Browse Source
This commit removes all the unnecessary dependencies (key, core, netbase, sync, ...) from bitcoin-cli. To do this it shards the chain parameters into BaseParams, which contains just the RPC port and data directory (as used by utils and bitcoin-cli) and Params, with the rest.0.10
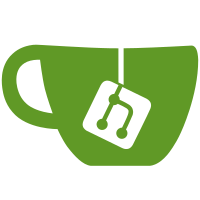
19 changed files with 232 additions and 89 deletions
@ -0,0 +1,93 @@
@@ -0,0 +1,93 @@
|
||||
// Copyright (c) 2010 Satoshi Nakamoto
|
||||
// Copyright (c) 2009-2014 The Bitcoin developers
|
||||
// Distributed under the MIT/X11 software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#include "chainparamsbase.h" |
||||
|
||||
#include "assert.h" |
||||
#include "util.h" |
||||
|
||||
#include <boost/assign/list_of.hpp> |
||||
|
||||
using namespace boost::assign; |
||||
|
||||
//
|
||||
// Main network
|
||||
//
|
||||
|
||||
class CBaseMainParams : public CBaseChainParams { |
||||
public: |
||||
CBaseMainParams() { |
||||
networkID = CBaseChainParams::MAIN; |
||||
nRPCPort = 8332; |
||||
} |
||||
}; |
||||
static CBaseMainParams mainParams; |
||||
|
||||
//
|
||||
// Testnet (v3)
|
||||
//
|
||||
class CBaseTestNetParams : public CBaseMainParams { |
||||
public: |
||||
CBaseTestNetParams() { |
||||
networkID = CBaseChainParams::TESTNET; |
||||
nRPCPort = 18332; |
||||
strDataDir = "testnet3"; |
||||
} |
||||
}; |
||||
static CBaseTestNetParams testNetParams; |
||||
|
||||
//
|
||||
// Regression test
|
||||
//
|
||||
class CBaseRegTestParams : public CBaseTestNetParams { |
||||
public: |
||||
CBaseRegTestParams() { |
||||
networkID = CBaseChainParams::REGTEST; |
||||
strDataDir = "regtest"; |
||||
} |
||||
}; |
||||
static CBaseRegTestParams regTestParams; |
||||
|
||||
static CBaseChainParams *pCurrentBaseParams = 0; |
||||
|
||||
const CBaseChainParams &BaseParams() { |
||||
assert(pCurrentBaseParams); |
||||
return *pCurrentBaseParams; |
||||
} |
||||
|
||||
void SelectBaseParams(CBaseChainParams::Network network) { |
||||
switch (network) { |
||||
case CBaseChainParams::MAIN: |
||||
pCurrentBaseParams = &mainParams; |
||||
break; |
||||
case CBaseChainParams::TESTNET: |
||||
pCurrentBaseParams = &testNetParams; |
||||
break; |
||||
case CBaseChainParams::REGTEST: |
||||
pCurrentBaseParams = ®TestParams; |
||||
break; |
||||
default: |
||||
assert(false && "Unimplemented network"); |
||||
return; |
||||
} |
||||
} |
||||
|
||||
bool SelectBaseParamsFromCommandLine() { |
||||
bool fRegTest = GetBoolArg("-regtest", false); |
||||
bool fTestNet = GetBoolArg("-testnet", false); |
||||
|
||||
if (fTestNet && fRegTest) { |
||||
return false; |
||||
} |
||||
|
||||
if (fRegTest) { |
||||
SelectBaseParams(CBaseChainParams::REGTEST); |
||||
} else if (fTestNet) { |
||||
SelectBaseParams(CBaseChainParams::TESTNET); |
||||
} else { |
||||
SelectBaseParams(CBaseChainParams::MAIN); |
||||
} |
||||
return true; |
||||
} |
@ -0,0 +1,52 @@
@@ -0,0 +1,52 @@
|
||||
// Copyright (c) 2014 The Bitcoin developers
|
||||
// Distributed under the MIT/X11 software license, see the accompanying
|
||||
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
||||
|
||||
#ifndef BITCOIN_CHAIN_PARAMS_BASE_H |
||||
#define BITCOIN_CHAIN_PARAMS_BASE_H |
||||
|
||||
#include <vector> |
||||
#include <string> |
||||
|
||||
/**
|
||||
* CBaseChainParams defines the base parameters (shared between bitcoin-cli and bitcoind) |
||||
* of a given instance of the Bitcoin system. |
||||
*/ |
||||
class CBaseChainParams |
||||
{ |
||||
public: |
||||
enum Network { |
||||
MAIN, |
||||
TESTNET, |
||||
REGTEST, |
||||
|
||||
MAX_NETWORK_TYPES |
||||
}; |
||||
|
||||
const std::string& DataDir() const { return strDataDir; } |
||||
int RPCPort() const { return nRPCPort; } |
||||
Network NetworkID() const { return networkID; } |
||||
protected: |
||||
CBaseChainParams() {} |
||||
|
||||
int nRPCPort; |
||||
std::string strDataDir; |
||||
Network networkID; |
||||
}; |
||||
|
||||
/**
|
||||
* Return the currently selected parameters. This won't change after app startup |
||||
* outside of the unit tests. |
||||
*/ |
||||
const CBaseChainParams &BaseParams(); |
||||
|
||||
/** Sets the params returned by Params() to those for the given network. */ |
||||
void SelectBaseParams(CBaseChainParams::Network network); |
||||
|
||||
/**
|
||||
* Looks for -regtest or -testnet and then calls SelectParams as appropriate. |
||||
* Returns false if an invalid combination is given. |
||||
*/ |
||||
bool SelectBaseParamsFromCommandLine(); |
||||
|
||||
#endif |
Loading…
Reference in new issue