Browse Source
Add "bip125-replaceable" output field to listtransactions and gettransaction which indicates if an unconfirmed transaction, or any unconfirmed parent, is signaling opt-in RBF according to BIP 125.0.13
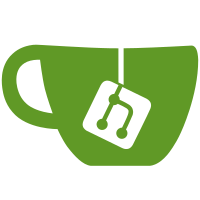
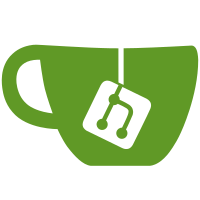
5 changed files with 199 additions and 0 deletions
@ -0,0 +1,46 @@ |
|||||||
|
// Copyright (c) 2016 The Bitcoin developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#include "policy/rbf.h" |
||||||
|
|
||||||
|
bool SignalsOptInRBF(const CTransaction &tx) |
||||||
|
{ |
||||||
|
BOOST_FOREACH(const CTxIn &txin, tx.vin) { |
||||||
|
if (txin.nSequence < std::numeric_limits<unsigned int>::max()-1) { |
||||||
|
return true; |
||||||
|
} |
||||||
|
} |
||||||
|
return false; |
||||||
|
} |
||||||
|
|
||||||
|
bool IsRBFOptIn(const CTxMemPoolEntry &entry, CTxMemPool &pool) |
||||||
|
{ |
||||||
|
AssertLockHeld(pool.cs); |
||||||
|
|
||||||
|
CTxMemPool::setEntries setAncestors; |
||||||
|
|
||||||
|
// First check the transaction itself.
|
||||||
|
if (SignalsOptInRBF(entry.GetTx())) { |
||||||
|
return true; |
||||||
|
} |
||||||
|
|
||||||
|
// If this transaction is not in our mempool, then we can't be sure
|
||||||
|
// we will know about all its inputs.
|
||||||
|
if (!pool.exists(entry.GetTx().GetHash())) { |
||||||
|
throw std::runtime_error("Cannot determine RBF opt-in signal for non-mempool transaction\n"); |
||||||
|
} |
||||||
|
|
||||||
|
// If all the inputs have nSequence >= maxint-1, it still might be
|
||||||
|
// signaled for RBF if any unconfirmed parents have signaled.
|
||||||
|
uint64_t noLimit = std::numeric_limits<uint64_t>::max(); |
||||||
|
std::string dummy; |
||||||
|
pool.CalculateMemPoolAncestors(entry, setAncestors, noLimit, noLimit, noLimit, noLimit, dummy, false); |
||||||
|
|
||||||
|
BOOST_FOREACH(CTxMemPool::txiter it, setAncestors) { |
||||||
|
if (SignalsOptInRBF(it->GetTx())) { |
||||||
|
return true; |
||||||
|
} |
||||||
|
} |
||||||
|
return false; |
||||||
|
} |
@ -0,0 +1,20 @@ |
|||||||
|
// Copyright (c) 2016 The Bitcoin developers
|
||||||
|
// Distributed under the MIT software license, see the accompanying
|
||||||
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||||
|
|
||||||
|
#ifndef BITCOIN_POLICY_RBF_H |
||||||
|
#define BITCOIN_POLICY_RBF_H |
||||||
|
|
||||||
|
#include "txmempool.h" |
||||||
|
|
||||||
|
// Check whether the sequence numbers on this transaction are signaling
|
||||||
|
// opt-in to replace-by-fee, according to BIP 125
|
||||||
|
bool SignalsOptInRBF(const CTransaction &tx); |
||||||
|
|
||||||
|
// Determine whether an in-mempool transaction is signaling opt-in to RBF
|
||||||
|
// according to BIP 125
|
||||||
|
// This involves checking sequence numbers of the transaction, as well
|
||||||
|
// as the sequence numbers of all in-mempool ancestors.
|
||||||
|
bool IsRBFOptIn(const CTxMemPoolEntry &entry, CTxMemPool &pool); |
||||||
|
|
||||||
|
#endif // BITCOIN_POLICY_RBF_H
|
Loading…
Reference in new issue