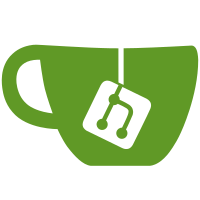
3 changed files with 0 additions and 360 deletions
@ -1,47 +0,0 @@
@@ -1,47 +0,0 @@
|
||||
# kvazar-network webapp |
||||
Web-oriented content exploring platform for Kevacoin Blockchain |
||||
|
||||
### requirements |
||||
``` |
||||
php-7.4 |
||||
php-curl |
||||
php-mbstring |
||||
php-mysql |
||||
php-pdo |
||||
php-bcmath |
||||
php-gd |
||||
``` |
||||
#### database |
||||
|
||||
https://github.com/kvazar-network/database |
||||
|
||||
##### MySQL |
||||
|
||||
https://github.com/kvazar-network/webapp/tree/master |
||||
|
||||
##### SQLite |
||||
|
||||
https://github.com/kvazar-network/webapp/tree/sqlite |
||||
|
||||
#### crontab |
||||
|
||||
``` |
||||
0 0 * * * /path-to/php /path-to/crontab/sitemap.php > /dev/null 2>&1 |
||||
``` |
||||
|
||||
### nginx sef_mode example |
||||
|
||||
``` |
||||
location / { |
||||
try_files $uri $uri/ =404 @sef; |
||||
} |
||||
|
||||
location @sef { |
||||
rewrite ^(/.*)$ /?$1 last; |
||||
} |
||||
``` |
||||
|
||||
### examples |
||||
|
||||
#### yggdrasil |
||||
[http://[203:9fd0:95df:54d7:29db:5ee1:fe2d:95c7]](http://[203:9fd0:95df:54d7:29db:5ee1:fe2d:95c7]) |
@ -1,183 +0,0 @@
@@ -1,183 +0,0 @@
|
||||
<?php |
||||
|
||||
class MySQL { |
||||
|
||||
private _$db; |
||||
|
||||
public function __construct() { |
||||
|
||||
try { |
||||
|
||||
$this->_db = new PDO('mysql:dbname=' . DB_NAME . ';host=' . DB_HOST . ';port=' . DB_PORT . ';charset=utf8', DB_USERNAME, DB_PASSWORD, [PDO::MYSQL_ATTR_INIT_COMMAND => 'SET NAMES utf8']); |
||||
$this->_db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); |
||||
$this->_db->setAttribute(PDO::ATTR_DEFAULT_FETCH_MODE, PDO::FETCH_ASSOC); |
||||
$this->_db->setAttribute(PDO::ATTR_TIMEOUT, 600); |
||||
|
||||
} catch(PDOException $e) { |
||||
trigger_error($e->getMessage()); |
||||
} |
||||
} |
||||
|
||||
public function getNamespaceValueByNS($ns) { |
||||
|
||||
try { |
||||
|
||||
$query = $this->_db->prepare('SELECT `data`.`value` AS `value` |
||||
|
||||
FROM `data` |
||||
JOIN `namespace` ON (`namespace`.`nameSpaceId` = `data`.`nameSpaceId`) |
||||
|
||||
WHERE `namespace`.`hash` = ? |
||||
AND `data`.`ns` = "1" |
||||
-- AND `data`.`deleted` = "0" -- |
||||
|
||||
ORDER BY `data`.`blockId` DESC |
||||
|
||||
LIMIT 1'); |
||||
|
||||
$query->execute([$ns]); |
||||
|
||||
return $query->rowCount() ? $query->fetch()['value'] : []; |
||||
|
||||
} catch(PDOException $e) { |
||||
|
||||
trigger_error($e->getMessage()); |
||||
return false; |
||||
} |
||||
} |
||||
|
||||
public function getNamespaceHashByTX($txid) { |
||||
|
||||
try { |
||||
|
||||
$query = $this->_db->prepare('SELECT `namespace`.`hash` |
||||
|
||||
FROM `namespace` |
||||
JOIN `data` ON (`data`.`nameSpaceId` = `namespace`.`nameSpaceId`) |
||||
|
||||
WHERE `data`.`txid` = ?'); |
||||
|
||||
$query->execute([$txid]); |
||||
|
||||
return $query->rowCount() ? $query->fetch()['hash'] : []; |
||||
|
||||
} catch(PDOException $e) { |
||||
|
||||
trigger_error($e->getMessage()); |
||||
return false; |
||||
} |
||||
} |
||||
|
||||
public function getData($namehash = false, $txid = false, $search = false, $start = 0, $limit = 10) { |
||||
|
||||
try { |
||||
|
||||
if ($txid) { |
||||
|
||||
$query = $this->_db->prepare('SELECT `block`.`blockId` AS `block`, |
||||
`namespace`.`hash` AS `namehash`, |
||||
`data`.`time` AS `time`, |
||||
`data`.`key` AS `key`, |
||||
`data`.`value` AS `value`, |
||||
`data`.`txid` AS `txid` |
||||
|
||||
FROM `data` |
||||
JOIN `block` ON (`block`.`blockId` = `data`.`blockId`) |
||||
JOIN `namespace` ON (`namespace`.`nameSpaceId` = `data`.`nameSpaceId`) |
||||
|
||||
WHERE `data`.`txid` = ? |
||||
AND `data`.`ns` = "0" |
||||
-- AND `data`.`deleted` = "0" -- |
||||
|
||||
ORDER BY `block`.`blockId` DESC |
||||
|
||||
LIMIT ' . (int) $start . ',' . (int) $limit); |
||||
|
||||
$query->execute([$txid]); |
||||
|
||||
} else if ($namehash) { |
||||
|
||||
$query = $this->_db->prepare('SELECT `block`.`blockId` AS `block`, |
||||
`namespace`.`hash` AS `namehash`, |
||||
`data`.`time` AS `time`, |
||||
`data`.`key` AS `key`, |
||||
`data`.`value` AS `value`, |
||||
`data`.`txid` AS `txid` |
||||
|
||||
FROM `data` |
||||
JOIN `block` ON (`block`.`blockId` = `data`.`blockId`) |
||||
JOIN `namespace` ON (`namespace`.`nameSpaceId` = `data`.`nameSpaceId`) |
||||
|
||||
WHERE `namespace`.`hash` = ? |
||||
AND `data`.`ns` = "0" |
||||
-- AND `data`.`deleted` = "0" -- |
||||
|
||||
ORDER BY `block`.`blockId` DESC |
||||
|
||||
LIMIT ' . (int) $start . ',' . (int) $limit); |
||||
|
||||
$query->execute([$namehash]); |
||||
|
||||
} else if ($search) { |
||||
|
||||
$query = $this->_db->prepare('SELECT `block`.`blockId` AS `block`, |
||||
`namespace`.`hash` AS `namehash`, |
||||
`data`.`time` AS `time`, |
||||
`data`.`key` AS `key`, |
||||
`data`.`value` AS `value`, |
||||
`data`.`txid` AS `txid` |
||||
|
||||
FROM `data` |
||||
JOIN `block` ON (`block`.`blockId` = `data`.`blockId`) |
||||
JOIN `namespace` ON (`namespace`.`nameSpaceId` = `data`.`nameSpaceId`) |
||||
|
||||
WHERE (`data`.`key` LIKE :search |
||||
OR `data`.`value` LIKE :search |
||||
OR `block`.`blockId` LIKE :search |
||||
OR `namespace`.`hash` LIKE :search |
||||
OR `data`.`txid` LIKE :search) |
||||
|
||||
AND `data`.`ns` = "0" |
||||
-- AND `data`.`deleted` = "0" -- |
||||
|
||||
ORDER BY `block`.`blockId` DESC |
||||
|
||||
LIMIT ' . (int) $start . ',' . (int) $limit); |
||||
|
||||
$query->bindValue(':search', '%' . $search . '%', PDO::PARAM_STR); |
||||
|
||||
$query->execute(); |
||||
|
||||
} else { |
||||
|
||||
$query = $this->_db->prepare('SELECT `block`.`blockId` AS `block`, |
||||
`namespace`.`hash` AS `namehash`, |
||||
`data`.`time` AS `time`, |
||||
`data`.`key` AS `key`, |
||||
`data`.`value` AS `value`, |
||||
`data`.`txid` AS `txid` |
||||
|
||||
FROM `data` |
||||
JOIN `block` ON (`block`.`blockId` = `data`.`blockId`) |
||||
JOIN `namespace` ON (`namespace`.`nameSpaceId` = `data`.`nameSpaceId`) |
||||
|
||||
WHERE `data`.`ns` = "0" |
||||
-- AND `data`.`deleted` = "0" -- |
||||
|
||||
ORDER BY `block`.`blockId` DESC |
||||
|
||||
LIMIT ' . (int) $start . ',' . (int) $limit); |
||||
|
||||
$query->execute(); |
||||
} |
||||
|
||||
|
||||
return $query->rowCount() ? $query->fetchAll() : []; |
||||
|
||||
} catch(PDOException $e) { |
||||
|
||||
trigger_error($e->getMessage()); |
||||
return false; |
||||
} |
||||
} |
||||
} |
@ -1,130 +0,0 @@
@@ -1,130 +0,0 @@
|
||||
<?php |
||||
|
||||
require_once('../config.php'); |
||||
require_once('../library/icon.php'); |
||||
require_once('../library/mysql.php'); |
||||
|
||||
$query = isset($_GET['q']) ? preg_replace('/[\W\D\S]+/', '', $_GET['q']) : false; |
||||
$ns = isset($_GET['ns']) ? preg_replace('/[^a-zA-Z0-9]+/', '', $_GET['ns']) : false; |
||||
$tx = isset($_GET['tx']) ? preg_replace('/[^a-zA-Z0-9]+/', '', $_GET['tx']) : false; |
||||
$page = (int) isset($_GET['page']) ? $_GET['page'] : 0; |
||||
|
||||
if (SEF_MODE && isset($_SERVER['QUERY_STRING'])) { |
||||
|
||||
$q = explode('/', $_SERVER['QUERY_STRING']); |
||||
|
||||
if (isset($q[1])) { |
||||
if (strlen($q[1]) == 34) { |
||||
$ns = preg_replace('/[^a-zA-Z0-9]+/', '', $q[1]); |
||||
} else if (strlen($q[1]) > 34) { |
||||
$tx = preg_replace('/[^a-zA-Z0-9]+/', '', $q[1]); |
||||
} else { |
||||
$page = (int) $q[1]; |
||||
} |
||||
} |
||||
|
||||
if (isset($q[2])) { |
||||
if (strlen($q[2]) == 34) { |
||||
$ns = preg_replace('/[^a-zA-Z0-9]+/', '', $q[2]); |
||||
} else { |
||||
$page = (int) $q[2]; |
||||
} |
||||
} |
||||
} |
||||
|
||||
if ($page > 0) { |
||||
$limit = PAGE_LIMIT * $page - PAGE_LIMIT; |
||||
} else { |
||||
$limit = PAGE_LIMIT * $page; |
||||
} |
||||
|
||||
$db = new MySQL(); |
||||
|
||||
if ($ns) { |
||||
|
||||
$namespaceHash = $ns; |
||||
$namespaceValue = $db->getNamespaceValueByNS($ns); |
||||
|
||||
} else if ($tx) { |
||||
|
||||
$namespaceHash = $db->getNamespaceHashByTX($tx); |
||||
$namespaceValue = $db->getNamespaceValueByNS($namespaceHash); |
||||
|
||||
} else { |
||||
|
||||
$namespaceHash = false; |
||||
$namespaceValue = false; |
||||
} |
||||
|
||||
$data = []; |
||||
foreach ($db->getData($ns, $tx, $query, $limit, PAGE_LIMIT) as $value) { |
||||
$data[] = [ |
||||
'namehash' => $value['namehash'], |
||||
'block' => $value['block'], |
||||
'txid' => $value['txid'], |
||||
'time' => date('d-m-Y H:i', $value['time']), |
||||
'key' => nl2br(trim($value['key'])), |
||||
'value' => nl2br(trim($value['value'])), |
||||
]; |
||||
} |
||||
|
||||
if (SEF_MODE) { |
||||
|
||||
if (in_array($page, [0, 1])) { |
||||
$newer = false; |
||||
} else { |
||||
if ($page == 2) { |
||||
$newer = ($ns ? $ns : ''); |
||||
} else { |
||||
$newer = ($ns ? $ns . '/' . ($page - 1) : ($page - 1)); |
||||
} |
||||
} |
||||
|
||||
if ($data) { |
||||
if (in_array($page, [0, 1])) { |
||||
$older = ($ns ? $ns . '/2' : '/2'); |
||||
} else { |
||||
$older = ($ns ? $ns . '/' . ($page + 1) : '/' . ($page + 1)); |
||||
} |
||||
} else { |
||||
$older = false; |
||||
} |
||||
|
||||
} else { |
||||
|
||||
if (in_array($page, [0, 1])) { |
||||
$newer = false; |
||||
} else { |
||||
if ($page == 2) { |
||||
$newer = ($ns ? '?ns=' . $ns : ($query ? '?q=' . $query : '')); |
||||
} else { |
||||
$newer = ($ns ? '?ns=' . $ns . '&page=' . ($page - 1) : '?page=' . ($page - 1) . ($query ? '&q=' . $query : '')); |
||||
} |
||||
} |
||||
|
||||
if ($data) { |
||||
if (in_array($page, [0, 1])) { |
||||
$older = ($ns ? '?ns=' . $ns . '&page=2' : '?page=2' . ($query ? '&q=' . $query : '')); |
||||
} else { |
||||
$older = ($ns ? '?ns=' . $ns . '&page=' . ($page + 1) : '?page=' . ($page + 1) . ($query ? '&q=' . $query : '')); |
||||
} |
||||
} else { |
||||
$older = false; |
||||
} |
||||
} |
||||
|
||||
if ($ns) { |
||||
if ($page) { |
||||
$hrefThisPage = $ns . '/' . $page; |
||||
} else { |
||||
$hrefThisPage = $ns; |
||||
} |
||||
} else { |
||||
if ($page) { |
||||
$hrefThisPage = $page; |
||||
} else { |
||||
$hrefThisPage = ''; |
||||
} |
||||
} |
||||
|
||||
require_once('index.phtml'); |
Loading…
Reference in new issue