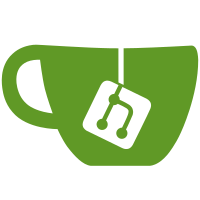
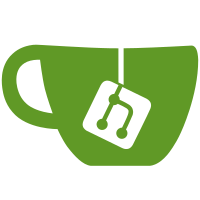
2 changed files with 63 additions and 49 deletions
@ -1,30 +1,44 @@
@@ -1,30 +1,44 @@
|
||||
/****************************************************************************
|
||||
** |
||||
** Copyright (c) 2007 Trolltech ASA <info@trolltech.com> |
||||
** |
||||
** Use, modification and distribution is allowed without limitation, |
||||
** warranty, liability or support of any kind. |
||||
** |
||||
****************************************************************************/ |
||||
/*
|
||||
* Bittorrent Client using Qt and libtorrent. |
||||
* Copyright (C) 2023 Vladimir Golovnev <glassez@yandex.ru> |
||||
* Copyright (c) 2007 Trolltech ASA <info@trolltech.com> |
||||
* |
||||
* This program is free software; you can redistribute it and/or |
||||
* modify it under the terms of the GNU General Public License |
||||
* as published by the Free Software Foundation; either version 2 |
||||
* of the License, or (at your option) any later version. |
||||
* |
||||
* This program is distributed in the hope that it will be useful, |
||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||
* GNU General Public License for more details. |
||||
* |
||||
* You should have received a copy of the GNU General Public License |
||||
* along with this program; if not, write to the Free Software |
||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||
* |
||||
* In addition, as a special exception, the copyright holders give permission to |
||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||
* modified versions of it that use the same license as the "OpenSSL" library), |
||||
* and distribute the linked executables. You must obey the GNU General Public |
||||
* License in all respects for all of the code used other than "OpenSSL". If you |
||||
* modify file(s), you may extend this exception to your version of the file(s), |
||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||
* exception statement from your version. |
||||
*/ |
||||
|
||||
#pragma once |
||||
|
||||
#include <QLineEdit> |
||||
|
||||
class QToolButton; |
||||
|
||||
class LineEdit final : public QLineEdit |
||||
{ |
||||
Q_OBJECT |
||||
Q_DISABLE_COPY_MOVE(LineEdit) |
||||
|
||||
public: |
||||
LineEdit(QWidget *parent = nullptr); |
||||
|
||||
protected: |
||||
void resizeEvent(QResizeEvent *e) override; |
||||
void keyPressEvent(QKeyEvent *event) override; |
||||
explicit LineEdit(QWidget *parent = nullptr); |
||||
|
||||
private: |
||||
QToolButton *m_searchButton = nullptr; |
||||
void keyPressEvent(QKeyEvent *event) override; |
||||
}; |
||||
|
Loading…
Reference in new issue