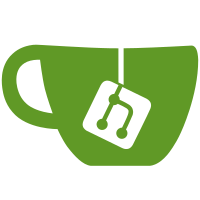
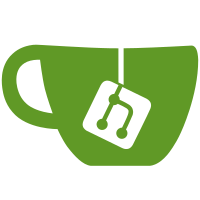
40 changed files with 1681 additions and 1073 deletions
@ -1,187 +0,0 @@ |
|||||||
/*
|
|
||||||
* Bittorrent Client using Qt and libtorrent. |
|
||||||
* Copyright (C) 2018 |
|
||||||
* |
|
||||||
* This program is free software; you can redistribute it and/or |
|
||||||
* modify it under the terms of the GNU General Public License |
|
||||||
* as published by the Free Software Foundation; either version 2 |
|
||||||
* of the License, or (at your option) any later version. |
|
||||||
* |
|
||||||
* This program is distributed in the hope that it will be useful, |
|
||||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|
||||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|
||||||
* GNU General Public License for more details. |
|
||||||
* |
|
||||||
* You should have received a copy of the GNU General Public License |
|
||||||
* along with this program; if not, write to the Free Software |
|
||||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
|
||||||
* |
|
||||||
* In addition, as a special exception, the copyright holders give permission to |
|
||||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
|
||||||
* modified versions of it that use the same license as the "OpenSSL" library), |
|
||||||
* and distribute the linked executables. You must obey the GNU General Public |
|
||||||
* License in all respects for all of the code used other than "OpenSSL". If you |
|
||||||
* modify file(s), you may extend this exception to your version of the file(s), |
|
||||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
|
||||||
* exception statement from your version. |
|
||||||
*/ |
|
||||||
|
|
||||||
#include "filesystemwatcher.h" |
|
||||||
|
|
||||||
#include <QtGlobal> |
|
||||||
|
|
||||||
#include <chrono> |
|
||||||
|
|
||||||
#if defined(Q_OS_MACOS) || defined(Q_OS_FREEBSD) || defined(Q_OS_OPENBSD) |
|
||||||
#include <cstring> |
|
||||||
#include <sys/mount.h> |
|
||||||
#include <sys/param.h> |
|
||||||
#endif |
|
||||||
|
|
||||||
#include "base/algorithm.h" |
|
||||||
#include "base/bittorrent/torrentinfo.h" |
|
||||||
#include "base/global.h" |
|
||||||
#include "base/logger.h" |
|
||||||
#include "base/utils/fs.h" |
|
||||||
|
|
||||||
using namespace std::chrono_literals; |
|
||||||
|
|
||||||
namespace |
|
||||||
{ |
|
||||||
const std::chrono::duration WATCH_INTERVAL = 10s; |
|
||||||
const int MAX_PARTIAL_RETRIES = 5; |
|
||||||
} |
|
||||||
|
|
||||||
FileSystemWatcher::FileSystemWatcher(QObject *parent) |
|
||||||
: QFileSystemWatcher(parent) |
|
||||||
{ |
|
||||||
connect(this, &QFileSystemWatcher::directoryChanged, this, &FileSystemWatcher::scanLocalFolder); |
|
||||||
|
|
||||||
m_partialTorrentTimer.setSingleShot(true); |
|
||||||
connect(&m_partialTorrentTimer, &QTimer::timeout, this, &FileSystemWatcher::processPartialTorrents); |
|
||||||
|
|
||||||
connect(&m_watchTimer, &QTimer::timeout, this, &FileSystemWatcher::scanNetworkFolders); |
|
||||||
} |
|
||||||
|
|
||||||
QStringList FileSystemWatcher::directories() const |
|
||||||
{ |
|
||||||
QStringList dirs = QFileSystemWatcher::directories(); |
|
||||||
for (const QDir &dir : asConst(m_watchedFolders)) |
|
||||||
dirs << dir.canonicalPath(); |
|
||||||
return dirs; |
|
||||||
} |
|
||||||
|
|
||||||
void FileSystemWatcher::addPath(const QString &path) |
|
||||||
{ |
|
||||||
if (path.isEmpty()) return; |
|
||||||
|
|
||||||
#if !defined Q_OS_HAIKU |
|
||||||
const QDir dir(path); |
|
||||||
if (!dir.exists()) return; |
|
||||||
|
|
||||||
// Check if the path points to a network file system or not
|
|
||||||
if (Utils::Fs::isNetworkFileSystem(path)) |
|
||||||
{ |
|
||||||
// Network mode
|
|
||||||
LogMsg(tr("Watching remote folder: \"%1\"").arg(Utils::Fs::toNativePath(path))); |
|
||||||
m_watchedFolders << dir; |
|
||||||
|
|
||||||
m_watchTimer.start(WATCH_INTERVAL); |
|
||||||
return; |
|
||||||
} |
|
||||||
#endif |
|
||||||
|
|
||||||
// Normal mode
|
|
||||||
LogMsg(tr("Watching local folder: \"%1\"").arg(Utils::Fs::toNativePath(path))); |
|
||||||
QFileSystemWatcher::addPath(path); |
|
||||||
scanLocalFolder(path); |
|
||||||
} |
|
||||||
|
|
||||||
void FileSystemWatcher::removePath(const QString &path) |
|
||||||
{ |
|
||||||
if (m_watchedFolders.removeOne(path)) |
|
||||||
{ |
|
||||||
if (m_watchedFolders.isEmpty()) |
|
||||||
m_watchTimer.stop(); |
|
||||||
return; |
|
||||||
} |
|
||||||
|
|
||||||
// Normal mode
|
|
||||||
QFileSystemWatcher::removePath(path); |
|
||||||
} |
|
||||||
|
|
||||||
void FileSystemWatcher::scanLocalFolder(const QString &path) |
|
||||||
{ |
|
||||||
QTimer::singleShot(2000, this, [this, path]() { processTorrentsInDir(path); }); |
|
||||||
} |
|
||||||
|
|
||||||
void FileSystemWatcher::scanNetworkFolders() |
|
||||||
{ |
|
||||||
for (const QDir &dir : asConst(m_watchedFolders)) |
|
||||||
processTorrentsInDir(dir); |
|
||||||
} |
|
||||||
|
|
||||||
void FileSystemWatcher::processPartialTorrents() |
|
||||||
{ |
|
||||||
QStringList noLongerPartial; |
|
||||||
|
|
||||||
// Check which torrents are still partial
|
|
||||||
Algorithm::removeIf(m_partialTorrents, [&noLongerPartial](const QString &torrentPath, int &value) |
|
||||||
{ |
|
||||||
if (!QFile::exists(torrentPath)) |
|
||||||
return true; |
|
||||||
|
|
||||||
if (BitTorrent::TorrentInfo::loadFromFile(torrentPath).isValid()) |
|
||||||
{ |
|
||||||
noLongerPartial << torrentPath; |
|
||||||
return true; |
|
||||||
} |
|
||||||
|
|
||||||
if (value >= MAX_PARTIAL_RETRIES) |
|
||||||
{ |
|
||||||
QFile::rename(torrentPath, torrentPath + ".qbt_rejected"); |
|
||||||
return true; |
|
||||||
} |
|
||||||
|
|
||||||
++value; |
|
||||||
return false; |
|
||||||
}); |
|
||||||
|
|
||||||
// Stop the partial timer if necessary
|
|
||||||
if (m_partialTorrents.empty()) |
|
||||||
{ |
|
||||||
m_partialTorrentTimer.stop(); |
|
||||||
qDebug("No longer any partial torrent."); |
|
||||||
} |
|
||||||
else |
|
||||||
{ |
|
||||||
qDebug("Still %d partial torrents after delayed processing.", m_partialTorrents.count()); |
|
||||||
m_partialTorrentTimer.start(WATCH_INTERVAL); |
|
||||||
} |
|
||||||
|
|
||||||
// Notify of new torrents
|
|
||||||
if (!noLongerPartial.isEmpty()) |
|
||||||
emit torrentsAdded(noLongerPartial); |
|
||||||
} |
|
||||||
|
|
||||||
void FileSystemWatcher::processTorrentsInDir(const QDir &dir) |
|
||||||
{ |
|
||||||
QStringList torrents; |
|
||||||
const QStringList files = dir.entryList({"*.torrent", "*.magnet"}, QDir::Files); |
|
||||||
for (const QString &file : files) |
|
||||||
{ |
|
||||||
const QString fileAbsPath = dir.absoluteFilePath(file); |
|
||||||
if (file.endsWith(".magnet", Qt::CaseInsensitive)) |
|
||||||
torrents << fileAbsPath; |
|
||||||
else if (BitTorrent::TorrentInfo::loadFromFile(fileAbsPath).isValid()) |
|
||||||
torrents << fileAbsPath; |
|
||||||
else if (!m_partialTorrents.contains(fileAbsPath)) |
|
||||||
m_partialTorrents[fileAbsPath] = 0; |
|
||||||
} |
|
||||||
|
|
||||||
if (!torrents.empty()) |
|
||||||
emit torrentsAdded(torrents); |
|
||||||
|
|
||||||
if (!m_partialTorrents.empty() && !m_partialTorrentTimer.isActive()) |
|
||||||
m_partialTorrentTimer.start(WATCH_INTERVAL); |
|
||||||
} |
|
@ -1,424 +0,0 @@ |
|||||||
/*
|
|
||||||
* Bittorrent Client using Qt and libtorrent. |
|
||||||
* Copyright (C) 2010 Christian Kandeler, Christophe Dumez <chris@qbittorrent.org> |
|
||||||
* |
|
||||||
* This program is free software; you can redistribute it and/or |
|
||||||
* modify it under the terms of the GNU General Public License |
|
||||||
* as published by the Free Software Foundation; either version 2 |
|
||||||
* of the License, or (at your option) any later version. |
|
||||||
* |
|
||||||
* This program is distributed in the hope that it will be useful, |
|
||||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|
||||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|
||||||
* GNU General Public License for more details. |
|
||||||
* |
|
||||||
* You should have received a copy of the GNU General Public License |
|
||||||
* along with this program; if not, write to the Free Software |
|
||||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
|
||||||
* |
|
||||||
* In addition, as a special exception, the copyright holders give permission to |
|
||||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
|
||||||
* modified versions of it that use the same license as the "OpenSSL" library), |
|
||||||
* and distribute the linked executables. You must obey the GNU General Public |
|
||||||
* License in all respects for all of the code used other than "OpenSSL". If you |
|
||||||
* modify file(s), you may extend this exception to your version of the file(s), |
|
||||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
|
||||||
* exception statement from your version. |
|
||||||
*/ |
|
||||||
|
|
||||||
#include "scanfoldersmodel.h" |
|
||||||
|
|
||||||
#include <QDir> |
|
||||||
#include <QFileInfo> |
|
||||||
#include <QStringList> |
|
||||||
#include <QTextStream> |
|
||||||
|
|
||||||
#include "bittorrent/session.h" |
|
||||||
#include "filesystemwatcher.h" |
|
||||||
#include "global.h" |
|
||||||
#include "preferences.h" |
|
||||||
#include "utils/fs.h" |
|
||||||
|
|
||||||
struct ScanFoldersModel::PathData |
|
||||||
{ |
|
||||||
PathData(const QString &watchPath, const PathType &type, const QString &downloadPath) |
|
||||||
: watchPath(watchPath) |
|
||||||
, downloadType(type) |
|
||||||
, downloadPath(downloadPath) |
|
||||||
{ |
|
||||||
if (this->downloadPath.isEmpty() && downloadType == CUSTOM_LOCATION) |
|
||||||
downloadType = DEFAULT_LOCATION; |
|
||||||
} |
|
||||||
|
|
||||||
QString watchPath; |
|
||||||
PathType downloadType; |
|
||||||
QString downloadPath; // valid for CUSTOM_LOCATION
|
|
||||||
}; |
|
||||||
|
|
||||||
ScanFoldersModel *ScanFoldersModel::m_instance = nullptr; |
|
||||||
|
|
||||||
void ScanFoldersModel::initInstance() |
|
||||||
{ |
|
||||||
if (!m_instance) |
|
||||||
m_instance = new ScanFoldersModel; |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersModel::freeInstance() |
|
||||||
{ |
|
||||||
delete m_instance; |
|
||||||
m_instance = nullptr; |
|
||||||
} |
|
||||||
|
|
||||||
ScanFoldersModel *ScanFoldersModel::instance() |
|
||||||
{ |
|
||||||
return m_instance; |
|
||||||
} |
|
||||||
|
|
||||||
ScanFoldersModel::ScanFoldersModel(QObject *parent) |
|
||||||
: QAbstractListModel(parent) |
|
||||||
, m_fsWatcher(nullptr) |
|
||||||
{ |
|
||||||
configure(); |
|
||||||
connect(Preferences::instance(), &Preferences::changed, this, &ScanFoldersModel::configure); |
|
||||||
} |
|
||||||
|
|
||||||
ScanFoldersModel::~ScanFoldersModel() |
|
||||||
{ |
|
||||||
qDeleteAll(m_pathList); |
|
||||||
} |
|
||||||
|
|
||||||
int ScanFoldersModel::rowCount(const QModelIndex &parent) const |
|
||||||
{ |
|
||||||
return parent.isValid() ? 0 : m_pathList.count(); |
|
||||||
} |
|
||||||
|
|
||||||
int ScanFoldersModel::columnCount(const QModelIndex &parent) const |
|
||||||
{ |
|
||||||
Q_UNUSED(parent); |
|
||||||
return NB_COLUMNS; |
|
||||||
} |
|
||||||
|
|
||||||
QVariant ScanFoldersModel::data(const QModelIndex &index, int role) const |
|
||||||
{ |
|
||||||
if (!index.isValid() || (index.row() >= rowCount())) |
|
||||||
return {}; |
|
||||||
|
|
||||||
const PathData *pathData = m_pathList.at(index.row()); |
|
||||||
QVariant value; |
|
||||||
|
|
||||||
switch (index.column()) |
|
||||||
{ |
|
||||||
case WATCH: |
|
||||||
if (role == Qt::DisplayRole) |
|
||||||
value = Utils::Fs::toNativePath(pathData->watchPath); |
|
||||||
break; |
|
||||||
case DOWNLOAD: |
|
||||||
if (role == Qt::UserRole) |
|
||||||
{ |
|
||||||
value = pathData->downloadType; |
|
||||||
} |
|
||||||
else if (role == Qt::DisplayRole) |
|
||||||
{ |
|
||||||
switch (pathData->downloadType) |
|
||||||
{ |
|
||||||
case DOWNLOAD_IN_WATCH_FOLDER: |
|
||||||
case DEFAULT_LOCATION: |
|
||||||
value = pathTypeDisplayName(pathData->downloadType); |
|
||||||
break; |
|
||||||
case CUSTOM_LOCATION: |
|
||||||
value = pathData->downloadPath; |
|
||||||
break; |
|
||||||
} |
|
||||||
} |
|
||||||
break; |
|
||||||
} |
|
||||||
|
|
||||||
return value; |
|
||||||
} |
|
||||||
|
|
||||||
QVariant ScanFoldersModel::headerData(int section, Qt::Orientation orientation, int role) const |
|
||||||
{ |
|
||||||
if ((orientation != Qt::Horizontal) || (role != Qt::DisplayRole) || (section < 0) || (section >= columnCount())) |
|
||||||
return {}; |
|
||||||
|
|
||||||
QVariant title; |
|
||||||
|
|
||||||
switch (section) |
|
||||||
{ |
|
||||||
case WATCH: |
|
||||||
title = tr("Monitored Folder"); |
|
||||||
break; |
|
||||||
case DOWNLOAD: |
|
||||||
title = tr("Override Save Location"); |
|
||||||
break; |
|
||||||
} |
|
||||||
|
|
||||||
return title; |
|
||||||
} |
|
||||||
|
|
||||||
Qt::ItemFlags ScanFoldersModel::flags(const QModelIndex &index) const |
|
||||||
{ |
|
||||||
if (!index.isValid() || (index.row() >= rowCount())) |
|
||||||
return QAbstractListModel::flags(index); |
|
||||||
|
|
||||||
Qt::ItemFlags flags; |
|
||||||
|
|
||||||
switch (index.column()) |
|
||||||
{ |
|
||||||
case WATCH: |
|
||||||
flags = QAbstractListModel::flags(index); |
|
||||||
break; |
|
||||||
case DOWNLOAD: |
|
||||||
flags = QAbstractListModel::flags(index) | Qt::ItemIsEditable; |
|
||||||
break; |
|
||||||
} |
|
||||||
|
|
||||||
return flags; |
|
||||||
} |
|
||||||
|
|
||||||
bool ScanFoldersModel::setData(const QModelIndex &index, const QVariant &value, int role) |
|
||||||
{ |
|
||||||
if (!index.isValid() || (index.row() >= rowCount()) || (index.column() >= columnCount()) |
|
||||||
|| (index.column() != DOWNLOAD)) |
|
||||||
return false; |
|
||||||
|
|
||||||
if (role == Qt::UserRole) |
|
||||||
{ |
|
||||||
const auto type = static_cast<PathType>(value.toInt()); |
|
||||||
if (type == CUSTOM_LOCATION) |
|
||||||
return false; |
|
||||||
|
|
||||||
m_pathList[index.row()]->downloadType = type; |
|
||||||
m_pathList[index.row()]->downloadPath.clear(); |
|
||||||
emit dataChanged(index, index); |
|
||||||
} |
|
||||||
else if (role == Qt::DisplayRole) |
|
||||||
{ |
|
||||||
const QString path = value.toString(); |
|
||||||
if (path.isEmpty()) // means we didn't pass CUSTOM_LOCATION type
|
|
||||||
return false; |
|
||||||
|
|
||||||
m_pathList[index.row()]->downloadType = CUSTOM_LOCATION; |
|
||||||
m_pathList[index.row()]->downloadPath = Utils::Fs::toNativePath(path); |
|
||||||
emit dataChanged(index, index); |
|
||||||
} |
|
||||||
else |
|
||||||
{ |
|
||||||
return false; |
|
||||||
} |
|
||||||
|
|
||||||
return true; |
|
||||||
} |
|
||||||
|
|
||||||
ScanFoldersModel::PathStatus ScanFoldersModel::addPath(const QString &watchPath, const PathType &downloadType, const QString &downloadPath, bool addToFSWatcher) |
|
||||||
{ |
|
||||||
const QDir watchDir(watchPath); |
|
||||||
if (!watchDir.exists()) return DoesNotExist; |
|
||||||
if (!watchDir.isReadable()) return CannotRead; |
|
||||||
|
|
||||||
const QString canonicalWatchPath = watchDir.canonicalPath(); |
|
||||||
if (findPathData(canonicalWatchPath) != -1) return AlreadyInList; |
|
||||||
|
|
||||||
const QDir downloadDir(downloadPath); |
|
||||||
const QString canonicalDownloadPath = downloadDir.canonicalPath(); |
|
||||||
|
|
||||||
if (!m_fsWatcher) |
|
||||||
{ |
|
||||||
m_fsWatcher = new FileSystemWatcher(this); |
|
||||||
connect(m_fsWatcher, &FileSystemWatcher::torrentsAdded, this, &ScanFoldersModel::addTorrentsToSession); |
|
||||||
} |
|
||||||
|
|
||||||
beginInsertRows(QModelIndex(), rowCount(), rowCount()); |
|
||||||
m_pathList << new PathData(Utils::Fs::toNativePath(canonicalWatchPath), downloadType, Utils::Fs::toNativePath(canonicalDownloadPath)); |
|
||||||
endInsertRows(); |
|
||||||
|
|
||||||
// Start scanning
|
|
||||||
if (addToFSWatcher) |
|
||||||
m_fsWatcher->addPath(canonicalWatchPath); |
|
||||||
return Ok; |
|
||||||
} |
|
||||||
|
|
||||||
ScanFoldersModel::PathStatus ScanFoldersModel::updatePath(const QString &watchPath, const PathType &downloadType, const QString &downloadPath) |
|
||||||
{ |
|
||||||
const QDir watchDir(watchPath); |
|
||||||
const QString canonicalWatchPath = watchDir.canonicalPath(); |
|
||||||
const int row = findPathData(canonicalWatchPath); |
|
||||||
if (row == -1) return DoesNotExist; |
|
||||||
|
|
||||||
const QDir downloadDir(downloadPath); |
|
||||||
const QString canonicalDownloadPath = downloadDir.canonicalPath(); |
|
||||||
|
|
||||||
m_pathList.at(row)->downloadType = downloadType; |
|
||||||
m_pathList.at(row)->downloadPath = Utils::Fs::toNativePath(canonicalDownloadPath); |
|
||||||
|
|
||||||
return Ok; |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersModel::addToFSWatcher(const QStringList &watchPaths) |
|
||||||
{ |
|
||||||
if (!m_fsWatcher) |
|
||||||
return; // addPath() wasn't called before this
|
|
||||||
|
|
||||||
for (const QString &path : watchPaths) |
|
||||||
{ |
|
||||||
const QDir watchDir(path); |
|
||||||
const QString canonicalWatchPath = watchDir.canonicalPath(); |
|
||||||
m_fsWatcher->addPath(canonicalWatchPath); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersModel::removePath(const int row, const bool removeFromFSWatcher) |
|
||||||
{ |
|
||||||
Q_ASSERT((row >= 0) && (row < rowCount())); |
|
||||||
beginRemoveRows(QModelIndex(), row, row); |
|
||||||
if (removeFromFSWatcher) |
|
||||||
m_fsWatcher->removePath(m_pathList.at(row)->watchPath); |
|
||||||
delete m_pathList.takeAt(row); |
|
||||||
endRemoveRows(); |
|
||||||
} |
|
||||||
|
|
||||||
bool ScanFoldersModel::removePath(const QString &path, const bool removeFromFSWatcher) |
|
||||||
{ |
|
||||||
const int row = findPathData(path); |
|
||||||
if (row == -1) return false; |
|
||||||
|
|
||||||
removePath(row, removeFromFSWatcher); |
|
||||||
return true; |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersModel::removeFromFSWatcher(const QStringList &watchPaths) |
|
||||||
{ |
|
||||||
for (const QString &path : watchPaths) |
|
||||||
m_fsWatcher->removePath(path); |
|
||||||
} |
|
||||||
|
|
||||||
bool ScanFoldersModel::downloadInWatchFolder(const QString &filePath) const |
|
||||||
{ |
|
||||||
const int row = findPathData(QFileInfo(filePath).dir().path()); |
|
||||||
Q_ASSERT(row != -1); |
|
||||||
const PathData *data = m_pathList.at(row); |
|
||||||
return (data->downloadType == DOWNLOAD_IN_WATCH_FOLDER); |
|
||||||
} |
|
||||||
|
|
||||||
bool ScanFoldersModel::downloadInDefaultFolder(const QString &filePath) const |
|
||||||
{ |
|
||||||
const int row = findPathData(QFileInfo(filePath).dir().path()); |
|
||||||
Q_ASSERT(row != -1); |
|
||||||
const PathData *data = m_pathList.at(row); |
|
||||||
return (data->downloadType == DEFAULT_LOCATION); |
|
||||||
} |
|
||||||
|
|
||||||
QString ScanFoldersModel::downloadPathTorrentFolder(const QString &filePath) const |
|
||||||
{ |
|
||||||
const int row = findPathData(QFileInfo(filePath).dir().path()); |
|
||||||
Q_ASSERT(row != -1); |
|
||||||
const PathData *data = m_pathList.at(row); |
|
||||||
if (data->downloadType == CUSTOM_LOCATION) |
|
||||||
return data->downloadPath; |
|
||||||
|
|
||||||
return {}; |
|
||||||
} |
|
||||||
|
|
||||||
int ScanFoldersModel::findPathData(const QString &path) const |
|
||||||
{ |
|
||||||
for (int i = 0; i < m_pathList.count(); ++i) |
|
||||||
if (m_pathList.at(i)->watchPath == Utils::Fs::toNativePath(path)) |
|
||||||
return i; |
|
||||||
|
|
||||||
return -1; |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersModel::makePersistent() |
|
||||||
{ |
|
||||||
QVariantHash dirs; |
|
||||||
|
|
||||||
for (const PathData *pathData : asConst(m_pathList)) |
|
||||||
{ |
|
||||||
if (pathData->downloadType == CUSTOM_LOCATION) |
|
||||||
dirs.insert(Utils::Fs::toUniformPath(pathData->watchPath), Utils::Fs::toUniformPath(pathData->downloadPath)); |
|
||||||
else |
|
||||||
dirs.insert(Utils::Fs::toUniformPath(pathData->watchPath), pathData->downloadType); |
|
||||||
} |
|
||||||
|
|
||||||
Preferences::instance()->setScanDirs(dirs); |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersModel::configure() |
|
||||||
{ |
|
||||||
const QVariantHash dirs = Preferences::instance()->getScanDirs(); |
|
||||||
|
|
||||||
for (auto i = dirs.cbegin(); i != dirs.cend(); ++i) |
|
||||||
{ |
|
||||||
if (i.value().type() == QVariant::Int) |
|
||||||
addPath(i.key(), static_cast<PathType>(i.value().toInt()), QString()); |
|
||||||
else |
|
||||||
addPath(i.key(), CUSTOM_LOCATION, i.value().toString()); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersModel::addTorrentsToSession(const QStringList &pathList) |
|
||||||
{ |
|
||||||
for (const QString &file : pathList) |
|
||||||
{ |
|
||||||
qDebug("File %s added", qUtf8Printable(file)); |
|
||||||
|
|
||||||
BitTorrent::AddTorrentParams params; |
|
||||||
if (downloadInWatchFolder(file)) |
|
||||||
{ |
|
||||||
params.savePath = QFileInfo(file).dir().path(); |
|
||||||
params.useAutoTMM = false; |
|
||||||
} |
|
||||||
else if (!downloadInDefaultFolder(file)) |
|
||||||
{ |
|
||||||
params.savePath = downloadPathTorrentFolder(file); |
|
||||||
params.useAutoTMM = false; |
|
||||||
} |
|
||||||
|
|
||||||
if (file.endsWith(".magnet", Qt::CaseInsensitive)) |
|
||||||
{ |
|
||||||
QFile f(file); |
|
||||||
if (f.open(QIODevice::ReadOnly | QIODevice::Text)) |
|
||||||
{ |
|
||||||
QTextStream str(&f); |
|
||||||
while (!str.atEnd()) |
|
||||||
BitTorrent::Session::instance()->addTorrent(str.readLine(), params); |
|
||||||
|
|
||||||
f.close(); |
|
||||||
Utils::Fs::forceRemove(file); |
|
||||||
} |
|
||||||
else |
|
||||||
{ |
|
||||||
qDebug("Failed to open magnet file: %s", qUtf8Printable(f.errorString())); |
|
||||||
} |
|
||||||
} |
|
||||||
else |
|
||||||
{ |
|
||||||
const BitTorrent::TorrentInfo torrentInfo = BitTorrent::TorrentInfo::loadFromFile(file); |
|
||||||
if (torrentInfo.isValid()) |
|
||||||
{ |
|
||||||
BitTorrent::Session::instance()->addTorrent(torrentInfo, params); |
|
||||||
Utils::Fs::forceRemove(file); |
|
||||||
} |
|
||||||
else |
|
||||||
{ |
|
||||||
qDebug("Ignoring incomplete torrent file: %s", qUtf8Printable(file)); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
QString ScanFoldersModel::pathTypeDisplayName(const PathType type) |
|
||||||
{ |
|
||||||
switch (type) |
|
||||||
{ |
|
||||||
case DOWNLOAD_IN_WATCH_FOLDER: |
|
||||||
return tr("Monitored folder"); |
|
||||||
case DEFAULT_LOCATION: |
|
||||||
return tr("Default save location"); |
|
||||||
case CUSTOM_LOCATION: |
|
||||||
return tr("Browse..."); |
|
||||||
default: |
|
||||||
qDebug("Invalid PathType: %d", type); |
|
||||||
}; |
|
||||||
return {}; |
|
||||||
} |
|
@ -1,111 +0,0 @@ |
|||||||
/*
|
|
||||||
* Bittorrent Client using Qt and libtorrent. |
|
||||||
* Copyright (C) 2010 Christian Kandeler, Christophe Dumez <chris@qbittorrent.org> |
|
||||||
* |
|
||||||
* This program is free software; you can redistribute it and/or |
|
||||||
* modify it under the terms of the GNU General Public License |
|
||||||
* as published by the Free Software Foundation; either version 2 |
|
||||||
* of the License, or (at your option) any later version. |
|
||||||
* |
|
||||||
* This program is distributed in the hope that it will be useful, |
|
||||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|
||||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|
||||||
* GNU General Public License for more details. |
|
||||||
* |
|
||||||
* You should have received a copy of the GNU General Public License |
|
||||||
* along with this program; if not, write to the Free Software |
|
||||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
|
||||||
* |
|
||||||
* In addition, as a special exception, the copyright holders give permission to |
|
||||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
|
||||||
* modified versions of it that use the same license as the "OpenSSL" library), |
|
||||||
* and distribute the linked executables. You must obey the GNU General Public |
|
||||||
* License in all respects for all of the code used other than "OpenSSL". If you |
|
||||||
* modify file(s), you may extend this exception to your version of the file(s), |
|
||||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
|
||||||
* exception statement from your version. |
|
||||||
*/ |
|
||||||
|
|
||||||
#pragma once |
|
||||||
|
|
||||||
#include <QAbstractListModel> |
|
||||||
#include <QList> |
|
||||||
#include <QtContainerFwd> |
|
||||||
|
|
||||||
class FileSystemWatcher; |
|
||||||
|
|
||||||
class ScanFoldersModel final : public QAbstractListModel |
|
||||||
{ |
|
||||||
Q_OBJECT |
|
||||||
Q_DISABLE_COPY(ScanFoldersModel) |
|
||||||
|
|
||||||
public: |
|
||||||
enum PathStatus |
|
||||||
{ |
|
||||||
Ok, |
|
||||||
DoesNotExist, |
|
||||||
CannotRead, |
|
||||||
CannotWrite, |
|
||||||
AlreadyInList |
|
||||||
}; |
|
||||||
|
|
||||||
enum Column |
|
||||||
{ |
|
||||||
WATCH, |
|
||||||
DOWNLOAD, |
|
||||||
NB_COLUMNS |
|
||||||
}; |
|
||||||
|
|
||||||
enum PathType |
|
||||||
{ |
|
||||||
DOWNLOAD_IN_WATCH_FOLDER, |
|
||||||
DEFAULT_LOCATION, |
|
||||||
CUSTOM_LOCATION |
|
||||||
}; |
|
||||||
|
|
||||||
static void initInstance(); |
|
||||||
static void freeInstance(); |
|
||||||
static ScanFoldersModel *instance(); |
|
||||||
|
|
||||||
static QString pathTypeDisplayName(PathType type); |
|
||||||
|
|
||||||
int rowCount(const QModelIndex &parent = {}) const override; |
|
||||||
int columnCount(const QModelIndex &parent = {}) const override; |
|
||||||
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override; |
|
||||||
QVariant headerData(int section, Qt::Orientation orientation, int role = Qt::DisplayRole) const override; |
|
||||||
Qt::ItemFlags flags(const QModelIndex &index) const override; |
|
||||||
|
|
||||||
// TODO: removePaths(); singular version becomes private helper functions;
|
|
||||||
// also: remove functions should take modelindexes
|
|
||||||
PathStatus addPath(const QString &watchPath, const PathType &downloadType, const QString &downloadPath, bool addToFSWatcher = true); |
|
||||||
PathStatus updatePath(const QString &watchPath, const PathType &downloadType, const QString &downloadPath); |
|
||||||
// PRECONDITION: The paths must have been added with addPath() first.
|
|
||||||
void addToFSWatcher(const QStringList &watchPaths); |
|
||||||
void removePath(int row, bool removeFromFSWatcher = true); |
|
||||||
bool removePath(const QString &path, bool removeFromFSWatcher = true); |
|
||||||
void removeFromFSWatcher(const QStringList &watchPaths); |
|
||||||
|
|
||||||
void makePersistent(); |
|
||||||
|
|
||||||
public slots: |
|
||||||
void configure(); |
|
||||||
|
|
||||||
private slots: |
|
||||||
void addTorrentsToSession(const QStringList &pathList); |
|
||||||
|
|
||||||
private: |
|
||||||
explicit ScanFoldersModel(QObject *parent = nullptr); |
|
||||||
~ScanFoldersModel(); |
|
||||||
|
|
||||||
virtual bool setData(const QModelIndex &index, const QVariant &value, int role = Qt::EditRole) override; |
|
||||||
bool downloadInWatchFolder(const QString &filePath) const; |
|
||||||
bool downloadInDefaultFolder(const QString &filePath) const; |
|
||||||
QString downloadPathTorrentFolder(const QString &filePath) const; |
|
||||||
int findPathData(const QString &path) const; |
|
||||||
|
|
||||||
static ScanFoldersModel *m_instance; |
|
||||||
struct PathData; |
|
||||||
|
|
||||||
QList<PathData*> m_pathList; |
|
||||||
FileSystemWatcher *m_fsWatcher; |
|
||||||
}; |
|
@ -0,0 +1,658 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2021 Vladimir Golovnev <glassez@yandex.ru> |
||||||
|
* Copyright (C) 2010 Christian Kandeler, Christophe Dumez <chris@qbittorrent.org> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "torrentfileswatcher.h" |
||||||
|
|
||||||
|
#include <chrono> |
||||||
|
|
||||||
|
#include <QtGlobal> |
||||||
|
#include <QDir> |
||||||
|
#include <QDirIterator> |
||||||
|
#include <QFile> |
||||||
|
#include <QFileSystemWatcher> |
||||||
|
#include <QJsonArray> |
||||||
|
#include <QJsonDocument> |
||||||
|
#include <QJsonObject> |
||||||
|
#include <QJsonValue> |
||||||
|
#include <QSaveFile> |
||||||
|
#include <QSet> |
||||||
|
#include <QTextStream> |
||||||
|
#include <QThread> |
||||||
|
#include <QTimer> |
||||||
|
#include <QVariant> |
||||||
|
|
||||||
|
#include "base/algorithm.h" |
||||||
|
#include "base/bittorrent/magneturi.h" |
||||||
|
#include "base/bittorrent/torrentcontentlayout.h" |
||||||
|
#include "base/bittorrent/session.h" |
||||||
|
#include "base/bittorrent/torrent.h" |
||||||
|
#include "base/bittorrent/torrentinfo.h" |
||||||
|
#include "base/exceptions.h" |
||||||
|
#include "base/global.h" |
||||||
|
#include "base/logger.h" |
||||||
|
#include "base/profile.h" |
||||||
|
#include "base/settingsstorage.h" |
||||||
|
#include "base/tagset.h" |
||||||
|
#include "base/utils/fs.h" |
||||||
|
#include "base/utils/string.h" |
||||||
|
|
||||||
|
using namespace std::chrono_literals; |
||||||
|
|
||||||
|
const std::chrono::duration WATCH_INTERVAL = 10s; |
||||||
|
const int MAX_FAILED_RETRIES = 5; |
||||||
|
const QString CONF_FILE_NAME {QStringLiteral("watched_folders.json")}; |
||||||
|
|
||||||
|
const QString OPTION_ADDTORRENTPARAMS {QStringLiteral("add_torrent_params")}; |
||||||
|
const QString OPTION_RECURSIVE {QStringLiteral("recursive")}; |
||||||
|
|
||||||
|
const QString PARAM_CATEGORY {QStringLiteral("category")}; |
||||||
|
const QString PARAM_TAGS {QStringLiteral("tags")}; |
||||||
|
const QString PARAM_SAVEPATH {QStringLiteral("save_path")}; |
||||||
|
const QString PARAM_OPERATINGMODE {QStringLiteral("operating_mode")}; |
||||||
|
const QString PARAM_STOPPED {QStringLiteral("stopped")}; |
||||||
|
const QString PARAM_CONTENTLAYOUT {QStringLiteral("content_layout")}; |
||||||
|
const QString PARAM_AUTOTMM {QStringLiteral("use_auto_tmm")}; |
||||||
|
const QString PARAM_UPLOADLIMIT {QStringLiteral("upload_limit")}; |
||||||
|
const QString PARAM_DOWNLOADLIMIT {QStringLiteral("download_limit")}; |
||||||
|
const QString PARAM_SEEDINGTIMELIMIT {QStringLiteral("seeding_time_limit")}; |
||||||
|
const QString PARAM_RATIOLIMIT {QStringLiteral("ratio_limit")}; |
||||||
|
|
||||||
|
namespace |
||||||
|
{ |
||||||
|
TagSet parseTagSet(const QJsonArray &jsonArr) |
||||||
|
{ |
||||||
|
TagSet tags; |
||||||
|
for (const QJsonValue &jsonVal : jsonArr) |
||||||
|
tags.insert(jsonVal.toString()); |
||||||
|
|
||||||
|
return tags; |
||||||
|
} |
||||||
|
|
||||||
|
QJsonArray serializeTagSet(const TagSet &tags) |
||||||
|
{ |
||||||
|
QJsonArray arr; |
||||||
|
for (const QString &tag : tags) |
||||||
|
arr.append(tag); |
||||||
|
|
||||||
|
return arr; |
||||||
|
} |
||||||
|
|
||||||
|
std::optional<bool> getOptionalBool(const QJsonObject &jsonObj, const QString &key) |
||||||
|
{ |
||||||
|
const QJsonValue jsonVal = jsonObj.value(key); |
||||||
|
if (jsonVal.isUndefined() || jsonVal.isNull()) |
||||||
|
return std::nullopt; |
||||||
|
|
||||||
|
return jsonVal.toBool(); |
||||||
|
} |
||||||
|
|
||||||
|
template <typename Enum> |
||||||
|
std::optional<Enum> getOptionalEnum(const QJsonObject &jsonObj, const QString &key) |
||||||
|
{ |
||||||
|
const QJsonValue jsonVal = jsonObj.value(key); |
||||||
|
if (jsonVal.isUndefined() || jsonVal.isNull()) |
||||||
|
return std::nullopt; |
||||||
|
|
||||||
|
return Utils::String::toEnum<Enum>(jsonVal.toString(), {}); |
||||||
|
} |
||||||
|
|
||||||
|
template <typename Enum> |
||||||
|
Enum getEnum(const QJsonObject &jsonObj, const QString &key) |
||||||
|
{ |
||||||
|
const QJsonValue jsonVal = jsonObj.value(key); |
||||||
|
return Utils::String::toEnum<Enum>(jsonVal.toString(), {}); |
||||||
|
} |
||||||
|
|
||||||
|
BitTorrent::AddTorrentParams parseAddTorrentParams(const QJsonObject &jsonObj) |
||||||
|
{ |
||||||
|
BitTorrent::AddTorrentParams params; |
||||||
|
params.category = jsonObj.value(PARAM_CATEGORY).toString(); |
||||||
|
params.tags = parseTagSet(jsonObj.value(PARAM_TAGS).toArray()); |
||||||
|
params.savePath = jsonObj.value(PARAM_SAVEPATH).toString(); |
||||||
|
params.addForced = (getEnum<BitTorrent::TorrentOperatingMode>(jsonObj, PARAM_OPERATINGMODE) == BitTorrent::TorrentOperatingMode::Forced); |
||||||
|
params.addPaused = getOptionalBool(jsonObj, PARAM_STOPPED); |
||||||
|
params.contentLayout = getOptionalEnum<BitTorrent::TorrentContentLayout>(jsonObj, PARAM_CONTENTLAYOUT); |
||||||
|
params.useAutoTMM = getOptionalBool(jsonObj, PARAM_AUTOTMM); |
||||||
|
params.uploadLimit = jsonObj.value(PARAM_UPLOADLIMIT).toInt(-1); |
||||||
|
params.downloadLimit = jsonObj.value(PARAM_DOWNLOADLIMIT).toInt(-1); |
||||||
|
params.seedingTimeLimit = jsonObj.value(PARAM_SEEDINGTIMELIMIT).toInt(BitTorrent::Torrent::USE_GLOBAL_SEEDING_TIME); |
||||||
|
params.ratioLimit = jsonObj.value(PARAM_RATIOLIMIT).toDouble(BitTorrent::Torrent::USE_GLOBAL_RATIO); |
||||||
|
|
||||||
|
return params; |
||||||
|
} |
||||||
|
|
||||||
|
QJsonObject serializeAddTorrentParams(const BitTorrent::AddTorrentParams ¶ms) |
||||||
|
{ |
||||||
|
QJsonObject jsonObj { |
||||||
|
{PARAM_CATEGORY, params.category}, |
||||||
|
{PARAM_TAGS, serializeTagSet(params.tags)}, |
||||||
|
{PARAM_SAVEPATH, params.savePath}, |
||||||
|
{PARAM_OPERATINGMODE, Utils::String::fromEnum(params.addForced |
||||||
|
? BitTorrent::TorrentOperatingMode::Forced : BitTorrent::TorrentOperatingMode::AutoManaged)}, |
||||||
|
{PARAM_UPLOADLIMIT, params.uploadLimit}, |
||||||
|
{PARAM_DOWNLOADLIMIT, params.downloadLimit}, |
||||||
|
{PARAM_SEEDINGTIMELIMIT, params.seedingTimeLimit}, |
||||||
|
{PARAM_RATIOLIMIT, params.ratioLimit} |
||||||
|
}; |
||||||
|
|
||||||
|
if (params.addPaused) |
||||||
|
jsonObj[PARAM_STOPPED] = *params.addPaused; |
||||||
|
if (params.contentLayout) |
||||||
|
jsonObj[PARAM_CONTENTLAYOUT] = Utils::String::fromEnum(*params.contentLayout); |
||||||
|
if (params.useAutoTMM) |
||||||
|
jsonObj[PARAM_AUTOTMM] = *params.useAutoTMM; |
||||||
|
|
||||||
|
return jsonObj; |
||||||
|
} |
||||||
|
|
||||||
|
TorrentFilesWatcher::WatchedFolderOptions parseWatchedFolderOptions(const QJsonObject &jsonObj) |
||||||
|
{ |
||||||
|
TorrentFilesWatcher::WatchedFolderOptions options; |
||||||
|
options.addTorrentParams = parseAddTorrentParams(jsonObj.value(OPTION_ADDTORRENTPARAMS).toObject()); |
||||||
|
options.recursive = jsonObj.value(OPTION_RECURSIVE).toBool(); |
||||||
|
|
||||||
|
return options; |
||||||
|
} |
||||||
|
|
||||||
|
QJsonObject serializeWatchedFolderOptions(const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
return { |
||||||
|
{OPTION_ADDTORRENTPARAMS, serializeAddTorrentParams(options.addTorrentParams)}, |
||||||
|
{OPTION_RECURSIVE, options.recursive} |
||||||
|
}; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
class TorrentFilesWatcher::Worker final : public QObject |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
Q_DISABLE_COPY(Worker) |
||||||
|
|
||||||
|
public: |
||||||
|
Worker(); |
||||||
|
|
||||||
|
public slots: |
||||||
|
void setWatchedFolder(const QString &path, const TorrentFilesWatcher::WatchedFolderOptions &options); |
||||||
|
void removeWatchedFolder(const QString &path); |
||||||
|
|
||||||
|
signals: |
||||||
|
void magnetFound(const BitTorrent::MagnetUri &magnetURI, const BitTorrent::AddTorrentParams &addTorrentParams); |
||||||
|
void torrentFound(const BitTorrent::TorrentInfo &torrentInfo, const BitTorrent::AddTorrentParams &addTorrentParams); |
||||||
|
|
||||||
|
private: |
||||||
|
void onTimeout(); |
||||||
|
void processWatchedFolder(const QString &path); |
||||||
|
void processFolder(const QString &path, const QString &watchedFolderPath, const TorrentFilesWatcher::WatchedFolderOptions &options); |
||||||
|
void processFailedTorrents(); |
||||||
|
void addWatchedFolder(const QString &watchedFolderID, const TorrentFilesWatcher::WatchedFolderOptions &options); |
||||||
|
void updateWatchedFolder(const QString &watchedFolderID, const TorrentFilesWatcher::WatchedFolderOptions &options); |
||||||
|
|
||||||
|
QFileSystemWatcher *m_watcher = nullptr; |
||||||
|
QTimer *m_watchTimer = nullptr; |
||||||
|
QHash<QString, TorrentFilesWatcher::WatchedFolderOptions> m_watchedFolders; |
||||||
|
QSet<QString> m_watchedByTimeoutFolders; |
||||||
|
|
||||||
|
// Failed torrents
|
||||||
|
QTimer *m_retryTorrentTimer = nullptr; |
||||||
|
QHash<QString, QHash<QString, int>> m_failedTorrents; |
||||||
|
}; |
||||||
|
|
||||||
|
TorrentFilesWatcher *TorrentFilesWatcher::m_instance = nullptr; |
||||||
|
|
||||||
|
void TorrentFilesWatcher::initInstance() |
||||||
|
{ |
||||||
|
if (!m_instance) |
||||||
|
m_instance = new TorrentFilesWatcher; |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::freeInstance() |
||||||
|
{ |
||||||
|
delete m_instance; |
||||||
|
m_instance = nullptr; |
||||||
|
} |
||||||
|
|
||||||
|
TorrentFilesWatcher *TorrentFilesWatcher::instance() |
||||||
|
{ |
||||||
|
return m_instance; |
||||||
|
} |
||||||
|
|
||||||
|
TorrentFilesWatcher::TorrentFilesWatcher(QObject *parent) |
||||||
|
: QObject {parent} |
||||||
|
, m_ioThread {new QThread(this)} |
||||||
|
, m_asyncWorker {new TorrentFilesWatcher::Worker} |
||||||
|
{ |
||||||
|
connect(m_asyncWorker, &TorrentFilesWatcher::Worker::magnetFound, this, &TorrentFilesWatcher::onMagnetFound); |
||||||
|
connect(m_asyncWorker, &TorrentFilesWatcher::Worker::torrentFound, this, &TorrentFilesWatcher::onTorrentFound); |
||||||
|
|
||||||
|
m_asyncWorker->moveToThread(m_ioThread); |
||||||
|
m_ioThread->start(); |
||||||
|
|
||||||
|
load(); |
||||||
|
} |
||||||
|
|
||||||
|
TorrentFilesWatcher::~TorrentFilesWatcher() |
||||||
|
{ |
||||||
|
m_ioThread->quit(); |
||||||
|
m_ioThread->wait(); |
||||||
|
delete m_asyncWorker; |
||||||
|
} |
||||||
|
|
||||||
|
QString TorrentFilesWatcher::makeCleanPath(const QString &path) |
||||||
|
{ |
||||||
|
if (path.isEmpty()) |
||||||
|
throw InvalidArgument(tr("Watched folder path cannot be empty.")); |
||||||
|
|
||||||
|
const QDir dir {path}; |
||||||
|
if (dir.isRelative()) |
||||||
|
throw InvalidArgument(tr("Watched folder path cannot be relative.")); |
||||||
|
|
||||||
|
return dir.canonicalPath(); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::load() |
||||||
|
{ |
||||||
|
QFile confFile {QDir(specialFolderLocation(SpecialFolder::Config)).absoluteFilePath(CONF_FILE_NAME)}; |
||||||
|
if (!confFile.exists()) |
||||||
|
{ |
||||||
|
loadLegacy(); |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
if (!confFile.open(QFile::ReadOnly)) |
||||||
|
{ |
||||||
|
LogMsg(tr("Couldn't load Watched Folders configuration from %1. Error: %2") |
||||||
|
.arg(confFile.fileName(), confFile.errorString()), Log::WARNING); |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
QJsonParseError jsonError; |
||||||
|
const QJsonDocument jsonDoc = QJsonDocument::fromJson(confFile.readAll(), &jsonError); |
||||||
|
if (jsonError.error != QJsonParseError::NoError) |
||||||
|
{ |
||||||
|
LogMsg(tr("Couldn't parse Watched Folders configuration from %1. Error: %2") |
||||||
|
.arg(confFile.fileName(), jsonError.errorString()), Log::WARNING); |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
if (!jsonDoc.isObject()) |
||||||
|
{ |
||||||
|
LogMsg(tr("Couldn't load Watched Folders configuration from %1. Invalid data format.") |
||||||
|
.arg(confFile.fileName()), Log::WARNING); |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
const QJsonObject jsonObj = jsonDoc.object(); |
||||||
|
for (auto it = jsonObj.constBegin(); it != jsonObj.constEnd(); ++it) |
||||||
|
{ |
||||||
|
const QString &watchedFolder = it.key(); |
||||||
|
const WatchedFolderOptions options = parseWatchedFolderOptions(it.value().toObject()); |
||||||
|
try |
||||||
|
{ |
||||||
|
doSetWatchedFolder(watchedFolder, options); |
||||||
|
} |
||||||
|
catch (const InvalidArgument &err) |
||||||
|
{ |
||||||
|
LogMsg(err.message(), Log::WARNING); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::loadLegacy() |
||||||
|
{ |
||||||
|
const auto dirs = SettingsStorage::instance()->loadValue<QVariantHash>("Preferences/Downloads/ScanDirsV2"); |
||||||
|
|
||||||
|
for (auto i = dirs.cbegin(); i != dirs.cend(); ++i) |
||||||
|
{ |
||||||
|
const QString watchedFolder = i.key(); |
||||||
|
BitTorrent::AddTorrentParams params; |
||||||
|
if (i.value().type() == QVariant::Int) |
||||||
|
{ |
||||||
|
if (i.value().toInt() == 0) |
||||||
|
{ |
||||||
|
params.savePath = watchedFolder; |
||||||
|
params.useAutoTMM = false; |
||||||
|
} |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
const QString customSavePath = i.value().toString(); |
||||||
|
params.savePath = customSavePath; |
||||||
|
params.useAutoTMM = false; |
||||||
|
} |
||||||
|
|
||||||
|
try |
||||||
|
{ |
||||||
|
doSetWatchedFolder(watchedFolder, {params, false}); |
||||||
|
} |
||||||
|
catch (const InvalidArgument &err) |
||||||
|
{ |
||||||
|
LogMsg(err.message(), Log::WARNING); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
store(); |
||||||
|
SettingsStorage::instance()->removeValue("Preferences/Downloads/ScanDirsV2"); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::store() const |
||||||
|
{ |
||||||
|
QJsonObject jsonObj; |
||||||
|
for (auto it = m_watchedFolders.cbegin(); it != m_watchedFolders.cend(); ++it) |
||||||
|
{ |
||||||
|
const QString &watchedFolder = it.key(); |
||||||
|
const WatchedFolderOptions &options = it.value(); |
||||||
|
jsonObj[watchedFolder] = serializeWatchedFolderOptions(options); |
||||||
|
} |
||||||
|
|
||||||
|
const QByteArray data = QJsonDocument(jsonObj).toJson(); |
||||||
|
|
||||||
|
QSaveFile confFile {QDir(specialFolderLocation(SpecialFolder::Config)).absoluteFilePath(CONF_FILE_NAME)}; |
||||||
|
if (!confFile.open(QIODevice::WriteOnly) || (confFile.write(data) != data.size()) || !confFile.commit()) |
||||||
|
{ |
||||||
|
LogMsg(tr("Couldn't store Watched Folders configuration to %1. Error: %2") |
||||||
|
.arg(confFile.fileName(), confFile.errorString()), Log::WARNING); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
QHash<QString, TorrentFilesWatcher::WatchedFolderOptions> TorrentFilesWatcher::folders() const |
||||||
|
{ |
||||||
|
return m_watchedFolders; |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::setWatchedFolder(const QString &path, const WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
doSetWatchedFolder(path, options); |
||||||
|
store(); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::doSetWatchedFolder(const QString &path, const WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
const QString cleanPath = makeCleanPath(path); |
||||||
|
m_watchedFolders[cleanPath] = options; |
||||||
|
|
||||||
|
QMetaObject::invokeMethod(m_asyncWorker, [this, path, options]() |
||||||
|
{ |
||||||
|
m_asyncWorker->setWatchedFolder(path, options); |
||||||
|
}); |
||||||
|
|
||||||
|
emit watchedFolderSet(cleanPath, options); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::removeWatchedFolder(const QString &path) |
||||||
|
{ |
||||||
|
const QString cleanPath = makeCleanPath(path); |
||||||
|
if (m_watchedFolders.remove(cleanPath)) |
||||||
|
{ |
||||||
|
QMetaObject::invokeMethod(m_asyncWorker, [this, cleanPath]() |
||||||
|
{ |
||||||
|
m_asyncWorker->removeWatchedFolder(cleanPath); |
||||||
|
}); |
||||||
|
|
||||||
|
emit watchedFolderRemoved(cleanPath); |
||||||
|
|
||||||
|
store(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::onMagnetFound(const BitTorrent::MagnetUri &magnetURI |
||||||
|
, const BitTorrent::AddTorrentParams &addTorrentParams) |
||||||
|
{ |
||||||
|
BitTorrent::Session::instance()->addTorrent(magnetURI, addTorrentParams); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::onTorrentFound(const BitTorrent::TorrentInfo &torrentInfo |
||||||
|
, const BitTorrent::AddTorrentParams &addTorrentParams) |
||||||
|
{ |
||||||
|
BitTorrent::Session::instance()->addTorrent(torrentInfo, addTorrentParams); |
||||||
|
} |
||||||
|
|
||||||
|
TorrentFilesWatcher::Worker::Worker() |
||||||
|
: m_watcher {new QFileSystemWatcher(this)} |
||||||
|
, m_watchTimer {new QTimer(this)} |
||||||
|
, m_retryTorrentTimer {new QTimer(this)} |
||||||
|
{ |
||||||
|
connect(m_watcher, &QFileSystemWatcher::directoryChanged, this, &Worker::processWatchedFolder); |
||||||
|
connect(m_watchTimer, &QTimer::timeout, this, &Worker::onTimeout); |
||||||
|
|
||||||
|
connect(m_retryTorrentTimer, &QTimer::timeout, this, &Worker::processFailedTorrents); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::onTimeout() |
||||||
|
{ |
||||||
|
for (const QString &path : asConst(m_watchedByTimeoutFolders)) |
||||||
|
processWatchedFolder(path); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::setWatchedFolder(const QString &path, const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
if (m_watchedFolders.contains(path)) |
||||||
|
updateWatchedFolder(path, options); |
||||||
|
else |
||||||
|
addWatchedFolder(path, options); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::removeWatchedFolder(const QString &path) |
||||||
|
{ |
||||||
|
m_watchedFolders.remove(path); |
||||||
|
|
||||||
|
m_watcher->removePath(path); |
||||||
|
m_watchedByTimeoutFolders.remove(path); |
||||||
|
if (m_watchedByTimeoutFolders.isEmpty()) |
||||||
|
m_watchTimer->stop(); |
||||||
|
|
||||||
|
m_failedTorrents.remove(path); |
||||||
|
if (m_failedTorrents.isEmpty()) |
||||||
|
m_retryTorrentTimer->stop(); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::processWatchedFolder(const QString &path) |
||||||
|
{ |
||||||
|
const TorrentFilesWatcher::WatchedFolderOptions options = m_watchedFolders.value(path); |
||||||
|
processFolder(path, path, options); |
||||||
|
|
||||||
|
if (!m_failedTorrents.empty() && !m_retryTorrentTimer->isActive()) |
||||||
|
m_retryTorrentTimer->start(WATCH_INTERVAL); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::processFolder(const QString &path, const QString &watchedFolderPath |
||||||
|
, const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
const QDir watchedDir {watchedFolderPath}; |
||||||
|
|
||||||
|
QDirIterator dirIter {path, {"*.torrent", "*.magnet"}, QDir::Files}; |
||||||
|
while (dirIter.hasNext()) |
||||||
|
{ |
||||||
|
const QString filePath = dirIter.next(); |
||||||
|
BitTorrent::AddTorrentParams addTorrentParams = options.addTorrentParams; |
||||||
|
if (path != watchedFolderPath) |
||||||
|
{ |
||||||
|
const QString subdirPath = watchedDir.relativeFilePath(path); |
||||||
|
addTorrentParams.savePath = QDir::cleanPath(QDir(addTorrentParams.savePath).filePath(subdirPath)); |
||||||
|
} |
||||||
|
|
||||||
|
if (filePath.endsWith(QLatin1String(".magnet"), Qt::CaseInsensitive)) |
||||||
|
{ |
||||||
|
QFile file {filePath}; |
||||||
|
if (file.open(QIODevice::ReadOnly | QIODevice::Text)) |
||||||
|
{ |
||||||
|
QTextStream str {&file}; |
||||||
|
while (!str.atEnd()) |
||||||
|
emit magnetFound(BitTorrent::MagnetUri(str.readLine()), addTorrentParams); |
||||||
|
|
||||||
|
file.close(); |
||||||
|
Utils::Fs::forceRemove(filePath); |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
LogMsg(tr("Failed to open magnet file: %1").arg(file.errorString())); |
||||||
|
} |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
const auto torrentInfo = BitTorrent::TorrentInfo::loadFromFile(filePath); |
||||||
|
if (torrentInfo.isValid()) |
||||||
|
{ |
||||||
|
emit torrentFound(torrentInfo, addTorrentParams); |
||||||
|
Utils::Fs::forceRemove(filePath); |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
if (!m_failedTorrents.value(path).contains(filePath)) |
||||||
|
{ |
||||||
|
m_failedTorrents[path][filePath] = 0; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
if (options.recursive) |
||||||
|
{ |
||||||
|
QDirIterator dirIter {path, (QDir::Dirs | QDir::NoDot | QDir::NoDotDot)}; |
||||||
|
while (dirIter.hasNext()) |
||||||
|
{ |
||||||
|
const QString folderPath = dirIter.next(); |
||||||
|
// Skip processing of subdirectory that is explicitly set as watched folder
|
||||||
|
if (!m_watchedFolders.contains(folderPath)) |
||||||
|
processFolder(folderPath, watchedFolderPath, options); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::processFailedTorrents() |
||||||
|
{ |
||||||
|
// Check which torrents are still partial
|
||||||
|
Algorithm::removeIf(m_failedTorrents, [this](const QString &watchedFolderPath, QHash<QString, int> &partialTorrents) |
||||||
|
{ |
||||||
|
const QDir dir {watchedFolderPath}; |
||||||
|
const TorrentFilesWatcher::WatchedFolderOptions options = m_watchedFolders.value(watchedFolderPath); |
||||||
|
Algorithm::removeIf(partialTorrents, [this, &dir, &options](const QString &torrentPath, int &value) |
||||||
|
{ |
||||||
|
if (!QFile::exists(torrentPath)) |
||||||
|
return true; |
||||||
|
|
||||||
|
const auto torrentInfo = BitTorrent::TorrentInfo::loadFromFile(torrentPath); |
||||||
|
if (torrentInfo.isValid()) |
||||||
|
{ |
||||||
|
BitTorrent::AddTorrentParams addTorrentParams = options.addTorrentParams; |
||||||
|
const QString exactDirPath = QFileInfo(torrentPath).canonicalPath(); |
||||||
|
if (exactDirPath != dir.path()) |
||||||
|
{ |
||||||
|
const QString subdirPath = dir.relativeFilePath(exactDirPath); |
||||||
|
addTorrentParams.savePath = QDir(addTorrentParams.savePath).filePath(subdirPath); |
||||||
|
} |
||||||
|
|
||||||
|
emit torrentFound(torrentInfo, addTorrentParams); |
||||||
|
Utils::Fs::forceRemove(torrentPath); |
||||||
|
|
||||||
|
return true; |
||||||
|
} |
||||||
|
|
||||||
|
if (value >= MAX_FAILED_RETRIES) |
||||||
|
{ |
||||||
|
LogMsg(tr("Rejecting failed torrent file: %1").arg(torrentPath)); |
||||||
|
QFile::rename(torrentPath, torrentPath + ".qbt_rejected"); |
||||||
|
return true; |
||||||
|
} |
||||||
|
|
||||||
|
++value; |
||||||
|
return false; |
||||||
|
}); |
||||||
|
|
||||||
|
if (partialTorrents.isEmpty()) |
||||||
|
return true; |
||||||
|
|
||||||
|
return false; |
||||||
|
}); |
||||||
|
|
||||||
|
// Stop the partial timer if necessary
|
||||||
|
if (m_failedTorrents.empty()) |
||||||
|
m_retryTorrentTimer->stop(); |
||||||
|
else |
||||||
|
m_retryTorrentTimer->start(WATCH_INTERVAL); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::addWatchedFolder(const QString &path, const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
#if !defined Q_OS_HAIKU |
||||||
|
// Check if the path points to a network file system or not
|
||||||
|
if (Utils::Fs::isNetworkFileSystem(path)) |
||||||
|
{ |
||||||
|
m_watchedByTimeoutFolders.insert(path); |
||||||
|
} |
||||||
|
else |
||||||
|
#endif |
||||||
|
if (options.recursive) |
||||||
|
{ |
||||||
|
m_watchedByTimeoutFolders.insert(path); |
||||||
|
if (!m_watchTimer->isActive()) |
||||||
|
m_watchTimer->start(WATCH_INTERVAL); |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
m_watcher->addPath(path); |
||||||
|
QTimer::singleShot(2000, this, [this, path]() { processWatchedFolder(path); }); |
||||||
|
} |
||||||
|
|
||||||
|
m_watchedFolders[path] = options; |
||||||
|
|
||||||
|
LogMsg(tr("Watching folder: \"%1\"").arg(Utils::Fs::toNativePath(path))); |
||||||
|
} |
||||||
|
|
||||||
|
void TorrentFilesWatcher::Worker::updateWatchedFolder(const QString &path, const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
const bool recursiveModeChanged = (m_watchedFolders[path].recursive != options.recursive); |
||||||
|
#if !defined Q_OS_HAIKU |
||||||
|
if (recursiveModeChanged && !Utils::Fs::isNetworkFileSystem(path)) |
||||||
|
#else |
||||||
|
if (recursiveModeChanged) |
||||||
|
#endif |
||||||
|
{ |
||||||
|
if (options.recursive) |
||||||
|
{ |
||||||
|
m_watcher->removePath(path); |
||||||
|
|
||||||
|
m_watchedByTimeoutFolders.insert(path); |
||||||
|
if (!m_watchTimer->isActive()) |
||||||
|
m_watchTimer->start(WATCH_INTERVAL); |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
m_watchedByTimeoutFolders.remove(path); |
||||||
|
if (m_watchedByTimeoutFolders.isEmpty()) |
||||||
|
m_watchTimer->stop(); |
||||||
|
|
||||||
|
m_watcher->addPath(path); |
||||||
|
QTimer::singleShot(2000, this, [this, path]() { processWatchedFolder(path); }); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
m_watchedFolders[path] = options; |
||||||
|
} |
||||||
|
|
||||||
|
#include "torrentfileswatcher.moc" |
@ -0,0 +1,96 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2021 Vladimir Golovnev <glassez@yandex.ru> |
||||||
|
* Copyright (C) 2010 Christian Kandeler, Christophe Dumez <chris@qbittorrent.org> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#pragma once |
||||||
|
|
||||||
|
#include <QHash> |
||||||
|
|
||||||
|
#include "base/bittorrent/addtorrentparams.h" |
||||||
|
|
||||||
|
class QThread; |
||||||
|
|
||||||
|
namespace BitTorrent |
||||||
|
{ |
||||||
|
class MagnetUri; |
||||||
|
} |
||||||
|
|
||||||
|
/*
|
||||||
|
* Watches the configured directories for new .torrent files in order |
||||||
|
* to add torrents to BitTorrent session. Supports Network File System |
||||||
|
* watching (NFS, CIFS) on Linux and Mac OS. |
||||||
|
*/ |
||||||
|
class TorrentFilesWatcher final : public QObject |
||||||
|
{ |
||||||
|
Q_OBJECT |
||||||
|
Q_DISABLE_COPY(TorrentFilesWatcher) |
||||||
|
|
||||||
|
public: |
||||||
|
struct WatchedFolderOptions |
||||||
|
{ |
||||||
|
BitTorrent::AddTorrentParams addTorrentParams; |
||||||
|
bool recursive = false; |
||||||
|
}; |
||||||
|
|
||||||
|
static void initInstance(); |
||||||
|
static void freeInstance(); |
||||||
|
static TorrentFilesWatcher *instance(); |
||||||
|
|
||||||
|
static QString makeCleanPath(const QString &path); |
||||||
|
|
||||||
|
QHash<QString, WatchedFolderOptions> folders() const; |
||||||
|
void setWatchedFolder(const QString &path, const WatchedFolderOptions &options); |
||||||
|
void removeWatchedFolder(const QString &path); |
||||||
|
|
||||||
|
signals: |
||||||
|
void watchedFolderSet(const QString &path, const WatchedFolderOptions &options); |
||||||
|
void watchedFolderRemoved(const QString &path); |
||||||
|
|
||||||
|
private slots: |
||||||
|
void onMagnetFound(const BitTorrent::MagnetUri &magnetURI, const BitTorrent::AddTorrentParams &addTorrentParams); |
||||||
|
void onTorrentFound(const BitTorrent::TorrentInfo &torrentInfo, const BitTorrent::AddTorrentParams &addTorrentParams); |
||||||
|
|
||||||
|
private: |
||||||
|
explicit TorrentFilesWatcher(QObject *parent = nullptr); |
||||||
|
~TorrentFilesWatcher() override; |
||||||
|
|
||||||
|
void load(); |
||||||
|
void loadLegacy(); |
||||||
|
void store() const; |
||||||
|
|
||||||
|
void doSetWatchedFolder(const QString &path, const WatchedFolderOptions &options); |
||||||
|
|
||||||
|
static TorrentFilesWatcher *m_instance; |
||||||
|
|
||||||
|
QHash<QString, WatchedFolderOptions> m_watchedFolders; |
||||||
|
|
||||||
|
QThread *m_ioThread = nullptr; |
||||||
|
|
||||||
|
class Worker; |
||||||
|
Worker *m_asyncWorker = nullptr; |
||||||
|
}; |
@ -1,118 +0,0 @@ |
|||||||
/*
|
|
||||||
* Bittorrent Client using Qt and libtorrent. |
|
||||||
* Copyright (C) 2015 sledgehammer999 <hammered999@gmail.com> |
|
||||||
* |
|
||||||
* This program is free software; you can redistribute it and/or |
|
||||||
* modify it under the terms of the GNU General Public License |
|
||||||
* as published by the Free Software Foundation; either version 2 |
|
||||||
* of the License, or (at your option) any later version. |
|
||||||
* |
|
||||||
* This program is distributed in the hope that it will be useful, |
|
||||||
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
|
||||||
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
|
||||||
* GNU General Public License for more details. |
|
||||||
* |
|
||||||
* You should have received a copy of the GNU General Public License |
|
||||||
* along with this program; if not, write to the Free Software |
|
||||||
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
|
||||||
* |
|
||||||
* In addition, as a special exception, the copyright holders give permission to |
|
||||||
* link this program with the OpenSSL project's "OpenSSL" library (or with |
|
||||||
* modified versions of it that use the same license as the "OpenSSL" library), |
|
||||||
* and distribute the linked executables. You must obey the GNU General Public |
|
||||||
* License in all respects for all of the code used other than "OpenSSL". If you |
|
||||||
* modify file(s), you may extend this exception to your version of the file(s), |
|
||||||
* but you are not obligated to do so. If you do not wish to do so, delete this |
|
||||||
* exception statement from your version. |
|
||||||
*/ |
|
||||||
|
|
||||||
#include "scanfoldersdelegate.h" |
|
||||||
|
|
||||||
#include <QComboBox> |
|
||||||
#include <QDebug> |
|
||||||
#include <QFileDialog> |
|
||||||
#include <QTreeView> |
|
||||||
|
|
||||||
#include "base/bittorrent/session.h" |
|
||||||
#include "base/scanfoldersmodel.h" |
|
||||||
|
|
||||||
ScanFoldersDelegate::ScanFoldersDelegate(QObject *parent, QTreeView *foldersView) |
|
||||||
: QStyledItemDelegate(parent) |
|
||||||
, m_folderView(foldersView) |
|
||||||
{ |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersDelegate::setEditorData(QWidget *editor, const QModelIndex &index) const |
|
||||||
{ |
|
||||||
auto *combobox = static_cast<QComboBox*>(editor); |
|
||||||
// Set combobox index
|
|
||||||
if (index.data(Qt::UserRole).toInt() == ScanFoldersModel::CUSTOM_LOCATION) |
|
||||||
combobox->setCurrentIndex(4); // '4' is the index of the item after the separator in the QComboBox menu
|
|
||||||
else |
|
||||||
combobox->setCurrentIndex(index.data(Qt::UserRole).toInt()); |
|
||||||
} |
|
||||||
|
|
||||||
QWidget *ScanFoldersDelegate::createEditor(QWidget *parent, const QStyleOptionViewItem &, const QModelIndex &index) const |
|
||||||
{ |
|
||||||
if (index.column() != ScanFoldersModel::DOWNLOAD) return nullptr; |
|
||||||
|
|
||||||
auto *editor = new QComboBox(parent); |
|
||||||
|
|
||||||
editor->setFocusPolicy(Qt::StrongFocus); |
|
||||||
editor->addItem(ScanFoldersModel::pathTypeDisplayName(ScanFoldersModel::DOWNLOAD_IN_WATCH_FOLDER)); |
|
||||||
editor->addItem(ScanFoldersModel::pathTypeDisplayName(ScanFoldersModel::DEFAULT_LOCATION)); |
|
||||||
editor->addItem(ScanFoldersModel::pathTypeDisplayName(ScanFoldersModel::CUSTOM_LOCATION)); |
|
||||||
if (index.data(Qt::UserRole).toInt() == ScanFoldersModel::CUSTOM_LOCATION) |
|
||||||
{ |
|
||||||
editor->insertSeparator(3); |
|
||||||
editor->addItem(index.data().toString()); |
|
||||||
} |
|
||||||
|
|
||||||
connect(editor, qOverload<int>(&QComboBox::currentIndexChanged) |
|
||||||
, this, &ScanFoldersDelegate::comboboxIndexChanged); |
|
||||||
return editor; |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersDelegate::comboboxIndexChanged(int index) |
|
||||||
{ |
|
||||||
if (index == ScanFoldersModel::CUSTOM_LOCATION) |
|
||||||
{ |
|
||||||
auto *w = static_cast<QWidget *>(sender()); |
|
||||||
if (w && w->parentWidget()) |
|
||||||
w->parentWidget()->setFocus(); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersDelegate::setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const |
|
||||||
{ |
|
||||||
auto *combobox = static_cast<QComboBox*>(editor); |
|
||||||
int value = combobox->currentIndex(); |
|
||||||
|
|
||||||
switch (value) |
|
||||||
{ |
|
||||||
case ScanFoldersModel::DOWNLOAD_IN_WATCH_FOLDER: |
|
||||||
case ScanFoldersModel::DEFAULT_LOCATION: |
|
||||||
model->setData(index, value, Qt::UserRole); |
|
||||||
break; |
|
||||||
|
|
||||||
case ScanFoldersModel::CUSTOM_LOCATION: |
|
||||||
model->setData( |
|
||||||
index, |
|
||||||
QFileDialog::getExistingDirectory( |
|
||||||
nullptr, tr("Select save location"), |
|
||||||
index.data(Qt::UserRole).toInt() == ScanFoldersModel::CUSTOM_LOCATION ? |
|
||||||
index.data().toString() : |
|
||||||
BitTorrent::Session::instance()->defaultSavePath()), |
|
||||||
Qt::DisplayRole); |
|
||||||
break; |
|
||||||
|
|
||||||
default: |
|
||||||
break; |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
void ScanFoldersDelegate::updateEditorGeometry(QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &) const |
|
||||||
{ |
|
||||||
qDebug("UpdateEditor Geometry called"); |
|
||||||
editor->setGeometry(option.rect); |
|
||||||
} |
|
@ -0,0 +1,153 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2021 Vladimir Golovnev <glassez@yandex.ru> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "watchedfolderoptionsdialog.h" |
||||||
|
|
||||||
|
#include <QDir> |
||||||
|
#include <QPushButton> |
||||||
|
|
||||||
|
#include "base/bittorrent/session.h" |
||||||
|
#include "base/global.h" |
||||||
|
#include "base/settingsstorage.h" |
||||||
|
#include "base/utils/fs.h" |
||||||
|
#include "ui_watchedfolderoptionsdialog.h" |
||||||
|
#include "utils.h" |
||||||
|
|
||||||
|
#define SETTINGS_KEY(name) "WatchedFolderOptionsDialog/" name |
||||||
|
|
||||||
|
WatchedFolderOptionsDialog::WatchedFolderOptionsDialog( |
||||||
|
const TorrentFilesWatcher::WatchedFolderOptions &watchedFolderOptions, QWidget *parent) |
||||||
|
: QDialog {parent} |
||||||
|
, m_ui {new Ui::WatchedFolderOptionsDialog} |
||||||
|
, m_savePath {watchedFolderOptions.addTorrentParams.savePath} |
||||||
|
, m_storeDialogSize {SETTINGS_KEY("DialogSize")} |
||||||
|
{ |
||||||
|
m_ui->setupUi(this); |
||||||
|
|
||||||
|
m_ui->savePath->setMode(FileSystemPathEdit::Mode::DirectorySave); |
||||||
|
m_ui->savePath->setDialogCaption(tr("Choose save path")); |
||||||
|
|
||||||
|
connect(m_ui->comboTTM, qOverload<int>(&QComboBox::currentIndexChanged), this, &WatchedFolderOptionsDialog::onTMMChanged); |
||||||
|
connect(m_ui->categoryComboBox, qOverload<int>(&QComboBox::currentIndexChanged), this, &WatchedFolderOptionsDialog::onCategoryChanged); |
||||||
|
|
||||||
|
m_ui->checkBoxRecursive->setChecked(watchedFolderOptions.recursive); |
||||||
|
populateSavePathComboBox(); |
||||||
|
|
||||||
|
const auto *session = BitTorrent::Session::instance(); |
||||||
|
const BitTorrent::AddTorrentParams &torrentParams = watchedFolderOptions.addTorrentParams; |
||||||
|
m_ui->startTorrentCheckBox->setChecked(!torrentParams.addPaused.value_or(session->isAddTorrentPaused())); |
||||||
|
m_ui->comboTTM->setCurrentIndex(torrentParams.useAutoTMM.value_or(!session->isAutoTMMDisabledByDefault())); |
||||||
|
m_ui->contentLayoutComboBox->setCurrentIndex( |
||||||
|
static_cast<int>(torrentParams.contentLayout.value_or(session->torrentContentLayout()))); |
||||||
|
|
||||||
|
// Load categories
|
||||||
|
QStringList categories = session->categories().keys(); |
||||||
|
std::sort(categories.begin(), categories.end(), Utils::Compare::NaturalLessThan<Qt::CaseInsensitive>()); |
||||||
|
|
||||||
|
if (!torrentParams.category.isEmpty()) |
||||||
|
m_ui->categoryComboBox->addItem(torrentParams.category); |
||||||
|
m_ui->categoryComboBox->addItem(""); |
||||||
|
|
||||||
|
for (const QString &category : asConst(categories)) |
||||||
|
{ |
||||||
|
if (category != torrentParams.category) |
||||||
|
m_ui->categoryComboBox->addItem(category); |
||||||
|
} |
||||||
|
|
||||||
|
loadState(); |
||||||
|
|
||||||
|
m_ui->buttonBox->button(QDialogButtonBox::Ok)->setFocus(); |
||||||
|
} |
||||||
|
|
||||||
|
WatchedFolderOptionsDialog::~WatchedFolderOptionsDialog() |
||||||
|
{ |
||||||
|
saveState(); |
||||||
|
delete m_ui; |
||||||
|
} |
||||||
|
|
||||||
|
TorrentFilesWatcher::WatchedFolderOptions WatchedFolderOptionsDialog::watchedFolderOptions() const |
||||||
|
{ |
||||||
|
TorrentFilesWatcher::WatchedFolderOptions watchedFolderOptions; |
||||||
|
watchedFolderOptions.recursive = m_ui->checkBoxRecursive->isChecked(); |
||||||
|
|
||||||
|
BitTorrent::AddTorrentParams ¶ms = watchedFolderOptions.addTorrentParams; |
||||||
|
params.useAutoTMM = (m_ui->comboTTM->currentIndex() == 1); |
||||||
|
if (!*params.useAutoTMM) |
||||||
|
params.savePath = m_ui->savePath->selectedPath(); |
||||||
|
params.category = m_ui->categoryComboBox->currentText();; |
||||||
|
params.addPaused = !m_ui->startTorrentCheckBox->isChecked(); |
||||||
|
params.contentLayout = static_cast<BitTorrent::TorrentContentLayout>(m_ui->contentLayoutComboBox->currentIndex()); |
||||||
|
|
||||||
|
return watchedFolderOptions; |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFolderOptionsDialog::loadState() |
||||||
|
{ |
||||||
|
Utils::Gui::resize(this, m_storeDialogSize); |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFolderOptionsDialog::saveState() |
||||||
|
{ |
||||||
|
m_storeDialogSize = size(); |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFolderOptionsDialog::onCategoryChanged(const int index) |
||||||
|
{ |
||||||
|
Q_UNUSED(index); |
||||||
|
|
||||||
|
const QString category = m_ui->categoryComboBox->currentText(); |
||||||
|
if (m_ui->comboTTM->currentIndex() == 1) |
||||||
|
{ |
||||||
|
const QString savePath = BitTorrent::Session::instance()->categorySavePath(category); |
||||||
|
m_ui->savePath->setSelectedPath(Utils::Fs::toNativePath(savePath)); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFolderOptionsDialog::populateSavePathComboBox() |
||||||
|
{ |
||||||
|
const QString defSavePath {BitTorrent::Session::instance()->defaultSavePath()}; |
||||||
|
m_ui->savePath->setSelectedPath(!m_savePath.isEmpty() ? m_savePath : defSavePath); |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFolderOptionsDialog::onTMMChanged(const int index) |
||||||
|
{ |
||||||
|
if (index != 1) |
||||||
|
{ // 0 is Manual mode and 1 is Automatic mode. Handle all non 1 values as manual mode.
|
||||||
|
populateSavePathComboBox(); |
||||||
|
m_ui->groupBoxSavePath->setEnabled(true); |
||||||
|
m_ui->savePath->blockSignals(false); |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
m_ui->groupBoxSavePath->setEnabled(false); |
||||||
|
m_ui->savePath->blockSignals(true); |
||||||
|
m_savePath = m_ui->savePath->selectedPath(); |
||||||
|
const QString savePath = BitTorrent::Session::instance()->categorySavePath(m_ui->categoryComboBox->currentText()); |
||||||
|
m_ui->savePath->setSelectedPath(savePath); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,315 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<ui version="4.0"> |
||||||
|
<class>WatchedFolderOptionsDialog</class> |
||||||
|
<widget class="QDialog" name="WatchedFolderOptionsDialog"> |
||||||
|
<property name="geometry"> |
||||||
|
<rect> |
||||||
|
<x>0</x> |
||||||
|
<y>0</y> |
||||||
|
<width>462</width> |
||||||
|
<height>306</height> |
||||||
|
</rect> |
||||||
|
</property> |
||||||
|
<property name="windowTitle"> |
||||||
|
<string>Watched Folder Options</string> |
||||||
|
</property> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout_3"> |
||||||
|
<item> |
||||||
|
<layout class="QHBoxLayout" name="horizontalLayout_6"> |
||||||
|
<item> |
||||||
|
<widget class="QCheckBox" name="checkBoxRecursive"> |
||||||
|
<property name="toolTip"> |
||||||
|
<string><html><head/><body><p>Will watch the folder and all its subfolders. In Manual torrent management mode it will also add subfolder name to the selected Save path.</p></body></html></string> |
||||||
|
</property> |
||||||
|
<property name="text"> |
||||||
|
<string>Recursive mode</string> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<spacer name="horizontalSpacer_2"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="sizeHint" stdset="0"> |
||||||
|
<size> |
||||||
|
<width>40</width> |
||||||
|
<height>20</height> |
||||||
|
</size> |
||||||
|
</property> |
||||||
|
</spacer> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QSplitter" name="splitter"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="childrenCollapsible"> |
||||||
|
<bool>false</bool> |
||||||
|
</property> |
||||||
|
<widget class="QFrame" name="torrentoptionsFrame"> |
||||||
|
<layout class="QVBoxLayout" name="mainlayout_addui"> |
||||||
|
<property name="leftMargin"> |
||||||
|
<number>0</number> |
||||||
|
</property> |
||||||
|
<property name="topMargin"> |
||||||
|
<number>0</number> |
||||||
|
</property> |
||||||
|
<property name="rightMargin"> |
||||||
|
<number>0</number> |
||||||
|
</property> |
||||||
|
<property name="bottomMargin"> |
||||||
|
<number>0</number> |
||||||
|
</property> |
||||||
|
<item> |
||||||
|
<widget class="QGroupBox" name="groupBoxParameters"> |
||||||
|
<property name="title"> |
||||||
|
<string>Torrent parameters</string> |
||||||
|
</property> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout_2"> |
||||||
|
<item> |
||||||
|
<layout class="QHBoxLayout" name="managementLayout"> |
||||||
|
<item> |
||||||
|
<widget class="QLabel" name="labelTorrentManagementMode"> |
||||||
|
<property name="text"> |
||||||
|
<string>Torrent Management Mode:</string> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QComboBox" name="comboTTM"> |
||||||
|
<property name="toolTip"> |
||||||
|
<string>Automatic mode means that various torrent properties(eg save path) will be decided by the associated category</string> |
||||||
|
</property> |
||||||
|
<item> |
||||||
|
<property name="text"> |
||||||
|
<string>Manual</string> |
||||||
|
</property> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<property name="text"> |
||||||
|
<string>Automatic</string> |
||||||
|
</property> |
||||||
|
</item> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<spacer name="horizontalSpacer"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="sizeHint" stdset="0"> |
||||||
|
<size> |
||||||
|
<width>20</width> |
||||||
|
<height>20</height> |
||||||
|
</size> |
||||||
|
</property> |
||||||
|
</spacer> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QGroupBox" name="groupBoxSavePath"> |
||||||
|
<property name="title"> |
||||||
|
<string>Save at</string> |
||||||
|
</property> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout"> |
||||||
|
<item> |
||||||
|
<widget class="FileSystemPathLineEdit" name="savePath" native="true"/> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<layout class="QHBoxLayout" name="categoryLayout"> |
||||||
|
<item> |
||||||
|
<widget class="QLabel" name="labelCategory"> |
||||||
|
<property name="text"> |
||||||
|
<string>Category:</string> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QComboBox" name="categoryComboBox"> |
||||||
|
<property name="sizePolicy"> |
||||||
|
<sizepolicy hsizetype="Expanding" vsizetype="Fixed"> |
||||||
|
<horstretch>0</horstretch> |
||||||
|
<verstretch>0</verstretch> |
||||||
|
</sizepolicy> |
||||||
|
</property> |
||||||
|
<property name="editable"> |
||||||
|
<bool>true</bool> |
||||||
|
</property> |
||||||
|
<property name="insertPolicy"> |
||||||
|
<enum>QComboBox::InsertAtTop</enum> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<spacer name="horizontalSpacer_7"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="sizeHint" stdset="0"> |
||||||
|
<size> |
||||||
|
<width>40</width> |
||||||
|
<height>20</height> |
||||||
|
</size> |
||||||
|
</property> |
||||||
|
</spacer> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<layout class="QVBoxLayout" name="verticalLayout_4"> |
||||||
|
<item> |
||||||
|
<layout class="QHBoxLayout" name="horizontalLayout_3"> |
||||||
|
<item> |
||||||
|
<widget class="QCheckBox" name="startTorrentCheckBox"> |
||||||
|
<property name="text"> |
||||||
|
<string>Start torrent</string> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<spacer name="horizontalSpacer_3"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="sizeHint" stdset="0"> |
||||||
|
<size> |
||||||
|
<width>40</width> |
||||||
|
<height>20</height> |
||||||
|
</size> |
||||||
|
</property> |
||||||
|
</spacer> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<layout class="QHBoxLayout" name="horizontalLayout_2"> |
||||||
|
<item> |
||||||
|
<widget class="QLabel" name="contentLayoutLabel"> |
||||||
|
<property name="text"> |
||||||
|
<string>Content layout:</string> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<widget class="QComboBox" name="contentLayoutComboBox"> |
||||||
|
<property name="currentIndex"> |
||||||
|
<number>0</number> |
||||||
|
</property> |
||||||
|
<item> |
||||||
|
<property name="text"> |
||||||
|
<string>Original</string> |
||||||
|
</property> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<property name="text"> |
||||||
|
<string>Create subfolder</string> |
||||||
|
</property> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<property name="text"> |
||||||
|
<string>Don't create subfolder</string> |
||||||
|
</property> |
||||||
|
</item> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<spacer name="horizontalSpacer_4"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Horizontal</enum> |
||||||
|
</property> |
||||||
|
<property name="sizeHint" stdset="0"> |
||||||
|
<size> |
||||||
|
<width>40</width> |
||||||
|
<height>20</height> |
||||||
|
</size> |
||||||
|
</property> |
||||||
|
</spacer> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<spacer name="verticalSpacer"> |
||||||
|
<property name="orientation"> |
||||||
|
<enum>Qt::Vertical</enum> |
||||||
|
</property> |
||||||
|
<property name="sizeHint" stdset="0"> |
||||||
|
<size> |
||||||
|
<width>20</width> |
||||||
|
<height>40</height> |
||||||
|
</size> |
||||||
|
</property> |
||||||
|
</spacer> |
||||||
|
</item> |
||||||
|
<item> |
||||||
|
<layout class="QHBoxLayout" name="horizontalLayout"> |
||||||
|
<item> |
||||||
|
<widget class="QDialogButtonBox" name="buttonBox"> |
||||||
|
<property name="standardButtons"> |
||||||
|
<set>QDialogButtonBox::Cancel|QDialogButtonBox::Ok</set> |
||||||
|
</property> |
||||||
|
</widget> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</item> |
||||||
|
</layout> |
||||||
|
</widget> |
||||||
|
<customwidgets> |
||||||
|
<customwidget> |
||||||
|
<class>FileSystemPathLineEdit</class> |
||||||
|
<extends>QWidget</extends> |
||||||
|
<header>gui/fspathedit.h</header> |
||||||
|
<container>1</container> |
||||||
|
</customwidget> |
||||||
|
</customwidgets> |
||||||
|
<resources/> |
||||||
|
<connections> |
||||||
|
<connection> |
||||||
|
<sender>buttonBox</sender> |
||||||
|
<signal>accepted()</signal> |
||||||
|
<receiver>WatchedFolderOptionsDialog</receiver> |
||||||
|
<slot>accept()</slot> |
||||||
|
<hints> |
||||||
|
<hint type="sourcelabel"> |
||||||
|
<x>928</x> |
||||||
|
<y>855</y> |
||||||
|
</hint> |
||||||
|
<hint type="destinationlabel"> |
||||||
|
<x>157</x> |
||||||
|
<y>274</y> |
||||||
|
</hint> |
||||||
|
</hints> |
||||||
|
</connection> |
||||||
|
<connection> |
||||||
|
<sender>buttonBox</sender> |
||||||
|
<signal>rejected()</signal> |
||||||
|
<receiver>WatchedFolderOptionsDialog</receiver> |
||||||
|
<slot>reject()</slot> |
||||||
|
<hints> |
||||||
|
<hint type="sourcelabel"> |
||||||
|
<x>928</x> |
||||||
|
<y>855</y> |
||||||
|
</hint> |
||||||
|
<hint type="destinationlabel"> |
||||||
|
<x>286</x> |
||||||
|
<y>274</y> |
||||||
|
</hint> |
||||||
|
</hints> |
||||||
|
</connection> |
||||||
|
</connections> |
||||||
|
</ui> |
@ -0,0 +1,178 @@ |
|||||||
|
/*
|
||||||
|
* Bittorrent Client using Qt and libtorrent. |
||||||
|
* Copyright (C) 2021 Vladimir Golovnev <glassez@yandex.ru> |
||||||
|
* |
||||||
|
* This program is free software; you can redistribute it and/or |
||||||
|
* modify it under the terms of the GNU General Public License |
||||||
|
* as published by the Free Software Foundation; either version 2 |
||||||
|
* of the License, or (at your option) any later version. |
||||||
|
* |
||||||
|
* This program is distributed in the hope that it will be useful, |
||||||
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of |
||||||
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
||||||
|
* GNU General Public License for more details. |
||||||
|
* |
||||||
|
* You should have received a copy of the GNU General Public License |
||||||
|
* along with this program; if not, write to the Free Software |
||||||
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. |
||||||
|
* |
||||||
|
* In addition, as a special exception, the copyright holders give permission to |
||||||
|
* link this program with the OpenSSL project's "OpenSSL" library (or with |
||||||
|
* modified versions of it that use the same license as the "OpenSSL" library), |
||||||
|
* and distribute the linked executables. You must obey the GNU General Public |
||||||
|
* License in all respects for all of the code used other than "OpenSSL". If you |
||||||
|
* modify file(s), you may extend this exception to your version of the file(s), |
||||||
|
* but you are not obligated to do so. If you do not wish to do so, delete this |
||||||
|
* exception statement from your version. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "watchedfoldersmodel.h" |
||||||
|
|
||||||
|
#include <QDir> |
||||||
|
|
||||||
|
#include "base/exceptions.h" |
||||||
|
#include "base/global.h" |
||||||
|
#include "base/utils/fs.h" |
||||||
|
|
||||||
|
WatchedFoldersModel::WatchedFoldersModel(TorrentFilesWatcher *fsWatcher, QObject *parent) |
||||||
|
: QAbstractListModel {parent} |
||||||
|
, m_fsWatcher {fsWatcher} |
||||||
|
, m_watchedFolders {m_fsWatcher->folders().keys()} |
||||||
|
, m_watchedFoldersOptions {m_fsWatcher->folders()} |
||||||
|
{ |
||||||
|
connect(m_fsWatcher, &TorrentFilesWatcher::watchedFolderSet, this, &WatchedFoldersModel::onFolderSet); |
||||||
|
connect(m_fsWatcher, &TorrentFilesWatcher::watchedFolderRemoved, this, &WatchedFoldersModel::onFolderRemoved); |
||||||
|
} |
||||||
|
|
||||||
|
int WatchedFoldersModel::rowCount(const QModelIndex &parent) const |
||||||
|
{ |
||||||
|
return parent.isValid() ? 0 : m_watchedFolders.count(); |
||||||
|
} |
||||||
|
|
||||||
|
int WatchedFoldersModel::columnCount(const QModelIndex &parent) const |
||||||
|
{ |
||||||
|
Q_UNUSED(parent); |
||||||
|
return 1; |
||||||
|
} |
||||||
|
|
||||||
|
QVariant WatchedFoldersModel::data(const QModelIndex &index, const int role) const |
||||||
|
{ |
||||||
|
if (!index.isValid() || (index.row() >= rowCount()) || (index.column() >= columnCount())) |
||||||
|
return {}; |
||||||
|
|
||||||
|
if (role == Qt::DisplayRole) |
||||||
|
return Utils::Fs::toNativePath(m_watchedFolders.at(index.row())); |
||||||
|
|
||||||
|
return {}; |
||||||
|
} |
||||||
|
|
||||||
|
QVariant WatchedFoldersModel::headerData(const int section, const Qt::Orientation orientation, const int role) const |
||||||
|
{ |
||||||
|
if ((orientation != Qt::Horizontal) || (role != Qt::DisplayRole) |
||||||
|
|| (section < 0) || (section >= columnCount())) |
||||||
|
{ |
||||||
|
return {}; |
||||||
|
} |
||||||
|
|
||||||
|
return tr("Watched Folder"); |
||||||
|
} |
||||||
|
|
||||||
|
bool WatchedFoldersModel::removeRows(const int row, const int count, const QModelIndex &parent) |
||||||
|
{ |
||||||
|
if (parent.isValid() || (row < 0) || (row >= rowCount()) |
||||||
|
|| (count <= 0) || ((row + count) > rowCount())) |
||||||
|
{ |
||||||
|
return false; |
||||||
|
} |
||||||
|
|
||||||
|
const int firstRow = row; |
||||||
|
const int lastRow = row + (count - 1); |
||||||
|
|
||||||
|
beginRemoveRows(parent, firstRow, lastRow); |
||||||
|
for (int i = firstRow; i <= lastRow; ++i) |
||||||
|
{ |
||||||
|
const QString folderPath = m_watchedFolders.takeAt(i); |
||||||
|
m_watchedFoldersOptions.remove(folderPath); |
||||||
|
m_deletedFolders.insert(folderPath); |
||||||
|
} |
||||||
|
endRemoveRows(); |
||||||
|
|
||||||
|
return true; |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFoldersModel::addFolder(const QString &path, const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
if (path.isEmpty()) |
||||||
|
throw InvalidArgument(tr("Watched folder path cannot be empty.")); |
||||||
|
|
||||||
|
const QDir watchDir {path}; |
||||||
|
const QString canonicalWatchPath = watchDir.canonicalPath(); |
||||||
|
if (m_watchedFoldersOptions.contains(canonicalWatchPath)) |
||||||
|
throw RuntimeError(tr("Folder '%1' is already in watch list.").arg(path)); |
||||||
|
if (!watchDir.exists()) |
||||||
|
throw RuntimeError(tr("Folder '%1' doesn't exist.").arg(path)); |
||||||
|
if (!watchDir.isReadable()) |
||||||
|
throw RuntimeError(tr("Folder '%1' isn't readable.").arg(path)); |
||||||
|
|
||||||
|
m_deletedFolders.remove(canonicalWatchPath); |
||||||
|
|
||||||
|
beginInsertRows(QModelIndex(), rowCount(), rowCount()); |
||||||
|
m_watchedFolders.append(canonicalWatchPath); |
||||||
|
m_watchedFoldersOptions[canonicalWatchPath] = options; |
||||||
|
endInsertRows(); |
||||||
|
} |
||||||
|
|
||||||
|
TorrentFilesWatcher::WatchedFolderOptions WatchedFoldersModel::folderOptions(const int row) const |
||||||
|
{ |
||||||
|
Q_ASSERT((row >= 0) && (row < rowCount())); |
||||||
|
|
||||||
|
const QString folderPath = m_watchedFolders.at(row); |
||||||
|
return m_watchedFoldersOptions[folderPath]; |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFoldersModel::setFolderOptions(const int row, const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
Q_ASSERT((row >= 0) && (row < rowCount())); |
||||||
|
|
||||||
|
const QString folderPath = m_watchedFolders.at(row); |
||||||
|
m_watchedFoldersOptions[folderPath] = options; |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFoldersModel::apply() |
||||||
|
{ |
||||||
|
const QSet<QString> deletedFolders {m_deletedFolders}; |
||||||
|
// We have to clear `m_deletedFolders` for optimization reason, otherwise
|
||||||
|
// it will be cleared one element at a time in `onFolderRemoved()` handler
|
||||||
|
m_deletedFolders.clear(); |
||||||
|
for (const QString &path : deletedFolders) |
||||||
|
m_fsWatcher->removeWatchedFolder(path); |
||||||
|
|
||||||
|
for (const QString &path : asConst(m_watchedFolders)) |
||||||
|
m_fsWatcher->setWatchedFolder(path, m_watchedFoldersOptions.value(path)); |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFoldersModel::onFolderSet(const QString &path, const TorrentFilesWatcher::WatchedFolderOptions &options) |
||||||
|
{ |
||||||
|
if (!m_watchedFoldersOptions.contains(path)) |
||||||
|
{ |
||||||
|
m_deletedFolders.remove(path); |
||||||
|
|
||||||
|
beginInsertRows(QModelIndex(), rowCount(), rowCount()); |
||||||
|
m_watchedFolders.append(path); |
||||||
|
m_watchedFoldersOptions[path] = options; |
||||||
|
endInsertRows(); |
||||||
|
} |
||||||
|
else |
||||||
|
{ |
||||||
|
m_watchedFoldersOptions[path] = options; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
void WatchedFoldersModel::onFolderRemoved(const QString &path) |
||||||
|
{ |
||||||
|
const int row = m_watchedFolders.indexOf(path); |
||||||
|
if (row >= 0) |
||||||
|
removeRows(row, 1); |
||||||
|
|
||||||
|
m_deletedFolders.remove(path); |
||||||
|
} |
Loading…
Reference in new issue