mirror of https://github.com/YGGverse/HLState.git
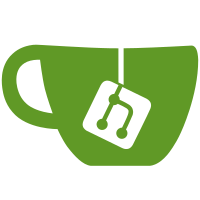
5 changed files with 290 additions and 11 deletions
@ -0,0 +1,31 @@
@@ -0,0 +1,31 @@
|
||||
<?php |
||||
|
||||
declare(strict_types=1); |
||||
|
||||
namespace DoctrineMigrations; |
||||
|
||||
use Doctrine\DBAL\Schema\Schema; |
||||
use Doctrine\Migrations\AbstractMigration; |
||||
|
||||
/** |
||||
* Auto-generated Migration: Please modify to your needs! |
||||
*/ |
||||
final class Version20240107151414 extends AbstractMigration |
||||
{ |
||||
public function getDescription(): string |
||||
{ |
||||
return ''; |
||||
} |
||||
|
||||
public function up(Schema $schema): void |
||||
{ |
||||
// this up() migration is auto-generated, please modify it to your needs |
||||
$this->addSql('CREATE TABLE player (id INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL, crc32server BIGINT NOT NULL, crc32name BIGINT NOT NULL, joined BIGINT NOT NULL, updated BIGINT NOT NULL, online BIGINT NOT NULL, frags BIGINT NOT NULL, name VARCHAR(255) NOT NULL)'); |
||||
} |
||||
|
||||
public function down(Schema $schema): void |
||||
{ |
||||
// this down() migration is auto-generated, please modify it to your needs |
||||
$this->addSql('DROP TABLE player'); |
||||
} |
||||
} |
@ -0,0 +1,126 @@
@@ -0,0 +1,126 @@
|
||||
<?php |
||||
|
||||
namespace App\Entity; |
||||
|
||||
use App\Repository\PlayerRepository; |
||||
use Doctrine\DBAL\Types\Types; |
||||
use Doctrine\ORM\Mapping as ORM; |
||||
|
||||
#[ORM\Entity(repositoryClass: PlayerRepository::class)] |
||||
class Player |
||||
{ |
||||
#[ORM\Id] |
||||
#[ORM\GeneratedValue] |
||||
#[ORM\Column] |
||||
private ?int $id = null; |
||||
|
||||
#[ORM\Column(type: Types::BIGINT)] |
||||
private ?string $crc32server = null; |
||||
|
||||
#[ORM\Column(type: Types::BIGINT)] |
||||
private ?string $crc32name = null; |
||||
|
||||
#[ORM\Column(type: Types::BIGINT)] |
||||
private ?string $joined = null; |
||||
|
||||
#[ORM\Column(type: Types::BIGINT)] |
||||
private ?string $updated = null; |
||||
|
||||
#[ORM\Column(type: Types::BIGINT)] |
||||
private ?string $online = null; |
||||
|
||||
#[ORM\Column(type: Types::BIGINT)] |
||||
private ?string $frags = null; |
||||
|
||||
#[ORM\Column(length: 255)] |
||||
private ?string $name = null; |
||||
|
||||
public function getId(): ?int |
||||
{ |
||||
return $this->id; |
||||
} |
||||
|
||||
public function getCrc32server(): ?string |
||||
{ |
||||
return $this->crc32server; |
||||
} |
||||
|
||||
public function setCrc32server(string $crc32server): static |
||||
{ |
||||
$this->crc32server = $crc32server; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getCrc32name(): ?string |
||||
{ |
||||
return $this->crc32name; |
||||
} |
||||
|
||||
public function setCrc32name(string $crc32name): static |
||||
{ |
||||
$this->crc32name = $crc32name; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getJoined(): ?string |
||||
{ |
||||
return $this->joined; |
||||
} |
||||
|
||||
public function setJoined(string $joined): static |
||||
{ |
||||
$this->joined = $joined; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getUpdated(): ?string |
||||
{ |
||||
return $this->updated; |
||||
} |
||||
|
||||
public function setUpdated(string $updated): static |
||||
{ |
||||
$this->updated = $updated; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getOnline(): ?string |
||||
{ |
||||
return $this->online; |
||||
} |
||||
|
||||
public function setOnline(string $online): static |
||||
{ |
||||
$this->online = $online; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getFrags(): ?string |
||||
{ |
||||
return $this->frags; |
||||
} |
||||
|
||||
public function setFrags(string $frags): static |
||||
{ |
||||
$this->frags = $frags; |
||||
|
||||
return $this; |
||||
} |
||||
|
||||
public function getName(): ?string |
||||
{ |
||||
return $this->name; |
||||
} |
||||
|
||||
public function setName(string $name): static |
||||
{ |
||||
$this->name = $name; |
||||
|
||||
return $this; |
||||
} |
||||
} |
@ -0,0 +1,23 @@
@@ -0,0 +1,23 @@
|
||||
<?php |
||||
|
||||
namespace App\Repository; |
||||
|
||||
use App\Entity\Player; |
||||
use Doctrine\Bundle\DoctrineBundle\Repository\ServiceEntityRepository; |
||||
use Doctrine\Persistence\ManagerRegistry; |
||||
|
||||
/** |
||||
* @extends ServiceEntityRepository<Player> |
||||
* |
||||
* @method Player|null find($id, $lockMode = null, $lockVersion = null) |
||||
* @method Player|null findOneBy(array $criteria, array $orderBy = null) |
||||
* @method Player[] findAll() |
||||
* @method Player[] findBy(array $criteria, array $orderBy = null, $limit = null, $offset = null) |
||||
*/ |
||||
class PlayerRepository extends ServiceEntityRepository |
||||
{ |
||||
public function __construct(ManagerRegistry $registry) |
||||
{ |
||||
parent::__construct($registry, Player::class); |
||||
} |
||||
} |
Loading…
Reference in new issue