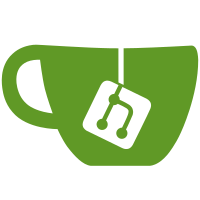
4 changed files with 4 additions and 126 deletions
@ -1,60 +0,0 @@
@@ -1,60 +0,0 @@
|
||||
#include "upnp.h" |
||||
|
||||
#define WAIT_TIMEOUT 5 |
||||
|
||||
// Control point registration callback
|
||||
int callBackCPRegister( Upnp_EventType EventType, void* Event, void* Cookie ){ |
||||
switch(EventType){ |
||||
case UPNP_DISCOVERY_ADVERTISEMENT_ALIVE: |
||||
case UPNP_DISCOVERY_SEARCH_RESULT: |
||||
{ |
||||
struct Upnp_Discovery *d_event = (struct Upnp_Discovery*) Event; |
||||
IXML_Document *DescDoc; |
||||
int ret = UpnpDownloadXmlDoc(d_event->Location, &DescDoc); |
||||
if(ret != UPNP_E_SUCCESS){ |
||||
// silently ignore?
|
||||
}else{ |
||||
((UPnPHandler*)Cookie)->addUPnPDevice(d_event, DescDoc); |
||||
// TvCtrlPointAddDevice(DescDoc, d_event->Location, d_event->Expires);
|
||||
} |
||||
if(DescDoc) ixmlDocument_free(DescDoc); |
||||
// TvCtrlPointPrintList();
|
||||
break; |
||||
} |
||||
case UPNP_DISCOVERY_SEARCH_TIMEOUT: |
||||
qDebug("UPnP devices discovery timed out..."); |
||||
break; |
||||
case UPNP_DISCOVERY_ADVERTISEMENT_BYEBYE: |
||||
{ |
||||
struct Upnp_Discovery *d_event = (struct Upnp_Discovery*) Event; |
||||
((UPnPHandler*)Cookie)->removeUPnPDevice(QString(d_event->DeviceId)); |
||||
// TvCtrlPointPrintList();
|
||||
break; |
||||
} |
||||
default: |
||||
qDebug("Debug: UPnP Unhandled event"); |
||||
} |
||||
return 0; |
||||
} |
||||
|
||||
void UPnPHandler::run(){ |
||||
// Initialize the SDK
|
||||
int ret = UpnpInit(NULL, 0); |
||||
if(ret != UPNP_E_SUCCESS){ |
||||
qDebug("Fatal error: UPnP initialization failed"); |
||||
UpnpFinish(); |
||||
return; |
||||
} |
||||
qDebug("UPnP successfully initialized"); |
||||
qDebug("UPnP bind on IP %s:%d", UpnpGetServerIpAddress(), UpnpGetServerPort()); |
||||
// Register the UPnP control point
|
||||
ret = UpnpRegisterClient(callBackCPRegister, this, &UPnPClientHandle); |
||||
if(ret != UPNP_E_SUCCESS){ |
||||
qDebug("Fatal error: UPnP control point registration failed"); |
||||
UpnpFinish(); |
||||
return; |
||||
} |
||||
qDebug("UPnP control point successfully registered"); |
||||
// Look for UPnP enabled routers (devices)
|
||||
ret = UpnpSearchAsync(UPnPClientHandle, WAIT_TIMEOUT, "upnp:rootdevice", this); |
||||
} |
@ -1,64 +0,0 @@
@@ -1,64 +0,0 @@
|
||||
/*
|
||||
* Bittorrent Client using Qt4 and libtorrent. |
||||
* Copyright (C) 2006 Christophe Dumez |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* Contact : chris@qbittorrent.org |
||||
*/ |
||||
#ifndef __QBT_UPNP__ |
||||
#define __QBT_UPNP__ |
||||
|
||||
#include <QThread> |
||||
#include <QHash> |
||||
|
||||
#include <upnp/upnp.h> |
||||
|
||||
class UPnPDevice{ |
||||
private: |
||||
struct Upnp_Discovery* device; |
||||
IXML_Document *doc; |
||||
|
||||
public: |
||||
UPnPDevice(struct Upnp_Discovery* device, IXML_Document* doc): device(device), doc(doc){} |
||||
IXML_Document* getDoc(){ return doc; } |
||||
struct Upnp_Discovery* getDevice(){ return device;} |
||||
}; |
||||
|
||||
class UPnPHandler : public QThread { |
||||
Q_OBJECT |
||||
|
||||
private: |
||||
UpnpClient_Handle UPnPClientHandle; |
||||
QHash<QString, UPnPDevice*> UPnPDevices; |
||||
|
||||
public: |
||||
UPnPHandler(){} |
||||
~UPnPHandler(){} |
||||
|
||||
public slots: |
||||
void addUPnPDevice(struct Upnp_Discovery* device, IXML_Document *doc){ |
||||
UPnPDevices.insert(QString(device->DeviceId), new UPnPDevice(device, doc)); |
||||
} |
||||
|
||||
void removeUPnPDevice(QString device_id){ |
||||
UPnPDevices.remove(device_id); |
||||
} |
||||
|
||||
private: |
||||
void run(); |
||||
}; |
||||
|
||||
#endif |
Loading…
Reference in new issue