Browse Source
0.1405a55a6
Added EVENT_CFLAGS to test makefile to explicitly include libevent headers. (Karl-Johan Alm)280a559
Added some simple tests for the RAII-style events. (Karl-Johan Alm)7f7f102
Switched bitcoin-cli.cpp to use RAII unique pointers with deleters. (Karl-Johan Alm)e5534d2
Added std::unique_ptr<> wrappers with deleters for libevent modules. (Karl-Johan Alm)
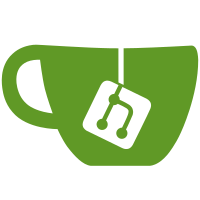
5 changed files with 160 additions and 22 deletions
@ -0,0 +1,56 @@
@@ -0,0 +1,56 @@
|
||||
// Copyright (c) 2016 The Bitcoin Core developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#ifndef BITCOIN_SUPPORT_EVENTS_H |
||||
#define BITCOIN_SUPPORT_EVENTS_H |
||||
|
||||
#include <ios> |
||||
#include <memory> |
||||
|
||||
#include <event2/event.h> |
||||
#include <event2/http.h> |
||||
|
||||
#define MAKE_RAII(type) \ |
||||
/* deleter */\ |
||||
struct type##_deleter {\ |
||||
void operator()(struct type* ob) {\ |
||||
type##_free(ob);\ |
||||
}\ |
||||
};\ |
||||
/* unique ptr typedef */\ |
||||
typedef std::unique_ptr<struct type, type##_deleter> raii_##type |
||||
|
||||
MAKE_RAII(event_base); |
||||
MAKE_RAII(event); |
||||
MAKE_RAII(evhttp); |
||||
MAKE_RAII(evhttp_request); |
||||
MAKE_RAII(evhttp_connection); |
||||
|
||||
raii_event_base obtain_event_base() { |
||||
auto result = raii_event_base(event_base_new()); |
||||
if (!result.get()) |
||||
throw std::runtime_error("cannot create event_base"); |
||||
return result; |
||||
} |
||||
|
||||
raii_event obtain_event(struct event_base* base, evutil_socket_t s, short events, event_callback_fn cb, void* arg) { |
||||
return raii_event(event_new(base, s, events, cb, arg)); |
||||
} |
||||
|
||||
raii_evhttp obtain_evhttp(struct event_base* base) { |
||||
return raii_evhttp(evhttp_new(base)); |
||||
} |
||||
|
||||
raii_evhttp_request obtain_evhttp_request(void(*cb)(struct evhttp_request *, void *), void *arg) { |
||||
return raii_evhttp_request(evhttp_request_new(cb, arg)); |
||||
} |
||||
|
||||
raii_evhttp_connection obtain_evhttp_connection_base(struct event_base* base, std::string host, uint16_t port) { |
||||
auto result = raii_evhttp_connection(evhttp_connection_base_new(base, NULL, host.c_str(), port)); |
||||
if (!result.get()) |
||||
throw std::runtime_error("create connection failed"); |
||||
return result; |
||||
} |
||||
|
||||
#endif // BITCOIN_SUPPORT_EVENTS_H
|
@ -0,0 +1,88 @@
@@ -0,0 +1,88 @@
|
||||
// Copyright (c) 2016 The Bitcoin Core developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#include <event2/event.h> |
||||
#include <map> |
||||
#include <stdlib.h> |
||||
|
||||
#include "support/events.h" |
||||
|
||||
#include "test/test_bitcoin.h" |
||||
|
||||
#include <vector> |
||||
|
||||
#include <boost/test/unit_test.hpp> |
||||
|
||||
static std::map<void*, short> tags; |
||||
static std::map<void*, uint16_t> orders; |
||||
static uint16_t tagSequence = 0; |
||||
|
||||
static void* tag_malloc(size_t sz) { |
||||
void* mem = malloc(sz); |
||||
if (!mem) return mem; |
||||
tags[mem]++; |
||||
orders[mem] = tagSequence++; |
||||
return mem; |
||||
} |
||||
|
||||
static void tag_free(void* mem) { |
||||
tags[mem]--; |
||||
orders[mem] = tagSequence++; |
||||
free(mem); |
||||
} |
||||
|
||||
BOOST_FIXTURE_TEST_SUITE(raii_event_tests, BasicTestingSetup) |
||||
|
||||
BOOST_AUTO_TEST_CASE(raii_event_creation) |
||||
{ |
||||
event_set_mem_functions(tag_malloc, realloc, tag_free); |
||||
|
||||
void* base_ptr = NULL; |
||||
{ |
||||
auto base = obtain_event_base(); |
||||
base_ptr = (void*)base.get(); |
||||
BOOST_CHECK(tags[base_ptr] == 1); |
||||
} |
||||
BOOST_CHECK(tags[base_ptr] == 0); |
||||
|
||||
void* event_ptr = NULL; |
||||
{ |
||||
auto base = obtain_event_base(); |
||||
auto event = obtain_event(base.get(), -1, 0, NULL, NULL); |
||||
|
||||
base_ptr = (void*)base.get(); |
||||
event_ptr = (void*)event.get(); |
||||
|
||||
BOOST_CHECK(tags[base_ptr] == 1); |
||||
BOOST_CHECK(tags[event_ptr] == 1); |
||||
} |
||||
BOOST_CHECK(tags[base_ptr] == 0); |
||||
BOOST_CHECK(tags[event_ptr] == 0); |
||||
|
||||
event_set_mem_functions(malloc, realloc, free); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(raii_event_order) |
||||
{ |
||||
event_set_mem_functions(tag_malloc, realloc, tag_free); |
||||
|
||||
void* base_ptr = NULL; |
||||
void* event_ptr = NULL; |
||||
{ |
||||
auto base = obtain_event_base(); |
||||
auto event = obtain_event(base.get(), -1, 0, NULL, NULL); |
||||
|
||||
base_ptr = (void*)base.get(); |
||||
event_ptr = (void*)event.get(); |
||||
|
||||
// base should have allocated before event
|
||||
BOOST_CHECK(orders[base_ptr] < orders[event_ptr]); |
||||
} |
||||
// base should be freed after event
|
||||
BOOST_CHECK(orders[base_ptr] > orders[event_ptr]); |
||||
|
||||
event_set_mem_functions(malloc, realloc, free); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_SUITE_END() |
Loading…
Reference in new issue