mirror of https://github.com/PurpleI2P/i2pd.git
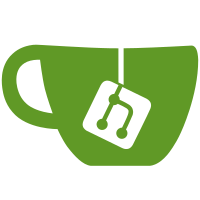
21 changed files with 12836 additions and 1406 deletions
@ -0,0 +1,22 @@
@@ -0,0 +1,22 @@
|
||||
#include "ClientTunnelPane.h" |
||||
|
||||
ClientTunnelPane::ClientTunnelPane() |
||||
{ |
||||
|
||||
} |
||||
|
||||
void ClientTunnelPane::deleteClientTunnelForm(QGridLayout *tunnelsFormGridLayout) { |
||||
throw "TODO"; |
||||
/*TODO
|
||||
tunnelsFormGridLayout->removeWidget(clientTunnelNameGroupBox); |
||||
|
||||
clientTunnelNameGroupBox->deleteLater(); |
||||
clientTunnelNameGroupBox=nullptr; |
||||
|
||||
gridLayoutWidget_2->deleteLater(); |
||||
gridLayoutWidget_2=nullptr; |
||||
*/ |
||||
} |
||||
|
||||
ServerTunnelPane* ClientTunnelPane::asServerTunnelPane(){return nullptr;} |
||||
ClientTunnelPane* ClientTunnelPane::asClientTunnelPane(){return this;} |
@ -0,0 +1,21 @@
@@ -0,0 +1,21 @@
|
||||
#ifndef CLIENTTUNNELPANE_H |
||||
#define CLIENTTUNNELPANE_H |
||||
|
||||
#include "QGridLayout" |
||||
|
||||
#include "TunnelPane.h" |
||||
|
||||
class ServerTunnelPane; |
||||
class TunnelPane; |
||||
|
||||
class ClientTunnelPane : public TunnelPane { |
||||
public: |
||||
ClientTunnelPane(); |
||||
virtual ServerTunnelPane* asServerTunnelPane(); |
||||
virtual ClientTunnelPane* asClientTunnelPane(); |
||||
void deleteClientTunnelForm(QGridLayout *tunnelsFormGridLayout); |
||||
protected slots: |
||||
virtual void setGroupBoxTitle(const QString & title){}//TODO
|
||||
}; |
||||
|
||||
#endif // CLIENTTUNNELPANE_H
|
@ -0,0 +1,2 @@
@@ -0,0 +1,2 @@
|
||||
#include "MainWindowItems.h" |
||||
|
@ -0,0 +1,17 @@
@@ -0,0 +1,17 @@
|
||||
#ifndef MAINWINDOWITEMS_H |
||||
#define MAINWINDOWITEMS_H |
||||
|
||||
#include <QString> |
||||
#include <QLineEdit> |
||||
#include <QPushButton> |
||||
#include <QComboBox> |
||||
#include <QCheckBox> |
||||
|
||||
#include <sstream> |
||||
#include <functional> |
||||
|
||||
#include "mainwindow.h" |
||||
|
||||
class MainWindow; |
||||
|
||||
#endif // MAINWINDOWITEMS_H
|
@ -0,0 +1,239 @@
@@ -0,0 +1,239 @@
|
||||
#include "ServerTunnelPane.h" |
||||
#include "../../ClientContext.h" |
||||
|
||||
ServerTunnelPane::ServerTunnelPane(): TunnelPane() {} |
||||
|
||||
void ServerTunnelPane::setGroupBoxTitle(const QString & title) { |
||||
serverTunnelNameGroupBox->setTitle(title); |
||||
} |
||||
|
||||
void ServerTunnelPane::appendServerTunnelForm( |
||||
ServerTunnelConfig* tunnelConfig, QWidget *tunnelsFormGridLayoutWidget, QGridLayout *tunnelsFormGridLayout) { |
||||
|
||||
tunnelsFormGridLayoutWidget->resize(527, 452); |
||||
|
||||
ServerTunnelPane& ui = *this; |
||||
|
||||
serverTunnelNameGroupBox = new QGroupBox(tunnelsFormGridLayoutWidget); |
||||
serverTunnelNameGroupBox->setObjectName(QStringLiteral("serverTunnelNameGroupBox")); |
||||
|
||||
//tunnel
|
||||
ui.gridLayoutWidget_2 = new QWidget(serverTunnelNameGroupBox); |
||||
|
||||
QComboBox *tunnelTypeComboBox = new QComboBox(gridLayoutWidget_2); |
||||
tunnelTypeComboBox->setObjectName(QStringLiteral("tunnelTypeComboBox")); |
||||
tunnelTypeComboBox->addItem("Server", i2p::client::I2P_TUNNELS_SECTION_TYPE_SERVER); |
||||
tunnelTypeComboBox->addItem("HTTP", i2p::client::I2P_TUNNELS_SECTION_TYPE_HTTP); |
||||
tunnelTypeComboBox->addItem("IRC", i2p::client::I2P_TUNNELS_SECTION_TYPE_IRC); |
||||
tunnelTypeComboBox->addItem("UDP Server", i2p::client::I2P_TUNNELS_SECTION_TYPE_UDPSERVER); |
||||
|
||||
gridLayoutWidget_2->setGeometry(QRect(0, 10, 561, 18*40+10)); |
||||
|
||||
setupTunnelPane(tunnelConfig, |
||||
serverTunnelNameGroupBox, |
||||
gridLayoutWidget_2, tunnelTypeComboBox, |
||||
tunnelsFormGridLayoutWidget, tunnelsFormGridLayout); |
||||
|
||||
{ |
||||
const QString& type = tunnelConfig->getType(); |
||||
int index=0; |
||||
if(type==i2p::client::I2P_TUNNELS_SECTION_TYPE_SERVER)tunnelTypeComboBox->setCurrentIndex(index); |
||||
++index; |
||||
if(type==i2p::client::I2P_TUNNELS_SECTION_TYPE_HTTP)tunnelTypeComboBox->setCurrentIndex(index); |
||||
++index; |
||||
if(type==i2p::client::I2P_TUNNELS_SECTION_TYPE_IRC)tunnelTypeComboBox->setCurrentIndex(index); |
||||
++index; |
||||
if(type==i2p::client::I2P_TUNNELS_SECTION_TYPE_UDPSERVER)tunnelTypeComboBox->setCurrentIndex(index); |
||||
++index; |
||||
} |
||||
|
||||
//host
|
||||
ui.horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.hostLabel = new QLabel(gridLayoutWidget_2); |
||||
hostLabel->setObjectName(QStringLiteral("hostLabel")); |
||||
horizontalLayout_2->addWidget(hostLabel); |
||||
ui.hostLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
hostLineEdit->setObjectName(QStringLiteral("hostLineEdit")); |
||||
hostLineEdit->setText(tunnelConfig->gethost().c_str()); |
||||
horizontalLayout_2->addWidget(hostLineEdit); |
||||
ui.hostHorizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(hostHorizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, 2, 0, 1, 1); |
||||
|
||||
int gridIndex = 2; |
||||
{ |
||||
int port = tunnelConfig->getport(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.portLabel = new QLabel(gridLayoutWidget_2); |
||||
portLabel->setObjectName(QStringLiteral("portLabel")); |
||||
horizontalLayout_2->addWidget(portLabel); |
||||
ui.portLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
portLineEdit->setObjectName(QStringLiteral("portLineEdit")); |
||||
portLineEdit->setText(QString::number(port)); |
||||
portLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(portLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
std::string keys = tunnelConfig->getkeys(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.keysLabel = new QLabel(gridLayoutWidget_2); |
||||
keysLabel->setObjectName(QStringLiteral("keysLabel")); |
||||
horizontalLayout_2->addWidget(keysLabel); |
||||
ui.keysLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
keysLineEdit->setObjectName(QStringLiteral("keysLineEdit")); |
||||
keysLineEdit->setText(keys.c_str()); |
||||
horizontalLayout_2->addWidget(keysLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
int inPort = tunnelConfig->getinPort(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.inPortLabel = new QLabel(gridLayoutWidget_2); |
||||
inPortLabel->setObjectName(QStringLiteral("inPortLabel")); |
||||
horizontalLayout_2->addWidget(inPortLabel); |
||||
ui.inPortLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
inPortLineEdit->setObjectName(QStringLiteral("inPortLineEdit")); |
||||
inPortLineEdit->setText(QString::number(inPort)); |
||||
inPortLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(inPortLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
std::string accessList = tunnelConfig->getaccessList(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.accessListLabel = new QLabel(gridLayoutWidget_2); |
||||
accessListLabel->setObjectName(QStringLiteral("accessListLabel")); |
||||
horizontalLayout_2->addWidget(accessListLabel); |
||||
ui.accessListLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
accessListLineEdit->setObjectName(QStringLiteral("accessListLineEdit")); |
||||
accessListLineEdit->setText(accessList.c_str()); |
||||
horizontalLayout_2->addWidget(accessListLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
std::string hostOverride = tunnelConfig->gethostOverride(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.hostOverrideLabel = new QLabel(gridLayoutWidget_2); |
||||
hostOverrideLabel->setObjectName(QStringLiteral("hostOverrideLabel")); |
||||
horizontalLayout_2->addWidget(hostOverrideLabel); |
||||
ui.hostOverrideLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
hostOverrideLineEdit->setObjectName(QStringLiteral("hostOverrideLineEdit")); |
||||
hostOverrideLineEdit->setText(hostOverride.c_str()); |
||||
horizontalLayout_2->addWidget(hostOverrideLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
std::string webIRCPass = tunnelConfig->getwebircpass(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.webIRCPassLabel = new QLabel(gridLayoutWidget_2); |
||||
webIRCPassLabel->setObjectName(QStringLiteral("webIRCPassLabel")); |
||||
horizontalLayout_2->addWidget(webIRCPassLabel); |
||||
ui.webIRCPassLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
webIRCPassLineEdit->setObjectName(QStringLiteral("webIRCPassLineEdit")); |
||||
webIRCPassLineEdit->setText(webIRCPass.c_str()); |
||||
horizontalLayout_2->addWidget(webIRCPassLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
bool gzip = tunnelConfig->getgzip(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.gzipCheckBox = new QCheckBox(gridLayoutWidget_2); |
||||
gzipCheckBox->setObjectName(QStringLiteral("gzipCheckBox")); |
||||
gzipCheckBox->setChecked(gzip); |
||||
horizontalLayout_2->addWidget(gzipCheckBox); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
i2p::data::SigningKeyType sigType = tunnelConfig->getsigType(); |
||||
//combo box
|
||||
//TODO sigtype
|
||||
} |
||||
{ |
||||
uint32_t maxConns = tunnelConfig->getmaxConns(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.maxConnsLabel = new QLabel(gridLayoutWidget_2); |
||||
maxConnsLabel->setObjectName(QStringLiteral("maxConnsLabel")); |
||||
horizontalLayout_2->addWidget(maxConnsLabel); |
||||
ui.maxConnsLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
maxConnsLineEdit->setObjectName(QStringLiteral("maxConnsLineEdit")); |
||||
maxConnsLineEdit->setText(QString::number(maxConns)); |
||||
maxConnsLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(maxConnsLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
std::string address = tunnelConfig->getaddress(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.addressLabel = new QLabel(gridLayoutWidget_2); |
||||
addressLabel->setObjectName(QStringLiteral("addressLabel")); |
||||
horizontalLayout_2->addWidget(addressLabel); |
||||
ui.addressLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
addressLineEdit->setObjectName(QStringLiteral("addressLineEdit")); |
||||
addressLineEdit->setText(address.c_str()); |
||||
horizontalLayout_2->addWidget(addressLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
bool isUniqueLocal = tunnelConfig->getisUniqueLocal(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
ui.isUniqueLocalCheckBox = new QCheckBox(gridLayoutWidget_2); |
||||
isUniqueLocalCheckBox->setObjectName(QStringLiteral("isUniqueLocalCheckBox")); |
||||
isUniqueLocalCheckBox->setChecked(isUniqueLocal); |
||||
horizontalLayout_2->addWidget(isUniqueLocalCheckBox); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
I2CPParameters& i2cpParameters = tunnelConfig->getI2cpParameters(); |
||||
appendControlsForI2CPParameters(i2cpParameters, gridIndex); |
||||
} |
||||
|
||||
tunnelsFormGridLayout->addWidget(serverTunnelNameGroupBox, 0, 0, 1, 1); |
||||
|
||||
retranslateServerTunnelForm(ui); |
||||
} |
||||
|
||||
void ServerTunnelPane::deleteServerTunnelForm(QGridLayout *tunnelsFormGridLayout) { |
||||
tunnelsFormGridLayout->removeWidget(tunnelGroupBox); |
||||
|
||||
tunnelGroupBox->deleteLater(); |
||||
tunnelGroupBox=nullptr; |
||||
|
||||
gridLayoutWidget_2->deleteLater(); |
||||
gridLayoutWidget_2=nullptr; |
||||
} |
||||
|
||||
|
||||
ServerTunnelPane* ServerTunnelPane::asServerTunnelPane(){return this;} |
||||
ClientTunnelPane* ServerTunnelPane::asClientTunnelPane(){return nullptr;} |
@ -0,0 +1,110 @@
@@ -0,0 +1,110 @@
|
||||
#ifndef SERVERTUNNELPANE_H |
||||
#define SERVERTUNNELPANE_H |
||||
|
||||
#include "TunnelPane.h" |
||||
#include "mainwindow.h" |
||||
|
||||
#include <QtCore/QVariant> |
||||
#include <QtWidgets/QAction> |
||||
#include <QtWidgets/QApplication> |
||||
#include <QtWidgets/QButtonGroup> |
||||
#include <QtWidgets/QComboBox> |
||||
#include <QtWidgets/QGridLayout> |
||||
#include <QtWidgets/QGroupBox> |
||||
#include <QtWidgets/QHBoxLayout> |
||||
#include <QtWidgets/QHeaderView> |
||||
#include <QtWidgets/QLabel> |
||||
#include <QtWidgets/QLineEdit> |
||||
#include <QtWidgets/QPushButton> |
||||
#include <QtWidgets/QSpacerItem> |
||||
#include <QtWidgets/QWidget> |
||||
|
||||
class ServerTunnelConfig; |
||||
|
||||
class ClientTunnelPane; |
||||
|
||||
class ServerTunnelPane : public TunnelPane { |
||||
Q_OBJECT |
||||
|
||||
public: |
||||
ServerTunnelPane(); |
||||
virtual ~ServerTunnelPane(){} |
||||
|
||||
virtual ServerTunnelPane* asServerTunnelPane(); |
||||
virtual ClientTunnelPane* asClientTunnelPane(); |
||||
|
||||
void appendServerTunnelForm(ServerTunnelConfig* tunnelConfig, QWidget *tunnelsFormGridLayoutWidget, QGridLayout *tunnelsFormGridLayout); |
||||
void deleteServerTunnelForm(QGridLayout *tunnelsFormGridLayout); |
||||
|
||||
private: |
||||
QGroupBox *serverTunnelNameGroupBox; |
||||
|
||||
//tunnel
|
||||
QWidget *gridLayoutWidget_2; |
||||
|
||||
//host
|
||||
QHBoxLayout *horizontalLayout_2; |
||||
QLabel *hostLabel; |
||||
QLineEdit *hostLineEdit; |
||||
QSpacerItem *hostHorizontalSpacer; |
||||
|
||||
//port
|
||||
QLabel * portLabel; |
||||
QLineEdit * portLineEdit; |
||||
|
||||
//keys
|
||||
QLabel * keysLabel; |
||||
QLineEdit * keysLineEdit; |
||||
|
||||
//inPort
|
||||
QLabel * inPortLabel; |
||||
QLineEdit * inPortLineEdit; |
||||
|
||||
//accessList
|
||||
QLabel * accessListLabel; |
||||
QLineEdit * accessListLineEdit; |
||||
|
||||
//hostOverride
|
||||
QLabel * hostOverrideLabel; |
||||
QLineEdit * hostOverrideLineEdit; |
||||
|
||||
//webIRCPass
|
||||
QLabel * webIRCPassLabel; |
||||
QLineEdit * webIRCPassLineEdit; |
||||
|
||||
//address
|
||||
QLabel * addressLabel; |
||||
QLineEdit * addressLineEdit; |
||||
|
||||
//maxConns
|
||||
QLabel * maxConnsLabel; |
||||
QLineEdit * maxConnsLineEdit; |
||||
|
||||
//gzip
|
||||
QCheckBox * gzipCheckBox; |
||||
|
||||
//isUniqueLocal
|
||||
QCheckBox * isUniqueLocalCheckBox; |
||||
|
||||
protected slots: |
||||
virtual void setGroupBoxTitle(const QString & title); |
||||
|
||||
private: |
||||
void retranslateServerTunnelForm(ServerTunnelPane& /*ui*/) { |
||||
hostLabel->setText(QApplication::translate("srvTunForm", "Host:", 0)); |
||||
portLabel->setText(QApplication::translate("srvTunForm", "Port:", 0)); |
||||
keysLabel->setText(QApplication::translate("srvTunForm", "Keys:", 0)); |
||||
inPortLabel->setText(QApplication::translate("srvTunForm", "InPort:", 0)); |
||||
accessListLabel->setText(QApplication::translate("srvTunForm", "Access list:", 0)); |
||||
hostOverrideLabel->setText(QApplication::translate("srvTunForm", "Host override:", 0)); |
||||
webIRCPassLabel->setText(QApplication::translate("srvTunForm", "WebIRC password:", 0)); |
||||
addressLabel->setText(QApplication::translate("srvTunForm", "Address:", 0)); |
||||
maxConnsLabel->setText(QApplication::translate("srvTunForm", "Max connections:", 0)); |
||||
|
||||
gzipCheckBox->setText(QApplication::translate("srvTunForm", "GZip", 0)); |
||||
isUniqueLocalCheckBox->setText(QApplication::translate("srvTunForm", "Is unique local", 0)); |
||||
} |
||||
|
||||
}; |
||||
|
||||
#endif // SERVERTUNNELPANE_H
|
@ -0,0 +1,2 @@
@@ -0,0 +1,2 @@
|
||||
#include "TunnelConfig.h" |
||||
|
@ -0,0 +1,221 @@
@@ -0,0 +1,221 @@
|
||||
#ifndef TUNNELCONFIG_H |
||||
#define TUNNELCONFIG_H |
||||
|
||||
#include "QString" |
||||
#include <string> |
||||
|
||||
#include "../../ClientContext.h" |
||||
|
||||
|
||||
class I2CPParameters{ |
||||
QString inbound_length;//number of hops of an inbound tunnel. 3 by default; lower value is faster but dangerous
|
||||
QString outbound_length;//number of hops of an outbound tunnel. 3 by default; lower value is faster but dangerous
|
||||
QString inbound_quantity; //number of inbound tunnels. 5 by default
|
||||
QString outbound_quantity; //number of outbound tunnels. 5 by default
|
||||
QString crypto_tagsToSend; //number of ElGamal/AES tags to send. 40 by default; too low value may cause problems with tunnel building
|
||||
QString explicitPeers; //list of comma-separated b64 addresses of peers to use, default: unset
|
||||
public: |
||||
I2CPParameters(): inbound_length(), |
||||
outbound_length(), |
||||
inbound_quantity(), |
||||
outbound_quantity(), |
||||
crypto_tagsToSend(), |
||||
explicitPeers() {} |
||||
const QString& getInbound_length(){return inbound_length;} |
||||
const QString& getOutbound_length(){return outbound_length;} |
||||
const QString& getInbound_quantity(){return inbound_quantity;} |
||||
const QString& getOutbound_quantity(){return outbound_quantity;} |
||||
const QString& getCrypto_tagsToSend(){return crypto_tagsToSend;} |
||||
const QString& getExplicitPeers(){return explicitPeers;} |
||||
void setInbound_length(QString inbound_length_){inbound_length=inbound_length_;} |
||||
void setOutbound_length(QString outbound_length_){outbound_length=outbound_length_;} |
||||
void setInbound_quantity(QString inbound_quantity_){inbound_quantity=inbound_quantity_;} |
||||
void setOutbound_quantity(QString outbound_quantity_){outbound_quantity=outbound_quantity_;} |
||||
void setCrypto_tagsToSend(QString crypto_tagsToSend_){crypto_tagsToSend=crypto_tagsToSend_;} |
||||
void setExplicitPeers(QString explicitPeers_){explicitPeers=explicitPeers_;} |
||||
}; |
||||
|
||||
|
||||
class ClientTunnelConfig; |
||||
class ServerTunnelConfig; |
||||
class TunnelConfig { |
||||
/*
|
||||
const char I2P_TUNNELS_SECTION_TYPE_CLIENT[] = "client"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_SERVER[] = "server"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_HTTP[] = "http"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_IRC[] = "irc"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_UDPCLIENT[] = "udpclient"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_UDPSERVER[] = "udpserver"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_SOCKS[] = "socks"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_WEBSOCKS[] = "websocks"; |
||||
const char I2P_TUNNELS_SECTION_TYPE_HTTPPROXY[] = "httpproxy"; |
||||
*/ |
||||
QString type; |
||||
std::string name; |
||||
public: |
||||
TunnelConfig(std::string name_, QString& type_, I2CPParameters& i2cpParameters_): type(type_), name(name_), i2cpParameters(i2cpParameters_) {} |
||||
const QString& getType(){return type;} |
||||
const std::string& getName(){return name;} |
||||
void setType(const QString& type_){type=type_;} |
||||
void setName(const std::string& name_){name=name_;} |
||||
I2CPParameters& getI2cpParameters(){return i2cpParameters;} |
||||
virtual ClientTunnelConfig* asClientTunnelConfig()=0; |
||||
virtual ServerTunnelConfig* asServerTunnelConfig()=0; |
||||
|
||||
private: |
||||
I2CPParameters i2cpParameters; |
||||
}; |
||||
|
||||
/*
|
||||
# mandatory parameters: |
||||
std::string dest; |
||||
if (type == I2P_TUNNELS_SECTION_TYPE_CLIENT || type == I2P_TUNNELS_SECTION_TYPE_UDPCLIENT) |
||||
dest = section.second.get<std::string> (I2P_CLIENT_TUNNEL_DESTINATION); |
||||
int port = section.second.get<int> (I2P_CLIENT_TUNNEL_PORT); |
||||
# optional parameters (may be omitted) |
||||
std::string keys = section.second.get (I2P_CLIENT_TUNNEL_KEYS, ""); |
||||
std::string address = section.second.get (I2P_CLIENT_TUNNEL_ADDRESS, "127.0.0.1"); |
||||
int destinationPort = section.second.get (I2P_CLIENT_TUNNEL_DESTINATION_PORT, 0); |
||||
i2p::data::SigningKeyType sigType = section.second.get (I2P_CLIENT_TUNNEL_SIGNATURE_TYPE, i2p::data::SIGNING_KEY_TYPE_ECDSA_SHA256_P256); |
||||
# * keys -- our identity, if unset, will be generated on every startup, |
||||
# if set and file missing, keys will be generated and placed to this file |
||||
# * address -- local interface to bind |
||||
# * signaturetype -- signature type for new destination. 0 (DSA/SHA1), 1 (EcDSA/SHA256) or 7 (EdDSA/SHA512) |
||||
[somelabel] |
||||
type = client |
||||
address = 127.0.0.1 |
||||
port = 6668 |
||||
destination = irc.postman.i2p |
||||
keys = irc-keys.dat |
||||
*/ |
||||
class ClientTunnelConfig : public TunnelConfig { |
||||
std::string dest; |
||||
int port; |
||||
std::string keys; |
||||
std::string address; |
||||
int destinationPort; |
||||
i2p::data::SigningKeyType sigType; |
||||
public: |
||||
ClientTunnelConfig(std::string name_, QString type_, I2CPParameters& i2cpParameters_, |
||||
std::string dest_, |
||||
int port_, |
||||
std::string keys_, |
||||
std::string address_, |
||||
int destinationPort_, |
||||
i2p::data::SigningKeyType sigType_): TunnelConfig(name_, type_, i2cpParameters_), |
||||
dest(dest_), |
||||
port(port_), |
||||
keys(keys_), |
||||
address(address_), |
||||
destinationPort(destinationPort_) {} |
||||
std::string& getdest(){return dest;} |
||||
int getport(){return port;} |
||||
std::string & getkeys(){return keys;} |
||||
std::string & getaddress(){return address;} |
||||
int getdestinationPort(){return destinationPort;} |
||||
i2p::data::SigningKeyType getsigType(){return sigType;} |
||||
void setdest(std::string& dest_){dest=dest_;} |
||||
void setport(int port_){port=port_;} |
||||
void setkeys(std::string & keys_){keys=keys_;} |
||||
void setaddress(std::string & address_){address=address_;} |
||||
void setdestinationPort(int destinationPort_){destinationPort=destinationPort_;} |
||||
void setsigType(i2p::data::SigningKeyType sigType_){sigType=sigType_;} |
||||
virtual ClientTunnelConfig* asClientTunnelConfig(){return this;} |
||||
virtual ServerTunnelConfig* asServerTunnelConfig(){return nullptr;} |
||||
}; |
||||
|
||||
/*
|
||||
# mandatory parameters: |
||||
# * host -- ip address of our service |
||||
# * port -- port of our service |
||||
# * keys -- file with LeaseSet of address in i2p |
||||
std::string host = section.second.get<std::string> (I2P_SERVER_TUNNEL_HOST); |
||||
int port = section.second.get<int> (I2P_SERVER_TUNNEL_PORT); |
||||
std::string keys = section.second.get<std::string> (I2P_SERVER_TUNNEL_KEYS); |
||||
# optional parameters (may be omitted) |
||||
int inPort = section.second.get (I2P_SERVER_TUNNEL_INPORT, 0); |
||||
std::string accessList = section.second.get (I2P_SERVER_TUNNEL_ACCESS_LIST, ""); |
||||
std::string hostOverride = section.second.get (I2P_SERVER_TUNNEL_HOST_OVERRIDE, ""); |
||||
std::string webircpass = section.second.get<std::string> (I2P_SERVER_TUNNEL_WEBIRC_PASSWORD, ""); |
||||
bool gzip = section.second.get (I2P_SERVER_TUNNEL_GZIP, true); |
||||
i2p::data::SigningKeyType sigType = section.second.get (I2P_SERVER_TUNNEL_SIGNATURE_TYPE, i2p::data::SIGNING_KEY_TYPE_ECDSA_SHA256_P256); |
||||
uint32_t maxConns = section.second.get(i2p::stream::I2CP_PARAM_STREAMING_MAX_CONNS_PER_MIN, i2p::stream::DEFAULT_MAX_CONNS_PER_MIN); |
||||
std::string address = section.second.get<std::string> (I2P_SERVER_TUNNEL_ADDRESS, "127.0.0.1"); |
||||
bool isUniqueLocal = section.second.get(I2P_SERVER_TUNNEL_ENABLE_UNIQUE_LOCAL, true); |
||||
# * inport -- optional, i2p service port, if unset - the same as 'port' |
||||
# * accesslist -- comma-separated list of i2p addresses, allowed to connect |
||||
# every address is b32 without '.b32.i2p' part |
||||
[somelabel] |
||||
type = server |
||||
host = 127.0.0.1 |
||||
port = 6667 |
||||
keys = irc.dat |
||||
*/ |
||||
class ServerTunnelConfig : public TunnelConfig { |
||||
std::string host; |
||||
int port; |
||||
std::string keys; |
||||
int inPort; |
||||
std::string accessList; |
||||
std::string hostOverride; |
||||
std::string webircpass; |
||||
bool gzip; |
||||
i2p::data::SigningKeyType sigType; |
||||
uint32_t maxConns; |
||||
std::string address; |
||||
bool isUniqueLocal; |
||||
public: |
||||
ServerTunnelConfig(std::string name_, QString type_, I2CPParameters& i2cpParameters_, |
||||
std::string host_, |
||||
int port_, |
||||
std::string keys_, |
||||
int inPort_, |
||||
std::string accessList_, |
||||
std::string hostOverride_, |
||||
std::string webircpass_, |
||||
bool gzip_, |
||||
i2p::data::SigningKeyType sigType_, |
||||
uint32_t maxConns_, |
||||
std::string address_, |
||||
bool isUniqueLocal_): TunnelConfig(name_, type_, i2cpParameters_), |
||||
host(host_), |
||||
port(port_), |
||||
keys(keys_), |
||||
inPort(inPort_), |
||||
accessList(accessList_), |
||||
hostOverride(hostOverride_), |
||||
webircpass(webircpass_), |
||||
gzip(gzip_), |
||||
sigType(sigType_), |
||||
maxConns(maxConns_), |
||||
address(address_) {} |
||||
std::string& gethost(){return host;} |
||||
int getport(){return port;} |
||||
std::string& getkeys(){return keys;} |
||||
int getinPort(){return inPort;} |
||||
std::string& getaccessList(){return accessList;} |
||||
std::string& gethostOverride(){return hostOverride;} |
||||
std::string& getwebircpass(){return webircpass;} |
||||
bool getgzip(){return gzip;} |
||||
i2p::data::SigningKeyType getsigType(){return sigType;} |
||||
uint32_t getmaxConns(){return maxConns;} |
||||
std::string& getaddress(){return address;} |
||||
bool getisUniqueLocal(){return isUniqueLocal;} |
||||
void sethost(std::string& host_){host=host_;} |
||||
void setport(int port_){port=port_;} |
||||
void setkeys(std::string& keys_){keys=keys_;} |
||||
void setinPort(int inPort_){inPort=inPort_;} |
||||
void setaccessList(std::string& accessList_){accessList=accessList_;} |
||||
void sethostOverride(std::string& hostOverride_){hostOverride=hostOverride_;} |
||||
void setwebircpass(std::string& webircpass_){webircpass=webircpass_;} |
||||
void setgzip(bool gzip_){gzip=gzip_;} |
||||
void setsigType(i2p::data::SigningKeyType sigType_){sigType=sigType_;} |
||||
void setmaxConns(uint32_t maxConns_){maxConns=maxConns_;} |
||||
void setaddress(std::string& address_){address=address_;} |
||||
void setisUniqueLocal(bool isUniqueLocal_){isUniqueLocal=isUniqueLocal_;} |
||||
virtual ClientTunnelConfig* asClientTunnelConfig(){return nullptr;} |
||||
virtual ServerTunnelConfig* asServerTunnelConfig(){return this;} |
||||
}; |
||||
|
||||
|
||||
#endif // TUNNELCONFIG_H
|
@ -0,0 +1,149 @@
@@ -0,0 +1,149 @@
|
||||
#include "TunnelPane.h" |
||||
|
||||
TunnelPane::TunnelPane(): QObject(),gridLayoutWidget_2(nullptr) { |
||||
} |
||||
|
||||
void TunnelPane::setupTunnelPane( |
||||
TunnelConfig* tunnelConfig, |
||||
QGroupBox *tunnelGroupBox, |
||||
QWidget* gridLayoutWidget_2, QComboBox * tunnelTypeComboBox, |
||||
QWidget */*tunnelsFormGridLayoutWidget*/, QGridLayout */*tunnelsFormGridLayout*/) { |
||||
this->tunnelGroupBox=tunnelGroupBox; |
||||
gridLayoutWidget_2->setObjectName(QStringLiteral("gridLayoutWidget_2")); |
||||
this->gridLayoutWidget_2=gridLayoutWidget_2; |
||||
tunnelGridLayout = new QGridLayout(gridLayoutWidget_2); |
||||
tunnelGridLayout->setObjectName(QStringLiteral("tunnelGridLayout")); |
||||
tunnelGridLayout->setContentsMargins(5, 5, 5, 5); |
||||
tunnelGridLayout->setVerticalSpacing(5); |
||||
|
||||
//header
|
||||
QHBoxLayout *headerHorizontalLayout = new QHBoxLayout(); |
||||
headerHorizontalLayout->setObjectName(QStringLiteral("headerHorizontalLayout")); |
||||
|
||||
nameLabel = new QLabel(gridLayoutWidget_2); |
||||
nameLabel->setObjectName(QStringLiteral("nameLabel")); |
||||
headerHorizontalLayout->addWidget(nameLabel); |
||||
nameLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
nameLineEdit->setObjectName(QStringLiteral("nameLineEdit")); |
||||
const QString& tunnelName=tunnelConfig->getName().c_str(); |
||||
nameLineEdit->setText(tunnelName); |
||||
setGroupBoxTitle(tunnelName); |
||||
|
||||
QObject::connect(nameLineEdit, SIGNAL(textChanged(const QString &)), |
||||
this, SLOT(setGroupBoxTitle(const QString &))); |
||||
|
||||
headerHorizontalLayout->addWidget(nameLineEdit); |
||||
headerHorizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
headerHorizontalLayout->addItem(headerHorizontalSpacer); |
||||
deletePushButton = new QPushButton(gridLayoutWidget_2);//TODO handle it
|
||||
deletePushButton->setObjectName(QStringLiteral("deletePushButton")); |
||||
headerHorizontalLayout->addWidget(deletePushButton); |
||||
tunnelGridLayout->addLayout(headerHorizontalLayout, 0, 0, 1, 1); |
||||
|
||||
//type
|
||||
{ |
||||
const QString& type = tunnelConfig->getType(); |
||||
QHBoxLayout * horizontalLayout_ = new QHBoxLayout(); |
||||
horizontalLayout_->setObjectName(QStringLiteral("horizontalLayout_")); |
||||
typeLabel = new QLabel(gridLayoutWidget_2); |
||||
typeLabel->setObjectName(QStringLiteral("typeLabel")); |
||||
horizontalLayout_->addWidget(typeLabel); |
||||
horizontalLayout_->addWidget(tunnelTypeComboBox); |
||||
this->tunnelTypeComboBox=tunnelTypeComboBox; |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_, 1, 0, 1, 1); |
||||
} |
||||
|
||||
retranslateTunnelForm(*this); |
||||
} |
||||
|
||||
void TunnelPane::appendControlsForI2CPParameters(I2CPParameters& i2cpParameters, int& gridIndex) { |
||||
{ |
||||
//number of hops of an inbound tunnel
|
||||
const QString& inbound_length=i2cpParameters.getInbound_length(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
inbound_lengthLabel = new QLabel(gridLayoutWidget_2); |
||||
inbound_lengthLabel->setObjectName(QStringLiteral("inbound_lengthLabel")); |
||||
horizontalLayout_2->addWidget(inbound_lengthLabel); |
||||
inbound_lengthLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
inbound_lengthLineEdit->setObjectName(QStringLiteral("inbound_lengthLineEdit")); |
||||
inbound_lengthLineEdit->setText(inbound_length); |
||||
inbound_lengthLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(inbound_lengthLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
//number of hops of an outbound tunnel
|
||||
const QString& outbound_length=i2cpParameters.getOutbound_length(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
outbound_lengthLabel = new QLabel(gridLayoutWidget_2); |
||||
outbound_lengthLabel->setObjectName(QStringLiteral("outbound_lengthLabel")); |
||||
horizontalLayout_2->addWidget(outbound_lengthLabel); |
||||
outbound_lengthLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
outbound_lengthLineEdit->setObjectName(QStringLiteral("outbound_lengthLineEdit")); |
||||
outbound_lengthLineEdit->setText(outbound_length); |
||||
outbound_lengthLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(outbound_lengthLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
//number of inbound tunnels
|
||||
const QString& inbound_quantity=i2cpParameters.getInbound_quantity(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
inbound_quantityLabel = new QLabel(gridLayoutWidget_2); |
||||
inbound_quantityLabel->setObjectName(QStringLiteral("inbound_quantityLabel")); |
||||
horizontalLayout_2->addWidget(inbound_quantityLabel); |
||||
inbound_quantityLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
inbound_quantityLineEdit->setObjectName(QStringLiteral("inbound_quantityLineEdit")); |
||||
inbound_quantityLineEdit->setText(inbound_quantity); |
||||
inbound_quantityLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(inbound_quantityLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
//number of outbound tunnels
|
||||
const QString& outbound_quantity=i2cpParameters.getOutbound_quantity(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
outbound_quantityLabel = new QLabel(gridLayoutWidget_2); |
||||
outbound_quantityLabel->setObjectName(QStringLiteral("outbound_quantityLabel")); |
||||
horizontalLayout_2->addWidget(outbound_quantityLabel); |
||||
outbound_quantityLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
outbound_quantityLineEdit->setObjectName(QStringLiteral("outbound_quantityLineEdit")); |
||||
outbound_quantityLineEdit->setText(outbound_quantity); |
||||
outbound_quantityLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(outbound_quantityLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
{ |
||||
//number of ElGamal/AES tags to send
|
||||
const QString& crypto_tagsToSend=i2cpParameters.getCrypto_tagsToSend(); |
||||
QHBoxLayout *horizontalLayout_2 = new QHBoxLayout(); |
||||
horizontalLayout_2->setObjectName(QStringLiteral("horizontalLayout_2")); |
||||
crypto_tagsToSendLabel = new QLabel(gridLayoutWidget_2); |
||||
crypto_tagsToSendLabel->setObjectName(QStringLiteral("crypto_tagsToSendLabel")); |
||||
horizontalLayout_2->addWidget(crypto_tagsToSendLabel); |
||||
crypto_tagsToSendLineEdit = new QLineEdit(gridLayoutWidget_2); |
||||
crypto_tagsToSendLineEdit->setObjectName(QStringLiteral("crypto_tagsToSendLineEdit")); |
||||
crypto_tagsToSendLineEdit->setText(crypto_tagsToSend); |
||||
crypto_tagsToSendLineEdit->setMaximumWidth(80); |
||||
horizontalLayout_2->addWidget(crypto_tagsToSendLineEdit); |
||||
QSpacerItem * horizontalSpacer = new QSpacerItem(40, 20, QSizePolicy::Expanding, QSizePolicy::Minimum); |
||||
horizontalLayout_2->addItem(horizontalSpacer); |
||||
tunnelGridLayout->addLayout(horizontalLayout_2, ++gridIndex, 0, 1, 1); |
||||
} |
||||
|
||||
retranslateI2CPParameters(); |
||||
} |
@ -0,0 +1,94 @@
@@ -0,0 +1,94 @@
|
||||
#ifndef TUNNELPANE_H |
||||
#define TUNNELPANE_H |
||||
|
||||
#include "QObject" |
||||
#include "QWidget" |
||||
#include "QComboBox" |
||||
#include "QGridLayout" |
||||
#include "QLabel" |
||||
#include "QPushButton" |
||||
#include "QApplication" |
||||
#include "QLineEdit" |
||||
#include "QGroupBox" |
||||
|
||||
#include "TunnelConfig.h" |
||||
|
||||
class ServerTunnelPane; |
||||
class ClientTunnelPane; |
||||
|
||||
class TunnelConfig; |
||||
class I2CPParameters; |
||||
|
||||
class TunnelPane : public QObject { |
||||
|
||||
Q_OBJECT |
||||
|
||||
public: |
||||
TunnelPane(); |
||||
virtual ~TunnelPane(){} |
||||
|
||||
virtual ServerTunnelPane* asServerTunnelPane()=0; |
||||
virtual ClientTunnelPane* asClientTunnelPane()=0; |
||||
|
||||
protected: |
||||
QGridLayout *tunnelGridLayout; |
||||
QGroupBox *tunnelGroupBox; |
||||
QWidget* gridLayoutWidget_2; |
||||
|
||||
//header
|
||||
QLabel *nameLabel; |
||||
QLineEdit *nameLineEdit; |
||||
QSpacerItem *headerHorizontalSpacer; |
||||
QPushButton *deletePushButton; |
||||
|
||||
//type
|
||||
QComboBox *tunnelTypeComboBox; |
||||
QLabel *typeLabel; |
||||
|
||||
//i2cp
|
||||
|
||||
QLabel * inbound_lengthLabel; |
||||
QLineEdit * inbound_lengthLineEdit; |
||||
|
||||
QLabel * outbound_lengthLabel; |
||||
QLineEdit * outbound_lengthLineEdit; |
||||
|
||||
QLabel * inbound_quantityLabel; |
||||
QLineEdit * inbound_quantityLineEdit; |
||||
|
||||
QLabel * outbound_quantityLabel; |
||||
QLineEdit * outbound_quantityLineEdit; |
||||
|
||||
QLabel * crypto_tagsToSendLabel; |
||||
QLineEdit * crypto_tagsToSendLineEdit; |
||||
|
||||
void setupTunnelPane( |
||||
TunnelConfig* tunnelConfig, |
||||
QGroupBox *tunnelGroupBox, |
||||
QWidget* gridLayoutWidget_2, QComboBox * tunnelTypeComboBox, |
||||
QWidget *tunnelsFormGridLayoutWidget, QGridLayout *tunnelsFormGridLayout); |
||||
void appendControlsForI2CPParameters(I2CPParameters& i2cpParameters, int& gridIndex); |
||||
public: |
||||
int height() { |
||||
return gridLayoutWidget_2?gridLayoutWidget_2->height():0; |
||||
} |
||||
|
||||
protected slots: |
||||
virtual void setGroupBoxTitle(const QString & title)=0; |
||||
private: |
||||
void retranslateTunnelForm(TunnelPane& ui) { |
||||
ui.deletePushButton->setText(QApplication::translate("tunForm", "Delete Tunnel", 0)); |
||||
ui.nameLabel->setText(QApplication::translate("tunForm", "Tunnel name:", 0)); |
||||
ui.typeLabel->setText(QApplication::translate("tunForm", "Server tunnel type:", 0)); |
||||
} |
||||
|
||||
void retranslateI2CPParameters() { |
||||
inbound_lengthLabel->setText(QApplication::translate("tunForm", "Number of hops of an inbound tunnel:", 0));; |
||||
outbound_lengthLabel->setText(QApplication::translate("tunForm", "Number of hops of an outbound tunnel:", 0));; |
||||
inbound_quantityLabel->setText(QApplication::translate("tunForm", "Number of inbound tunnels:", 0));; |
||||
outbound_quantityLabel->setText(QApplication::translate("tunForm", "Number of outbound tunnels:", 0));; |
||||
crypto_tagsToSendLabel->setText(QApplication::translate("tunForm", "Number of ElGamal/AES tags to send:", 0));; |
||||
} |
||||
}; |
||||
|
||||
#endif // TUNNELPANE_H
|
File diff suppressed because it is too large
Load Diff
File diff suppressed because it is too large
Load Diff
File diff suppressed because it is too large
Load Diff
@ -0,0 +1,104 @@
@@ -0,0 +1,104 @@
|
||||
<?xml version="1.0" encoding="UTF-8"?> |
||||
<ui version="4.0"> |
||||
<class>Form</class> |
||||
<widget class="QWidget" name="Form"> |
||||
<property name="geometry"> |
||||
<rect> |
||||
<x>0</x> |
||||
<y>0</y> |
||||
<width>527</width> |
||||
<height>452</height> |
||||
</rect> |
||||
</property> |
||||
<property name="windowTitle"> |
||||
<string>Form</string> |
||||
</property> |
||||
<widget class="QWidget" name="gridLayoutWidget"> |
||||
<property name="geometry"> |
||||
<rect> |
||||
<x>0</x> |
||||
<y>0</y> |
||||
<width>521</width> |
||||
<height>451</height> |
||||
</rect> |
||||
</property> |
||||
<layout class="QGridLayout" name="formGridLayout"> |
||||
<item row="0" column="0"> |
||||
<widget class="QGroupBox" name="serverTunnelNameGroupBox"> |
||||
<property name="title"> |
||||
<string>server_tunnel_name</string> |
||||
</property> |
||||
<widget class="QWidget" name="gridLayoutWidget_2"> |
||||
<property name="geometry"> |
||||
<rect> |
||||
<x>0</x> |
||||
<y>20</y> |
||||
<width>511</width> |
||||
<height>421</height> |
||||
</rect> |
||||
</property> |
||||
<layout class="QGridLayout" name="tunnelGridLayout"> |
||||
<item row="0" column="0"> |
||||
<layout class="QHBoxLayout" name="headerHorizontalLayout"> |
||||
<item> |
||||
<widget class="QComboBox" name="tunnelTypeComboBox"/> |
||||
</item> |
||||
<item> |
||||
<spacer name="headerHorizontalSpacer"> |
||||
<property name="orientation"> |
||||
<enum>Qt::Horizontal</enum> |
||||
</property> |
||||
<property name="sizeHint" stdset="0"> |
||||
<size> |
||||
<width>40</width> |
||||
<height>20</height> |
||||
</size> |
||||
</property> |
||||
</spacer> |
||||
</item> |
||||
<item> |
||||
<widget class="QPushButton" name="deletePushButton"> |
||||
<property name="text"> |
||||
<string>Delete</string> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
</layout> |
||||
</item> |
||||
<item row="1" column="0"> |
||||
<layout class="QHBoxLayout" name="horizontalLayout_2"> |
||||
<item> |
||||
<widget class="QLabel" name="hostLabel"> |
||||
<property name="text"> |
||||
<string>Host:</string> |
||||
</property> |
||||
</widget> |
||||
</item> |
||||
<item> |
||||
<widget class="QLineEdit" name="hostLineEdit"/> |
||||
</item> |
||||
<item> |
||||
<spacer name="hostHorizontalSpacer"> |
||||
<property name="orientation"> |
||||
<enum>Qt::Horizontal</enum> |
||||
</property> |
||||
<property name="sizeHint" stdset="0"> |
||||
<size> |
||||
<width>40</width> |
||||
<height>20</height> |
||||
</size> |
||||
</property> |
||||
</spacer> |
||||
</item> |
||||
</layout> |
||||
</item> |
||||
</layout> |
||||
</widget> |
||||
</widget> |
||||
</item> |
||||
</layout> |
||||
</widget> |
||||
</widget> |
||||
<resources/> |
||||
<connections/> |
||||
</ui> |
Loading…
Reference in new issue