mirror of https://github.com/PurpleI2P/i2pd.git
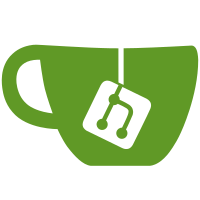
3 changed files with 149 additions and 0 deletions
@ -0,0 +1,3 @@
@@ -0,0 +1,3 @@
|
||||
#include "Log.h" |
||||
|
||||
i2p::util::MsgQueue<LogMsg> g_Log; |
@ -0,0 +1,45 @@
@@ -0,0 +1,45 @@
|
||||
#ifndef LOG_H__ |
||||
#define LOG_H__ |
||||
|
||||
#include <iostream> |
||||
#include <sstream> |
||||
#include "Queue.h" |
||||
|
||||
struct LogMsg |
||||
{ |
||||
std::stringstream s; |
||||
std::ostream& output; |
||||
|
||||
LogMsg (std::ostream& o = std::cout): output (o) {}; |
||||
|
||||
void Process () |
||||
{ |
||||
output << s.str (); |
||||
} |
||||
}; |
||||
|
||||
extern i2p::util::MsgQueue<LogMsg> g_Log; |
||||
|
||||
template<typename TValue> |
||||
void LogPrint (std::stringstream& s, TValue arg) |
||||
{ |
||||
s << arg; |
||||
} |
||||
|
||||
template<typename TValue, typename... TArgs> |
||||
void LogPrint (std::stringstream& s, TValue arg, TArgs... args) |
||||
{ |
||||
LogPrint (s, arg); |
||||
LogPrint (s, args...); |
||||
} |
||||
|
||||
template<typename... TArgs> |
||||
void LogPrint (TArgs... args) |
||||
{ |
||||
LogMsg * msg = new LogMsg (); |
||||
LogPrint (msg->s, args...); |
||||
msg->s << std::endl; |
||||
g_Log.Put (msg); |
||||
} |
||||
|
||||
#endif |
@ -0,0 +1,101 @@
@@ -0,0 +1,101 @@
|
||||
#ifndef QUEUE_H__ |
||||
#define QUEUE_H__ |
||||
|
||||
#include <queue> |
||||
#include <mutex> |
||||
#include <thread> |
||||
#include <condition_variable> |
||||
|
||||
namespace i2p |
||||
{ |
||||
namespace util |
||||
{ |
||||
template<typename Element> |
||||
class Queue |
||||
{ |
||||
public: |
||||
|
||||
void Put (Element * e) |
||||
{ |
||||
std::unique_lock<std::mutex> l(m_QueueMutex); |
||||
m_Queue.push (e); |
||||
m_NonEmpty.notify_one (); |
||||
} |
||||
|
||||
Element * GetNext () |
||||
{ |
||||
std::unique_lock<std::mutex> l(m_QueueMutex); |
||||
Element * el = GetNonThreadSafe (); |
||||
if (!el) |
||||
{ |
||||
m_NonEmpty.wait (l); |
||||
el = GetNonThreadSafe (); |
||||
} |
||||
return el; |
||||
} |
||||
|
||||
Element * GetNextWithTimeout (int usec) |
||||
{ |
||||
std::unique_lock<std::mutex> l(m_QueueMutex); |
||||
Element * el = GetNonThreadSafe (); |
||||
if (!el) |
||||
{ |
||||
m_NonEmpty.wait_for (l, std::chrono::milliseconds (usec)); |
||||
el = GetNonThreadSafe (); |
||||
} |
||||
return el; |
||||
} |
||||
|
||||
void WakeUp () { m_NonEmpty.notify_one (); }; |
||||
|
||||
Element * Get () |
||||
{ |
||||
std::unique_lock<std::mutex> l(m_QueueMutex); |
||||
return GetNonThreadSafe (); |
||||
} |
||||
|
||||
private: |
||||
|
||||
Element * GetNonThreadSafe () |
||||
{ |
||||
if (!m_Queue.empty ()) |
||||
{ |
||||
Element * el = m_Queue.front (); |
||||
m_Queue.pop (); |
||||
return el; |
||||
} |
||||
return nullptr; |
||||
} |
||||
|
||||
private: |
||||
|
||||
std::queue<Element *> m_Queue; |
||||
std::mutex m_QueueMutex; |
||||
std::condition_variable m_NonEmpty; |
||||
}; |
||||
|
||||
template<class Msg> |
||||
class MsgQueue: public Queue<Msg> |
||||
{ |
||||
public: |
||||
|
||||
MsgQueue (): m_Thread (std::bind (&MsgQueue<Msg>::Run, this)) {}; |
||||
|
||||
private: |
||||
void Run () |
||||
{ |
||||
Msg * msg = nullptr; |
||||
while ((msg = Queue<Msg>::GetNext ()) != nullptr) |
||||
{ |
||||
msg->Process (); |
||||
delete msg; |
||||
} |
||||
} |
||||
|
||||
private: |
||||
std::thread m_Thread; |
||||
}; |
||||
} |
||||
} |
||||
|
||||
#endif |
Loading…
Reference in new issue