Browse Source
Simplify code, no functionality changes. Remove debug messages. Capitalize dialog name. Capitalize class name. Update license text.adaptive-webui-19844
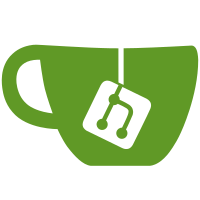
8 changed files with 178 additions and 131 deletions
@ -0,0 +1,107 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2006 Christophe Dumez <chris@qbittorrent.org> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "downloadfromurldialog.h" |
||||||
|
|
||||||
|
#include <QClipboard> |
||||||
|
#include <QMessageBox> |
||||||
|
#include <QPushButton> |
||||||
|
#include <QRegExp> |
||||||
|
#include <QString> |
||||||
|
#include <QStringList> |
||||||
|
|
||||||
|
#include "ui_downloadfromurldialog.h" |
||||||
|
#include "utils.h" |
||||||
|
|
||||||
|
DownloadFromURLDialog::DownloadFromURLDialog(QWidget *parent) |
||||||
|
: QDialog(parent) |
||||||
|
, m_ui(new Ui::DownloadFromURLDialog) |
||||||
|
{ |
||||||
|
m_ui->setupUi(this); |
||||||
|
setAttribute(Qt::WA_DeleteOnClose); |
||||||
|
setModal(true); |
||||||
|
|
||||||
|
m_ui->buttonBox->button(QDialogButtonBox::Ok)->setText(tr("Download")); |
||||||
|
connect(m_ui->buttonBox, &QDialogButtonBox::accepted, this, &DownloadFromURLDialog::downloadButtonClicked); |
||||||
|
connect(m_ui->buttonBox, &QDialogButtonBox::rejected, this, &QDialog::reject); |
||||||
|
|
||||||
|
m_ui->textUrls->setWordWrapMode(QTextOption::NoWrap); |
||||||
|
|
||||||
|
// Paste clipboard if there is an URL in it
|
||||||
|
const QStringList clipboardList = qApp->clipboard()->text().split('\n'); |
||||||
|
|
||||||
|
QStringList clipboardListCleaned; |
||||||
|
for (QString str : clipboardList) { |
||||||
|
str = str.trimmed(); |
||||||
|
if (str.isEmpty()) continue; |
||||||
|
|
||||||
|
if (!clipboardListCleaned.contains(str, Qt::CaseInsensitive)) { |
||||||
|
if (str.startsWith("http://", Qt::CaseInsensitive) |
||||||
|
|| str.startsWith("https://", Qt::CaseInsensitive) |
||||||
|
|| str.startsWith("ftp://", Qt::CaseInsensitive) |
||||||
|
|| str.startsWith("magnet:", Qt::CaseInsensitive) |
||||||
|
|| str.startsWith("bc://bt/", Qt::CaseInsensitive) |
||||||
|
|| (str.size() == 40 && !str.contains(QRegExp("[^0-9A-Fa-f]"))) |
||||||
|
|| (str.size() == 32 && !str.contains(QRegExp("[^2-7A-Za-z]")))) { |
||||||
|
clipboardListCleaned << str; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
if (!clipboardListCleaned.isEmpty()) |
||||||
|
m_ui->textUrls->setText(clipboardListCleaned.join('\n')); |
||||||
|
|
||||||
|
Utils::Gui::resize(this); |
||||||
|
show(); |
||||||
|
} |
||||||
|
|
||||||
|
DownloadFromURLDialog::~DownloadFromURLDialog() |
||||||
|
{ |
||||||
|
delete m_ui; |
||||||
|
} |
||||||
|
|
||||||
|
void DownloadFromURLDialog::downloadButtonClicked() |
||||||
|
{ |
||||||
|
const QStringList urls = m_ui->textUrls->toPlainText().split('\n'); |
||||||
|
|
||||||
|
QStringList uniqueURLs; |
||||||
|
for (QString url : urls) { |
||||||
|
url = url.trimmed(); |
||||||
|
if (url.isEmpty()) continue; |
||||||
|
|
||||||
|
if (!uniqueURLs.contains(url, Qt::CaseInsensitive)) |
||||||
|
uniqueURLs << url; |
||||||
|
} |
||||||
|
|
||||||
|
if (uniqueURLs.isEmpty()) { |
||||||
|
QMessageBox::warning(this, tr("No URL entered"), tr("Please type at least one URL.")); |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
emit urlsReadyToBeDownloaded(uniqueURLs); |
||||||
|
accept(); |
||||||
|
} |
@ -0,0 +1,58 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2006 Christophe Dumez <chris@qbittorrent.org> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#ifndef DOWNLOADFROMURL_H |
||||||
|
#define DOWNLOADFROMURL_H |
||||||
|
|
||||||
|
#include <QDialog> |
||||||
|
|
||||||
|
namespace Ui |
||||||
|
{ |
||||||
|
class DownloadFromURLDialog; |
||||||
|
} |
||||||
|
|
||||||
|
class DownloadFromURLDialog : public QDialog |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
Q_DISABLE_COPY(DownloadFromURLDialog) |
||||||
|
|
||||||
|
public: |
||||||
|
explicit DownloadFromURLDialog(QWidget *parent); |
||||||
|
~DownloadFromURLDialog(); |
||||||
|
|
||||||
|
signals: |
||||||
|
void urlsReadyToBeDownloaded(const QStringList &torrentURLs); |
||||||
|
|
||||||
|
private slots: |
||||||
|
void downloadButtonClicked(); |
||||||
|
|
||||||
|
private: |
||||||
|
Ui::DownloadFromURLDialog *m_ui; |
||||||
|
}; |
||||||
|
|
||||||
|
#endif |
@ -1,7 +1,7 @@ |
|||||||
<?xml version="1.0" encoding="UTF-8"?> |
<?xml version="1.0" encoding="UTF-8"?> |
||||||
<ui version="4.0"> |
<ui version="4.0"> |
||||||
<class>downloadFromURL</class> |
<class>DownloadFromURLDialog</class> |
||||||
<widget class="QDialog" name="downloadFromURL"> |
<widget class="QDialog" name="DownloadFromURLDialog"> |
||||||
<property name="geometry"> |
<property name="geometry"> |
||||||
<rect> |
<rect> |
||||||
<x>0</x> |
<x>0</x> |
@ -1,120 +0,0 @@ |
|||||||
/*
|
|
||||||
* Bittorrent Client using Qt4 and libtorrent. |
|
||||||
* Copyright (C) 2006 Christophe Dumez |
|
||||||
* |
|
||||||
* This program is free software; you can redistribute it and/or |
|
||||||
* modify it under the terms of the GNU General Public License |
|
||||||
* as published by the Free Software Foundation; either version 2 |
|
||||||
* of the License, or (at your option) any later version. |
|
||||||
* |
|
||||||
* This program is distributed in the hope that it will be useful, |
|
||||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|
||||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|
||||||
* GNU General Public License for more details. |
|
||||||
* |
|
||||||
* You should have received a copy of the GNU General Public License |
|
||||||
* along with this program; if not, write to the Free Software |
|
||||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
|
||||||
* |
|
||||||
* In addition, as a special exception, the copyright holders give permission to |
|
||||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
|
||||||
* modified versions of it that use the same license as the "OpenSSL" library), |
|
||||||
* and distribute the linked executables. You must obey the GNU General Public |
|
||||||
* License in all respects for all of the code used other than "OpenSSL". If you |
|
||||||
* modify file(s), you may extend this exception to your version of the file(s), |
|
||||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
|
||||||
* exception statement from your version. |
|
||||||
* |
|
||||||
* Contact : chris@qbittorrent.org |
|
||||||
*/ |
|
||||||
|
|
||||||
#ifndef DOWNLOADFROMURL_H |
|
||||||
#define DOWNLOADFROMURL_H |
|
||||||
|
|
||||||
#include <QClipboard> |
|
||||||
#include <QDialog> |
|
||||||
#include <QMessageBox> |
|
||||||
#include <QPushButton> |
|
||||||
#include <QRegExp> |
|
||||||
#include <QString> |
|
||||||
#include <QStringList> |
|
||||||
|
|
||||||
#include "ui_downloadfromurldlg.h" |
|
||||||
#include "utils.h" |
|
||||||
|
|
||||||
class downloadFromURL : public QDialog, private Ui::downloadFromURL |
|
||||||
{ |
|
||||||
Q_OBJECT |
|
||||||
|
|
||||||
public: |
|
||||||
downloadFromURL(QWidget *parent): QDialog(parent) |
|
||||||
{ |
|
||||||
setupUi(this); |
|
||||||
setAttribute(Qt::WA_DeleteOnClose); |
|
||||||
setModal(true); |
|
||||||
|
|
||||||
buttonBox->button(QDialogButtonBox::Ok)->setText(tr("Download")); |
|
||||||
connect(buttonBox, &QDialogButtonBox::accepted, this, &downloadFromURL::downloadButtonClicked); |
|
||||||
connect(buttonBox, &QDialogButtonBox::rejected, this, &QDialog::reject); |
|
||||||
|
|
||||||
textUrls->setWordWrapMode(QTextOption::NoWrap); |
|
||||||
|
|
||||||
// Paste clipboard if there is an URL in it
|
|
||||||
QString clip_txt = qApp->clipboard()->text(); |
|
||||||
QStringList clip_txt_list = clip_txt.split(QLatin1Char('\n')); |
|
||||||
clip_txt.clear(); |
|
||||||
QStringList clip_txt_list_cleaned; |
|
||||||
foreach (clip_txt, clip_txt_list) { |
|
||||||
clip_txt = clip_txt.trimmed(); |
|
||||||
if (!clip_txt.isEmpty()) { |
|
||||||
if (clip_txt_list_cleaned.indexOf(QRegExp(clip_txt, Qt::CaseInsensitive, QRegExp::FixedString)) < 0) { |
|
||||||
if (clip_txt.startsWith("http://", Qt::CaseInsensitive) |
|
||||||
|| clip_txt.startsWith("https://", Qt::CaseInsensitive) |
|
||||||
|| clip_txt.startsWith("ftp://", Qt::CaseInsensitive) |
|
||||||
|| clip_txt.startsWith("magnet:", Qt::CaseInsensitive) |
|
||||||
|| clip_txt.startsWith("bc://bt/", Qt::CaseInsensitive) |
|
||||||
|| (clip_txt.size() == 40 && !clip_txt.contains(QRegExp("[^0-9A-Fa-f]"))) |
|
||||||
|| (clip_txt.size() == 32 && !clip_txt.contains(QRegExp("[^2-7A-Za-z]")))) { |
|
||||||
clip_txt_list_cleaned << clip_txt; |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
if (clip_txt_list_cleaned.size() > 0) |
|
||||||
textUrls->setText(clip_txt_list_cleaned.join("\n")); |
|
||||||
|
|
||||||
Utils::Gui::resize(this); |
|
||||||
show(); |
|
||||||
} |
|
||||||
|
|
||||||
~downloadFromURL() {} |
|
||||||
|
|
||||||
signals: |
|
||||||
void urlsReadyToBeDownloaded(const QStringList& torrent_urls); |
|
||||||
|
|
||||||
private slots: |
|
||||||
void downloadButtonClicked() |
|
||||||
{ |
|
||||||
QString urls = textUrls->toPlainText(); |
|
||||||
QStringList url_list = urls.split(QLatin1Char('\n')); |
|
||||||
QString url; |
|
||||||
QStringList url_list_cleaned; |
|
||||||
foreach (url, url_list) { |
|
||||||
url = url.trimmed(); |
|
||||||
if (!url.isEmpty()) { |
|
||||||
if (url_list_cleaned.indexOf(QRegExp(url, Qt::CaseInsensitive, QRegExp::FixedString)) < 0) { |
|
||||||
url_list_cleaned << url; |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
if (url_list_cleaned.isEmpty()) { |
|
||||||
QMessageBox::warning(this, tr("No URL entered"), tr("Please type at least one URL.")); |
|
||||||
return; |
|
||||||
} |
|
||||||
emit urlsReadyToBeDownloaded(url_list_cleaned); |
|
||||||
qDebug("Emitted urlsReadytobedownloaded signal"); |
|
||||||
accept(); |
|
||||||
} |
|
||||||
}; |
|
||||||
|
|
||||||
#endif |
|
Loading…
Reference in new issue