mirror of https://github.com/PurpleI2P/i2pd.git
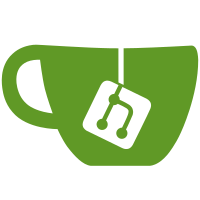
3 changed files with 110 additions and 1 deletions
@ -0,0 +1,74 @@
@@ -0,0 +1,74 @@
|
||||
#include <endian.h> |
||||
#include <string> |
||||
#include <cryptopp/gzip.h> |
||||
#include "Log.h" |
||||
#include "RouterInfo.h" |
||||
#include "Streaming.h" |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace stream |
||||
{ |
||||
void StreamingDestination::HandleNextPacket (const uint8_t * buf, size_t len) |
||||
{ |
||||
const uint8_t * end = buf + len; |
||||
buf += 4; // sendStreamID
|
||||
buf += 4; // receiveStreamID
|
||||
buf += 4; // sequenceNum
|
||||
buf += 4; // ackThrough
|
||||
int nackCount = buf[0]; |
||||
buf++; // NACK count
|
||||
buf += 4*nackCount; // NACKs
|
||||
buf++; // resendDelay
|
||||
uint16_t flags = be16toh (*(uint16_t *)buf); |
||||
buf += 2; // flags
|
||||
uint16_t optionalSize = be16toh (*(uint16_t *)buf); |
||||
buf += 2; // optional size
|
||||
const uint8_t * optionalData = buf; |
||||
buf += optionalSize; |
||||
|
||||
// process flags
|
||||
if (flags & PACKET_FLAG_SYNCHRONIZE) |
||||
{ |
||||
LogPrint ("Synchronize"); |
||||
} |
||||
|
||||
if (flags & PACKET_FLAG_SIGNATURE_INCLUDED) |
||||
{ |
||||
LogPrint ("Signature"); |
||||
optionalData += 40; |
||||
} |
||||
|
||||
if (flags & PACKET_FLAG_FROM_INCLUDED) |
||||
{ |
||||
LogPrint ("From identity"); |
||||
optionalData += sizeof (i2p::data::RouterIdentity); |
||||
} |
||||
|
||||
// we have reached payload section
|
||||
std::string str((const char *)buf, end-buf); |
||||
LogPrint ("Payload: ", str); |
||||
} |
||||
|
||||
|
||||
void HandleDataMessage (i2p::data::IdentHash * destination, const uint8_t * buf, size_t len) |
||||
{ |
||||
uint32_t length = be32toh (*(uint32_t *)buf); |
||||
buf += 4; |
||||
// we assume I2CP payload
|
||||
if (buf[9] == 6) // streaming protocol
|
||||
{ |
||||
// unzip it
|
||||
CryptoPP::Gunzip decompressor; |
||||
decompressor.Put (buf, length); |
||||
decompressor.MessageEnd(); |
||||
uint8_t uncompressed[2048]; |
||||
int uncomressedSize = decompressor.MaxRetrievable (); |
||||
decompressor.Get (uncompressed, uncomressedSize); |
||||
// then forward to streaming engine
|
||||
} |
||||
else |
||||
LogPrint ("Data: protocol ", buf[9], " is not supported"); |
||||
} |
||||
} |
||||
} |
@ -0,0 +1,35 @@
@@ -0,0 +1,35 @@
|
||||
#ifndef STREAMING_H__ |
||||
#define STREAMING_H__ |
||||
|
||||
#include <inttypes.h> |
||||
#include "LeaseSet.h" |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace stream |
||||
{ |
||||
const uint16_t PACKET_FLAG_SYNCHRONIZE = 0x0001; |
||||
const uint16_t PACKET_FLAG_CLOSE = 0x0002; |
||||
const uint16_t PACKET_FLAG_RESET = 0x0004; |
||||
const uint16_t PACKET_FLAG_SIGNATURE_INCLUDED = 0x0008; |
||||
const uint16_t PACKET_FLAG_SIGNATURE_REQUESTED = 0x0010; |
||||
const uint16_t PACKET_FLAG_FROM_INCLUDED = 0x0020; |
||||
const uint16_t PACKET_FLAG_DELAY_REQUESTED = 0x0040; |
||||
const uint16_t PACKET_FLAG_MAX_PACKET_SIZE_INCLUDED = 0x0080; |
||||
const uint16_t PACKET_FLAG_PROFILE_INTERACTIVE = 0x0100; |
||||
const uint16_t PACKET_FLAG_ECHO = 0x0200; |
||||
const uint16_t PACKET_FLAG_NO_ACK = 0x0400; |
||||
|
||||
class StreamingDestination |
||||
{ |
||||
public: |
||||
|
||||
void HandleNextPacket (const uint8_t * buf, size_t len); |
||||
}; |
||||
|
||||
// assuming data is I2CP message
|
||||
void HandleDataMessage (i2p::data::IdentHash * destination, const uint8_t * buf, size_t len); |
||||
} |
||||
} |
||||
|
||||
#endif |
Loading…
Reference in new issue