/ |
||||
val LocalTimelineItemPresenterFactories = staticCompositionLocalOf { |
||||
TimelineItemPresenterFactories(emptyMap()) |
||||
} |
Loading…
Reference in new issue