Browse Source
<!-- Please read [CONTRIBUTING.md](https://github.com/vector-im/element-x-android/blob/develop/CONTRIBUTING.md) before submitting your pull request --> ## Type of change - [ ] Feature - [x] Bugfix - [ ] Technical - [ ] Other : ## Content Code now checks whether the mx uri is invalid and handles the error condition properly. ## Motivation and context https://github.com/vector-im/element-x-android/pull/1748#discussion_r1384557443 ## Screenshots / GIFs <!-- We have screenshot tests in the project, so attaching screenshots to a PR is not mandatory, as far as there is a Composable Preview covering the changes. In this case, the change will appear in the file diff. Note that all the UI composables should be covered by a Composable Preview. Providing a video of the change is still very useful for the reviewer and for the history of the project. You can use a table like this to show screenshots comparison. Uncomment this markdown table below and edit the last line `|||`: |copy screenshot of before here|copy screenshot of after here| |Before|After| |-|-| ||| --> ## Tests <!-- Explain how you tested your development --> - Step 1 - Step 2 - Step ... ## Tested devices - [ ] Physical - [ ] Emulator - OS version(s): ## Checklist <!-- Depending on the Pull Request content, it can be acceptable if some of the following checkboxes stay unchecked. --> - [ ] Changes have been tested on an Android device or Android emulator with API 23 - [ ] UI change has been tested on both light and dark themes - [ ] Accessibility has been taken into account. See https://github.com/vector-im/element-x-android/blob/develop/CONTRIBUTING.md#accessibility - [ ] Pull request is based on the develop branch - [ ] Pull request includes a new file under ./changelog.d. See https://github.com/vector-im/element-x-android/blob/develop/CONTRIBUTING.md#changelog - [ ] Pull request includes screenshots or videos if containing UI changes - [ ] Pull request includes a [sign off](https://matrix-org.github.io/synapse/latest/development/contributing_guide.html#sign-off) - [ ] You've made a self review of your PRpull/1766/head
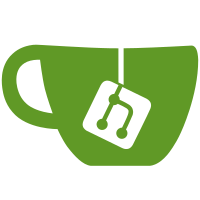
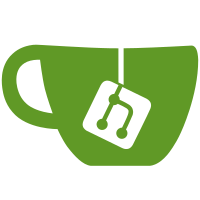
2 changed files with 29 additions and 15 deletions
Loading…
Reference in new issue