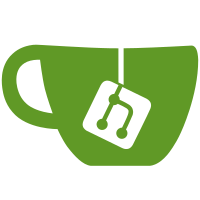
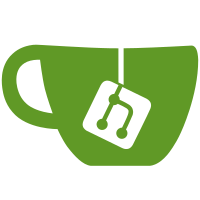
42 changed files with 931 additions and 637 deletions
@ -0,0 +1,217 @@
@@ -0,0 +1,217 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt and libtorrent. |
||||
* Copyright (C) 2015-2023 Vladimir Golovnev <glassez@yandex.ru> |
||||
* Copyright (C) 2006 Christophe Dumez <chris@qbittorrent.org> |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
*/ |
||||
|
||||
#include "addtorrentmanager.h" |
||||
|
||||
#include "base/bittorrent/infohash.h" |
||||
#include "base/bittorrent/session.h" |
||||
#include "base/bittorrent/torrentdescriptor.h" |
||||
#include "base/logger.h" |
||||
#include "base/net/downloadmanager.h" |
||||
#include "base/preferences.h" |
||||
|
||||
AddTorrentManager::AddTorrentManager(IApplication *app, BitTorrent::Session *btSession, QObject *parent) |
||||
: ApplicationComponent(app, parent) |
||||
, m_btSession {btSession} |
||||
{ |
||||
Q_ASSERT(btSession); |
||||
connect(btSession, &BitTorrent::Session::torrentAdded, this, &AddTorrentManager::onSessionTorrentAdded); |
||||
connect(btSession, &BitTorrent::Session::addTorrentFailed, this, &AddTorrentManager::onSessionAddTorrentFailed); |
||||
} |
||||
|
||||
BitTorrent::Session *AddTorrentManager::btSession() const |
||||
{ |
||||
return m_btSession; |
||||
} |
||||
|
||||
bool AddTorrentManager::addTorrent(const QString &source, const BitTorrent::AddTorrentParams ¶ms) |
||||
{ |
||||
// `source`: .torrent file path, magnet URI or URL
|
||||
|
||||
if (source.isEmpty()) |
||||
return false; |
||||
|
||||
if (Net::DownloadManager::hasSupportedScheme(source)) |
||||
{ |
||||
LogMsg(tr("Downloading torrent... Source: \"%1\"").arg(source)); |
||||
const auto *pref = Preferences::instance(); |
||||
// Launch downloader
|
||||
Net::DownloadManager::instance()->download(Net::DownloadRequest(source).limit(pref->getTorrentFileSizeLimit()) |
||||
, pref->useProxyForGeneralPurposes(), this, &AddTorrentManager::onDownloadFinished); |
||||
m_downloadedTorrents[source] = params; |
||||
return true; |
||||
} |
||||
|
||||
if (const auto parseResult = BitTorrent::TorrentDescriptor::parse(source)) |
||||
{ |
||||
return processTorrent(source, parseResult.value(), params); |
||||
} |
||||
else if (source.startsWith(u"magnet:", Qt::CaseInsensitive)) |
||||
{ |
||||
handleAddTorrentFailed(source, parseResult.error()); |
||||
return false; |
||||
} |
||||
|
||||
const Path decodedPath {source.startsWith(u"file://", Qt::CaseInsensitive) |
||||
? QUrl::fromEncoded(source.toLocal8Bit()).toLocalFile() : source}; |
||||
auto torrentFileGuard = std::make_shared<TorrentFileGuard>(decodedPath); |
||||
if (const auto loadResult = BitTorrent::TorrentDescriptor::loadFromFile(decodedPath)) |
||||
{ |
||||
setTorrentFileGuard(source, torrentFileGuard); |
||||
return processTorrent(source, loadResult.value(), params); |
||||
} |
||||
else |
||||
{ |
||||
handleAddTorrentFailed(source, loadResult.error()); |
||||
return false; |
||||
} |
||||
|
||||
return false; |
||||
} |
||||
|
||||
bool AddTorrentManager::addTorrentToSession(const QString &source, const BitTorrent::TorrentDescriptor &torrentDescr |
||||
, const BitTorrent::AddTorrentParams &addTorrentParams) |
||||
{ |
||||
const bool result = btSession()->addTorrent(torrentDescr, addTorrentParams); |
||||
if (result) |
||||
m_sourcesByInfoHash[torrentDescr.infoHash()] = source; |
||||
|
||||
return result; |
||||
} |
||||
|
||||
void AddTorrentManager::onDownloadFinished(const Net::DownloadResult &result) |
||||
{ |
||||
const QString &source = result.url; |
||||
const BitTorrent::AddTorrentParams addTorrentParams = m_downloadedTorrents.take(source); |
||||
|
||||
switch (result.status) |
||||
{ |
||||
case Net::DownloadStatus::Success: |
||||
if (const auto loadResult = BitTorrent::TorrentDescriptor::load(result.data)) |
||||
processTorrent(source, loadResult.value(), addTorrentParams); |
||||
else |
||||
handleAddTorrentFailed(source, loadResult.error()); |
||||
break; |
||||
case Net::DownloadStatus::RedirectedToMagnet: |
||||
if (const auto parseResult = BitTorrent::TorrentDescriptor::parse(result.magnetURI)) |
||||
processTorrent(source, parseResult.value(), addTorrentParams); |
||||
else |
||||
handleAddTorrentFailed(source, parseResult.error()); |
||||
break; |
||||
default: |
||||
handleAddTorrentFailed(source, result.errorString); |
||||
} |
||||
} |
||||
|
||||
void AddTorrentManager::onSessionTorrentAdded(BitTorrent::Torrent *torrent) |
||||
{ |
||||
if (const QString source = m_sourcesByInfoHash.take(torrent->infoHash()); !source.isEmpty()) |
||||
{ |
||||
auto torrentFileGuard = m_guardedTorrentFiles.take(source); |
||||
if (torrentFileGuard) |
||||
torrentFileGuard->markAsAddedToSession(); |
||||
emit torrentAdded(source, torrent); |
||||
} |
||||
} |
||||
|
||||
void AddTorrentManager::onSessionAddTorrentFailed(const BitTorrent::InfoHash &infoHash, const QString &reason) |
||||
{ |
||||
if (const QString source = m_sourcesByInfoHash.take(infoHash); !source.isEmpty()) |
||||
{ |
||||
auto torrentFileGuard = m_guardedTorrentFiles.take(source); |
||||
if (torrentFileGuard) |
||||
torrentFileGuard->setAutoRemove(false); |
||||
emit addTorrentFailed(source, reason); |
||||
} |
||||
} |
||||
|
||||
void AddTorrentManager::handleAddTorrentFailed(const QString &source, const QString &reason) |
||||
{ |
||||
LogMsg(tr("Failed to add torrent. Source: \"%1\". Reason: \"%2\"").arg(source, reason), Log::WARNING); |
||||
emit addTorrentFailed(source, reason); |
||||
} |
||||
|
||||
void AddTorrentManager::handleDuplicateTorrent(const QString &source, BitTorrent::Torrent *torrent, const QString &message) |
||||
{ |
||||
LogMsg(tr("Detected an attempt to add a duplicate torrent. Source: %1. Existing torrent: %2. Result: %3") |
||||
.arg(source, torrent->name(), message)); |
||||
emit addTorrentFailed(source, message); |
||||
} |
||||
|
||||
void AddTorrentManager::setTorrentFileGuard(const QString &source, std::shared_ptr<TorrentFileGuard> torrentFileGuard) |
||||
{ |
||||
m_guardedTorrentFiles.emplace(source, std::move(torrentFileGuard)); |
||||
} |
||||
|
||||
void AddTorrentManager::releaseTorrentFileGuard(const QString &source) |
||||
{ |
||||
auto torrentFileGuard = m_guardedTorrentFiles.take(source); |
||||
if (torrentFileGuard) |
||||
torrentFileGuard->setAutoRemove(false); |
||||
} |
||||
|
||||
bool AddTorrentManager::processTorrent(const QString &source, const BitTorrent::TorrentDescriptor &torrentDescr |
||||
, const BitTorrent::AddTorrentParams &addTorrentParams) |
||||
{ |
||||
const BitTorrent::InfoHash infoHash = torrentDescr.infoHash(); |
||||
|
||||
if (BitTorrent::Torrent *torrent = btSession()->findTorrent(infoHash)) |
||||
{ |
||||
// a duplicate torrent is being added
|
||||
|
||||
const bool hasMetadata = torrentDescr.info().has_value(); |
||||
if (hasMetadata) |
||||
{ |
||||
// Trying to set metadata to existing torrent in case if it has none
|
||||
torrent->setMetadata(*torrentDescr.info()); |
||||
} |
||||
|
||||
if (!btSession()->isMergeTrackersEnabled()) |
||||
{ |
||||
handleDuplicateTorrent(source, torrent, tr("Merging of trackers is disabled")); |
||||
return false; |
||||
} |
||||
|
||||
const bool isPrivate = torrent->isPrivate() || (hasMetadata && torrentDescr.info()->isPrivate()); |
||||
if (isPrivate) |
||||
{ |
||||
handleDuplicateTorrent(source, torrent, tr("Trackers cannot be merged because it is a private torrent")); |
||||
return false; |
||||
} |
||||
|
||||
// merge trackers and web seeds
|
||||
torrent->addTrackers(torrentDescr.trackers()); |
||||
torrent->addUrlSeeds(torrentDescr.urlSeeds()); |
||||
|
||||
handleDuplicateTorrent(source, torrent, tr("Trackers are merged from new source")); |
||||
return false; |
||||
} |
||||
|
||||
return addTorrentToSession(source, torrentDescr, addTorrentParams); |
||||
} |
@ -0,0 +1,86 @@
@@ -0,0 +1,86 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt and libtorrent. |
||||
* Copyright (C) 2015-2023 Vladimir Golovnev <glassez@yandex.ru> |
||||
* Copyright (C) 2006 Christophe Dumez <chris@qbittorrent.org> |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
*/ |
||||
|
||||
#pragma once |
||||
|
||||
#include <memory> |
||||
|
||||
#include <QHash> |
||||
#include <QObject> |
||||
|
||||
#include "base/applicationcomponent.h" |
||||
#include "base/bittorrent/addtorrentparams.h" |
||||
#include "base/torrentfileguard.h" |
||||
|
||||
namespace Net |
||||
{ |
||||
struct DownloadResult; |
||||
} |
||||
|
||||
namespace BitTorrent |
||||
{ |
||||
class Session; |
||||
class TorrentDescriptor; |
||||
} |
||||
|
||||
class AddTorrentManager : public ApplicationComponent<QObject> |
||||
{ |
||||
Q_OBJECT |
||||
Q_DISABLE_COPY_MOVE(AddTorrentManager) |
||||
|
||||
public: |
||||
AddTorrentManager(IApplication *app, BitTorrent::Session *btSession, QObject *parent = nullptr); |
||||
|
||||
BitTorrent::Session *btSession() const; |
||||
bool addTorrent(const QString &source, const BitTorrent::AddTorrentParams ¶ms = {}); |
||||
|
||||
signals: |
||||
void torrentAdded(const QString &source, BitTorrent::Torrent *torrent); |
||||
void addTorrentFailed(const QString &source, const QString &reason); |
||||
|
||||
protected: |
||||
bool addTorrentToSession(const QString &source, const BitTorrent::TorrentDescriptor &torrentDescr |
||||
, const BitTorrent::AddTorrentParams &addTorrentParams); |
||||
void handleAddTorrentFailed(const QString &source, const QString &reason); |
||||
void handleDuplicateTorrent(const QString &source, BitTorrent::Torrent *torrent, const QString &message); |
||||
void setTorrentFileGuard(const QString &source, std::shared_ptr<TorrentFileGuard> torrentFileGuard); |
||||
void releaseTorrentFileGuard(const QString &source); |
||||
|
||||
private: |
||||
void onDownloadFinished(const Net::DownloadResult &result); |
||||
void onSessionTorrentAdded(BitTorrent::Torrent *torrent); |
||||
void onSessionAddTorrentFailed(const BitTorrent::InfoHash &infoHash, const QString &reason); |
||||
bool processTorrent(const QString &source, const BitTorrent::TorrentDescriptor &torrentDescr |
||||
, const BitTorrent::AddTorrentParams &addTorrentParams); |
||||
|
||||
BitTorrent::Session *m_btSession = nullptr; |
||||
QHash<QString, BitTorrent::AddTorrentParams> m_downloadedTorrents; |
||||
QHash<BitTorrent::InfoHash, QString> m_sourcesByInfoHash; |
||||
QHash<QString, std::shared_ptr<TorrentFileGuard>> m_guardedTorrentFiles; |
||||
}; |
@ -0,0 +1,200 @@
@@ -0,0 +1,200 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt and libtorrent. |
||||
* Copyright (C) 2015-2023 Vladimir Golovnev <glassez@yandex.ru> |
||||
* Copyright (C) 2006 Christophe Dumez <chris@qbittorrent.org> |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
*/ |
||||
|
||||
#include "guiaddtorrentmanager.h" |
||||
|
||||
#include "base/bittorrent/session.h" |
||||
#include "base/bittorrent/torrentdescriptor.h" |
||||
#include "base/logger.h" |
||||
#include "base/net/downloadmanager.h" |
||||
#include "base/preferences.h" |
||||
#include "base/torrentfileguard.h" |
||||
#include "addnewtorrentdialog.h" |
||||
#include "interfaces/iguiapplication.h" |
||||
#include "mainwindow.h" |
||||
#include "raisedmessagebox.h" |
||||
|
||||
GUIAddTorrentManager::GUIAddTorrentManager(IGUIApplication *app, BitTorrent::Session *session, QObject *parent) |
||||
: GUIApplicationComponent(app, session, parent) |
||||
{ |
||||
connect(btSession(), &BitTorrent::Session::metadataDownloaded, this, &GUIAddTorrentManager::onMetadataDownloaded); |
||||
} |
||||
|
||||
bool GUIAddTorrentManager::addTorrent(const QString &source, const BitTorrent::AddTorrentParams ¶ms, const AddTorrentOption option) |
||||
{ |
||||
// `source`: .torrent file path, magnet URI or URL
|
||||
|
||||
if (source.isEmpty()) |
||||
return false; |
||||
|
||||
const auto *pref = Preferences::instance(); |
||||
|
||||
if ((option == AddTorrentOption::SkipDialog) |
||||
|| ((option == AddTorrentOption::Default) && !pref->isAddNewTorrentDialogEnabled())) |
||||
{ |
||||
return AddTorrentManager::addTorrent(source, params); |
||||
} |
||||
|
||||
if (Net::DownloadManager::hasSupportedScheme(source)) |
||||
{ |
||||
LogMsg(tr("Downloading torrent... Source: \"%1\"").arg(source)); |
||||
// Launch downloader
|
||||
Net::DownloadManager::instance()->download(Net::DownloadRequest(source).limit(pref->getTorrentFileSizeLimit()) |
||||
, pref->useProxyForGeneralPurposes(), this, &GUIAddTorrentManager::onDownloadFinished); |
||||
m_downloadedTorrents[source] = params; |
||||
|
||||
return true; |
||||
} |
||||
|
||||
if (const auto parseResult = BitTorrent::TorrentDescriptor::parse(source)) |
||||
{ |
||||
return processTorrent(source, parseResult.value(), params); |
||||
} |
||||
else if (source.startsWith(u"magnet:", Qt::CaseInsensitive)) |
||||
{ |
||||
handleAddTorrentFailed(source, parseResult.error()); |
||||
return false; |
||||
} |
||||
|
||||
const Path decodedPath {source.startsWith(u"file://", Qt::CaseInsensitive) |
||||
? QUrl::fromEncoded(source.toLocal8Bit()).toLocalFile() : source}; |
||||
auto torrentFileGuard = std::make_shared<TorrentFileGuard>(decodedPath); |
||||
if (const auto loadResult = BitTorrent::TorrentDescriptor::loadFromFile(decodedPath)) |
||||
{ |
||||
const BitTorrent::TorrentDescriptor &torrentDescriptor = loadResult.value(); |
||||
const bool isProcessing = processTorrent(source, torrentDescriptor, params); |
||||
if (isProcessing) |
||||
setTorrentFileGuard(source, torrentFileGuard); |
||||
return isProcessing; |
||||
} |
||||
else |
||||
{ |
||||
handleAddTorrentFailed(decodedPath.toString(), loadResult.error()); |
||||
return false; |
||||
} |
||||
|
||||
return false; |
||||
} |
||||
|
||||
void GUIAddTorrentManager::onDownloadFinished(const Net::DownloadResult &result) |
||||
{ |
||||
const QString &source = result.url; |
||||
const BitTorrent::AddTorrentParams addTorrentParams = m_downloadedTorrents.take(source); |
||||
|
||||
switch (result.status) |
||||
{ |
||||
case Net::DownloadStatus::Success: |
||||
if (const auto loadResult = BitTorrent::TorrentDescriptor::load(result.data)) |
||||
processTorrent(source, loadResult.value(), addTorrentParams); |
||||
else |
||||
handleAddTorrentFailed(source, loadResult.error()); |
||||
break; |
||||
case Net::DownloadStatus::RedirectedToMagnet: |
||||
if (const auto parseResult = BitTorrent::TorrentDescriptor::parse(result.magnetURI)) |
||||
processTorrent(source, parseResult.value(), addTorrentParams); |
||||
else |
||||
handleAddTorrentFailed(source, parseResult.error()); |
||||
break; |
||||
default: |
||||
handleAddTorrentFailed(source, result.errorString); |
||||
} |
||||
} |
||||
|
||||
void GUIAddTorrentManager::onMetadataDownloaded(const BitTorrent::TorrentInfo &metadata) |
||||
{ |
||||
Q_ASSERT(metadata.isValid()); |
||||
if (!metadata.isValid()) [[unlikely]] |
||||
return; |
||||
|
||||
for (const auto &[infoHash, dialog] : m_dialogs.asKeyValueRange()) |
||||
{ |
||||
if (metadata.matchesInfoHash(infoHash)) |
||||
dialog->updateMetadata(metadata); |
||||
} |
||||
} |
||||
|
||||
bool GUIAddTorrentManager::processTorrent(const QString &source, const BitTorrent::TorrentDescriptor &torrentDescr, const BitTorrent::AddTorrentParams ¶ms) |
||||
{ |
||||
const bool hasMetadata = torrentDescr.info().has_value(); |
||||
const BitTorrent::InfoHash infoHash = torrentDescr.infoHash(); |
||||
|
||||
// Prevent showing the dialog if download is already present
|
||||
if (BitTorrent::Torrent *torrent = btSession()->findTorrent(infoHash)) |
||||
{ |
||||
if (hasMetadata) |
||||
{ |
||||
// Trying to set metadata to existing torrent in case if it has none
|
||||
torrent->setMetadata(*torrentDescr.info()); |
||||
} |
||||
|
||||
if (torrent->isPrivate() || (hasMetadata && torrentDescr.info()->isPrivate())) |
||||
{ |
||||
handleDuplicateTorrent(source, torrent, tr("Trackers cannot be merged because it is a private torrent")); |
||||
} |
||||
else |
||||
{ |
||||
bool mergeTrackers = btSession()->isMergeTrackersEnabled(); |
||||
if (Preferences::instance()->confirmMergeTrackers()) |
||||
{ |
||||
const QMessageBox::StandardButton btn = RaisedMessageBox::question(app()->mainWindow(), tr("Torrent is already present") |
||||
, tr("Torrent '%1' is already in the transfer list. Do you want to merge trackers from new source?").arg(torrent->name()) |
||||
, (QMessageBox::Yes | QMessageBox::No), QMessageBox::Yes); |
||||
mergeTrackers = (btn == QMessageBox::Yes); |
||||
} |
||||
|
||||
if (mergeTrackers) |
||||
{ |
||||
torrent->addTrackers(torrentDescr.trackers()); |
||||
torrent->addUrlSeeds(torrentDescr.urlSeeds()); |
||||
} |
||||
} |
||||
|
||||
return false; |
||||
} |
||||
|
||||
if (!hasMetadata) |
||||
btSession()->downloadMetadata(torrentDescr); |
||||
|
||||
auto *dlg = new AddNewTorrentDialog(torrentDescr, params, app()->mainWindow()); |
||||
dlg->setAttribute(Qt::WA_DeleteOnClose); |
||||
m_dialogs[infoHash] = dlg; |
||||
connect(dlg, &QDialog::finished, this, [this, source, infoHash, dlg](int result) |
||||
{ |
||||
if (dlg->isDoNotDeleteTorrentChecked()) |
||||
releaseTorrentFileGuard(source); |
||||
|
||||
if (result == QDialog::Accepted) |
||||
addTorrentToSession(source, dlg->torrentDescriptor(), dlg->addTorrentParams()); |
||||
|
||||
m_dialogs.remove(infoHash); |
||||
}); |
||||
dlg->show(); |
||||
|
||||
return true; |
||||
} |
Loading…
Reference in new issue