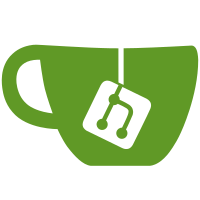
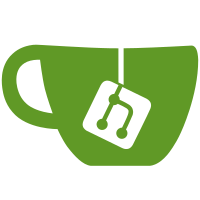
14 changed files with 456 additions and 6 deletions
@ -0,0 +1,42 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2016 Alexandr Milovantsev <dzmat@yandex.ru> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "net.h" |
||||||
|
#include <QHostAddress> |
||||||
|
#include <QString> |
||||||
|
|
||||||
|
namespace Utils |
||||||
|
{ |
||||||
|
namespace Net |
||||||
|
{ |
||||||
|
bool isValidIP(const QString &ip) |
||||||
|
{ |
||||||
|
return !QHostAddress(ip).isNull(); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,41 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2016 Alexandr Milovantsev <dzmat@yandex.ru> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#ifndef BASE_UTILS_NET_H |
||||||
|
#define BASE_UTILS_NET_H |
||||||
|
class QString; |
||||||
|
|
||||||
|
namespace Utils |
||||||
|
{ |
||||||
|
namespace Net |
||||||
|
{ |
||||||
|
bool isValidIP(const QString &ip); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
#endif // BASE_UTILS_NET_H
|
@ -0,0 +1,99 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2016 Alexandr Milovantsev <dzmat@yandex.ru> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "banlistoptions.h" |
||||||
|
#include "ui_banlistoptions.h" |
||||||
|
|
||||||
|
#include <QMessageBox> |
||||||
|
#include <QHostAddress> |
||||||
|
|
||||||
|
#include "base/bittorrent/session.h" |
||||||
|
#include "base/utils/net.h" |
||||||
|
|
||||||
|
BanListOptions::BanListOptions(QWidget *parent) |
||||||
|
: QDialog(parent) |
||||||
|
, m_ui(new Ui::BanListOptions) |
||||||
|
, m_modified(false) |
||||||
|
{ |
||||||
|
m_ui->setupUi(this); |
||||||
|
m_ui->bannedIPList->addItems(BitTorrent::Session::instance()->bannedIPs()); |
||||||
|
m_ui->buttonBanIP->setEnabled(false); |
||||||
|
} |
||||||
|
|
||||||
|
BanListOptions::~BanListOptions() |
||||||
|
{ |
||||||
|
delete m_ui; |
||||||
|
} |
||||||
|
|
||||||
|
void BanListOptions::on_buttonBox_accepted() |
||||||
|
{ |
||||||
|
if (m_modified) { |
||||||
|
// save to session
|
||||||
|
QStringList IPList; |
||||||
|
for (int i = 0; i < m_ui->bannedIPList->count(); ++i) |
||||||
|
IPList << m_ui->bannedIPList->item(i)->text(); |
||||||
|
BitTorrent::Session::instance()->setBannedIPs(IPList); |
||||||
|
} |
||||||
|
QDialog::accept(); |
||||||
|
} |
||||||
|
|
||||||
|
void BanListOptions::on_buttonBanIP_clicked() |
||||||
|
{ |
||||||
|
QString ip=m_ui->txtIP->text(); |
||||||
|
if (!Utils::Net::isValidIP(ip)) { |
||||||
|
QMessageBox::warning(this, tr("Warning"), tr("The entered IP address is invalid.")); |
||||||
|
return; |
||||||
|
} |
||||||
|
// the same IPv6 addresses could be written in different forms;
|
||||||
|
// QHostAddress::toString() result format follows RFC5952;
|
||||||
|
// thus we avoid duplicate entries pointing to the same address
|
||||||
|
ip = QHostAddress(ip).toString(); |
||||||
|
QList<QListWidgetItem *> findres = m_ui->bannedIPList->findItems(ip, Qt::MatchExactly); |
||||||
|
if (!findres.isEmpty()) { |
||||||
|
QMessageBox::warning(this, tr("Warning"), tr("The entered IP is already banned.")); |
||||||
|
return; |
||||||
|
} |
||||||
|
m_ui->bannedIPList->addItem(ip); |
||||||
|
m_ui->txtIP->clear(); |
||||||
|
m_modified = true; |
||||||
|
} |
||||||
|
|
||||||
|
void BanListOptions::on_buttonDeleteIP_clicked() |
||||||
|
{ |
||||||
|
QList<QListWidgetItem *> selection = m_ui->bannedIPList->selectedItems(); |
||||||
|
for (auto &i : selection) { |
||||||
|
m_ui->bannedIPList->removeItemWidget(i); |
||||||
|
delete i; |
||||||
|
} |
||||||
|
m_modified = true; |
||||||
|
} |
||||||
|
|
||||||
|
void BanListOptions::on_txtIP_textChanged(const QString &ip) |
||||||
|
{ |
||||||
|
m_ui->buttonBanIP->setEnabled(Utils::Net::isValidIP(ip)); |
||||||
|
} |
@ -0,0 +1,58 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2016 Alexandr Milovantsev <dzmat@yandex.ru> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#ifndef OPTIONS_BANLIST_H |
||||||
|
#define OPTIONS_BANLIST_H |
||||||
|
|
||||||
|
#include <QDialog> |
||||||
|
|
||||||
|
namespace Ui |
||||||
|
{ |
||||||
|
class BanListOptions; |
||||||
|
} |
||||||
|
|
||||||
|
class BanListOptions: public QDialog |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
|
||||||
|
public: |
||||||
|
explicit BanListOptions(QWidget *parent = 0); |
||||||
|
~BanListOptions(); |
||||||
|
|
||||||
|
private slots: |
||||||
|
void on_buttonBox_accepted(); |
||||||
|
void on_buttonBanIP_clicked(); |
||||||
|
void on_buttonDeleteIP_clicked(); |
||||||
|
void on_txtIP_textChanged(const QString &ip); |
||||||
|
|
||||||
|
private: |
||||||
|
Ui::BanListOptions *m_ui; |
||||||
|
bool m_modified; |
||||||
|
}; |
||||||
|
|
||||||
|
#endif // OPTIONS_BANLIST_H
|
@ -0,0 +1,115 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<ui version="4.0"> |
||||||
|
<class>BanListOptions</class> |
||||||
|
<widget class="QDialog" name="BanListOptions"> |
||||||
|
<property name="geometry"> |
||||||
|
<rect> |
||||||
|
<x>0</x> |
||||||
|
<y>0</y> |
||||||
|
<width>360</width> |
||||||
|
<height>450</height> |
||||||
|
</rect> |
||||||
|
</property> |
||||||
|
<property name="windowTitle"> |
||||||
|
<string>List of banned IP addresses</string> |
||||||
|
</property> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout"> |
||||||
|
<item> |
||||||
|
<widget class="QFrame" name="bannedIPBox"> |
||||||
|
<property name="enabled"> |
||||||
|
<bool>true</bool> |
||||||
|
</property> |
||||||
|
<property name="sizePolicy"> |
||||||
|
<sizepolicy hsizetype="Expanding" vsizetype="Expanding"> |
||||||
|
<horstretch>0</horstretch> |
||||||
|
<verstretch>0</verstretch> |
||||||
|
</sizepolicy> |
||||||
|
</property> |
||||||
|
<property name="autoFillBackground"> |
||||||
|
<bool>true</bool> |
||||||
|
</property> |
||||||
|
<property name="frameShape"> |
||||||
|
<enum>QFrame::Panel</enum> |
||||||
|
</property> |
||||||
|
<property name="frameShadow"> |
||||||
|
<enum>QFrame::Raised</enum> |
||||||
|
</property> |
||||||
|
<property name="lineWidth"> |
||||||
|
<number>1</number> |
||||||
|
</property> |
||||||
|
<property name="midLineWidth"> |
||||||
|
<number>0</number> |
||||||
|
</property> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout_21"> |
||||||
|
<item> |
||||||
|
<widget class="QListWidget" name="bannedIPList"> |
||||||
|
<property name="sizePolicy"> |
||||||
|
<sizepolicy hsizetype="Expanding" vsizetype="Expanding"> |
||||||
|
<horstretch>0</horstretch> |
||||||
|
<verstretch>0</verstretch> |
||||||
|
</sizepolicy> |
||||||
|
</property> |
||||||
|
<property name="sortingEnabled"> |
||||||
|
<bool>true</bool> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<layout class="QHBoxLayout" name="horizontalLayout_18"> |
||||||
|
<item> |
||||||
|
<widget class="QLineEdit" name="txtIP"/> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QPushButton" name="buttonBanIP"> |
||||||
|
<property name="text"> |
||||||
|
<string>Ban IP</string> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QPushButton" name="buttonDeleteIP"> |
||||||
|
<property name="text"> |
||||||
|
<string>Delete</string> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QDialogButtonBox" name="buttonBox"> |
||||||
|
<property name="standardButtons"> |
||||||
|
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
<tabstops> |
||||||
|
<tabstop>bannedIPList</tabstop> |
||||||
|
<tabstop>txtIP</tabstop> |
||||||
|
<tabstop>buttonBanIP</tabstop> |
||||||
|
<tabstop>buttonDeleteIP</tabstop> |
||||||
|
</tabstops> |
||||||
|
<resources/> |
||||||
|
<connections> |
||||||
|
<connection> |
||||||
|
<sender>buttonBox</sender> |
||||||
|
<signal>rejected()</signal> |
||||||
|
<receiver>BanListOptions</receiver> |
||||||
|
<slot>reject()</slot> |
||||||
|
<hints> |
||||||
|
<hint type="sourcelabel"> |
||||||
|
<x>179</x> |
||||||
|
<y>427</y> |
||||||
|
</hint> |
||||||
|
<hint type="destinationlabel"> |
||||||
|
<x>179</x> |
||||||
|
<y>224</y> |
||||||
|
</hint> |
||||||
|
</hints> |
||||||
|
</connection> |
||||||
|
</connections> |
||||||
|
</ui> |
Loading…
Reference in new issue