mirror of https://github.com/PurpleI2P/i2pd.git
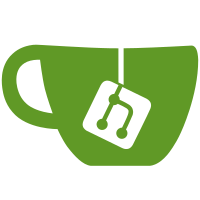
8 changed files with 256 additions and 182 deletions
@ -0,0 +1,149 @@
@@ -0,0 +1,149 @@
|
||||
#include "Log.h" |
||||
#include "I2NPProtocol.h" |
||||
#include "Transports.h" |
||||
#include "NetDb.h" |
||||
#include "NetDbRequests.h" |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace data |
||||
{ |
||||
I2NPMessage * RequestedDestination::CreateRequestMessage (std::shared_ptr<const RouterInfo> router, |
||||
std::shared_ptr<const i2p::tunnel::InboundTunnel> replyTunnel) |
||||
{ |
||||
I2NPMessage * msg = i2p::CreateRouterInfoDatabaseLookupMsg (m_Destination, |
||||
replyTunnel->GetNextIdentHash (), replyTunnel->GetNextTunnelID (), m_IsExploratory, |
||||
&m_ExcludedPeers); |
||||
m_ExcludedPeers.insert (router->GetIdentHash ()); |
||||
m_CreationTime = i2p::util::GetSecondsSinceEpoch (); |
||||
return msg; |
||||
} |
||||
|
||||
I2NPMessage * RequestedDestination::CreateRequestMessage (const IdentHash& floodfill) |
||||
{ |
||||
I2NPMessage * msg = i2p::CreateRouterInfoDatabaseLookupMsg (m_Destination, |
||||
i2p::context.GetRouterInfo ().GetIdentHash () , 0, false, &m_ExcludedPeers); |
||||
m_ExcludedPeers.insert (floodfill); |
||||
m_CreationTime = i2p::util::GetSecondsSinceEpoch (); |
||||
return msg; |
||||
} |
||||
|
||||
void RequestedDestination::ClearExcludedPeers () |
||||
{ |
||||
m_ExcludedPeers.clear (); |
||||
} |
||||
|
||||
void RequestedDestination::Success (std::shared_ptr<RouterInfo> r) |
||||
{ |
||||
if (m_RequestComplete) |
||||
{ |
||||
m_RequestComplete (r); |
||||
m_RequestComplete = nullptr; |
||||
} |
||||
} |
||||
|
||||
void RequestedDestination::Fail () |
||||
{ |
||||
if (m_RequestComplete) |
||||
{ |
||||
m_RequestComplete (nullptr); |
||||
m_RequestComplete = nullptr; |
||||
} |
||||
} |
||||
|
||||
void NetDbRequests::Start () |
||||
{ |
||||
} |
||||
|
||||
void NetDbRequests::Stop () |
||||
{ |
||||
m_RequestedDestinations.clear (); |
||||
} |
||||
|
||||
|
||||
std::shared_ptr<RequestedDestination> NetDbRequests::CreateRequest (const IdentHash& destination, bool isExploratory, RequestedDestination::RequestComplete requestComplete) |
||||
{ |
||||
// request RouterInfo directly
|
||||
auto dest = std::make_shared<RequestedDestination> (destination, isExploratory); |
||||
dest->SetRequestComplete (requestComplete); |
||||
{ |
||||
std::unique_lock<std::mutex> l(m_RequestedDestinationsMutex); |
||||
if (!m_RequestedDestinations.insert (std::make_pair (destination, |
||||
std::shared_ptr<RequestedDestination> (dest))).second) // not inserted
|
||||
return nullptr; |
||||
} |
||||
return dest; |
||||
} |
||||
|
||||
void NetDbRequests::RequestComplete (const IdentHash& ident, std::shared_ptr<RouterInfo> r) |
||||
{ |
||||
auto it = m_RequestedDestinations.find (ident); |
||||
if (it != m_RequestedDestinations.end ()) |
||||
{ |
||||
if (r) |
||||
it->second->Success (r); |
||||
else |
||||
it->second->Fail (); |
||||
std::unique_lock<std::mutex> l(m_RequestedDestinationsMutex); |
||||
m_RequestedDestinations.erase (it); |
||||
} |
||||
} |
||||
|
||||
std::shared_ptr<RequestedDestination> NetDbRequests::FindRequest (const IdentHash& ident) const |
||||
{ |
||||
auto it = m_RequestedDestinations.find (ident); |
||||
if (it != m_RequestedDestinations.end ()) |
||||
return it->second; |
||||
return nullptr; |
||||
} |
||||
|
||||
void NetDbRequests::ManageRequests () |
||||
{ |
||||
uint64_t ts = i2p::util::GetSecondsSinceEpoch (); |
||||
std::unique_lock<std::mutex> l(m_RequestedDestinationsMutex); |
||||
for (auto it = m_RequestedDestinations.begin (); it != m_RequestedDestinations.end ();) |
||||
{ |
||||
auto& dest = it->second; |
||||
bool done = false; |
||||
if (ts < dest->GetCreationTime () + 60) // request is worthless after 1 minute
|
||||
{ |
||||
if (ts > dest->GetCreationTime () + 5) // no response for 5 seconds
|
||||
{ |
||||
auto count = dest->GetExcludedPeers ().size (); |
||||
if (!dest->IsExploratory () && count < 7) |
||||
{ |
||||
auto pool = i2p::tunnel::tunnels.GetExploratoryPool (); |
||||
auto outbound = pool->GetNextOutboundTunnel (); |
||||
auto inbound = pool->GetNextInboundTunnel (); |
||||
auto nextFloodfill = netdb.GetClosestFloodfill (dest->GetDestination (), dest->GetExcludedPeers ()); |
||||
if (nextFloodfill && outbound && inbound) |
||||
outbound->SendTunnelDataMsg (nextFloodfill->GetIdentHash (), 0, |
||||
dest->CreateRequestMessage (nextFloodfill, inbound)); |
||||
else |
||||
{ |
||||
done = true; |
||||
if (!inbound) LogPrint (eLogWarning, "No inbound tunnels"); |
||||
if (!outbound) LogPrint (eLogWarning, "No outbound tunnels"); |
||||
if (!nextFloodfill) LogPrint (eLogWarning, "No more floodfills"); |
||||
} |
||||
} |
||||
else |
||||
{ |
||||
if (!dest->IsExploratory ()) |
||||
LogPrint (eLogWarning, dest->GetDestination ().ToBase64 (), " not found after 7 attempts"); |
||||
done = true; |
||||
} |
||||
} |
||||
} |
||||
else // delete obsolete request
|
||||
done = true; |
||||
|
||||
if (done) |
||||
it = m_RequestedDestinations.erase (it); |
||||
else |
||||
it++; |
||||
} |
||||
} |
||||
} |
||||
} |
||||
|
@ -0,0 +1,69 @@
@@ -0,0 +1,69 @@
|
||||
#ifndef NETDB_REQUESTS_H__ |
||||
#define NETDB_REQUESTS_H__ |
||||
|
||||
#include <memory> |
||||
#include <set> |
||||
#include <map> |
||||
#include "Identity.h" |
||||
#include "RouterInfo.h" |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace data |
||||
{ |
||||
class RequestedDestination |
||||
{ |
||||
public: |
||||
|
||||
typedef std::function<void (std::shared_ptr<RouterInfo>)> RequestComplete; |
||||
|
||||
RequestedDestination (const IdentHash& destination, bool isExploratory = false): |
||||
m_Destination (destination), m_IsExploratory (isExploratory), m_CreationTime (0) {}; |
||||
~RequestedDestination () { if (m_RequestComplete) m_RequestComplete (nullptr); }; |
||||
|
||||
const IdentHash& GetDestination () const { return m_Destination; }; |
||||
int GetNumExcludedPeers () const { return m_ExcludedPeers.size (); }; |
||||
const std::set<IdentHash>& GetExcludedPeers () { return m_ExcludedPeers; }; |
||||
void ClearExcludedPeers (); |
||||
bool IsExploratory () const { return m_IsExploratory; }; |
||||
bool IsExcluded (const IdentHash& ident) const { return m_ExcludedPeers.count (ident); }; |
||||
uint64_t GetCreationTime () const { return m_CreationTime; }; |
||||
I2NPMessage * CreateRequestMessage (std::shared_ptr<const RouterInfo>, std::shared_ptr<const i2p::tunnel::InboundTunnel> replyTunnel); |
||||
I2NPMessage * CreateRequestMessage (const IdentHash& floodfill); |
||||
|
||||
void SetRequestComplete (const RequestComplete& requestComplete) { m_RequestComplete = requestComplete; }; |
||||
bool IsRequestComplete () const { return m_RequestComplete != nullptr; }; |
||||
void Success (std::shared_ptr<RouterInfo> r); |
||||
void Fail (); |
||||
|
||||
private: |
||||
|
||||
IdentHash m_Destination; |
||||
bool m_IsExploratory; |
||||
std::set<IdentHash> m_ExcludedPeers; |
||||
uint64_t m_CreationTime; |
||||
RequestComplete m_RequestComplete; |
||||
}; |
||||
|
||||
class NetDbRequests |
||||
{ |
||||
public: |
||||
|
||||
void Start (); |
||||
void Stop (); |
||||
|
||||
std::shared_ptr<RequestedDestination> CreateRequest (const IdentHash& destination, bool isExploratory, RequestedDestination::RequestComplete requestComplete = nullptr); |
||||
void RequestComplete (const IdentHash& ident, std::shared_ptr<RouterInfo> r); |
||||
std::shared_ptr<RequestedDestination> FindRequest (const IdentHash& ident) const; |
||||
void ManageRequests (); |
||||
|
||||
private: |
||||
|
||||
std::mutex m_RequestedDestinationsMutex; |
||||
std::map<IdentHash, std::shared_ptr<RequestedDestination> > m_RequestedDestinations; |
||||
}; |
||||
} |
||||
} |
||||
|
||||
#endif |
||||
|
Loading…
Reference in new issue