mirror of https://github.com/YGGverse/net-php.git
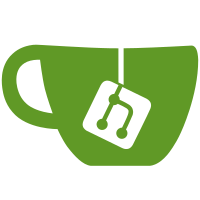
2 changed files with 189 additions and 0 deletions
@ -0,0 +1,168 @@
@@ -0,0 +1,168 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Net; |
||||
|
||||
class Address |
||||
{ |
||||
private ?string $_scheme = null; |
||||
private ?string $_host = null; |
||||
private ?string $_user = null; |
||||
private ?string $_pass = null; |
||||
private ?int $_port = null; |
||||
private ?string $_path = null; |
||||
private ?string $_query = null; |
||||
|
||||
private array $_dirs = []; |
||||
|
||||
public function __construct(?string $address = null) |
||||
{ |
||||
if ($address) |
||||
{ |
||||
if ($scheme = parse_url($address, PHP_URL_SCHEME)) |
||||
{ |
||||
$this->setScheme( |
||||
(string) $scheme |
||||
); |
||||
} |
||||
|
||||
if ($user = parse_url($address, PHP_URL_USER)) |
||||
{ |
||||
$this->setUser( |
||||
(string) $user |
||||
); |
||||
} |
||||
|
||||
if ($pass = parse_url($address, PHP_URL_PASS)) |
||||
{ |
||||
$this->setPath( |
||||
(string) $pass |
||||
); |
||||
} |
||||
|
||||
if ($host = parse_url($address, PHP_URL_HOST)) |
||||
{ |
||||
$this->setHost( |
||||
(string) $host |
||||
); |
||||
} |
||||
|
||||
if ($port = parse_url($address, PHP_URL_PORT)) |
||||
{ |
||||
$this->setPort( |
||||
(int) $port |
||||
); |
||||
} |
||||
|
||||
if ($path = parse_url($address, PHP_URL_PATH)) |
||||
{ |
||||
$this->setPath( |
||||
(string) $path |
||||
); |
||||
} |
||||
|
||||
if ($query = parse_url($address, PHP_URL_QUERY)) |
||||
{ |
||||
$this->setQuery( |
||||
(string) $query |
||||
); |
||||
} |
||||
} |
||||
} |
||||
|
||||
public function isAbsolute(): bool |
||||
{ |
||||
return ($this->_scheme && $this->_host); |
||||
} |
||||
|
||||
public function isRelative(): bool |
||||
{ |
||||
return !$this->isAbsolute(); |
||||
} |
||||
|
||||
public function getScheme(): string |
||||
{ |
||||
return $this->_scheme; |
||||
} |
||||
|
||||
public function setScheme(string $value): void |
||||
{ |
||||
$this->_scheme = $value; |
||||
} |
||||
|
||||
public function getHost(): string |
||||
{ |
||||
return $this->_host; |
||||
} |
||||
|
||||
public function setHost(string $value): void |
||||
{ |
||||
$this->_host = $value; |
||||
} |
||||
|
||||
public function getUser(): string |
||||
{ |
||||
return $this->_user; |
||||
} |
||||
|
||||
public function setUser(string $value): void |
||||
{ |
||||
$this->_user = $value; |
||||
} |
||||
|
||||
public function getPass(): string |
||||
{ |
||||
return $this->_pass; |
||||
} |
||||
|
||||
public function setPass(string $value): void |
||||
{ |
||||
$this->_pass = $value; |
||||
} |
||||
|
||||
public function getPort(): int |
||||
{ |
||||
return $this->_port; |
||||
} |
||||
|
||||
public function setPort(int $value): void |
||||
{ |
||||
$this->_port = $value; |
||||
} |
||||
|
||||
public function getPath(): string |
||||
{ |
||||
return $this->_path; |
||||
} |
||||
|
||||
public function setPath(string $value): void |
||||
{ |
||||
$this->_path = $value; |
||||
|
||||
$this->_dirs = explode( |
||||
'/', |
||||
$value |
||||
); |
||||
} |
||||
|
||||
public function getDirs(): array |
||||
{ |
||||
return $this->_dirs; |
||||
} |
||||
|
||||
public function getQuery(): string |
||||
{ |
||||
return $this->_query; |
||||
} |
||||
|
||||
public function setQuery(string $value): void |
||||
{ |
||||
$this->_query = $value; |
||||
} |
||||
|
||||
public function absolute(string $address): string |
||||
{ |
||||
// @TODO |
||||
} |
||||
} |
Loading…
Reference in new issue