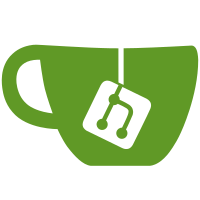
15 changed files with 355 additions and 31 deletions
After Width: | Height: | Size: 483 B |
@ -0,0 +1,45 @@
@@ -0,0 +1,45 @@
|
||||
#include "updownratiodlg.h" |
||||
#include "ui_updownratiodlg.h" |
||||
|
||||
#include "preferences.h" |
||||
|
||||
UpDownRatioDlg::UpDownRatioDlg(bool useDefault, qreal initialValue, |
||||
qreal maxValue, QWidget *parent) |
||||
: QDialog(parent), ui(new Ui::UpDownRatioDlg) |
||||
{ |
||||
ui->setupUi(this); |
||||
if (useDefault) { |
||||
ui->useDefaultButton->setChecked(true); |
||||
} else if (initialValue == -1) { |
||||
ui->noLimitButton->setChecked(true); |
||||
initialValue = Preferences().getGlobalMaxRatio(); |
||||
} else { |
||||
ui->torrentLimitButton->setChecked(true); |
||||
} |
||||
ui->ratioSpinBox->setMinimum(0); |
||||
ui->ratioSpinBox->setMaximum(maxValue); |
||||
ui->ratioSpinBox->setValue(initialValue); |
||||
connect(ui->buttonGroup, SIGNAL(buttonClicked(int)), |
||||
SLOT(handleRatioTypeChanged())); |
||||
handleRatioTypeChanged(); |
||||
} |
||||
|
||||
bool UpDownRatioDlg::useDefault() const |
||||
{ |
||||
return ui->useDefaultButton->isChecked(); |
||||
} |
||||
|
||||
qreal UpDownRatioDlg::ratio() const |
||||
{ |
||||
return ui->noLimitButton->isChecked() ? -1 : ui->ratioSpinBox->value(); |
||||
} |
||||
|
||||
void UpDownRatioDlg::handleRatioTypeChanged() |
||||
{ |
||||
ui->ratioSpinBox->setEnabled(ui->torrentLimitButton->isChecked()); |
||||
} |
||||
|
||||
UpDownRatioDlg::~UpDownRatioDlg() |
||||
{ |
||||
delete ui; |
||||
} |
@ -0,0 +1,31 @@
@@ -0,0 +1,31 @@
|
||||
#ifndef UPDOWNRATIODLG_H |
||||
#define UPDOWNRATIODLG_H |
||||
|
||||
#include <QtGui/QDialog> |
||||
|
||||
QT_BEGIN_NAMESPACE |
||||
namespace Ui { |
||||
class UpDownRatioDlg; |
||||
} |
||||
QT_END_NAMESPACE |
||||
|
||||
class UpDownRatioDlg : public QDialog |
||||
{ |
||||
Q_OBJECT |
||||
|
||||
public: |
||||
explicit UpDownRatioDlg(bool useDefault, qreal initialValue, qreal maxValue, |
||||
QWidget *parent = 0); |
||||
~UpDownRatioDlg(); |
||||
|
||||
bool useDefault() const; |
||||
qreal ratio() const; |
||||
|
||||
private slots: |
||||
void handleRatioTypeChanged(); |
||||
|
||||
private: |
||||
Ui::UpDownRatioDlg *ui; |
||||
}; |
||||
|
||||
#endif // UPDOWNRATIODLG_H
|
@ -0,0 +1,137 @@
@@ -0,0 +1,137 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?> |
||||
<ui version="4.0"> |
||||
<class>UpDownRatioDlg</class> |
||||
<widget class="QDialog" name="UpDownRatioDlg"> |
||||
<property name="geometry"> |
||||
<rect> |
||||
<x>0</x> |
||||
<y>0</y> |
||||
<width>317</width> |
||||
<height>152</height> |
||||
</rect> |
||||
</property> |
||||
<property name="windowTitle"> |
||||
<string>Torrent Upload/Download Ratio Limiting</string> |
||||
</property> |
||||
<layout class="QVBoxLayout" name="verticalLayout"> |
||||
<item> |
||||
<widget class="QRadioButton" name="useDefaultButton"> |
||||
<property name="text"> |
||||
<string>Use global ratio limit</string> |
||||
</property> |
||||
<attribute name="buttonGroup"> |
||||
<string>buttonGroup</string> |
||||
</attribute> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QRadioButton" name="noLimitButton"> |
||||
<property name="text"> |
||||
<string>Set no ratio limit</string> |
||||
</property> |
||||
<attribute name="buttonGroup"> |
||||
<string>buttonGroup</string> |
||||
</attribute> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<layout class="QHBoxLayout" name="horizontalLayout"> |
||||
<item> |
||||
<widget class="QRadioButton" name="torrentLimitButton"> |
||||
<property name="text"> |
||||
<string>Set ratio limit to</string> |
||||
</property> |
||||
<attribute name="buttonGroup"> |
||||
<string>buttonGroup</string> |
||||
</attribute> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QDoubleSpinBox" name="ratioSpinBox"> |
||||
<property name="decimals"> |
||||
<number>1</number> |
||||
</property> |
||||
<property name="singleStep"> |
||||
<double>0.100000000000000</double> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<spacer name="horizontalSpacer"> |
||||
<property name="orientation"> |
||||
<enum>Qt::Horizontal</enum> |
||||
</property> |
||||
<property name="sizeHint" stdset="0"> |
||||
<size> |
||||
<width>40</width> |
||||
<height>20</height> |
||||
</size> |
||||
</property> |
||||
</spacer> |
||||
</item> |
||||
</layout> |
||||
</item> |
||||
<item> |
||||
<spacer name="verticalSpacer"> |
||||
<property name="orientation"> |
||||
<enum>Qt::Vertical</enum> |
||||
</property> |
||||
<property name="sizeHint" stdset="0"> |
||||
<size> |
||||
<width>20</width> |
||||
<height>40</height> |
||||
</size> |
||||
</property> |
||||
</spacer> |
||||
</item> |
||||
<item> |
||||
<widget class="QDialogButtonBox" name="buttonBox"> |
||||
<property name="orientation"> |
||||
<enum>Qt::Horizontal</enum> |
||||
</property> |
||||
<property name="standardButtons"> |
||||
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
</layout> |
||||
</widget> |
||||
<resources/> |
||||
<connections> |
||||
<connection> |
||||
<sender>buttonBox</sender> |
||||
<signal>accepted()</signal> |
||||
<receiver>UpDownRatioDlg</receiver> |
||||
<slot>accept()</slot> |
||||
<hints> |
||||
<hint type="sourcelabel"> |
||||
<x>248</x> |
||||
<y>254</y> |
||||
</hint> |
||||
<hint type="destinationlabel"> |
||||
<x>157</x> |
||||
<y>274</y> |
||||
</hint> |
||||
</hints> |
||||
</connection> |
||||
<connection> |
||||
<sender>buttonBox</sender> |
||||
<signal>rejected()</signal> |
||||
<receiver>UpDownRatioDlg</receiver> |
||||
<slot>reject()</slot> |
||||
<hints> |
||||
<hint type="sourcelabel"> |
||||
<x>316</x> |
||||
<y>260</y> |
||||
</hint> |
||||
<hint type="destinationlabel"> |
||||
<x>286</x> |
||||
<y>274</y> |
||||
</hint> |
||||
</hints> |
||||
</connection> |
||||
</connections> |
||||
<buttongroups> |
||||
<buttongroup name="buttonGroup"/> |
||||
</buttongroups> |
||||
</ui> |
Loading…
Reference in new issue