Browse Source
Stops temporary containers being created needlessly due to API misuse. For example, it’s common for developers to assume QHash::values() and QHash::keys() are free and abuse them, failing to realize their implementation internally actually iterates the whole container, allocates memory, and fills a new QList. Added a removeIf generic algorithm, similar to std ones. We can't use std algorithms with Qt dictionaries because Qt iterators have different behavior from the std ones. Found using clazy.adaptive-webui-19844
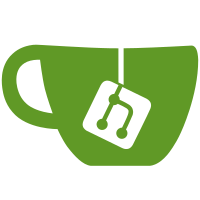
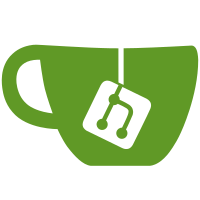
14 changed files with 121 additions and 61 deletions
@ -0,0 +1,41 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2018 Vladimir Golovnev <glassez@yandex.ru> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#pragma once |
||||||
|
|
||||||
|
namespace Dict |
||||||
|
{ |
||||||
|
// To be used with QMap, QHash and it's variants
|
||||||
|
template <typename Dictionary, typename BinaryPredicate> |
||||||
|
void removeIf(Dictionary &&dict, BinaryPredicate p) |
||||||
|
{ |
||||||
|
auto it = dict.begin(); |
||||||
|
while (it != dict.end()) |
||||||
|
it = (p(it.key(), it.value()) ? dict.erase(it) : it + 1); |
||||||
|
} |
||||||
|
} |
Loading…
Reference in new issue