Browse Source
Now getting piece information for a specific torrent is possible via: * Returns an array of states (integers) of pieces in order. Defined as: "0=not downloaded", "1=downloading", "2=downloaded". GET /query/getPieceStates/<torrent_hash> * Returns an array of hashes (strings) of pieces in order: GET /query/getPieceHashes/<torrent_hash>adaptive-webui-19844
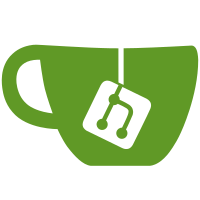
6 changed files with 88 additions and 1 deletions
Loading…
Reference in new issue