Browse Source
0.137aac6db
[QT] dump banlist to disk in case of ban/unban over QT (Jonas Schnelli)7f90ea7
[QA] adabt QT_NO_KEYWORDS for QT ban implementation (Jonas Schnelli)07f70b2
[QA] fix netbase tests because of new CSubNet::ToString() output (Jonas Schnelli)4ed0510
[Qt] call DumpBanlist() when baning unbaning nodes (Philip Kaufmann)be89292
[Qt] reenabling hotkeys for ban context menu, use different words (Jonas Schnelli)b1189cf
[Qt] adapt QT ban option to banlist.dat changes (Jonas Schnelli)65abe91
[Qt] add sorting for bantable (Philip Kaufmann)51654de
[Qt] bantable polish (Philip Kaufmann)cdd72cd
[Qt] simplify ban list signal handling (Philip Kaufmann)43c1f5b
[Qt] remove unused timer-code from banlistmodel.cpp (Jonas Schnelli)e2b8028
net: Fix CIDR notation in ToString() (Wladimir J. van der Laan)9e521c1
[Qt] polish ban table (Philip Kaufmann)607809f
net: use CIDR notation in CSubNet::ToString() (Jonas Schnelli)53caec6
[Qt] bantable overhaul (Jonas Schnelli)f0bcbc4
[Qt] bantable fix timestamp 64bit issue (Jonas Schnelli)6135309
[Qt] banlist, UI optimizing and better signal handling (Jonas Schnelli)770ca79
[Qt] add context menu with unban option to ban table (Jonas Schnelli)5f42132
[Qt] add ui signal for banlist changes (Jonas Schnelli)ad204df
[Qt] add banlist table below peers table (Jonas Schnelli)50f0908
[Qt] add ban functions to peers window (Jonas Schnelli)
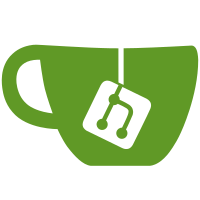
14 changed files with 654 additions and 56 deletions
@ -0,0 +1,181 @@
@@ -0,0 +1,181 @@
|
||||
// Copyright (c) 2011-2015 The Bitcoin Core developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#include "bantablemodel.h" |
||||
|
||||
#include "clientmodel.h" |
||||
#include "guiconstants.h" |
||||
#include "guiutil.h" |
||||
|
||||
#include "sync.h" |
||||
#include "utiltime.h" |
||||
|
||||
#include <QDebug> |
||||
#include <QList> |
||||
|
||||
bool BannedNodeLessThan::operator()(const CCombinedBan& left, const CCombinedBan& right) const |
||||
{ |
||||
const CCombinedBan* pLeft = &left; |
||||
const CCombinedBan* pRight = &right; |
||||
|
||||
if (order == Qt::DescendingOrder) |
||||
std::swap(pLeft, pRight); |
||||
|
||||
switch(column) |
||||
{ |
||||
case BanTableModel::Address: |
||||
return pLeft->subnet.ToString().compare(pRight->subnet.ToString()) < 0; |
||||
case BanTableModel::Bantime: |
||||
return pLeft->banEntry.nBanUntil < pRight->banEntry.nBanUntil; |
||||
} |
||||
|
||||
return false; |
||||
} |
||||
|
||||
// private implementation
|
||||
class BanTablePriv |
||||
{ |
||||
public: |
||||
/** Local cache of peer information */ |
||||
QList<CCombinedBan> cachedBanlist; |
||||
/** Column to sort nodes by */ |
||||
int sortColumn; |
||||
/** Order (ascending or descending) to sort nodes by */ |
||||
Qt::SortOrder sortOrder; |
||||
|
||||
/** Pull a full list of banned nodes from CNode into our cache */ |
||||
void refreshBanlist() |
||||
{ |
||||
banmap_t banMap; |
||||
CNode::GetBanned(banMap); |
||||
|
||||
cachedBanlist.clear(); |
||||
#if QT_VERSION >= 0x040700 |
||||
cachedBanlist.reserve(banMap.size()); |
||||
#endif |
||||
for (banmap_t::iterator it = banMap.begin(); it != banMap.end(); it++) |
||||
{ |
||||
CCombinedBan banEntry; |
||||
banEntry.subnet = (*it).first; |
||||
banEntry.banEntry = (*it).second; |
||||
cachedBanlist.append(banEntry); |
||||
} |
||||
|
||||
if (sortColumn >= 0) |
||||
// sort cachedBanlist (use stable sort to prevent rows jumping around unneceesarily)
|
||||
qStableSort(cachedBanlist.begin(), cachedBanlist.end(), BannedNodeLessThan(sortColumn, sortOrder)); |
||||
} |
||||
|
||||
int size() const |
||||
{ |
||||
return cachedBanlist.size(); |
||||
} |
||||
|
||||
CCombinedBan *index(int idx) |
||||
{ |
||||
if (idx >= 0 && idx < cachedBanlist.size()) |
||||
return &cachedBanlist[idx]; |
||||
|
||||
return 0; |
||||
} |
||||
}; |
||||
|
||||
BanTableModel::BanTableModel(ClientModel *parent) : |
||||
QAbstractTableModel(parent), |
||||
clientModel(parent) |
||||
{ |
||||
columns << tr("IP/Netmask") << tr("Banned Until"); |
||||
priv = new BanTablePriv(); |
||||
// default to unsorted
|
||||
priv->sortColumn = -1; |
||||
|
||||
// load initial data
|
||||
refresh(); |
||||
} |
||||
|
||||
int BanTableModel::rowCount(const QModelIndex &parent) const |
||||
{ |
||||
Q_UNUSED(parent); |
||||
return priv->size(); |
||||
} |
||||
|
||||
int BanTableModel::columnCount(const QModelIndex &parent) const |
||||
{ |
||||
Q_UNUSED(parent); |
||||
return columns.length();; |
||||
} |
||||
|
||||
QVariant BanTableModel::data(const QModelIndex &index, int role) const |
||||
{ |
||||
if(!index.isValid()) |
||||
return QVariant(); |
||||
|
||||
CCombinedBan *rec = static_cast<CCombinedBan*>(index.internalPointer()); |
||||
|
||||
if (role == Qt::DisplayRole) { |
||||
switch(index.column()) |
||||
{ |
||||
case Address: |
||||
return QString::fromStdString(rec->subnet.ToString()); |
||||
case Bantime: |
||||
QDateTime date = QDateTime::fromMSecsSinceEpoch(0); |
||||
date = date.addSecs(rec->banEntry.nBanUntil); |
||||
return date.toString(Qt::SystemLocaleLongDate); |
||||
} |
||||
} |
||||
|
||||
return QVariant(); |
||||
} |
||||
|
||||
QVariant BanTableModel::headerData(int section, Qt::Orientation orientation, int role) const |
||||
{ |
||||
if(orientation == Qt::Horizontal) |
||||
{ |
||||
if(role == Qt::DisplayRole && section < columns.size()) |
||||
{ |
||||
return columns[section]; |
||||
} |
||||
} |
||||
return QVariant(); |
||||
} |
||||
|
||||
Qt::ItemFlags BanTableModel::flags(const QModelIndex &index) const |
||||
{ |
||||
if(!index.isValid()) |
||||
return 0; |
||||
|
||||
Qt::ItemFlags retval = Qt::ItemIsSelectable | Qt::ItemIsEnabled; |
||||
return retval; |
||||
} |
||||
|
||||
QModelIndex BanTableModel::index(int row, int column, const QModelIndex &parent) const |
||||
{ |
||||
Q_UNUSED(parent); |
||||
CCombinedBan *data = priv->index(row); |
||||
|
||||
if (data) |
||||
return createIndex(row, column, data); |
||||
return QModelIndex(); |
||||
} |
||||
|
||||
void BanTableModel::refresh() |
||||
{ |
||||
Q_EMIT layoutAboutToBeChanged(); |
||||
priv->refreshBanlist(); |
||||
Q_EMIT layoutChanged(); |
||||
} |
||||
|
||||
void BanTableModel::sort(int column, Qt::SortOrder order) |
||||
{ |
||||
priv->sortColumn = column; |
||||
priv->sortOrder = order; |
||||
refresh(); |
||||
} |
||||
|
||||
bool BanTableModel::shouldShow() |
||||
{ |
||||
if (priv->size() > 0) |
||||
return true; |
||||
return false; |
||||
} |
@ -0,0 +1,72 @@
@@ -0,0 +1,72 @@
|
||||
// Copyright (c) 2011-2013 The Bitcoin Core developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#ifndef BITCOIN_QT_BANTABLEMODEL_H |
||||
#define BITCOIN_QT_BANTABLEMODEL_H |
||||
|
||||
#include "net.h" |
||||
|
||||
#include <QAbstractTableModel> |
||||
#include <QStringList> |
||||
|
||||
class ClientModel; |
||||
class BanTablePriv; |
||||
|
||||
struct CCombinedBan { |
||||
CSubNet subnet; |
||||
CBanEntry banEntry; |
||||
}; |
||||
|
||||
class BannedNodeLessThan |
||||
{ |
||||
public: |
||||
BannedNodeLessThan(int nColumn, Qt::SortOrder fOrder) : |
||||
column(nColumn), order(fOrder) {} |
||||
bool operator()(const CCombinedBan& left, const CCombinedBan& right) const; |
||||
|
||||
private: |
||||
int column; |
||||
Qt::SortOrder order; |
||||
}; |
||||
|
||||
/**
|
||||
Qt model providing information about connected peers, similar to the |
||||
"getpeerinfo" RPC call. Used by the rpc console UI. |
||||
*/ |
||||
class BanTableModel : public QAbstractTableModel |
||||
{ |
||||
Q_OBJECT |
||||
|
||||
public: |
||||
explicit BanTableModel(ClientModel *parent = 0); |
||||
void startAutoRefresh(); |
||||
void stopAutoRefresh(); |
||||
|
||||
enum ColumnIndex { |
||||
Address = 0, |
||||
Bantime = 1 |
||||
}; |
||||
|
||||
/** @name Methods overridden from QAbstractTableModel
|
||||
@{*/ |
||||
int rowCount(const QModelIndex &parent) const; |
||||
int columnCount(const QModelIndex &parent) const; |
||||
QVariant data(const QModelIndex &index, int role) const; |
||||
QVariant headerData(int section, Qt::Orientation orientation, int role) const; |
||||
QModelIndex index(int row, int column, const QModelIndex &parent) const; |
||||
Qt::ItemFlags flags(const QModelIndex &index) const; |
||||
void sort(int column, Qt::SortOrder order); |
||||
bool shouldShow(); |
||||
/*@}*/ |
||||
|
||||
public Q_SLOTS: |
||||
void refresh(); |
||||
|
||||
private: |
||||
ClientModel *clientModel; |
||||
QStringList columns; |
||||
BanTablePriv *priv; |
||||
}; |
||||
|
||||
#endif // BITCOIN_QT_BANTABLEMODEL_H
|
Loading…
Reference in new issue