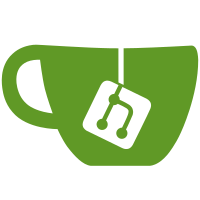
4 changed files with 111 additions and 0 deletions
@ -0,0 +1,34 @@
@@ -0,0 +1,34 @@
|
||||
// Copyright (c) 2014 The Bitcoin developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#include "crypto/hmac_sha256.h" |
||||
|
||||
#include <string.h> |
||||
|
||||
CHMAC_SHA256::CHMAC_SHA256(const unsigned char* key, size_t keylen) |
||||
{ |
||||
unsigned char rkey[64]; |
||||
if (keylen <= 64) { |
||||
memcpy(rkey, key, keylen); |
||||
memset(rkey + keylen, 0, 64 - keylen); |
||||
} else { |
||||
CSHA256().Write(key, keylen).Finalize(rkey); |
||||
memset(rkey + 32, 0, 32); |
||||
} |
||||
|
||||
for (int n = 0; n < 64; n++) |
||||
rkey[n] ^= 0x5c; |
||||
outer.Write(rkey, 64); |
||||
|
||||
for (int n = 0; n < 64; n++) |
||||
rkey[n] ^= 0x5c ^ 0x36; |
||||
inner.Write(rkey, 64); |
||||
} |
||||
|
||||
void CHMAC_SHA256::Finalize(unsigned char hash[OUTPUT_SIZE]) |
||||
{ |
||||
unsigned char temp[32]; |
||||
inner.Finalize(temp); |
||||
outer.Write(temp, 32).Finalize(hash); |
||||
} |
@ -0,0 +1,32 @@
@@ -0,0 +1,32 @@
|
||||
// Copyright (c) 2014 The Bitcoin developers
|
||||
// Distributed under the MIT software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#ifndef BITCOIN_HMAC_SHA256_H |
||||
#define BITCOIN_HMAC_SHA256_H |
||||
|
||||
#include "crypto/sha256.h" |
||||
|
||||
#include <stdint.h> |
||||
#include <stdlib.h> |
||||
|
||||
/** A hasher class for HMAC-SHA-512. */ |
||||
class CHMAC_SHA256 |
||||
{ |
||||
private: |
||||
CSHA256 outer; |
||||
CSHA256 inner; |
||||
|
||||
public: |
||||
static const size_t OUTPUT_SIZE = 32; |
||||
|
||||
CHMAC_SHA256(const unsigned char* key, size_t keylen); |
||||
CHMAC_SHA256& Write(const unsigned char* data, size_t len) |
||||
{ |
||||
inner.Write(data, len); |
||||
return *this; |
||||
} |
||||
void Finalize(unsigned char hash[OUTPUT_SIZE]); |
||||
}; |
||||
|
||||
#endif // BITCOIN_SHA256_H
|
Loading…
Reference in new issue