mirror of git://erdgeist.org/opentracker
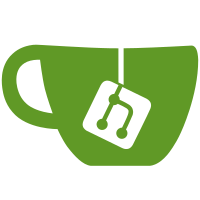
commit
ad472597c5
3 changed files with 572 additions and 0 deletions
@ -0,0 +1,11 @@
@@ -0,0 +1,11 @@
|
||||
CC?=gcc |
||||
CFLAGS+=-I../libowfat -Wall -O2 -pipe |
||||
LDFLAGS+=-L../libowfat/ -lowfat -s |
||||
|
||||
SOURCES=opentracker.c trackerlogic.c |
||||
|
||||
opentracker: $(SOURCES) |
||||
$(CC) $(SOURCES) -o opentracker $(CFLAGS) $(LDFLAGS) |
||||
|
||||
clean: |
||||
rm -rf opentracker |
@ -0,0 +1,288 @@
@@ -0,0 +1,288 @@
|
||||
#include "socket.h" |
||||
#include "io.h" |
||||
#include "buffer.h" |
||||
#include "ip6.h" |
||||
#include "array.h" |
||||
#include "case.h" |
||||
#include "fmt.h" |
||||
#include "iob.h" |
||||
#include "str.h" |
||||
#include <sys/types.h> |
||||
#include <sys/stat.h> |
||||
#include <unistd.h> |
||||
#include <stdlib.h> |
||||
#include <errno.h> |
||||
|
||||
static void carp(const char* routine) { |
||||
buffer_puts(buffer_2,routine); |
||||
buffer_puts(buffer_2,": "); |
||||
buffer_puterror(buffer_2); |
||||
buffer_putnlflush(buffer_2); |
||||
} |
||||
|
||||
static void panic(const char* routine) { |
||||
carp(routine); |
||||
exit(111); |
||||
} |
||||
|
||||
struct http_data { |
||||
array r; |
||||
io_batch iob; |
||||
char* hdrbuf; |
||||
int hlen; |
||||
int keepalive; |
||||
}; |
||||
|
||||
int header_complete(struct http_data* r) { |
||||
long i; |
||||
long l=array_bytes(&r->r); |
||||
const char* c=array_start(&r->r); |
||||
for (i=0; i+1<l; ++i) { |
||||
if (c[i]=='\n' && c[i+1]=='\n') |
||||
return i+2; |
||||
if (i+3<l && |
||||
c[i]=='\r' && c[i+1]=='\n' && |
||||
c[i+2]=='\r' && c[i+3]=='\n') |
||||
return i+4; |
||||
} |
||||
return 0; |
||||
} |
||||
|
||||
void httperror(struct http_data* r,const char* title,const char* message) { |
||||
char* c; |
||||
c=r->hdrbuf=(char*)malloc(strlen(message)+strlen(title)+200); |
||||
if (!c) { |
||||
r->hdrbuf="HTTP/1.0 500 internal error\r\nContent-Type: text/plain\r\nConnection: close\r\n\r\nout of memory\n"; |
||||
r->hlen=strlen(r->hdrbuf); |
||||
} else { |
||||
c+=fmt_str(c,"HTTP/1.0 "); |
||||
c+=fmt_str(c,title); |
||||
c+=fmt_str(c,"\r\nContent-Type: text/html\r\nConnection: "); |
||||
c+=fmt_str(c,r->keepalive?"keep-alive":"close"); |
||||
c+=fmt_str(c,"\r\nContent-Length: "); |
||||
c+=fmt_ulong(c,strlen(message)+strlen(title)+16-4); |
||||
c+=fmt_str(c,"\r\n\r\n<title>"); |
||||
c+=fmt_str(c,title+4); |
||||
c+=fmt_str(c,"</title>\n"); |
||||
r->hlen=c - r->hdrbuf; |
||||
} |
||||
iob_addbuf(&r->iob,r->hdrbuf,r->hlen); |
||||
} |
||||
|
||||
static struct mimeentry { const char* name, *type; } mimetab[] = { |
||||
{ "html", "text/html" }, |
||||
{ "css", "text/css" }, |
||||
{ "dvi", "application/x-dvi" }, |
||||
{ "ps", "application/postscript" }, |
||||
{ "pdf", "application/pdf" }, |
||||
{ "gif", "image/gif" }, |
||||
{ "png", "image/png" }, |
||||
{ "jpeg", "image/jpeg" }, |
||||
{ "jpg", "image/jpeg" }, |
||||
{ "mpeg", "video/mpeg" }, |
||||
{ "mpg", "video/mpeg" }, |
||||
{ "avi", "video/x-msvideo" }, |
||||
{ "mov", "video/quicktime" }, |
||||
{ "qt", "video/quicktime" }, |
||||
{ "mp3", "audio/mpeg" }, |
||||
{ "ogg", "audio/x-oggvorbis" }, |
||||
{ "wav", "audio/x-wav" }, |
||||
{ "pac", "application/x-ns-proxy-autoconfig" }, |
||||
{ "sig", "application/pgp-signature" }, |
||||
{ "torrent", "application/x-bittorrent" }, |
||||
{ "class", "application/octet-stream" }, |
||||
{ "js", "application/x-javascript" }, |
||||
{ "tar", "application/x-tar" }, |
||||
{ "zip", "application/zip" }, |
||||
{ "dtd", "text/xml" }, |
||||
{ "xml", "text/xml" }, |
||||
{ "xbm", "image/x-xbitmap" }, |
||||
{ "xpm", "image/x-xpixmap" }, |
||||
{ "xwd", "image/x-xwindowdump" }, |
||||
{ 0,0 } }; |
||||
|
||||
const char* mimetype(const char* filename) { |
||||
int i,e=str_rchr(filename,'.'); |
||||
if (filename[e]==0) return "text/plain"; |
||||
++e; |
||||
for (i=0; mimetab[i].name; ++i) |
||||
if (str_equal(mimetab[i].name,filename+e)) |
||||
return mimetab[i].type; |
||||
return "application/octet-stream"; |
||||
} |
||||
|
||||
const char* http_header(struct http_data* r,const char* h) { |
||||
long i; |
||||
long l=array_bytes(&r->r); |
||||
long sl=strlen(h); |
||||
const char* c=array_start(&r->r); |
||||
for (i=0; i+sl+2<l; ++i) |
||||
if (c[i]=='\n' && case_equalb(c+i+1,sl,h) && c[i+sl+1]==':') { |
||||
c+=i+sl+1; |
||||
if (*c==' ' || *c=='\t') ++c; |
||||
return c; |
||||
} |
||||
return 0; |
||||
} |
||||
|
||||
void httpresponse(struct http_data* h,int64 s) { |
||||
char* c; |
||||
const char* m; |
||||
array_cat0(&h->r); |
||||
c=array_start(&h->r); |
||||
if (byte_diff(c,4,"GET ")) { |
||||
e400: |
||||
httperror(h,"400 Invalid Request","This server only understands GET."); |
||||
} else { |
||||
char *d; |
||||
int64 fd; |
||||
struct stat s; |
||||
c+=4; |
||||
for (d=c; *d!=' '&&*d!='\t'&&*d!='\n'&&*d!='\r'; ++d) ; |
||||
if (*d!=' ') goto e400; |
||||
*d=0; |
||||
if (c[0]!='/') goto e404; |
||||
while (c[1]=='/') ++c; |
||||
if (!io_readfile(&fd,c+1)) { |
||||
e404: |
||||
httperror(h,"404 Not Found","No such file or directory."); |
||||
} else { |
||||
if (fstat(fd,&s)==-1) { |
||||
io_close(fd); |
||||
goto e404; |
||||
} |
||||
if ((m=http_header(h,"Connection"))) { |
||||
if (str_equal(m,"keep-alive")) |
||||
h->keepalive=1; |
||||
else |
||||
h->keepalive=0; |
||||
} else { |
||||
if (byte_equal(d+1,8,"HTTP/1.0")) |
||||
h->keepalive=0; |
||||
else |
||||
h->keepalive=1; |
||||
} |
||||
m=mimetype(c); |
||||
c=h->hdrbuf=(char*)malloc(500); |
||||
c+=fmt_str(c,"HTTP/1.1 Coming Up\r\nContent-Type: "); |
||||
c+=fmt_str(c,m); |
||||
c+=fmt_str(c,"\r\nContent-Length: "); |
||||
c+=fmt_ulonglong(c,s.st_size); |
||||
c+=fmt_str(c,"\r\nLast-Modified: "); |
||||
c+=fmt_httpdate(c,s.st_mtime); |
||||
c+=fmt_str(c,"\r\nConnection: "); |
||||
c+=fmt_str(c,h->keepalive?"keep-alive":"close"); |
||||
c+=fmt_str(c,"\r\n\r\n"); |
||||
iob_addbuf(&h->iob,h->hdrbuf,c - h->hdrbuf); |
||||
iob_addfile(&h->iob,fd,0,s.st_size); |
||||
} |
||||
} |
||||
io_dontwantread(s); |
||||
io_wantwrite(s); |
||||
} |
||||
|
||||
int main() { |
||||
int s=socket_tcp6b(); |
||||
uint32 scope_id; |
||||
char ip[16]; |
||||
uint16 port; |
||||
if (socket_bind6_reuse(s,V6any,8000,0)==-1) |
||||
panic("socket_bind6_reuse"); |
||||
if (socket_listen(s,16)==-1) |
||||
panic("socket_listen"); |
||||
if (!io_fd(s)) |
||||
panic("io_fd"); |
||||
io_wantread(s); |
||||
for (;;) { |
||||
int64 i; |
||||
io_wait(); |
||||
while ((i=io_canread())!=-1) { |
||||
if (i==s) { |
||||
int n; |
||||
while ((n=socket_accept6(s,ip,&port,&scope_id))!=-1) { |
||||
char buf[IP6_FMT]; |
||||
buffer_puts(buffer_2,"accepted new connection from "); |
||||
buffer_put(buffer_2,buf,fmt_ip6(buf,ip)); |
||||
buffer_puts(buffer_2,":"); |
||||
buffer_putulong(buffer_2,port); |
||||
buffer_puts(buffer_2," (fd "); |
||||
buffer_putulong(buffer_2,n); |
||||
buffer_puts(buffer_2,")"); |
||||
if (io_fd(n)) { |
||||
struct http_data* h=(struct http_data*)malloc(sizeof(struct http_data)); |
||||
io_wantread(n); |
||||
if (h) { |
||||
byte_zero(h,sizeof(struct http_data)); |
||||
io_setcookie(n,h); |
||||
} else |
||||
io_close(n); |
||||
} else { |
||||
buffer_puts(buffer_2,", but io_fd failed."); |
||||
io_close(n); |
||||
} |
||||
buffer_putnlflush(buffer_2); |
||||
} |
||||
if (errno==EAGAIN) |
||||
io_eagain(s); |
||||
else |
||||
carp("socket_accept6"); |
||||
} else { |
||||
char buf[8192]; |
||||
struct http_data* h=io_getcookie(i); |
||||
int l=io_tryread(i,buf,sizeof buf); |
||||
if (l==-3) { |
||||
if (h) { |
||||
array_reset(&h->r); |
||||
iob_reset(&h->iob); |
||||
free(h->hdrbuf); h->hdrbuf=0; |
||||
} |
||||
buffer_puts(buffer_2,"io_tryread("); |
||||
buffer_putulong(buffer_2,i); |
||||
buffer_puts(buffer_2,"): "); |
||||
buffer_puterror(buffer_2); |
||||
buffer_putnlflush(buffer_2); |
||||
io_close(i); |
||||
} else if (l==0) { |
||||
if (h) { |
||||
array_reset(&h->r); |
||||
iob_reset(&h->iob); |
||||
free(h->hdrbuf); h->hdrbuf=0; |
||||
} |
||||
buffer_puts(buffer_2,"eof on fd #"); |
||||
buffer_putulong(buffer_2,i); |
||||
buffer_putnlflush(buffer_2); |
||||
io_close(i); |
||||
} else if (l>0) { |
||||
array_catb(&h->r,buf,l); |
||||
if (array_failed(&h->r)) { |
||||
httperror(h,"500 Server Error","request too long."); |
||||
emerge: |
||||
io_dontwantread(i); |
||||
io_wantwrite(i); |
||||
} else if (array_bytes(&h->r)>8192) { |
||||
httperror(h,"500 request too long","You sent too much headers"); |
||||
goto emerge; |
||||
} else if ((l=header_complete(h))) |
||||
httpresponse(h,i); |
||||
} |
||||
} |
||||
} |
||||
while ((i=io_canwrite())!=-1) { |
||||
struct http_data* h=io_getcookie(i); |
||||
int64 r=iob_send(i,&h->iob); |
||||
/* printf("iob_send returned %lld\n",r); */ |
||||
if (r==-1) io_eagain(i); else |
||||
if (r<=0) { |
||||
array_trunc(&h->r); |
||||
iob_reset(&h->iob); |
||||
free(h->hdrbuf); h->hdrbuf=0; |
||||
if (h->keepalive) { |
||||
io_dontwantwrite(i); |
||||
io_wantread(i); |
||||
} else |
||||
io_close(i); |
||||
} |
||||
} |
||||
} |
||||
return 0; |
||||
} |
@ -0,0 +1,273 @@
@@ -0,0 +1,273 @@
|
||||
// THIS REALLY BELONGS INTO A HEADER FILE
|
||||
//
|
||||
//
|
||||
#include <string.h> |
||||
#include <stdio.h> |
||||
#include <fcntl.h> |
||||
#include <sys/types.h> |
||||
#include <sys/mman.h> |
||||
|
||||
typedef unsigned char ot_hash[20]; |
||||
typedef unsigned char ot_ip[ 4/*0*/ ]; |
||||
typedef unsigned long ot_time; |
||||
// tunables
|
||||
const unsigned long OT_TIMEOUT = 2700; |
||||
const unsigned long OT_HUGE_FILESIZE = 1024*1024*256; // Thats 256MB per file, enough for 204800 peers of 128 bytes
|
||||
|
||||
#define OT_COMPACT_ONLY |
||||
|
||||
#define MEMMOVE memmove |
||||
#define BZERO bzero |
||||
#define FORMAT_FIXED_STRING sprintf |
||||
#define FORMAT_FORMAT_STRING sprintf |
||||
#define BINARY_FIND binary_search |
||||
|
||||
typedef struct { |
||||
#ifndef OT_COMPACT_ONLY |
||||
ot_hash id; |
||||
ot_hash key; |
||||
#endif |
||||
ot_ip ip; |
||||
unsigned short port; |
||||
ot_time death; |
||||
unsigned char flags; |
||||
} ot_peer; |
||||
unsigned char PEER_FLAG_SEEDING = 0x80; |
||||
unsigned char PEER_IP_LENGTH_MASK = 0x3f; |
||||
|
||||
typedef struct { |
||||
ot_hash hash; |
||||
ot_peer *peer_list; |
||||
unsigned long peer_count; |
||||
unsigned long seed_count; |
||||
} ot_torrent; |
||||
|
||||
void *map_file( char *file_name ); |
||||
|
||||
// This behaves quite like bsearch but allows to find
|
||||
// the insertion point for inserts after unsuccessful searches
|
||||
// in this case exactmatch is 0 on exit
|
||||
//
|
||||
void *binary_search( const void *key, const void *base, |
||||
const unsigned long member_count, const unsigned long member_size, |
||||
int (*compar) (const void *, const void *), |
||||
int *exactmatch ); |
||||
|
||||
int compare_hash( const void *hash1, const void *hash2 ) { return memcmp( hash1, hash2, sizeof( ot_hash )); } |
||||
int compare_ip_port( const void *peer1, const void *peer2 ) { return memcmp( peer1, peer2, 6); } |
||||
|
||||
//
|
||||
//
|
||||
// END OF STUFF THAT BELONGS INTO A HEADER FILE
|
||||
|
||||
ot_torrent *torrents_pointer = 0; |
||||
unsigned long torrents_count = 0; |
||||
unsigned char *scratchspace; |
||||
|
||||
ot_torrent *add_peer_to_torrent( ot_hash hash, ot_peer *peer ) { |
||||
ot_torrent *torrent; |
||||
ot_peer *peer_dest; |
||||
int exactmatch; |
||||
|
||||
torrent = BINARY_FIND( hash, torrents_pointer, torrents_count, sizeof( ot_torrent ), compare_hash, &exactmatch ); |
||||
if( !exactmatch ) { |
||||
// Assume, OS will provide us with space, after all, this is file backed
|
||||
MEMMOVE( torrent + 1, torrent, ( torrents_pointer + torrents_count ) - torrent ); |
||||
|
||||
// Create a new torrent entry, then
|
||||
MEMMOVE( &torrent->hash, hash, sizeof( ot_hash ) ); |
||||
torrent->peer_list = map_file( hash ); |
||||
torrent->peer_count = 0; |
||||
torrent->seed_count = 0; |
||||
} |
||||
|
||||
peer_dest = BINARY_FIND( peer, torrent->peer_list, torrent->peer_count, sizeof( ot_peer ), compare_ip_port, &exactmatch ); |
||||
if( exactmatch ) { |
||||
// If peer was a seeder but isn't anymore, decrease seeder count
|
||||
if( ( peer_dest->flags & PEER_FLAG_SEEDING ) && !( peer->flags & PEER_FLAG_SEEDING ) ) |
||||
torrent->seed_count--; |
||||
if( !( peer_dest->flags & PEER_FLAG_SEEDING ) && ( peer->flags & PEER_FLAG_SEEDING ) ) |
||||
torrent->seed_count++; |
||||
} else { |
||||
// Assume, OS will provide us with space, after all, this is file backed
|
||||
MEMMOVE( peer_dest + 1, peer_dest, ( torrent->peer_list + torrent->peer_count ) - peer_dest ); |
||||
|
||||
// Create a new peer entry, then
|
||||
MEMMOVE( peer_dest, peer, sizeof( ot_peer ) ); |
||||
|
||||
torrent->peer_count++; |
||||
torrent->seed_count+= ( peer->flags & PEER_FLAG_SEEDING ) ? 1 : 0; |
||||
} |
||||
|
||||
// Set new time out time
|
||||
peer_dest->death = now() + OT_TIMEOUT; |
||||
|
||||
return torrent; |
||||
} |
||||
|
||||
#define SETINVALID( i ) (scratchspace[index] = 3); |
||||
#define SETSELECTED( i ) (scratchspace[index] = 1); |
||||
#define TESTSELECTED( i ) (scratchspace[index] == 1 ) |
||||
#define TESTSET( i ) (scratchspace[index]) |
||||
#define RANDOM random() |
||||
|
||||
inline int TESTVALIDPEER( ot_peer *p ) { return p->death > now(); } |
||||
|
||||
// Compiles a list of random peers for a torrent
|
||||
// * scratch space keeps track of death or already selected peers
|
||||
// * reply must have enough space to hold 1+(1+16+2+1)*amount+1 bytes
|
||||
// * Selector function can be anything, maybe test for seeds, etc.
|
||||
// * that RANDOM may return huge values
|
||||
// * does not yet check not to return self
|
||||
// * it is not guaranteed to see all peers, so no assumptions on active seeders/peers may be done
|
||||
// * since compact format cannot handle v6 addresses, it must be enabled by OT_COMPACT_ONLY
|
||||
//
|
||||
void return_peers_for_torrent( ot_torrent *torrent, unsigned long amount, char *reply ) { |
||||
register ot_peer *peer_base = torrent->peer_list; |
||||
unsigned long peer_count = torrent->peer_count; |
||||
unsigned long selected_count = 0, invalid_count = 0; |
||||
unsigned long index = 0; |
||||
|
||||
// optimize later ;)
|
||||
BZERO( scratchspace, peer_count ); |
||||
|
||||
while( ( selected_count < amount ) && ( selected_count + invalid_count < peer_count ) ) { |
||||
// skip to first non-flagged peer
|
||||
while( TESTSET(index) ) index = ( index + 1 ) % peer_count; |
||||
|
||||
if( TESTVALIDPEER( peer_base + index ) ) { |
||||
SETINVALID(index); invalid_count++; |
||||
} else { |
||||
SETSELECTED(index); selected_count++; |
||||
index = ( index + RANDOM ) % peer_count; |
||||
} |
||||
} |
||||
|
||||
// Now our scratchspace contains a list of selected_count valid peers
|
||||
// Collect them into a reply string
|
||||
index = 0; |
||||
|
||||
#ifndef OT_COMPACT_ONLY |
||||
reply += FORMAT_FIXED_STRING( reply, "d5:peersl" ); |
||||
#else |
||||
reply += FORMAT_FORMAT_STRING( reply, "d5:peers%i:",6*selected_count ); |
||||
#endif |
||||
|
||||
while( selected_count-- ) { |
||||
ot_peer *peer; |
||||
while( !TESTSELECTED( index ) ) ++index; |
||||
peer = peer_base + index; |
||||
#ifdef OT_COMPACT_ONLY |
||||
MEMMOVE( reply, &peer->ip, 4 ); |
||||
MEMMOVE( reply+4, &peer->port, 2 ); |
||||
reply += 6; |
||||
#else |
||||
reply += FORMAT_FORMAT_STRING( reply, "d2:ip%d:%s7:peer id20:%20c4:porti%ie", |
||||
peer->flags & PEER_IP_LENGTH_MASK, |
||||
peer->ip, |
||||
peer->id, |
||||
peer->port ); |
||||
#endif |
||||
} |
||||
#ifndef OT_COMPACT_ONLY |
||||
reply += FORMAT_FIXED_STRING( reply, "ee" ); |
||||
#else |
||||
reply += FORMAT_FIXED_STRING( reply, "e" ); |
||||
#endif |
||||
} |
||||
|
||||
// Compacts a torrents peer list
|
||||
// * torrents older than OT_TIMEOUT are being kicked
|
||||
// * is rather expansive
|
||||
// * if this fails, torrent file is invalid, should add flag
|
||||
//
|
||||
void heal_torrent( ot_torrent *torrent ) { |
||||
unsigned long index = 0, base = 0, end, seed_count = 0; |
||||
|
||||
// Initialize base to first dead peer.
|
||||
while( ( base < torrent->peer_count ) && torrent->peer_list[base].death <= now() ) { |
||||
seed_count += ( torrent->peer_list[base].flags & PEER_FLAG_SEEDING ) ? 1 : 0; |
||||
base++; |
||||
} |
||||
|
||||
// No dead peers? Home.
|
||||
if( base == torrent->peer_count ) return; |
||||
|
||||
// From now index always looks to the next living peer while base keeps track of
|
||||
// the dead peer that marks the beginning of insert space.
|
||||
index = base + 1; |
||||
|
||||
while( 1 ) { |
||||
// Let index search for next living peer
|
||||
while( ( index < torrent->peer_count ) && torrent->peer_list[index].death > now() ) index++; |
||||
|
||||
// No further living peers found - base is our new peer count
|
||||
if( index == torrent->peer_count ) { |
||||
torrent->peer_count = base; |
||||
torrent->seed_count = seed_count; |
||||
return; |
||||
} |
||||
|
||||
end = index + 1; |
||||
|
||||
// Let end search for next dead peer (end of living peers)
|
||||
while( ( end < torrent->peer_count ) && torrent->peer_list[end].death <= now() ) { |
||||
seed_count += ( torrent->peer_list[end].flags & PEER_FLAG_SEEDING ) ? 1 : 0; |
||||
end++; |
||||
} |
||||
|
||||
// We either hit a dead peer or the end of our peers
|
||||
// In both cases: move block towards base
|
||||
MEMMOVE( torrent->peer_list + base, torrent->peer_list + index, ( end - index ) * sizeof( ot_peer ) ); |
||||
base += end - index; |
||||
|
||||
index = end; |
||||
} |
||||
} |
||||
|
||||
void *binary_search( const void *key, const void *base, |
||||
unsigned long member_count, const unsigned long member_size, |
||||
int (*compar) (const void *, const void *), |
||||
int *exactmatch ) { |
||||
unsigned char *lookat = ((unsigned char*)base) + member_size * (member_count >> 1); |
||||
*exactmatch = 1; |
||||
|
||||
while( member_count ) { |
||||
int cmp = compar((void*)lookat, key); |
||||
if (cmp == 0) return (void *)lookat; |
||||
if (cmp < 0) { |
||||
base = (void*)(lookat + member_size); |
||||
--member_count; |
||||
} |
||||
member_count >>= 1; |
||||
lookat = ((unsigned char*)base) + member_size * (member_count >> 1); |
||||
} |
||||
*exactmatch = 0; |
||||
return (void*)lookat; |
||||
|
||||
} |
||||
|
||||
// This function maps a "huge" file into process space
|
||||
// * I guess, we should be checking for more errors...
|
||||
void *map_file( char *file_name ) { |
||||
char *map; |
||||
int file_desc=open(file_name,O_RDWR|O_CREAT|O_NDELAY,0644); |
||||
|
||||
if( file_desc < 0) return 0; |
||||
|
||||
map=mmap(0,OT_HUGE_FILESIZE,PROT_READ|PROT_WRITE,MAP_SHARED,file_desc,0); |
||||
close(file_desc); |
||||
|
||||
return (map == (char*)-1) ? 0 : map; |
||||
} |
||||
|
||||
int init_logic( ) { |
||||
unlink( "./opentracker_map_index.idx" ); |
||||
torrents_pointer = map_file( "./opentracker_map_index.idx" ); |
||||
torrents_count = 0; |
||||
scratchspace = map_file( "./scratchspace" ); |
||||
} |
||||
|
||||
void deinit_logic( ) { |
||||
unmap_file( torrents_pointer ); |
||||
} |
Loading…
Reference in new issue