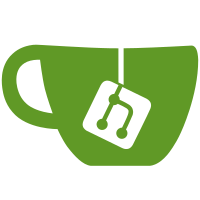
10 changed files with 140 additions and 16 deletions
@ -1,20 +1,11 @@
@@ -1,20 +1,11 @@
|
||||
cmake_minimum_required (VERSION 2.8.11) |
||||
project(pool) |
||||
|
||||
if (DEFINED ENV{MONERO_DIR}) |
||||
get_filename_component(MONERO_DIR $ENV{MONERO_DIR} ABSOLUTE) |
||||
message("Using Monero source from env ${MONERO_DIR}") |
||||
else() |
||||
get_filename_component(MONERO_DIR "${CMAKE_SOURCE_DIR}/../monero" ABSOLUTE) |
||||
message("Monero surce directory is not defined, using default ${MONERO_DIR}") |
||||
endif() |
||||
|
||||
add_subdirectory(hashing) |
||||
add_subdirectory(cnutil) |
||||
|
||||
add_custom_command( |
||||
OUTPUT build/bin |
||||
COMMAND build/env.sh go get -v ./... |
||||
) |
||||
|
||||
add_custom_target(build ALL DEPENDS hashing cnutil ${CMAKE_CURRENT_BINARY_DIR}/build/bin) |
||||
add_custom_target(build ALL DEPENDS hashing ${CMAKE_CURRENT_BINARY_DIR}/build/bin) |
||||
|
@ -0,0 +1,5 @@
@@ -0,0 +1,5 @@
|
||||
Makefile |
||||
CmakeCache.txt |
||||
cmake_install.cmake |
||||
CMakeFiles |
||||
libhashing.a |
@ -0,0 +1,6 @@
@@ -0,0 +1,6 @@
|
||||
set(LIB "hashing") |
||||
|
||||
set(CMAKE_C_FLAGS "${CMAKE_C_FLAGS} -std=c11 -D_GNU_SOURCE") |
||||
|
||||
add_library(${LIB} src/hashing.c) |
||||
|
@ -0,0 +1,23 @@
@@ -0,0 +1,23 @@
|
||||
package hashing |
||||
|
||||
// #cgo CFLAGS: -std=c11 -D_GNU_SOURCE
|
||||
// #cgo LDFLAGS: -L${SRCDIR} -lhashing -Wl,-rpath ${SRCDIR} -lstdc++
|
||||
// #include <stdlib.h>
|
||||
// #include <stdint.h>
|
||||
// #include "src/hashing.h"
|
||||
import "C" |
||||
import "unsafe" |
||||
|
||||
func Hash(blob []byte, fast bool) []byte { |
||||
output := make([]byte, 32) |
||||
if fast { |
||||
C.cryptonight_fast_hash((*C.char)(unsafe.Pointer(&blob[0])), (*C.char)(unsafe.Pointer(&output[0])), (C.uint32_t)(len(blob))) |
||||
} else { |
||||
C.cryptonight_hash((*C.char)(unsafe.Pointer(&blob[0])), (*C.char)(unsafe.Pointer(&output[0])), (C.uint32_t)(len(blob))) |
||||
} |
||||
return output |
||||
} |
||||
|
||||
func FastHash(blob []byte) []byte { |
||||
return Hash(append([]byte{byte(len(blob))}, blob...), true) |
||||
} |
@ -0,0 +1,82 @@
@@ -0,0 +1,82 @@
|
||||
package hashing |
||||
|
||||
import "testing" |
||||
import "log" |
||||
import "encoding/hex" |
||||
|
||||
func TestHash(t *testing.T) { |
||||
blob, _ := hex.DecodeString("01009091e4aa05ff5fe4801727ed0c1b8b339e1a0054d75568fec6ba9c4346e88b10d59edbf6858b2b00008a63b2865b65b84d28bb31feb057b16a21e2eda4bf6cc6377e3310af04debe4a01") |
||||
hashBytes := Hash(blob, false) |
||||
hash := hex.EncodeToString(hashBytes) |
||||
log.Println(hash) |
||||
|
||||
expectedHash := "a70a96f64a266f0f59e4f67c4a92f24fe8237c1349f377fd2720c9e1f2970400" |
||||
|
||||
if hash != expectedHash { |
||||
t.Error("Invalid hash") |
||||
} |
||||
} |
||||
|
||||
func TestHash_fast(t *testing.T) { |
||||
blob, _ := hex.DecodeString("01009091e4aa05ff5fe4801727ed0c1b8b339e1a0054d75568fec6ba9c4346e88b10d59edbf6858b2b00008a63b2865b65b84d28bb31feb057b16a21e2eda4bf6cc6377e3310af04debe4a01") |
||||
hashBytes := Hash(blob, true) |
||||
hash := hex.EncodeToString(hashBytes) |
||||
log.Println(hash) |
||||
|
||||
expectedHash := "7591f4d8ff9d86ea44873e89a5fb6f380f4410be6206030010567ac9d0d4b0e1" |
||||
|
||||
if hash != expectedHash { |
||||
t.Error("Invalid fast hash") |
||||
} |
||||
} |
||||
|
||||
func TestFastHash(t *testing.T) { |
||||
blob, _ := hex.DecodeString("01009091e4aa05ff5fe4801727ed0c1b8b339e1a0054d75568fec6ba9c4346e88b10d59edbf6858b2b00008a63b2865b65b84d28bb31feb057b16a21e2eda4bf6cc6377e3310af04debe4a01") |
||||
hashBytes := FastHash(blob) |
||||
hash := hex.EncodeToString(hashBytes) |
||||
log.Println(hash) |
||||
|
||||
expectedHash := "8706c697d9fc8a48b14ea93a31c6f0750c48683e585ec1a534e9c57c97193fa6" |
||||
|
||||
if hash != expectedHash { |
||||
t.Error("Invalid fast hash") |
||||
} |
||||
} |
||||
|
||||
func BenchmarkHash(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
for i := 0; i < b.N; i++ { |
||||
Hash(blob, false) |
||||
} |
||||
} |
||||
|
||||
func BenchmarkHashParallel(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
b.RunParallel(func(pb *testing.PB) { |
||||
for pb.Next() { |
||||
Hash(blob, false) |
||||
} |
||||
}) |
||||
} |
||||
|
||||
func BenchmarkHash_fast(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
for i := 0; i < b.N; i++ { |
||||
Hash(blob, true) |
||||
} |
||||
} |
||||
|
||||
func BenchmarkFastHash(b *testing.B) { |
||||
blob, _ := hex.DecodeString("0100b69bb3aa050a3106491f858f8646d3a8d13fd9924403bf07af95e6e7cc9e4ad105d76da27241565555866b1baa9db8f027cf57cd45d6835c11287b210d9ddb407deda565f8112e19e501") |
||||
b.ResetTimer() |
||||
|
||||
for i := 0; i < b.N; i++ { |
||||
FastHash(blob) |
||||
} |
||||
} |
@ -0,0 +1,13 @@
@@ -0,0 +1,13 @@
|
||||
#include <stdlib.h> |
||||
#include <stdint.h> |
||||
#include "hashing.h" |
||||
|
||||
void cryptonight_hash(const char* input, char* output, uint32_t len) { |
||||
//const int variant = input[0] >= 7 ? input[0] - 6 : 0;
|
||||
//cn_slow_hash(input, len, output, variant, 0);
|
||||
} |
||||
|
||||
void cryptonight_fast_hash(const char* input, char* output, uint32_t len) { |
||||
//cn_fast_hash(input, len, output);
|
||||
} |
||||
|
@ -0,0 +1,3 @@
@@ -0,0 +1,3 @@
|
||||
|
||||
void cryptonight_hash(const char* input, char* output, uint32_t len); |
||||
void cryptonight_fast_hash(const char* input, char* output, uint32_t len); |
Loading…
Reference in new issue