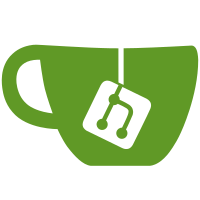
9 changed files with 52 additions and 74 deletions
@ -1,13 +0,0 @@
@@ -1,13 +0,0 @@
|
||||
#include <stdint.h> |
||||
#include "stdbool.h" |
||||
#include <stdio.h> |
||||
#include <stdlib.h> |
||||
#include "cnutilxx/main.h" |
||||
|
||||
uint32_t convert_blob(const char *blob, size_t len, char *out) { |
||||
return cn_convert_blob(blob, len, out); |
||||
} |
||||
|
||||
bool validate_address(const char *addr, size_t len) { |
||||
return cn_validate_address(addr, len); |
||||
} |
@ -1,12 +0,0 @@
@@ -1,12 +0,0 @@
|
||||
#include <stdint.h> |
||||
|
||||
#ifdef __cplusplus |
||||
extern "C" { |
||||
#endif |
||||
|
||||
uint32_t cn_convert_blob(const char *blob, uint32_t len, char *out); |
||||
bool cn_validate_address(const char *addr, uint32_t len); |
||||
|
||||
#ifdef __cplusplus |
||||
} |
||||
#endif |
@ -1,7 +1,13 @@
@@ -1,7 +1,13 @@
|
||||
#include <stdio.h> |
||||
#include <stdint.h> |
||||
#include <stdlib.h> |
||||
#include "stdbool.h" |
||||
|
||||
#ifdef __cplusplus |
||||
extern "C" { |
||||
#endif |
||||
|
||||
uint32_t convert_blob(const char *blob, uint32_t len, char *out); |
||||
bool validate_address(const char *addr, uint32_t len); |
||||
|
||||
#ifdef __cplusplus |
||||
} |
||||
#endif |
Loading…
Reference in new issue