mirror of https://github.com/PurpleI2P/i2pd.git
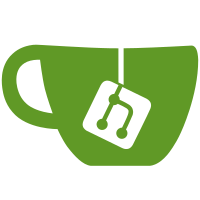
19 changed files with 336 additions and 62 deletions
@ -0,0 +1,73 @@
@@ -0,0 +1,73 @@
|
||||
#ifndef ADDRESS_BOOK_H__ |
||||
#define ADDRESS_BOOK_H__ |
||||
|
||||
#include <string.h> |
||||
#include <string> |
||||
#include <map> |
||||
#include "base64.h" |
||||
#include "util.h" |
||||
#include "Identity.h" |
||||
#include "Log.h" |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace data |
||||
{ |
||||
class AddressBook |
||||
{ |
||||
public: |
||||
|
||||
AddressBook (): m_IsLoaded (false) {}; |
||||
|
||||
const IdentHash * FindAddress (const std::string& address) |
||||
{ |
||||
if (!m_IsLoaded) |
||||
LoadHosts (); |
||||
auto it = m_Addresses.find (address); |
||||
if (it != m_Addresses.end ()) |
||||
return &it->second; |
||||
else |
||||
return nullptr; |
||||
} |
||||
|
||||
private: |
||||
|
||||
void LoadHosts () |
||||
{ |
||||
m_IsLoaded = true; |
||||
std::ifstream f (i2p::util::filesystem::GetFullPath ("hosts.txt").c_str (), std::ofstream::in); // in text mode
|
||||
if (!f.is_open ()) |
||||
{ |
||||
LogPrint ("hosts.txt not found"); |
||||
return; |
||||
} |
||||
int numAddresses = 0; |
||||
char str[1024]; |
||||
while (!f.eof ()) |
||||
{ |
||||
f.getline (str, 1024); |
||||
char * key = strchr (str, '='); |
||||
if (key) |
||||
{ |
||||
*key = 0; |
||||
key++; |
||||
Identity ident; |
||||
Base64ToByteStream (key, strlen(key), (uint8_t *)&ident, sizeof (ident)); |
||||
m_Addresses[str] = CalculateIdentHash (ident); |
||||
numAddresses++; |
||||
} |
||||
} |
||||
LogPrint (numAddresses, " addresses loaded"); |
||||
} |
||||
|
||||
private: |
||||
|
||||
std::map<std::string, IdentHash> m_Addresses; |
||||
bool m_IsLoaded; |
||||
}; |
||||
} |
||||
} |
||||
|
||||
#endif |
||||
|
||||
|
@ -0,0 +1,73 @@
@@ -0,0 +1,73 @@
|
||||
#include "Tunnel.h" |
||||
#include "NetDb.h" |
||||
#include "Timestamp.h" |
||||
#include "TunnelPool.h" |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace tunnel |
||||
{ |
||||
TunnelPool::TunnelPool (i2p::data::LocalDestination * owner, int numTunnels): |
||||
m_Owner (owner), m_NumTunnels (numTunnels) |
||||
{ |
||||
} |
||||
|
||||
TunnelPool::~TunnelPool () |
||||
{ |
||||
for (auto it: m_InboundTunnels) |
||||
it->SetTunnelPool (nullptr); |
||||
} |
||||
|
||||
void TunnelPool::TunnelCreated (InboundTunnel * createdTunnel) |
||||
{ |
||||
m_InboundTunnels.insert (createdTunnel); |
||||
if (m_Owner) |
||||
m_Owner->UpdateLeaseSet (); |
||||
} |
||||
|
||||
void TunnelPool::TunnelExpired (InboundTunnel * expiredTunnel) |
||||
{ |
||||
m_InboundTunnels.erase (expiredTunnel); |
||||
if (m_Owner) |
||||
m_Owner->UpdateLeaseSet (); |
||||
} |
||||
|
||||
std::vector<InboundTunnel *> TunnelPool::GetInboundTunnels (int num) const |
||||
{ |
||||
std::vector<InboundTunnel *> v; |
||||
int i = 0; |
||||
for (auto it : m_InboundTunnels) |
||||
{ |
||||
if (i >= num) break; |
||||
v.push_back (it); |
||||
i++; |
||||
} |
||||
return v; |
||||
} |
||||
|
||||
void TunnelPool::CreateTunnels () |
||||
{ |
||||
int num = m_InboundTunnels.size (); |
||||
for (int i = num; i < m_NumTunnels; i++) |
||||
CreateInboundTunnel (); |
||||
} |
||||
|
||||
void TunnelPool::CreateInboundTunnel () |
||||
{ |
||||
OutboundTunnel * outboundTunnel = tunnels.GetNextOutboundTunnel (); |
||||
LogPrint ("Creating destination inbound tunnel..."); |
||||
auto firstHop = i2p::data::netdb.GetRandomRouter (outboundTunnel ? outboundTunnel->GetEndpointRouter () : nullptr); |
||||
auto secondHop = i2p::data::netdb.GetRandomRouter (firstHop); |
||||
auto * tunnel = tunnels.CreateTunnel<InboundTunnel> ( |
||||
new TunnelConfig (std::vector<const i2p::data::RouterInfo *> |
||||
{ |
||||
firstHop, |
||||
secondHop |
||||
// TODO: swithc to 3-hops later
|
||||
/*i2p::data::netdb.GetRandomRouter (secondHop) */ |
||||
}), |
||||
outboundTunnel); |
||||
tunnel->SetTunnelPool (this); |
||||
} |
||||
} |
||||
} |
@ -0,0 +1,46 @@
@@ -0,0 +1,46 @@
|
||||
#ifndef TUNNEL_POOL__ |
||||
#define TUNNEL_POOL__ |
||||
|
||||
#include <set> |
||||
#include <vector> |
||||
#include "Identity.h" |
||||
#include "LeaseSet.h" |
||||
#include "I2NPProtocol.h" |
||||
#include "TunnelBase.h" |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace tunnel |
||||
{ |
||||
class Tunnel; |
||||
class InboundTunnel; |
||||
class OutboundTunnel; |
||||
|
||||
class TunnelPool // per local destination
|
||||
{ |
||||
public: |
||||
|
||||
TunnelPool (i2p::data::LocalDestination * owner, int numTunnels = 5); |
||||
~TunnelPool (); |
||||
|
||||
void CreateTunnels (); |
||||
void TunnelCreated (InboundTunnel * createdTunnel); |
||||
void TunnelExpired (InboundTunnel * expiredTunnel); |
||||
std::vector<InboundTunnel *> GetInboundTunnels (int num) const; |
||||
|
||||
private: |
||||
|
||||
void CreateInboundTunnel (); |
||||
|
||||
private: |
||||
|
||||
i2p::data::LocalDestination * m_Owner; |
||||
int m_NumTunnels; |
||||
std::set<InboundTunnel *, TunnelCreationTimeCmp> m_InboundTunnels; // recent tunnel appears first
|
||||
|
||||
}; |
||||
} |
||||
} |
||||
|
||||
#endif |
||||
|
Loading…
Reference in new issue