Browse Source
0.139a1dcea
Use CScheduler for net's DumpAddresses (Gavin Andresen)ddd0acd
Create a scheduler thread for lightweight tasks (Gavin Andresen)68d370b
CScheduler unit test (Gavin Andresen)cfefe5b
scheduler: fix with boost <= 1.50 (Cory Fields)ca66717
build: make libboost_chrono mandatory (Cory Fields)928b950
CScheduler class for lightweight task scheduling (Gavin Andresen)e656560
[Qt] add defaultConfirmTarget constant to sendcoinsdialog (Philip Kaufmann)
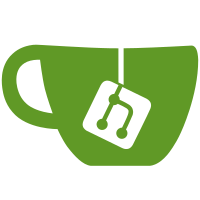
13 changed files with 304 additions and 51 deletions
@ -0,0 +1,98 @@
@@ -0,0 +1,98 @@
|
||||
// Copyright (c) 2015 The Bitcoin Core developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#include "scheduler.h" |
||||
|
||||
#include <assert.h> |
||||
#include <boost/bind.hpp> |
||||
#include <utility> |
||||
|
||||
CScheduler::CScheduler() : nThreadsServicingQueue(0) |
||||
{ |
||||
} |
||||
|
||||
CScheduler::~CScheduler() |
||||
{ |
||||
assert(nThreadsServicingQueue == 0); |
||||
} |
||||
|
||||
|
||||
#if BOOST_VERSION < 105000 |
||||
static boost::system_time toPosixTime(const boost::chrono::system_clock::time_point& t) |
||||
{ |
||||
return boost::posix_time::from_time_t(boost::chrono::system_clock::to_time_t(t)); |
||||
} |
||||
#endif |
||||
|
||||
void CScheduler::serviceQueue() |
||||
{ |
||||
boost::unique_lock<boost::mutex> lock(newTaskMutex); |
||||
++nThreadsServicingQueue; |
||||
|
||||
// newTaskMutex is locked throughout this loop EXCEPT
|
||||
// when the thread is waiting or when the user's function
|
||||
// is called.
|
||||
while (1) { |
||||
try { |
||||
while (taskQueue.empty()) { |
||||
// Wait until there is something to do.
|
||||
newTaskScheduled.wait(lock); |
||||
} |
||||
// Wait until either there is a new task, or until
|
||||
// the time of the first item on the queue:
|
||||
|
||||
// wait_until needs boost 1.50 or later; older versions have timed_wait:
|
||||
#if BOOST_VERSION < 105000 |
||||
while (!taskQueue.empty() && newTaskScheduled.timed_wait(lock, toPosixTime(taskQueue.begin()->first))) { |
||||
// Keep waiting until timeout
|
||||
} |
||||
#else |
||||
while (!taskQueue.empty() && newTaskScheduled.wait_until(lock, taskQueue.begin()->first) != boost::cv_status::timeout) { |
||||
// Keep waiting until timeout
|
||||
} |
||||
#endif |
||||
// If there are multiple threads, the queue can empty while we're waiting (another
|
||||
// thread may service the task we were waiting on).
|
||||
if (taskQueue.empty()) |
||||
continue; |
||||
|
||||
Function f = taskQueue.begin()->second; |
||||
taskQueue.erase(taskQueue.begin()); |
||||
|
||||
// Unlock before calling f, so it can reschedule itself or another task
|
||||
// without deadlocking:
|
||||
lock.unlock(); |
||||
f(); |
||||
lock.lock(); |
||||
} catch (...) { |
||||
--nThreadsServicingQueue; |
||||
throw; |
||||
} |
||||
} |
||||
} |
||||
|
||||
void CScheduler::schedule(CScheduler::Function f, boost::chrono::system_clock::time_point t) |
||||
{ |
||||
{ |
||||
boost::unique_lock<boost::mutex> lock(newTaskMutex); |
||||
taskQueue.insert(std::make_pair(t, f)); |
||||
} |
||||
newTaskScheduled.notify_one(); |
||||
} |
||||
|
||||
void CScheduler::scheduleFromNow(CScheduler::Function f, int64_t deltaSeconds) |
||||
{ |
||||
schedule(f, boost::chrono::system_clock::now() + boost::chrono::seconds(deltaSeconds)); |
||||
} |
||||
|
||||
static void Repeat(CScheduler* s, CScheduler::Function f, int64_t deltaSeconds) |
||||
{ |
||||
f(); |
||||
s->scheduleFromNow(boost::bind(&Repeat, s, f, deltaSeconds), deltaSeconds); |
||||
} |
||||
|
||||
void CScheduler::scheduleEvery(CScheduler::Function f, int64_t deltaSeconds) |
||||
{ |
||||
scheduleFromNow(boost::bind(&Repeat, this, f, deltaSeconds), deltaSeconds); |
||||
} |
@ -0,0 +1,70 @@
@@ -0,0 +1,70 @@
|
||||
// Copyright (c) 2015 The Bitcoin Core developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#ifndef BITCOIN_SCHEDULER_H |
||||
#define BITCOIN_SCHEDULER_H |
||||
|
||||
//
|
||||
// NOTE:
|
||||
// boost::thread / boost::function / boost::chrono should be ported to
|
||||
// std::thread / std::function / std::chrono when we support C++11.
|
||||
//
|
||||
#include <boost/function.hpp> |
||||
#include <boost/chrono/chrono.hpp> |
||||
#include <boost/thread.hpp> |
||||
#include <map> |
||||
|
||||
//
|
||||
// Simple class for background tasks that should be run
|
||||
// periodically or once "after a while"
|
||||
//
|
||||
// Usage:
|
||||
//
|
||||
// CScheduler* s = new CScheduler();
|
||||
// s->scheduleFromNow(doSomething, 11); // Assuming a: void doSomething() { }
|
||||
// s->scheduleFromNow(boost::bind(Class::func, this, argument), 3);
|
||||
// boost::thread* t = new boost::thread(boost::bind(CScheduler::serviceQueue, s));
|
||||
//
|
||||
// ... then at program shutdown, clean up the thread running serviceQueue:
|
||||
// t->interrupt();
|
||||
// t->join();
|
||||
// delete t;
|
||||
// delete s; // Must be done after thread is interrupted/joined.
|
||||
//
|
||||
|
||||
class CScheduler |
||||
{ |
||||
public: |
||||
CScheduler(); |
||||
~CScheduler(); |
||||
|
||||
typedef boost::function<void(void)> Function; |
||||
|
||||
// Call func at/after time t
|
||||
void schedule(Function f, boost::chrono::system_clock::time_point t); |
||||
|
||||
// Convenience method: call f once deltaSeconds from now
|
||||
void scheduleFromNow(Function f, int64_t deltaSeconds); |
||||
|
||||
// Another convenience method: call f approximately
|
||||
// every deltaSeconds forever, starting deltaSeconds from now.
|
||||
// To be more precise: every time f is finished, it
|
||||
// is rescheduled to run deltaSeconds later. If you
|
||||
// need more accurate scheduling, don't use this method.
|
||||
void scheduleEvery(Function f, int64_t deltaSeconds); |
||||
|
||||
// To keep things as simple as possible, there is no unschedule.
|
||||
|
||||
// Services the queue 'forever'. Should be run in a thread,
|
||||
// and interrupted using boost::interrupt_thread
|
||||
void serviceQueue(); |
||||
|
||||
private: |
||||
std::multimap<boost::chrono::system_clock::time_point, Function> taskQueue; |
||||
boost::condition_variable newTaskScheduled; |
||||
boost::mutex newTaskMutex; |
||||
int nThreadsServicingQueue; |
||||
}; |
||||
|
||||
#endif |
@ -0,0 +1,110 @@
@@ -0,0 +1,110 @@
|
||||
// Copyright (c) 2012-2013 The Bitcoin Core developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#include "random.h" |
||||
#include "scheduler.h" |
||||
|
||||
#include "test/test_bitcoin.h" |
||||
|
||||
#include <boost/bind.hpp> |
||||
#include <boost/random/mersenne_twister.hpp> |
||||
#include <boost/random/uniform_int_distribution.hpp> |
||||
#include <boost/thread.hpp> |
||||
#include <boost/test/unit_test.hpp> |
||||
|
||||
BOOST_AUTO_TEST_SUITE(scheduler_tests) |
||||
|
||||
static void microTask(CScheduler& s, boost::mutex& mutex, int& counter, int delta, boost::chrono::system_clock::time_point rescheduleTime) |
||||
{ |
||||
{ |
||||
boost::unique_lock<boost::mutex> lock(mutex); |
||||
counter += delta; |
||||
} |
||||
boost::chrono::system_clock::time_point noTime = boost::chrono::system_clock::time_point::min(); |
||||
if (rescheduleTime != noTime) { |
||||
CScheduler::Function f = boost::bind(µTask, boost::ref(s), boost::ref(mutex), boost::ref(counter), -delta + 1, noTime); |
||||
s.schedule(f, rescheduleTime); |
||||
} |
||||
} |
||||
|
||||
static void MicroSleep(uint64_t n) |
||||
{ |
||||
#if defined(HAVE_WORKING_BOOST_SLEEP_FOR) |
||||
boost::this_thread::sleep_for(boost::chrono::microseconds(n)); |
||||
#elif defined(HAVE_WORKING_BOOST_SLEEP) |
||||
boost::this_thread::sleep(boost::posix_time::microseconds(n)); |
||||
#else |
||||
//should never get here
|
||||
#error missing boost sleep implementation |
||||
#endif |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(manythreads) |
||||
{ |
||||
// Stress test: hundreds of microsecond-scheduled tasks,
|
||||
// serviced by 10 threads.
|
||||
//
|
||||
// So... ten shared counters, which if all the tasks execute
|
||||
// properly will sum to the number of tasks done.
|
||||
// Each task adds or subtracts from one of the counters a
|
||||
// random amount, and then schedules another task 0-1000
|
||||
// microseconds in the future to subtract or add from
|
||||
// the counter -random_amount+1, so in the end the shared
|
||||
// counters should sum to the number of initial tasks performed.
|
||||
CScheduler microTasks; |
||||
|
||||
boost::thread_group microThreads; |
||||
for (int i = 0; i < 5; i++) |
||||
microThreads.create_thread(boost::bind(&CScheduler::serviceQueue, µTasks)); |
||||
|
||||
boost::mutex counterMutex[10]; |
||||
int counter[10] = { 0 }; |
||||
boost::random::mt19937 rng(insecure_rand()); |
||||
boost::random::uniform_int_distribution<> zeroToNine(0, 9); |
||||
boost::random::uniform_int_distribution<> randomMsec(-11, 1000); |
||||
boost::random::uniform_int_distribution<> randomDelta(-1000, 1000); |
||||
|
||||
boost::chrono::system_clock::time_point start = boost::chrono::system_clock::now(); |
||||
boost::chrono::system_clock::time_point now = start; |
||||
|
||||
for (int i = 0; i < 100; i++) { |
||||
boost::chrono::system_clock::time_point t = now + boost::chrono::microseconds(randomMsec(rng)); |
||||
boost::chrono::system_clock::time_point tReschedule = now + boost::chrono::microseconds(500 + randomMsec(rng)); |
||||
int whichCounter = zeroToNine(rng); |
||||
CScheduler::Function f = boost::bind(µTask, boost::ref(microTasks), |
||||
boost::ref(counterMutex[whichCounter]), boost::ref(counter[whichCounter]), |
||||
randomDelta(rng), tReschedule); |
||||
microTasks.schedule(f, t); |
||||
} |
||||
|
||||
MicroSleep(600); |
||||
now = boost::chrono::system_clock::now(); |
||||
// More threads and more tasks:
|
||||
for (int i = 0; i < 5; i++) |
||||
microThreads.create_thread(boost::bind(&CScheduler::serviceQueue, µTasks)); |
||||
for (int i = 0; i < 100; i++) { |
||||
boost::chrono::system_clock::time_point t = now + boost::chrono::microseconds(randomMsec(rng)); |
||||
boost::chrono::system_clock::time_point tReschedule = now + boost::chrono::microseconds(500 + randomMsec(rng)); |
||||
int whichCounter = zeroToNine(rng); |
||||
CScheduler::Function f = boost::bind(µTask, boost::ref(microTasks), |
||||
boost::ref(counterMutex[whichCounter]), boost::ref(counter[whichCounter]), |
||||
randomDelta(rng), tReschedule); |
||||
microTasks.schedule(f, t); |
||||
} |
||||
|
||||
// All 2,000 tasks should be finished within 2 milliseconds. Sleep a bit longer.
|
||||
MicroSleep(2100); |
||||
|
||||
microThreads.interrupt_all(); |
||||
microThreads.join_all(); |
||||
|
||||
int counterSum = 0; |
||||
for (int i = 0; i < 10; i++) { |
||||
BOOST_CHECK(counter[i] != 0); |
||||
counterSum += counter[i]; |
||||
} |
||||
BOOST_CHECK_EQUAL(counterSum, 200); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_SUITE_END() |
Loading…
Reference in new issue