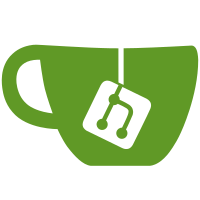
11 changed files with 283 additions and 62 deletions
@ -0,0 +1,75 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt4 and libtorrent. |
||||||
|
* Copyright (C) 2006 Christophe Dumez |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
* |
||||||
|
* Contact : chris@qbittorrent.org |
||||||
|
*/ |
||||||
|
|
||||||
|
#ifndef PEERLISTDELEGATE_H |
||||||
|
#define PEERLISTDELEGATE_H |
||||||
|
|
||||||
|
#include <QItemDelegate> |
||||||
|
#include "misc.h" |
||||||
|
|
||||||
|
enum PeerListColumns {IP, CLIENT, PROGRESS, DOWN_SPEED, UP_SPEED, TOT_DOWN, TOT_UP}; |
||||||
|
|
||||||
|
class PeerListDelegate: public QItemDelegate { |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
public: |
||||||
|
PeerListDelegate(QObject *parent) : QItemDelegate(parent){} |
||||||
|
|
||||||
|
~PeerListDelegate(){} |
||||||
|
|
||||||
|
void paint(QPainter * painter, const QStyleOptionViewItem & option, const QModelIndex & index) const{ |
||||||
|
QStyleOptionViewItemV2 opt = QItemDelegate::setOptions(index, option); |
||||||
|
switch(index.column()){ |
||||||
|
case TOT_DOWN: |
||||||
|
case TOT_UP: |
||||||
|
QItemDelegate::drawBackground(painter, opt, index); |
||||||
|
QItemDelegate::drawDisplay(painter, opt, option.rect, misc::friendlyUnit(index.data().toLongLong())); |
||||||
|
break; |
||||||
|
case DOWN_SPEED: |
||||||
|
case UP_SPEED:{ |
||||||
|
QItemDelegate::drawBackground(painter, opt, index); |
||||||
|
double speed = index.data().toDouble(); |
||||||
|
QItemDelegate::drawDisplay(painter, opt, opt.rect, QString::number(speed/1024., 'f', 1)+" "+tr("KiB/s")); |
||||||
|
break; |
||||||
|
} |
||||||
|
case PROGRESS:{ |
||||||
|
QItemDelegate::drawBackground(painter, opt, index); |
||||||
|
double progress = index.data().toDouble(); |
||||||
|
QItemDelegate::drawDisplay(painter, opt, opt.rect, QString::number(progress*100., 'f', 1)+"%"); |
||||||
|
break; |
||||||
|
} |
||||||
|
default: |
||||||
|
QItemDelegate::paint(painter, option, index); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
}; |
||||||
|
|
||||||
|
#endif // PEERLISTDELEGATE_H
|
@ -0,0 +1,120 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt4 and libtorrent. |
||||||
|
* Copyright (C) 2006 Christophe Dumez |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
* |
||||||
|
* Contact : chris@qbittorrent.org |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "peerlistwidget.h" |
||||||
|
#include "peerlistdelegate.h" |
||||||
|
#include "misc.h" |
||||||
|
#include <QStandardItemModel> |
||||||
|
#include <QSortFilterProxyModel> |
||||||
|
#include <QSet> |
||||||
|
#include <vector> |
||||||
|
|
||||||
|
PeerListWidget::PeerListWidget() { |
||||||
|
// Visual settings
|
||||||
|
setRootIsDecorated(false); |
||||||
|
setItemsExpandable(false); |
||||||
|
setAllColumnsShowFocus(true); |
||||||
|
// List Model
|
||||||
|
listModel = new QStandardItemModel(0, 7); |
||||||
|
listModel->setHeaderData(IP, Qt::Horizontal, tr("IP")); |
||||||
|
listModel->setHeaderData(CLIENT, Qt::Horizontal, tr("Client", "i.e.: Client application")); |
||||||
|
listModel->setHeaderData(PROGRESS, Qt::Horizontal, tr("Progress", "i.e: % downloaded")); |
||||||
|
listModel->setHeaderData(DOWN_SPEED, Qt::Horizontal, tr("Down Speed", "i.e: Download speed")); |
||||||
|
listModel->setHeaderData(UP_SPEED, Qt::Horizontal, tr("Up Speed", "i.e: Upload speed")); |
||||||
|
listModel->setHeaderData(TOT_DOWN, Qt::Horizontal, tr("Downloaded", "i.e: total data downloaded")); |
||||||
|
listModel->setHeaderData(TOT_UP, Qt::Horizontal, tr("Uploaded", "i.e: total data uploaded")); |
||||||
|
// Proxy model to support sorting without actually altering the underlying model
|
||||||
|
proxyModel = new QSortFilterProxyModel(); |
||||||
|
proxyModel->setDynamicSortFilter(true); |
||||||
|
proxyModel->setSourceModel(listModel); |
||||||
|
setModel(proxyModel); |
||||||
|
// List delegate
|
||||||
|
listDelegate = new PeerListDelegate(this); |
||||||
|
setItemDelegate(listDelegate); |
||||||
|
// Enable sorting
|
||||||
|
setSortingEnabled(true); |
||||||
|
} |
||||||
|
|
||||||
|
PeerListWidget::~PeerListWidget() { |
||||||
|
delete proxyModel; |
||||||
|
delete listModel; |
||||||
|
delete listDelegate; |
||||||
|
} |
||||||
|
|
||||||
|
void PeerListWidget::loadPeers(QTorrentHandle &h) { |
||||||
|
std::vector<peer_info> peers; |
||||||
|
h.get_peer_info(peers); |
||||||
|
std::vector<peer_info>::iterator itr; |
||||||
|
QSet<QString> old_peers_set = peerItems.keys().toSet(); |
||||||
|
for(itr = peers.begin(); itr != peers.end(); itr++) { |
||||||
|
peer_info peer = *itr; |
||||||
|
QString peer_ip = misc::toQString(peer.ip.address().to_string()); |
||||||
|
if(peerItems.contains(peer_ip)) { |
||||||
|
// Update existing peer
|
||||||
|
updatePeer(peer_ip, peer); |
||||||
|
old_peers_set.remove(peer_ip); |
||||||
|
} else { |
||||||
|
// Add new peer
|
||||||
|
peerItems[peer_ip] = addPeer(peer_ip, peer); |
||||||
|
} |
||||||
|
} |
||||||
|
// Delete peers that are gone
|
||||||
|
QSetIterator<QString> it(old_peers_set); |
||||||
|
while(it.hasNext()) { |
||||||
|
QStandardItem *item = peerItems.take(it.next()); |
||||||
|
listModel->removeRow(item->row()); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
QStandardItem* PeerListWidget::addPeer(QString ip, peer_info peer) { |
||||||
|
int row = listModel->rowCount(); |
||||||
|
// Adding Peer to peer list
|
||||||
|
listModel->insertRow(row); |
||||||
|
listModel->setData(listModel->index(row, IP), ip); |
||||||
|
listModel->setData(listModel->index(row, CLIENT), misc::toQString(peer.client)); |
||||||
|
listModel->setData(listModel->index(row, PROGRESS), peer.progress); |
||||||
|
listModel->setData(listModel->index(row, DOWN_SPEED), peer.payload_down_speed); |
||||||
|
listModel->setData(listModel->index(row, UP_SPEED), peer.payload_up_speed); |
||||||
|
listModel->setData(listModel->index(row, TOT_DOWN), peer.total_download); |
||||||
|
listModel->setData(listModel->index(row, TOT_UP), peer.total_upload); |
||||||
|
return listModel->item(row, 0); |
||||||
|
} |
||||||
|
|
||||||
|
void PeerListWidget::updatePeer(QString ip, peer_info peer) { |
||||||
|
QStandardItem *item = peerItems.value(ip); |
||||||
|
int row = item->row(); |
||||||
|
listModel->setData(listModel->index(row, IP), ip); |
||||||
|
listModel->setData(listModel->index(row, CLIENT), misc::toQString(peer.client)); |
||||||
|
listModel->setData(listModel->index(row, PROGRESS), peer.progress); |
||||||
|
listModel->setData(listModel->index(row, DOWN_SPEED), peer.payload_down_speed); |
||||||
|
listModel->setData(listModel->index(row, UP_SPEED), peer.payload_up_speed); |
||||||
|
listModel->setData(listModel->index(row, TOT_DOWN), peer.total_download); |
||||||
|
listModel->setData(listModel->index(row, TOT_UP), peer.total_upload); |
||||||
|
} |
@ -0,0 +1,61 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt4 and libtorrent. |
||||||
|
* Copyright (C) 2006 Christophe Dumez |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
* |
||||||
|
* Contact : chris@qbittorrent.org |
||||||
|
*/ |
||||||
|
|
||||||
|
#ifndef PEERLISTWIDGET_H |
||||||
|
#define PEERLISTWIDGET_H |
||||||
|
|
||||||
|
#include <QTreeView> |
||||||
|
#include <QHash> |
||||||
|
#include "qtorrenthandle.h" |
||||||
|
|
||||||
|
class QStandardItemModel; |
||||||
|
class QStandardItem; |
||||||
|
class QSortFilterProxyModel; |
||||||
|
class PeerListDelegate; |
||||||
|
|
||||||
|
class PeerListWidget : public QTreeView { |
||||||
|
|
||||||
|
private: |
||||||
|
QStandardItemModel *listModel; |
||||||
|
PeerListDelegate *listDelegate; |
||||||
|
QSortFilterProxyModel * proxyModel; |
||||||
|
QHash<QString, QStandardItem*> peerItems; |
||||||
|
|
||||||
|
public: |
||||||
|
PeerListWidget(); |
||||||
|
~PeerListWidget(); |
||||||
|
|
||||||
|
public slots: |
||||||
|
void loadPeers(QTorrentHandle &h); |
||||||
|
QStandardItem* addPeer(QString ip, peer_info peer); |
||||||
|
void updatePeer(QString ip, peer_info peer); |
||||||
|
}; |
||||||
|
|
||||||
|
#endif // PEERLISTWIDGET_H
|
Loading…
Reference in new issue