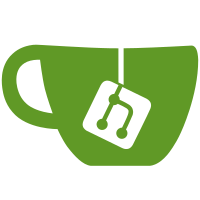
21 changed files with 824 additions and 544 deletions
@ -0,0 +1,74 @@
@@ -0,0 +1,74 @@
|
||||
#include <boost/test/unit_test.hpp> |
||||
#include <boost/foreach.hpp> |
||||
|
||||
#include "base58.h" |
||||
#include "util.h" |
||||
#include "json/json_spirit_reader_template.h" |
||||
#include "json/json_spirit_writer_template.h" |
||||
#include "json/json_spirit_utils.h" |
||||
|
||||
using namespace std; |
||||
using namespace json_spirit; |
||||
|
||||
typedef Value(*rpcfn_type)(const Array& params, bool fHelp); |
||||
extern map<string, rpcfn_type> mapCallTable; |
||||
|
||||
BOOST_AUTO_TEST_SUITE(rpc_tests) |
||||
|
||||
static Array |
||||
createArgs(int nRequired, const char* address1=NULL, const char* address2=NULL) |
||||
{ |
||||
Array result; |
||||
result.push_back(nRequired); |
||||
Array addresses; |
||||
if (address1) addresses.push_back(address1); |
||||
if (address2) addresses.push_back(address1); |
||||
result.push_back(addresses); |
||||
return result; |
||||
} |
||||
|
||||
// This can be removed this when addmultisigaddress is enabled on main net:
|
||||
struct TestNetFixture |
||||
{ |
||||
TestNetFixture() { fTestNet = true; } |
||||
~TestNetFixture() { fTestNet = false; } |
||||
}; |
||||
|
||||
BOOST_FIXTURE_TEST_CASE(rpc_addmultisig, TestNetFixture) |
||||
{ |
||||
rpcfn_type addmultisig = mapCallTable["addmultisigaddress"]; |
||||
|
||||
// old, 65-byte-long:
|
||||
const char* address1Hex = "0434e3e09f49ea168c5bbf53f877ff4206923858aab7c7e1df25bc263978107c95e35065a27ef6f1b27222db0ec97e0e895eaca603d3ee0d4c060ce3d8a00286c8"; |
||||
// new, compressed:
|
||||
const char* address2Hex = "0388c2037017c62240b6b72ac1a2a5f94da790596ebd06177c8572752922165cb4"; |
||||
|
||||
Value v; |
||||
CBitcoinAddress address; |
||||
BOOST_CHECK_NO_THROW(v = addmultisig(createArgs(1, address1Hex), false)); |
||||
address.SetString(v.get_str()); |
||||
BOOST_CHECK(address.IsValid() && address.IsScript()); |
||||
|
||||
BOOST_CHECK_NO_THROW(v = addmultisig(createArgs(1, address1Hex, address2Hex), false)); |
||||
address.SetString(v.get_str()); |
||||
BOOST_CHECK(address.IsValid() && address.IsScript()); |
||||
|
||||
BOOST_CHECK_NO_THROW(v = addmultisig(createArgs(2, address1Hex, address2Hex), false)); |
||||
address.SetString(v.get_str()); |
||||
BOOST_CHECK(address.IsValid() && address.IsScript()); |
||||
|
||||
BOOST_CHECK_THROW(addmultisig(createArgs(0), false), runtime_error); |
||||
BOOST_CHECK_THROW(addmultisig(createArgs(1), false), runtime_error); |
||||
BOOST_CHECK_THROW(addmultisig(createArgs(2, address1Hex), false), runtime_error); |
||||
|
||||
BOOST_CHECK_THROW(addmultisig(createArgs(1, ""), false), runtime_error); |
||||
BOOST_CHECK_THROW(addmultisig(createArgs(1, "NotAValidPubkey"), false), runtime_error); |
||||
|
||||
string short1(address1Hex, address1Hex+sizeof(address1Hex)-2); // last byte missing
|
||||
BOOST_CHECK_THROW(addmultisig(createArgs(2, short1.c_str()), false), runtime_error); |
||||
|
||||
string short2(address1Hex+2, address1Hex+sizeof(address1Hex)); // first byte missing
|
||||
BOOST_CHECK_THROW(addmultisig(createArgs(2, short2.c_str()), false), runtime_error); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_SUITE_END() |
@ -0,0 +1,310 @@
@@ -0,0 +1,310 @@
|
||||
#include <boost/assert.hpp> |
||||
#include <boost/assign/list_of.hpp> |
||||
#include <boost/assign/list_inserter.hpp> |
||||
#include <boost/assign/std/vector.hpp> |
||||
#include <boost/test/unit_test.hpp> |
||||
#include <boost/foreach.hpp> |
||||
|
||||
#include "../main.h" |
||||
#include "../script.h" |
||||
#include "../wallet.h" |
||||
|
||||
using namespace std; |
||||
|
||||
// Test routines internal to script.cpp:
|
||||
extern uint256 SignatureHash(CScript scriptCode, const CTransaction& txTo, unsigned int nIn, int nHashType); |
||||
extern bool VerifyScript(const CScript& scriptSig, const CScript& scriptPubKey, const CTransaction& txTo, unsigned int nIn, |
||||
bool fValidatePayToScriptHash, int nHashType); |
||||
|
||||
// Helpers:
|
||||
static std::vector<unsigned char> |
||||
Serialize(const CScript& s) |
||||
{ |
||||
std::vector<unsigned char> sSerialized(s); |
||||
return sSerialized; |
||||
} |
||||
|
||||
static bool |
||||
Verify(const CScript& scriptSig, const CScript& scriptPubKey, bool fStrict) |
||||
{ |
||||
// Create dummy to/from transactions:
|
||||
CTransaction txFrom; |
||||
txFrom.vout.resize(1); |
||||
txFrom.vout[0].scriptPubKey = scriptPubKey; |
||||
|
||||
CTransaction txTo; |
||||
txTo.vin.resize(1); |
||||
txTo.vout.resize(1); |
||||
txTo.vin[0].prevout.n = 0; |
||||
txTo.vin[0].prevout.hash = txFrom.GetHash(); |
||||
txTo.vin[0].scriptSig = scriptSig; |
||||
txTo.vout[0].nValue = 1; |
||||
|
||||
return VerifyScript(scriptSig, scriptPubKey, txTo, 0, fStrict, 0); |
||||
} |
||||
|
||||
|
||||
BOOST_AUTO_TEST_SUITE(script_P2SH_tests) |
||||
|
||||
BOOST_AUTO_TEST_CASE(sign) |
||||
{ |
||||
// Pay-to-script-hash looks like this:
|
||||
// scriptSig: <sig> <sig...> <serialized_script>
|
||||
// scriptPubKey: HASH160 <hash> EQUAL
|
||||
|
||||
// Test SignSignature() (and therefore the version of Solver() that signs transactions)
|
||||
CBasicKeyStore keystore; |
||||
CKey key[4]; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
key[i].MakeNewKey(); |
||||
keystore.AddKey(key[i]); |
||||
} |
||||
|
||||
// 8 Scripts: checking all combinations of
|
||||
// different keys, straight/P2SH, pubkey/pubkeyhash
|
||||
CScript standardScripts[4]; |
||||
standardScripts[0] << key[0].GetPubKey() << OP_CHECKSIG; |
||||
standardScripts[1].SetBitcoinAddress(key[1].GetPubKey()); |
||||
standardScripts[2] << key[1].GetPubKey() << OP_CHECKSIG; |
||||
standardScripts[3].SetBitcoinAddress(key[2].GetPubKey()); |
||||
CScript evalScripts[4]; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
keystore.AddCScript(standardScripts[i]); |
||||
evalScripts[i].SetPayToScriptHash(standardScripts[i]); |
||||
} |
||||
|
||||
CTransaction txFrom; // Funding transaction:
|
||||
txFrom.vout.resize(8); |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
txFrom.vout[i].scriptPubKey = evalScripts[i]; |
||||
txFrom.vout[i+4].scriptPubKey = standardScripts[i]; |
||||
} |
||||
BOOST_CHECK(txFrom.IsStandard()); |
||||
|
||||
CTransaction txTo[8]; // Spending transactions
|
||||
for (int i = 0; i < 8; i++) |
||||
{ |
||||
txTo[i].vin.resize(1); |
||||
txTo[i].vout.resize(1); |
||||
txTo[i].vin[0].prevout.n = i; |
||||
txTo[i].vin[0].prevout.hash = txFrom.GetHash(); |
||||
txTo[i].vout[0].nValue = 1; |
||||
BOOST_CHECK_MESSAGE(IsMine(keystore, txFrom.vout[i].scriptPubKey), strprintf("IsMine %d", i)); |
||||
} |
||||
for (int i = 0; i < 8; i++) |
||||
{ |
||||
BOOST_CHECK_MESSAGE(SignSignature(keystore, txFrom, txTo[i], 0), strprintf("SignSignature %d", i)); |
||||
} |
||||
// All of the above should be OK, and the txTos have valid signatures
|
||||
// Check to make sure signature verification fails if we use the wrong ScriptSig:
|
||||
for (int i = 0; i < 8; i++) |
||||
for (int j = 0; j < 8; j++) |
||||
{ |
||||
CScript sigSave = txTo[i].vin[0].scriptSig; |
||||
txTo[i].vin[0].scriptSig = txTo[j].vin[0].scriptSig; |
||||
bool sigOK = VerifySignature(txFrom, txTo[i], 0, true, 0); |
||||
if (i == j) |
||||
BOOST_CHECK_MESSAGE(sigOK, strprintf("VerifySignature %d %d", i, j)); |
||||
else |
||||
BOOST_CHECK_MESSAGE(!sigOK, strprintf("VerifySignature %d %d", i, j)); |
||||
txTo[i].vin[0].scriptSig = sigSave; |
||||
} |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(norecurse) |
||||
{ |
||||
// Make sure only the outer pay-to-script-hash does the
|
||||
// extra-validation thing:
|
||||
CScript invalidAsScript; |
||||
invalidAsScript << OP_INVALIDOPCODE << OP_INVALIDOPCODE; |
||||
|
||||
CScript p2sh; |
||||
p2sh.SetPayToScriptHash(invalidAsScript); |
||||
|
||||
CScript scriptSig; |
||||
scriptSig << Serialize(invalidAsScript); |
||||
|
||||
// Should not verify, because it will try to execute OP_INVALIDOPCODE
|
||||
BOOST_CHECK(!Verify(scriptSig, p2sh, true)); |
||||
|
||||
// Try to recurse, and verification should succeed because
|
||||
// the inner HASH160 <> EQUAL should only check the hash:
|
||||
CScript p2sh2; |
||||
p2sh2.SetPayToScriptHash(p2sh); |
||||
CScript scriptSig2; |
||||
scriptSig2 << Serialize(invalidAsScript) << Serialize(p2sh); |
||||
|
||||
BOOST_CHECK(Verify(scriptSig2, p2sh2, true)); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(set) |
||||
{ |
||||
// Test the CScript::Set* methods
|
||||
CBasicKeyStore keystore; |
||||
CKey key[4]; |
||||
std::vector<CKey> keys; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
key[i].MakeNewKey(); |
||||
keystore.AddKey(key[i]); |
||||
keys.push_back(key[i]); |
||||
} |
||||
|
||||
CScript inner[4]; |
||||
inner[0].SetBitcoinAddress(key[0].GetPubKey()); |
||||
inner[1].SetMultisig(2, std::vector<CKey>(keys.begin(), keys.begin()+2)); |
||||
inner[2].SetMultisig(1, std::vector<CKey>(keys.begin(), keys.begin()+2)); |
||||
inner[3].SetMultisig(2, std::vector<CKey>(keys.begin(), keys.begin()+3)); |
||||
|
||||
CScript outer[4]; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
outer[i].SetPayToScriptHash(inner[i]); |
||||
keystore.AddCScript(inner[i]); |
||||
} |
||||
|
||||
CTransaction txFrom; // Funding transaction:
|
||||
txFrom.vout.resize(4); |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
txFrom.vout[i].scriptPubKey = outer[i]; |
||||
} |
||||
BOOST_CHECK(txFrom.IsStandard()); |
||||
|
||||
CTransaction txTo[4]; // Spending transactions
|
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
txTo[i].vin.resize(1); |
||||
txTo[i].vout.resize(1); |
||||
txTo[i].vin[0].prevout.n = i; |
||||
txTo[i].vin[0].prevout.hash = txFrom.GetHash(); |
||||
txTo[i].vout[0].nValue = 1; |
||||
txTo[i].vout[0].scriptPubKey = inner[i]; |
||||
BOOST_CHECK_MESSAGE(IsMine(keystore, txFrom.vout[i].scriptPubKey), strprintf("IsMine %d", i)); |
||||
} |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
BOOST_CHECK_MESSAGE(SignSignature(keystore, txFrom, txTo[i], 0), strprintf("SignSignature %d", i)); |
||||
BOOST_CHECK_MESSAGE(txTo[i].IsStandard(), strprintf("txTo[%d].IsStandard", i)); |
||||
} |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(is) |
||||
{ |
||||
// Test CScript::IsPayToScriptHash()
|
||||
uint160 dummy; |
||||
CScript p2sh; |
||||
p2sh << OP_HASH160 << dummy << OP_EQUAL; |
||||
BOOST_CHECK(p2sh.IsPayToScriptHash()); |
||||
|
||||
// Not considered pay-to-script-hash if using one of the OP_PUSHDATA opcodes:
|
||||
static const unsigned char direct[] = { OP_HASH160, 20, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, OP_EQUAL }; |
||||
BOOST_CHECK(CScript(direct, direct+sizeof(direct)).IsPayToScriptHash()); |
||||
static const unsigned char pushdata1[] = { OP_HASH160, OP_PUSHDATA1, 20, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, OP_EQUAL }; |
||||
BOOST_CHECK(!CScript(pushdata1, pushdata1+sizeof(pushdata1)).IsPayToScriptHash()); |
||||
static const unsigned char pushdata2[] = { OP_HASH160, OP_PUSHDATA2, 20,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, OP_EQUAL }; |
||||
BOOST_CHECK(!CScript(pushdata2, pushdata2+sizeof(pushdata2)).IsPayToScriptHash()); |
||||
static const unsigned char pushdata4[] = { OP_HASH160, OP_PUSHDATA4, 20,0,0,0, 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0, OP_EQUAL }; |
||||
BOOST_CHECK(!CScript(pushdata4, pushdata4+sizeof(pushdata4)).IsPayToScriptHash()); |
||||
|
||||
CScript not_p2sh; |
||||
BOOST_CHECK(!not_p2sh.IsPayToScriptHash()); |
||||
|
||||
not_p2sh.clear(); not_p2sh << OP_HASH160 << dummy << dummy << OP_EQUAL; |
||||
BOOST_CHECK(!not_p2sh.IsPayToScriptHash()); |
||||
|
||||
not_p2sh.clear(); not_p2sh << OP_NOP << dummy << OP_EQUAL; |
||||
BOOST_CHECK(!not_p2sh.IsPayToScriptHash()); |
||||
|
||||
not_p2sh.clear(); not_p2sh << OP_HASH160 << dummy << OP_CHECKSIG; |
||||
BOOST_CHECK(!not_p2sh.IsPayToScriptHash()); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(switchover) |
||||
{ |
||||
// Test switchover code
|
||||
CScript notValid; |
||||
notValid << OP_11 << OP_12 << OP_EQUALVERIFY; |
||||
CScript scriptSig; |
||||
scriptSig << Serialize(notValid); |
||||
|
||||
CScript fund; |
||||
fund.SetPayToScriptHash(notValid); |
||||
|
||||
|
||||
// Validation should succeed under old rules (hash is correct):
|
||||
BOOST_CHECK(Verify(scriptSig, fund, false)); |
||||
// Fail under new:
|
||||
BOOST_CHECK(!Verify(scriptSig, fund, true)); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(AreInputsStandard) |
||||
{ |
||||
std::map<uint256, std::pair<CTxIndex, CTransaction> > mapInputs; |
||||
CBasicKeyStore keystore; |
||||
CKey key[3]; |
||||
vector<CKey> keys; |
||||
for (int i = 0; i < 3; i++) |
||||
{ |
||||
key[i].MakeNewKey(); |
||||
keystore.AddKey(key[i]); |
||||
keys.push_back(key[i]); |
||||
} |
||||
|
||||
CTransaction txFrom; |
||||
txFrom.vout.resize(5); |
||||
|
||||
// First three are standard:
|
||||
CScript pay1; pay1.SetBitcoinAddress(key[0].GetPubKey()); |
||||
keystore.AddCScript(pay1); |
||||
CScript payScriptHash1; payScriptHash1.SetPayToScriptHash(pay1); |
||||
CScript pay1of3; pay1of3.SetMultisig(1, keys); |
||||
|
||||
txFrom.vout[0].scriptPubKey = payScriptHash1; |
||||
txFrom.vout[1].scriptPubKey = pay1; |
||||
txFrom.vout[2].scriptPubKey = pay1of3; |
||||
|
||||
// Last two non-standard:
|
||||
CScript empty; |
||||
keystore.AddCScript(empty); |
||||
txFrom.vout[3].scriptPubKey = empty; |
||||
// Can't use SetPayToScriptHash, it checks for the empty Script. So:
|
||||
txFrom.vout[4].scriptPubKey << OP_HASH160 << Hash160(empty) << OP_EQUAL; |
||||
|
||||
mapInputs[txFrom.GetHash()] = make_pair(CTxIndex(), txFrom); |
||||
|
||||
CTransaction txTo; |
||||
txTo.vout.resize(1); |
||||
txTo.vout[0].scriptPubKey.SetBitcoinAddress(key[1].GetPubKey()); |
||||
|
||||
txTo.vin.resize(3); |
||||
txTo.vin[0].prevout.n = 0; |
||||
txTo.vin[0].prevout.hash = txFrom.GetHash(); |
||||
BOOST_CHECK(SignSignature(keystore, txFrom, txTo, 0)); |
||||
txTo.vin[1].prevout.n = 1; |
||||
txTo.vin[1].prevout.hash = txFrom.GetHash(); |
||||
BOOST_CHECK(SignSignature(keystore, txFrom, txTo, 1)); |
||||
txTo.vin[2].prevout.n = 2; |
||||
txTo.vin[2].prevout.hash = txFrom.GetHash(); |
||||
BOOST_CHECK(SignSignature(keystore, txFrom, txTo, 2)); |
||||
|
||||
BOOST_CHECK(txTo.AreInputsStandard(mapInputs)); |
||||
|
||||
CTransaction txToNonStd; |
||||
txToNonStd.vout.resize(1); |
||||
txToNonStd.vout[0].scriptPubKey.SetBitcoinAddress(key[1].GetPubKey()); |
||||
txToNonStd.vin.resize(1); |
||||
txToNonStd.vin[0].prevout.n = 4; |
||||
txToNonStd.vin[0].prevout.hash = txFrom.GetHash(); |
||||
txToNonStd.vin[0].scriptSig << Serialize(empty); |
||||
|
||||
BOOST_CHECK(!txToNonStd.AreInputsStandard(mapInputs)); |
||||
|
||||
txToNonStd.vin[0].scriptSig.clear(); |
||||
BOOST_CHECK(!txToNonStd.AreInputsStandard(mapInputs)); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_SUITE_END() |
@ -1,268 +0,0 @@
@@ -1,268 +0,0 @@
|
||||
#include <boost/assert.hpp> |
||||
#include <boost/assign/list_of.hpp> |
||||
#include <boost/assign/list_inserter.hpp> |
||||
#include <boost/assign/std/vector.hpp> |
||||
#include <boost/test/unit_test.hpp> |
||||
#include <boost/foreach.hpp> |
||||
|
||||
#include "../main.h" |
||||
#include "../script.h" |
||||
#include "../wallet.h" |
||||
|
||||
using namespace std; |
||||
|
||||
// Test routines internal to script.cpp:
|
||||
extern uint256 SignatureHash(CScript scriptCode, const CTransaction& txTo, unsigned int nIn, int nHashType); |
||||
extern bool VerifyScript(const CScript& scriptSig, const CScript& scriptPubKey, const CTransaction& txTo, unsigned int nIn, int& nSigOps, |
||||
int nHashType, bool fStrictOpEval); |
||||
|
||||
BOOST_AUTO_TEST_SUITE(script_op_eval_tests) |
||||
|
||||
BOOST_AUTO_TEST_CASE(script_op_eval1) |
||||
{ |
||||
// OP_EVAL looks like this:
|
||||
// scriptSig: <sig> <sig...> <serialized_script>
|
||||
// scriptPubKey: DUP HASH160 <hash> EQUALVERIFY EVAL
|
||||
|
||||
// Test SignSignature() (and therefore the version of Solver() that signs transactions)
|
||||
CBasicKeyStore keystore; |
||||
CKey key[4]; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
key[i].MakeNewKey(); |
||||
keystore.AddKey(key[i]); |
||||
} |
||||
|
||||
// 8 Scripts: checking all combinations of
|
||||
// different keys, straight/EVAL, pubkey/pubkeyhash
|
||||
CScript standardScripts[4]; |
||||
standardScripts[0] << key[0].GetPubKey() << OP_CHECKSIG; |
||||
standardScripts[1].SetBitcoinAddress(key[1].GetPubKey()); |
||||
standardScripts[2] << key[1].GetPubKey() << OP_CHECKSIG; |
||||
standardScripts[3].SetBitcoinAddress(key[2].GetPubKey()); |
||||
CScript evalScripts[4]; |
||||
uint160 sigScriptHashes[4]; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
sigScriptHashes[i] = Hash160(standardScripts[i]); |
||||
keystore.AddCScript(sigScriptHashes[i], standardScripts[i]); |
||||
evalScripts[i] << OP_DUP << OP_HASH160 << sigScriptHashes[i] << OP_EQUALVERIFY << OP_EVAL; |
||||
} |
||||
|
||||
CTransaction txFrom; // Funding transaction:
|
||||
txFrom.vout.resize(8); |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
txFrom.vout[i].scriptPubKey = evalScripts[i]; |
||||
txFrom.vout[i+4].scriptPubKey = standardScripts[i]; |
||||
} |
||||
BOOST_CHECK(txFrom.IsStandard()); |
||||
|
||||
CTransaction txTo[8]; // Spending transactions
|
||||
for (int i = 0; i < 8; i++) |
||||
{ |
||||
txTo[i].vin.resize(1); |
||||
txTo[i].vout.resize(1); |
||||
txTo[i].vin[0].prevout.n = i; |
||||
txTo[i].vin[0].prevout.hash = txFrom.GetHash(); |
||||
txTo[i].vout[0].nValue = 1; |
||||
BOOST_CHECK_MESSAGE(IsMine(keystore, txFrom.vout[i].scriptPubKey), strprintf("IsMine %d", i)); |
||||
} |
||||
for (int i = 0; i < 8; i++) |
||||
{ |
||||
BOOST_CHECK_MESSAGE(SignSignature(keystore, txFrom, txTo[i], 0), strprintf("SignSignature %d", i)); |
||||
} |
||||
// All of the above should be OK, and the txTos have valid signatures
|
||||
// Check to make sure signature verification fails if we use the wrong ScriptSig:
|
||||
for (int i = 0; i < 8; i++) |
||||
for (int j = 0; j < 8; j++) |
||||
{ |
||||
CScript sigSave = txTo[i].vin[0].scriptSig; |
||||
txTo[i].vin[0].scriptSig = txTo[j].vin[0].scriptSig; |
||||
int nUnused = 0; |
||||
bool sigOK = VerifySignature(txFrom, txTo[i], 0, nUnused); |
||||
if (i == j) |
||||
BOOST_CHECK_MESSAGE(sigOK, strprintf("VerifySignature %d %d", i, j)); |
||||
else |
||||
BOOST_CHECK_MESSAGE(!sigOK, strprintf("VerifySignature %d %d", i, j)); |
||||
txTo[i].vin[0].scriptSig = sigSave; |
||||
} |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(script_op_eval2) |
||||
{ |
||||
// Test OP_EVAL edge cases
|
||||
|
||||
// Make sure infinite recursion fails to validate:
|
||||
CScript infiniteRecurse; |
||||
infiniteRecurse << OP_DUP << OP_EVAL; |
||||
|
||||
uint160 infiniteRecurseHash = Hash160(infiniteRecurse); |
||||
|
||||
CScript fund1; |
||||
fund1 << OP_DUP << OP_HASH160 << infiniteRecurseHash << OP_EQUALVERIFY << OP_EVAL; |
||||
|
||||
CTransaction txFrom1; // Funding transaction:
|
||||
txFrom1.vout.resize(1); |
||||
txFrom1.vout[0].scriptPubKey = fund1; |
||||
|
||||
BOOST_CHECK(txFrom1.IsStandard()); // Looks like a standard transaction until you try to spend it
|
||||
|
||||
std::vector<unsigned char> infiniteRecurseSerialized(infiniteRecurse); |
||||
|
||||
CTransaction txTo1; |
||||
txTo1.vin.resize(1); |
||||
txTo1.vout.resize(1); |
||||
txTo1.vin[0].prevout.n = 0; |
||||
txTo1.vin[0].prevout.hash = txFrom1.GetHash(); |
||||
txTo1.vin[0].scriptSig = CScript() << infiniteRecurseSerialized << infiniteRecurseSerialized; |
||||
txTo1.vout[0].nValue = 1; |
||||
|
||||
int nUnused1 = 0; |
||||
BOOST_CHECK(!VerifyScript(txTo1.vin[0].scriptSig, txFrom1.vout[0].scriptPubKey, txTo1, 0, nUnused1, 0, true)); |
||||
BOOST_CHECK(!VerifySignature(txFrom1, txTo1, 0, nUnused1, true)); |
||||
|
||||
// Make sure 3-level-deep recursion fails to validate:
|
||||
CScript recurse3; |
||||
recurse3 << OP_EVAL; |
||||
|
||||
uint160 recurse3Hash = Hash160(recurse3); |
||||
|
||||
CScript fund2; |
||||
fund2 << OP_DUP << OP_HASH160 << recurse3Hash << OP_EQUALVERIFY << OP_EVAL; |
||||
|
||||
CTransaction txFrom2; // Funding transaction:
|
||||
txFrom2.vout.resize(1); |
||||
txFrom2.vout[0].scriptPubKey = fund2; |
||||
|
||||
BOOST_CHECK(txFrom2.IsStandard()); // Looks like a standard transaction until you try to spend it
|
||||
|
||||
std::vector<unsigned char> recurse3Serialized(recurse3); |
||||
CScript op1Script = CScript() << OP_1; |
||||
std::vector<unsigned char> op1Serialized(op1Script); |
||||
|
||||
CTransaction txTo2; |
||||
txTo2.vin.resize(1); |
||||
txTo2.vout.resize(1); |
||||
txTo2.vin[0].prevout.n = 0; |
||||
txTo2.vin[0].prevout.hash = txFrom2.GetHash(); |
||||
txTo2.vin[0].scriptSig = CScript() << op1Serialized << recurse3Serialized << recurse3Serialized; |
||||
txTo2.vout[0].nValue = 1; |
||||
|
||||
int nUnused2 = 0; |
||||
BOOST_CHECK(!VerifyScript(txTo2.vin[0].scriptSig, txFrom2.vout[0].scriptPubKey, txTo2, 0, nUnused2, 0, true)); |
||||
BOOST_CHECK(!VerifySignature(txFrom2, txTo2, 0, nUnused2, true)); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(script_op_eval3) |
||||
{ |
||||
// Test the CScript::Set* methods
|
||||
CBasicKeyStore keystore; |
||||
CKey key[4]; |
||||
std::vector<CKey> keys; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
key[i].MakeNewKey(); |
||||
keystore.AddKey(key[i]); |
||||
keys.push_back(key[i]); |
||||
} |
||||
|
||||
CScript inner[4]; |
||||
inner[0].SetBitcoinAddress(key[0].GetPubKey()); |
||||
inner[1].SetMultisig(2, std::vector<CKey>(keys.begin(), keys.begin()+2)); |
||||
inner[2].SetMultisig(1, std::vector<CKey>(keys.begin(), keys.begin()+2)); |
||||
inner[3].SetMultisig(2, std::vector<CKey>(keys.begin(), keys.begin()+3)); |
||||
|
||||
CScript outer[4]; |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
outer[i].SetEval(inner[i]); |
||||
keystore.AddCScript(Hash160(inner[i]), inner[i]); |
||||
} |
||||
|
||||
CTransaction txFrom; // Funding transaction:
|
||||
txFrom.vout.resize(4); |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
txFrom.vout[i].scriptPubKey = outer[i]; |
||||
} |
||||
BOOST_CHECK(txFrom.IsStandard()); |
||||
|
||||
CTransaction txTo[4]; // Spending transactions
|
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
txTo[i].vin.resize(1); |
||||
txTo[i].vout.resize(1); |
||||
txTo[i].vin[0].prevout.n = i; |
||||
txTo[i].vin[0].prevout.hash = txFrom.GetHash(); |
||||
txTo[i].vout[0].nValue = 1; |
||||
txTo[i].vout[0].scriptPubKey = inner[i]; |
||||
BOOST_CHECK_MESSAGE(IsMine(keystore, txFrom.vout[i].scriptPubKey), strprintf("IsMine %d", i)); |
||||
} |
||||
for (int i = 0; i < 4; i++) |
||||
{ |
||||
BOOST_CHECK_MESSAGE(SignSignature(keystore, txFrom, txTo[i], 0), strprintf("SignSignature %d", i)); |
||||
BOOST_CHECK_MESSAGE(txTo[i].IsStandard(), strprintf("txTo[%d].IsStandard", i)); |
||||
} |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(script_op_eval_backcompat1) |
||||
{ |
||||
// Check backwards-incompatibility-testing code
|
||||
CScript returnsEleven; |
||||
returnsEleven << OP_11; |
||||
|
||||
// This should validate on new clients, but will
|
||||
// be invalid on old clients (that interpret OP_EVAL as a no-op)
|
||||
// ... except there's a special rule that makes new clients reject
|
||||
// it.
|
||||
CScript fund; |
||||
fund << OP_EVAL << OP_11 << OP_EQUAL; |
||||
|
||||
CTransaction txFrom; // Funding transaction:
|
||||
txFrom.vout.resize(1); |
||||
txFrom.vout[0].scriptPubKey = fund; |
||||
|
||||
CTransaction txTo; |
||||
txTo.vin.resize(1); |
||||
txTo.vout.resize(1); |
||||
txTo.vin[0].prevout.n = 0; |
||||
txTo.vin[0].prevout.hash = txFrom.GetHash(); |
||||
txTo.vin[0].scriptSig = CScript() << static_cast<std::vector<unsigned char> >(returnsEleven); |
||||
txTo.vout[0].nValue = 1; |
||||
|
||||
int nUnused = 0; |
||||
BOOST_CHECK(!VerifyScript(txTo.vin[0].scriptSig, txFrom.vout[0].scriptPubKey, txTo, 0, nUnused, 0, true)); |
||||
BOOST_CHECK(!VerifySignature(txFrom, txTo, 0, nUnused, true)); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_CASE(script_op_eval_switchover) |
||||
{ |
||||
// Test OP_EVAL switchover code
|
||||
CScript notValid; |
||||
notValid << OP_11 << OP_12 << OP_EQUALVERIFY; |
||||
|
||||
// This will be valid under old rules, invalid under new:
|
||||
CScript fund; |
||||
fund << OP_EVAL; |
||||
|
||||
CTransaction txFrom; // Funding transaction:
|
||||
txFrom.vout.resize(1); |
||||
txFrom.vout[0].scriptPubKey = fund; |
||||
|
||||
CTransaction txTo; |
||||
txTo.vin.resize(1); |
||||
txTo.vout.resize(1); |
||||
txTo.vin[0].prevout.n = 0; |
||||
txTo.vin[0].prevout.hash = txFrom.GetHash(); |
||||
txTo.vin[0].scriptSig = CScript() << static_cast<std::vector<unsigned char> >(notValid); |
||||
txTo.vout[0].nValue = 1; |
||||
|
||||
int nUnused = 0; |
||||
BOOST_CHECK(VerifyScript(txTo.vin[0].scriptSig, txFrom.vout[0].scriptPubKey, txTo, 0, nUnused, 0, false)); |
||||
|
||||
// Under strict op_eval switchover, it should be considered invalid:
|
||||
BOOST_CHECK(!VerifyScript(txTo.vin[0].scriptSig, txFrom.vout[0].scriptPubKey, txTo, 0, nUnused, 0, true)); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_SUITE_END() |
@ -0,0 +1,60 @@
@@ -0,0 +1,60 @@
|
||||
#include <vector> |
||||
#include <boost/test/unit_test.hpp> |
||||
#include <boost/foreach.hpp> |
||||
|
||||
#include "script.h" |
||||
#include "key.h" |
||||
|
||||
using namespace std; |
||||
|
||||
// Helpers:
|
||||
static std::vector<unsigned char> |
||||
Serialize(const CScript& s) |
||||
{ |
||||
std::vector<unsigned char> sSerialized(s); |
||||
return sSerialized; |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_SUITE(sigopcount_tests) |
||||
|
||||
BOOST_AUTO_TEST_CASE(GetSigOpCount) |
||||
{ |
||||
// Test CScript::GetSigOpCount()
|
||||
CScript s1; |
||||
BOOST_CHECK_EQUAL(s1.GetSigOpCount(false), 0); |
||||
BOOST_CHECK_EQUAL(s1.GetSigOpCount(true), 0); |
||||
|
||||
uint160 dummy; |
||||
s1 << OP_1 << dummy << dummy << OP_2 << OP_CHECKMULTISIG; |
||||
BOOST_CHECK_EQUAL(s1.GetSigOpCount(true), 2); |
||||
s1 << OP_IF << OP_CHECKSIG << OP_ENDIF; |
||||
BOOST_CHECK_EQUAL(s1.GetSigOpCount(true), 3); |
||||
BOOST_CHECK_EQUAL(s1.GetSigOpCount(false), 21); |
||||
|
||||
CScript p2sh; |
||||
p2sh.SetPayToScriptHash(s1); |
||||
CScript scriptSig; |
||||
scriptSig << OP_0 << Serialize(s1); |
||||
BOOST_CHECK_EQUAL(p2sh.GetSigOpCount(scriptSig), 3); |
||||
|
||||
std::vector<CKey> keys; |
||||
for (int i = 0; i < 3; i++) |
||||
{ |
||||
CKey k; |
||||
k.MakeNewKey(); |
||||
keys.push_back(k); |
||||
} |
||||
CScript s2; |
||||
s2.SetMultisig(1, keys); |
||||
BOOST_CHECK_EQUAL(s2.GetSigOpCount(true), 3); |
||||
BOOST_CHECK_EQUAL(s2.GetSigOpCount(false), 20); |
||||
|
||||
p2sh.SetPayToScriptHash(s2); |
||||
BOOST_CHECK_EQUAL(p2sh.GetSigOpCount(true), 0); |
||||
BOOST_CHECK_EQUAL(p2sh.GetSigOpCount(false), 0); |
||||
CScript scriptSig2; |
||||
scriptSig2 << OP_1 << dummy << dummy << Serialize(s2); |
||||
BOOST_CHECK_EQUAL(p2sh.GetSigOpCount(scriptSig2), 3); |
||||
} |
||||
|
||||
BOOST_AUTO_TEST_SUITE_END() |
Loading…
Reference in new issue