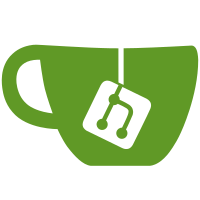
5 changed files with 277 additions and 36 deletions
@ -0,0 +1,80 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Entity; |
||||||
|
|
||||||
|
use App\Repository\TorrentBookmarkRepository; |
||||||
|
use Doctrine\ORM\Mapping as ORM; |
||||||
|
|
||||||
|
#[ORM\Entity(repositoryClass: TorrentBookmarkRepository::class)] |
||||||
|
class TorrentBookmark |
||||||
|
{ |
||||||
|
#[ORM\Id] |
||||||
|
#[ORM\GeneratedValue] |
||||||
|
#[ORM\Column] |
||||||
|
private ?int $id = null; |
||||||
|
|
||||||
|
#[ORM\Column] |
||||||
|
private ?int $torrentId = null; |
||||||
|
|
||||||
|
#[ORM\Column] |
||||||
|
private ?int $userId = null; |
||||||
|
|
||||||
|
#[ORM\Column] |
||||||
|
private ?int $added = null; |
||||||
|
|
||||||
|
#[ORM\Column] |
||||||
|
private ?bool $value = null; |
||||||
|
|
||||||
|
public function getId(): ?int |
||||||
|
{ |
||||||
|
return $this->id; |
||||||
|
} |
||||||
|
|
||||||
|
public function getTorrentId(): ?int |
||||||
|
{ |
||||||
|
return $this->torrentId; |
||||||
|
} |
||||||
|
|
||||||
|
public function setTorrentId(int $torrentId): static |
||||||
|
{ |
||||||
|
$this->torrentId = $torrentId; |
||||||
|
|
||||||
|
return $this; |
||||||
|
} |
||||||
|
|
||||||
|
public function getUserId(): ?int |
||||||
|
{ |
||||||
|
return $this->userId; |
||||||
|
} |
||||||
|
|
||||||
|
public function setUserId(int $userId): static |
||||||
|
{ |
||||||
|
$this->userId = $userId; |
||||||
|
|
||||||
|
return $this; |
||||||
|
} |
||||||
|
|
||||||
|
public function getAdded(): ?int |
||||||
|
{ |
||||||
|
return $this->added; |
||||||
|
} |
||||||
|
|
||||||
|
public function setAdded(int $added): static |
||||||
|
{ |
||||||
|
$this->added = $added; |
||||||
|
|
||||||
|
return $this; |
||||||
|
} |
||||||
|
|
||||||
|
public function isValue(): ?bool |
||||||
|
{ |
||||||
|
return $this->value; |
||||||
|
} |
||||||
|
|
||||||
|
public function setValue(bool $value): static |
||||||
|
{ |
||||||
|
$this->value = $value; |
||||||
|
|
||||||
|
return $this; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,40 @@ |
|||||||
|
<?php |
||||||
|
|
||||||
|
namespace App\Repository; |
||||||
|
|
||||||
|
use App\Entity\TorrentBookmark; |
||||||
|
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||||
|
use Doctrine\Persistence\ManagerRegistry; |
||||||
|
|
||||||
|
/** |
||||||
|
* @extends ServiceEntityRepository<TorrentBookmark> |
||||||
|
* |
||||||
|
* @method TorrentBookmark|null find($id, $lockMode = null, $lockVersion = null) |
||||||
|
* @method TorrentBookmark|null findOneBy(array $criteria, array $orderBy = null) |
||||||
|
* @method TorrentBookmark[] findAll() |
||||||
|
* @method TorrentBookmark[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||||
|
*/ |
||||||
|
class TorrentBookmarkRepository extends ServiceEntityRepository |
||||||
|
{ |
||||||
|
public function __construct(ManagerRegistry $registry) |
||||||
|
{ |
||||||
|
parent::__construct($registry, TorrentBookmark::class); |
||||||
|
} |
||||||
|
|
||||||
|
public function findUserLastTorrentBookmark( |
||||||
|
int $torrentId, |
||||||
|
int $userId |
||||||
|
): ?TorrentBookmark |
||||||
|
{ |
||||||
|
return $this->createQueryBuilder('tb') |
||||||
|
->where('tb.torrentId = :torrentId') |
||||||
|
->andWhere('tb.userId = :userId') |
||||||
|
->setParameter('torrentId', $torrentId) |
||||||
|
->setParameter('userId', $userId) |
||||||
|
->orderBy('tb.id', 'DESC') // same to ts.added |
||||||
|
->setMaxResults(1) |
||||||
|
->getQuery() |
||||||
|
->getOneOrNullResult() |
||||||
|
; |
||||||
|
} |
||||||
|
} |
Loading…
Reference in new issue