mirror of https://github.com/YGGverse/Yoda.git
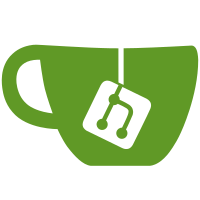
10 changed files with 267 additions and 206 deletions
@ -1,161 +1,22 @@
@@ -1,161 +1,22 @@
|
||||
#include "gemini.hpp" |
||||
#include "gemini/markup.hpp" |
||||
|
||||
using namespace app::browser::main::tab::page::content::text; |
||||
|
||||
Gemini::Gemini( |
||||
const Glib::ustring & GEMTEXT |
||||
) : Gtk::Viewport( // add scrolled window features support
|
||||
) : Gtk::Viewport( // add scrolled window features to childs
|
||||
NULL, |
||||
NULL |
||||
) { |
||||
// Init widget
|
||||
set_scroll_to_focus( |
||||
false |
||||
); |
||||
|
||||
auto label = Gtk::make_managed<Gtk::Label>( // @TODO separated file?
|
||||
Markup::make( |
||||
GEMTEXT |
||||
) |
||||
); |
||||
|
||||
// Init widget
|
||||
label->set_valign( |
||||
Gtk::Align::START |
||||
); |
||||
|
||||
label->set_wrap( |
||||
true |
||||
); |
||||
|
||||
label->set_selectable( |
||||
true |
||||
); |
||||
|
||||
label->set_use_markup( |
||||
true |
||||
); |
||||
|
||||
// Connect signals
|
||||
label->signal_activate_link().connect( |
||||
[label](const Glib::ustring & URI) -> bool |
||||
{ |
||||
// @TODO follow action
|
||||
|
||||
return false; |
||||
}, |
||||
false // after
|
||||
); |
||||
|
||||
set_child( |
||||
* label |
||||
); |
||||
} |
||||
|
||||
// Match tools
|
||||
bool Gemini::Line::Match::link( |
||||
const Glib::ustring & GEMTEXT, |
||||
Glib::ustring & address, |
||||
Glib::ustring & date, |
||||
Glib::ustring & alt |
||||
) { |
||||
auto match = Glib::Regex::split_simple( |
||||
R"regex(^=>\s*([^\s]+)(\s(\d{4}-\d{2}-\d{2}))?(\s(.+))?$)regex", |
||||
GEMTEXT |
||||
); |
||||
|
||||
int index = 0; for (const Glib::ustring & MATCH : match) |
||||
{ |
||||
switch (index) |
||||
{ |
||||
case 1: address = MATCH; break; |
||||
case 3: date = MATCH; break; |
||||
case 5: alt = MATCH; break; |
||||
} |
||||
|
||||
index++; |
||||
} |
||||
|
||||
return !address.empty(); |
||||
} |
||||
|
||||
// Markup tools
|
||||
Glib::ustring Gemini::Markup::make( |
||||
const Glib::ustring & GEMTEXT |
||||
) { |
||||
Glib::ustring pango; |
||||
|
||||
std::istringstream stream( |
||||
GEMTEXT |
||||
); |
||||
|
||||
std::string line; |
||||
|
||||
while (std::getline(stream, line)) |
||||
{ |
||||
// Links
|
||||
Glib::ustring address; |
||||
Glib::ustring date; |
||||
Glib::ustring alt; |
||||
|
||||
if (Line::Match::link(line, address, date, alt)) |
||||
{ |
||||
pango.append( |
||||
Markup::Make::link( |
||||
address, |
||||
date, |
||||
alt |
||||
) |
||||
); |
||||
} |
||||
|
||||
else |
||||
{ |
||||
pango.append( |
||||
line |
||||
); |
||||
} |
||||
|
||||
// @TODO other tags..
|
||||
|
||||
pango.append( |
||||
"\n" // @TODO
|
||||
); |
||||
} |
||||
|
||||
return pango; |
||||
} |
||||
|
||||
Glib::ustring Gemini::Markup::Make::link( |
||||
const Glib::ustring & ADDRESS, |
||||
const Glib::ustring & DATE, |
||||
const Glib::ustring & ALT |
||||
) { |
||||
Glib::ustring description; |
||||
|
||||
if (!DATE.empty()) |
||||
{ |
||||
description.append( |
||||
DATE |
||||
); |
||||
} |
||||
|
||||
if (!ALT.empty()) |
||||
{ |
||||
description.append( |
||||
description.empty() ? ALT : description + " " + ALT // append (to date)
|
||||
); |
||||
} |
||||
|
||||
return Glib::ustring::sprintf( |
||||
"<a href=\"%s\" title=\"%s\">%s</a>", |
||||
Glib::Markup::escape_text( |
||||
ADDRESS // @TODO to absolute
|
||||
), |
||||
Glib::Markup::escape_text( |
||||
ADDRESS |
||||
), |
||||
Glib::Markup::escape_text( |
||||
description |
||||
* Gtk::make_managed<gemini::Markup>( |
||||
GEMTEXT |
||||
) |
||||
); |
||||
} |
@ -0,0 +1,150 @@
@@ -0,0 +1,150 @@
|
||||
#include "markup.hpp" |
||||
|
||||
using namespace app::browser::main::tab::page::content::text::gemini; |
||||
|
||||
Markup::Markup( |
||||
const Glib::ustring & GEMTEXT |
||||
) { |
||||
// Init widget
|
||||
set_valign( |
||||
Gtk::Align::START |
||||
); |
||||
|
||||
set_wrap( |
||||
true |
||||
); |
||||
|
||||
set_selectable( |
||||
true |
||||
); |
||||
|
||||
set_use_markup( |
||||
true |
||||
); |
||||
|
||||
set_markup( |
||||
Markup::make( |
||||
GEMTEXT |
||||
) |
||||
); |
||||
|
||||
// Connect signals
|
||||
signal_activate_link().connect( |
||||
[this](const Glib::ustring & URI) -> bool |
||||
{ |
||||
// @TODO follow action
|
||||
|
||||
return false; |
||||
}, |
||||
false // after
|
||||
); |
||||
} |
||||
|
||||
// Match tools
|
||||
bool Markup::Line::Match::link( |
||||
const Glib::ustring & GEMTEXT, |
||||
Glib::ustring & address, |
||||
Glib::ustring & date, |
||||
Glib::ustring & alt |
||||
) { |
||||
auto match = Glib::Regex::split_simple( |
||||
R"regex(^=>\s*([^\s]+)(\s(\d{4}-\d{2}-\d{2}))?(\s(.+))?$)regex", |
||||
GEMTEXT |
||||
); |
||||
|
||||
int index = 0; for (const Glib::ustring & MATCH : match) |
||||
{ |
||||
switch (index) |
||||
{ |
||||
case 1: address = MATCH; break; |
||||
case 3: date = MATCH; break; |
||||
case 5: alt = MATCH; break; |
||||
} |
||||
|
||||
index++; |
||||
} |
||||
|
||||
return !address.empty(); |
||||
} |
||||
|
||||
// Markup tools
|
||||
Glib::ustring Markup::make( |
||||
const Glib::ustring & GEMTEXT |
||||
) { |
||||
Glib::ustring pango; |
||||
|
||||
std::istringstream stream( |
||||
GEMTEXT |
||||
); |
||||
|
||||
std::string line; |
||||
|
||||
while (std::getline(stream, line)) |
||||
{ |
||||
// Links
|
||||
Glib::ustring address; |
||||
Glib::ustring date; |
||||
Glib::ustring alt; |
||||
|
||||
if (Line::Match::link(line, address, date, alt)) |
||||
{ |
||||
pango.append( |
||||
Markup::Make::link( |
||||
address, |
||||
date, |
||||
alt |
||||
) |
||||
); |
||||
} |
||||
|
||||
else |
||||
{ |
||||
pango.append( |
||||
line |
||||
); |
||||
} |
||||
|
||||
// @TODO other tags..
|
||||
|
||||
pango.append( |
||||
"\n" // @TODO
|
||||
); |
||||
} |
||||
|
||||
return pango; |
||||
} |
||||
|
||||
Glib::ustring Markup::Make::link( |
||||
const Glib::ustring & ADDRESS, |
||||
const Glib::ustring & DATE, |
||||
const Glib::ustring & ALT |
||||
) { |
||||
Glib::ustring description; |
||||
|
||||
if (!DATE.empty()) |
||||
{ |
||||
description.append( |
||||
DATE |
||||
); |
||||
} |
||||
|
||||
if (!ALT.empty()) |
||||
{ |
||||
description.append( |
||||
description.empty() ? ALT : description + " " + ALT // append (to date)
|
||||
); |
||||
} |
||||
|
||||
return Glib::ustring::sprintf( |
||||
"<a href=\"%s\" title=\"%s\">%s</a>", |
||||
Glib::Markup::escape_text( |
||||
ADDRESS // @TODO to absolute
|
||||
), |
||||
Glib::Markup::escape_text( |
||||
ADDRESS |
||||
), |
||||
Glib::Markup::escape_text( |
||||
description |
||||
) |
||||
); |
||||
} |
@ -0,0 +1,53 @@
@@ -0,0 +1,53 @@
|
||||
#ifndef APP_BROWSER_MAIN_TAB_PAGE_CONTENT_TEXT_GEMINI_MARKUP_HPP |
||||
#define APP_BROWSER_MAIN_TAB_PAGE_CONTENT_TEXT_GEMINI_MARKUP_HPP |
||||
|
||||
#include <glibmm/markup.h> |
||||
#include <glibmm/regex.h> |
||||
#include <glibmm/ustring.h> |
||||
#include <gtkmm/label.h> |
||||
|
||||
namespace app::browser::main::tab::page::content::text::gemini |
||||
{ |
||||
class Markup : public Gtk::Label |
||||
{ |
||||
/*
|
||||
* Tools (currently is private) |
||||
*/ |
||||
struct Line |
||||
{ |
||||
struct Match |
||||
{ |
||||
static bool link( |
||||
const Glib::ustring & GEMTEXT, |
||||
Glib::ustring & address, |
||||
Glib::ustring & date, |
||||
Glib::ustring & alt |
||||
); |
||||
}; |
||||
}; |
||||
|
||||
struct Make |
||||
{ |
||||
static Glib::ustring link( |
||||
const Glib::ustring & ADDRESS, |
||||
const Glib::ustring & DATE, |
||||
const Glib::ustring & ALT |
||||
); |
||||
}; |
||||
|
||||
static Glib::ustring make( |
||||
const Glib::ustring & GEMTEXT |
||||
); |
||||
|
||||
/*
|
||||
* Gemini class API |
||||
*/ |
||||
public: |
||||
|
||||
Markup( |
||||
const Glib::ustring & GEMTEXT |
||||
); |
||||
}; |
||||
} |
||||
|
||||
#endif // APP_BROWSER_MAIN_TAB_PAGE_CONTENT_TEXT_GEMINI_MARKUP_HPP
|
@ -0,0 +1,28 @@
@@ -0,0 +1,28 @@
|
||||
#include "markup.hpp" |
||||
|
||||
using namespace app::browser::main::tab::page::content::text::plain; |
||||
|
||||
Markup::Markup( |
||||
const Glib::ustring & TEXT |
||||
) { |
||||
// Init widget
|
||||
set_valign( |
||||
Gtk::Align::START |
||||
); |
||||
|
||||
set_wrap( |
||||
true |
||||
); |
||||
|
||||
set_selectable( |
||||
true |
||||
); |
||||
|
||||
set_use_markup( |
||||
false // @TODO
|
||||
); |
||||
|
||||
set_text( |
||||
TEXT |
||||
); |
||||
} |
@ -0,0 +1,23 @@
@@ -0,0 +1,23 @@
|
||||
#ifndef APP_BROWSER_MAIN_TAB_PAGE_CONTENT_TEXT_PLAIN_MARKUP_HPP |
||||
#define APP_BROWSER_MAIN_TAB_PAGE_CONTENT_TEXT_PLAIN_MARKUP_HPP |
||||
|
||||
#include <glibmm/markup.h> |
||||
#include <glibmm/ustring.h> |
||||
#include <gtkmm/label.h> |
||||
|
||||
namespace app::browser::main::tab::page::content::text::plain |
||||
{ |
||||
class Markup : public Gtk::Label |
||||
{ |
||||
/*
|
||||
* Gemini class API |
||||
*/ |
||||
public: |
||||
|
||||
Markup( |
||||
const Glib::ustring & TEXT |
||||
); |
||||
}; |
||||
} |
||||
|
||||
#endif // APP_BROWSER_MAIN_TAB_PAGE_CONTENT_TEXT_PLAIN_MARKUP_HPP
|
Loading…
Reference in new issue