Browse Source
Logic: - If sending a transaction, assign its timestamp to the current time. - If receiving a transaction outside a block, assign its timestamp to the current time. - If receiving a block with a future timestamp, assign all its (not already known) transactions' timestamps to the current time. - If receiving a block with a past timestamp, before the most recent known transaction (that we care about), assign all its (not already known) transactions' timestamps to the same timestamp as that most-recent-known transaction. - If receiving a block with a past timestamp, but after the most recent known transaction, assign all its (not already known) transactions' timestamps to the block time.0.8
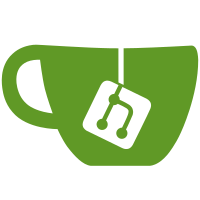
3 changed files with 88 additions and 23 deletions
Loading…
Reference in new issue