Browse Source
The new TriStateAction class is an improvement of the old one in the sense that: 1. Have public method to set states. 2. Can connect to the usual Qt slots. 3. Draws checkbox at the correct offset (where QAction draws) in menu and better handling of mouse clicking and keyboard navigating.adaptive-webui-19844
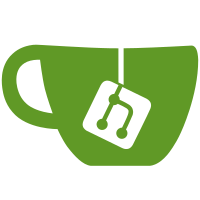
8 changed files with 396 additions and 188 deletions
@ -0,0 +1,144 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2019 Mike Tzou (Chocobo1) |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "tristatewidget.h" |
||||||
|
|
||||||
|
#include <QApplication> |
||||||
|
#include <QMouseEvent> |
||||||
|
#include <QPainter> |
||||||
|
#include <QPaintEvent> |
||||||
|
#include <QString> |
||||||
|
#include <QStyle> |
||||||
|
#include <QStyleOptionMenuItem> |
||||||
|
|
||||||
|
TriStateWidget::TriStateWidget(const QString &text, QWidget *parent) |
||||||
|
: QWidget {parent} |
||||||
|
, m_closeOnTriggered {true} |
||||||
|
, m_checkState {Qt::Unchecked} |
||||||
|
, m_text {text} |
||||||
|
{ |
||||||
|
setMouseTracking(true); // for visual effects via mouse navigation
|
||||||
|
setFocusPolicy(Qt::TabFocus); // for visual effects via keyboard navigation
|
||||||
|
} |
||||||
|
|
||||||
|
void TriStateWidget::setCheckState(const Qt::CheckState checkState) |
||||||
|
{ |
||||||
|
m_checkState = checkState; |
||||||
|
} |
||||||
|
|
||||||
|
void TriStateWidget::setCloseOnTriggered(const bool enabled) |
||||||
|
{ |
||||||
|
m_closeOnTriggered = enabled; |
||||||
|
} |
||||||
|
|
||||||
|
QSize TriStateWidget::minimumSizeHint() const |
||||||
|
{ |
||||||
|
QStyleOptionMenuItem opt; |
||||||
|
opt.initFrom(this); |
||||||
|
opt.menuHasCheckableItems = true; |
||||||
|
const QSize contentSize = fontMetrics().size(Qt::TextSingleLine, m_text); |
||||||
|
return style()->sizeFromContents(QStyle::CT_MenuItem, &opt, contentSize, this); |
||||||
|
} |
||||||
|
|
||||||
|
void TriStateWidget::paintEvent(QPaintEvent *) |
||||||
|
{ |
||||||
|
QStyleOptionMenuItem opt; |
||||||
|
opt.initFrom(this); |
||||||
|
opt.menuItemType = QStyleOptionMenuItem::Normal; |
||||||
|
opt.checkType = QStyleOptionMenuItem::NonExclusive; |
||||||
|
opt.menuHasCheckableItems = true; |
||||||
|
opt.text = m_text; |
||||||
|
|
||||||
|
switch (m_checkState) { |
||||||
|
case Qt::PartiallyChecked: |
||||||
|
opt.state |= QStyle::State_NoChange; |
||||||
|
break; |
||||||
|
case Qt::Checked: |
||||||
|
opt.checked = true; |
||||||
|
break; |
||||||
|
case Qt::Unchecked: |
||||||
|
opt.checked = false; |
||||||
|
break; |
||||||
|
}; |
||||||
|
|
||||||
|
if ((opt.state & QStyle::State_HasFocus) |
||||||
|
|| rect().contains(mapFromGlobal(QCursor::pos()))) { |
||||||
|
opt.state |= QStyle::State_Selected; |
||||||
|
|
||||||
|
if (QApplication::mouseButtons() != Qt::NoButton) |
||||||
|
opt.state |= QStyle::State_Sunken; |
||||||
|
} |
||||||
|
|
||||||
|
QPainter painter {this}; |
||||||
|
style()->drawControl(QStyle::CE_MenuItem, &opt, &painter, this); |
||||||
|
} |
||||||
|
|
||||||
|
void TriStateWidget::mouseReleaseEvent(QMouseEvent *event) |
||||||
|
{ |
||||||
|
toggleCheckState(); |
||||||
|
|
||||||
|
if (m_closeOnTriggered) { |
||||||
|
// parent `triggered` signal will be emitted
|
||||||
|
QWidget::mouseReleaseEvent(event); |
||||||
|
} |
||||||
|
else { |
||||||
|
update(); |
||||||
|
// need to emit parent `triggered` signal manually
|
||||||
|
emit triggered(m_checkState == Qt::Checked); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void TriStateWidget::keyPressEvent(QKeyEvent *event) |
||||||
|
{ |
||||||
|
if ((event->key() == Qt::Key_Return) |
||||||
|
|| (event->key() == Qt::Key_Enter)) { |
||||||
|
toggleCheckState(); |
||||||
|
|
||||||
|
if (!m_closeOnTriggered) { |
||||||
|
update(); |
||||||
|
// need to emit parent `triggered` signal manually
|
||||||
|
emit triggered(m_checkState == Qt::Checked); |
||||||
|
return; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
QWidget::keyPressEvent(event); |
||||||
|
} |
||||||
|
|
||||||
|
void TriStateWidget::toggleCheckState() |
||||||
|
{ |
||||||
|
switch (m_checkState) { |
||||||
|
case Qt::Unchecked: |
||||||
|
case Qt::PartiallyChecked: |
||||||
|
m_checkState = Qt::Checked; |
||||||
|
break; |
||||||
|
case Qt::Checked: |
||||||
|
m_checkState = Qt::Unchecked; |
||||||
|
break; |
||||||
|
}; |
||||||
|
} |
@ -0,0 +1,61 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2019 Mike Tzou (Chocobo1) |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#pragma once |
||||||
|
|
||||||
|
#include <QWidget> |
||||||
|
|
||||||
|
class QString; |
||||||
|
|
||||||
|
class TriStateWidget : public QWidget |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
Q_DISABLE_COPY(TriStateWidget) |
||||||
|
|
||||||
|
public: |
||||||
|
TriStateWidget(const QString &text, QWidget *parent); |
||||||
|
|
||||||
|
void setCheckState(Qt::CheckState checkState); |
||||||
|
void setCloseOnTriggered(bool enabled); |
||||||
|
|
||||||
|
signals: |
||||||
|
void triggered(bool checked) const; |
||||||
|
|
||||||
|
private: |
||||||
|
QSize minimumSizeHint() const override; |
||||||
|
|
||||||
|
void paintEvent(QPaintEvent *) override; |
||||||
|
void mouseReleaseEvent(QMouseEvent *event) override; |
||||||
|
void keyPressEvent(QKeyEvent *event) override; |
||||||
|
|
||||||
|
void toggleCheckState(); |
||||||
|
|
||||||
|
bool m_closeOnTriggered; |
||||||
|
Qt::CheckState m_checkState; |
||||||
|
const QString m_text; |
||||||
|
}; |
@ -0,0 +1,62 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2019 Mike Tzou (Chocobo1) |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "tristateaction.h" |
||||||
|
|
||||||
|
#include <QString> |
||||||
|
#include <QWidget> |
||||||
|
|
||||||
|
#include "private/tristatewidget.h" |
||||||
|
|
||||||
|
TriStateAction::TriStateAction(const QString &text, QWidget *parent) |
||||||
|
: QWidgetAction {parent} |
||||||
|
, m_triStateWidget {new TriStateWidget {text, parent}} |
||||||
|
{ |
||||||
|
setCheckable(true); |
||||||
|
|
||||||
|
// required for QAction::setChecked(bool) to work
|
||||||
|
connect(this, &QAction::toggled, this, [this](const bool checked) |
||||||
|
{ |
||||||
|
m_triStateWidget->setCheckState(checked ? Qt::Checked : Qt::Unchecked); |
||||||
|
}); |
||||||
|
|
||||||
|
connect(m_triStateWidget, &TriStateWidget::triggered, this, &QAction::setChecked); |
||||||
|
connect(m_triStateWidget, &TriStateWidget::triggered, this, &QAction::triggered); |
||||||
|
setDefaultWidget(m_triStateWidget); |
||||||
|
} |
||||||
|
|
||||||
|
void TriStateAction::setCheckState(const Qt::CheckState checkState) |
||||||
|
{ |
||||||
|
QWidgetAction::setChecked((checkState == Qt::Checked) ? true : false); |
||||||
|
m_triStateWidget->setCheckState(checkState); |
||||||
|
} |
||||||
|
|
||||||
|
void TriStateAction::setCloseOnTriggered(const bool enabled) |
||||||
|
{ |
||||||
|
m_triStateWidget->setCloseOnTriggered(enabled); |
||||||
|
} |
@ -0,0 +1,53 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2019 Mike Tzou (Chocobo1) |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#pragma once |
||||||
|
|
||||||
|
#include <QWidgetAction> |
||||||
|
|
||||||
|
class QString; |
||||||
|
class QWidget; |
||||||
|
|
||||||
|
class TriStateWidget; |
||||||
|
|
||||||
|
// TriStateWidget is responsible for checkbox state (tri-state) and paint events while
|
||||||
|
// TriStateAction will keep in sync with it. This allows connecting with the usual
|
||||||
|
// QAction::triggered slot.
|
||||||
|
class TriStateAction : public QWidgetAction |
||||||
|
{ |
||||||
|
public: |
||||||
|
TriStateAction(const QString &text, QWidget *parent); |
||||||
|
|
||||||
|
// should use this function instead of QAction::setChecked(bool)
|
||||||
|
void setCheckState(Qt::CheckState checkState); |
||||||
|
|
||||||
|
void setCloseOnTriggered(bool enabled); |
||||||
|
|
||||||
|
private: |
||||||
|
TriStateWidget *m_triStateWidget; |
||||||
|
}; |
Loading…
Reference in new issue