mirror of https://github.com/YGGverse/Yoda.git
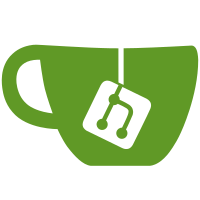
6 changed files with 219 additions and 191 deletions
@ -0,0 +1,77 @@
@@ -0,0 +1,77 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Yoda\Abstract\Entity\Browser\Container\Page\Content; |
||||
|
||||
use \Yggverse\Yoda\Entity\Browser\Container\Page\Content; |
||||
|
||||
abstract class Markup |
||||
{ |
||||
public \GtkLabel $gtk; |
||||
|
||||
// Dependencies |
||||
public Content $content; |
||||
|
||||
// Defaults |
||||
protected int $_wrap = 140; |
||||
|
||||
public function __construct( |
||||
Content $content |
||||
) { |
||||
// Init dependency |
||||
$this->content = $content; |
||||
|
||||
// Init markup label |
||||
$this->gtk = new \GtkLabel; |
||||
|
||||
$this->gtk->set_use_markup( |
||||
true |
||||
); |
||||
|
||||
$this->gtk->set_selectable( |
||||
true |
||||
); |
||||
|
||||
$this->gtk->set_can_focus( |
||||
false |
||||
); |
||||
|
||||
$this->gtk->set_track_visited_links( |
||||
true |
||||
); |
||||
|
||||
$this->gtk->set_xalign( |
||||
0 |
||||
); |
||||
|
||||
$this->gtk->set_yalign( |
||||
0 |
||||
); |
||||
|
||||
$this->gtk->show(); |
||||
|
||||
// Init events |
||||
$this->gtk->connect( |
||||
'activate-link', |
||||
function( |
||||
\GtkLabel $label, |
||||
string $href |
||||
) { |
||||
$this->_onActivateLink( |
||||
$label, |
||||
$href |
||||
); |
||||
} |
||||
); |
||||
} |
||||
|
||||
abstract protected function _onActivateLink( |
||||
\GtkLabel $label, |
||||
string $href |
||||
); |
||||
|
||||
abstract public function setSource( |
||||
string $value |
||||
): void; |
||||
} |
@ -0,0 +1,24 @@
@@ -0,0 +1,24 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace Yggverse\Yoda\Entity\Browser\Container\Page\Content; |
||||
|
||||
class Plain extends \Yggverse\Yoda\Abstract\Entity\Browser\Container\Page\Content\Markup |
||||
{ |
||||
public function setSource( |
||||
string $value |
||||
): void |
||||
{ |
||||
$this->_source = $value; |
||||
|
||||
$this->gtk->set_markup( |
||||
sprintf( |
||||
'<tt>%s</tt>', |
||||
htmlspecialchars( |
||||
$value |
||||
) |
||||
) |
||||
); |
||||
} |
||||
} |
Loading…
Reference in new issue