mirror of https://github.com/GOSTSec/sgminer
Browse Source
- Fixed Lyra2REv2, Neoscrypt & WhirlpoolX algo - Changed default algo from scrypt to x11windows^2
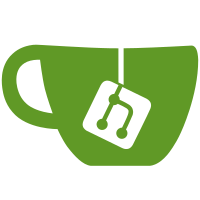
35 changed files with 2630 additions and 687 deletions
@ -1,10 +0,0 @@
@@ -1,10 +0,0 @@
|
||||
#ifndef LYRA2REOLD_H |
||||
#define LYRA2REOLD_H |
||||
|
||||
#include "miner.h" |
||||
|
||||
extern int lyra2reold_test(unsigned char *pdata, const unsigned char *ptarget, |
||||
uint32_t nonce); |
||||
extern void lyra2reold_regenhash(struct work *work); |
||||
|
||||
#endif /* LYRA2RE_H */ |
@ -0,0 +1,11 @@
@@ -0,0 +1,11 @@
|
||||
#ifndef LYRA2REV2_H |
||||
#define LYRA2REV2_H |
||||
|
||||
#include "miner.h" |
||||
#define LYRA_SCRATCHBUF_SIZE (1536) // matrix size [12][4][4] uint64_t or equivalent
|
||||
#define LYRA_SECBUF_SIZE (4) // (not used)
|
||||
extern int lyra2rev2_test(unsigned char *pdata, const unsigned char *ptarget, |
||||
uint32_t nonce); |
||||
extern void lyra2rev2_regenhash(struct work *work); |
||||
|
||||
#endif /* LYRA2REV2_H */ |
@ -0,0 +1,213 @@
@@ -0,0 +1,213 @@
|
||||
/**
|
||||
* Implementation of the Lyra2 Password Hashing Scheme (PHS). |
||||
* |
||||
* Author: The Lyra PHC team (http://www.lyra-kdf.net/) -- 2014.
|
||||
* |
||||
* This software is hereby placed in the public domain. |
||||
* |
||||
* THIS SOFTWARE IS PROVIDED BY THE AUTHORS ''AS IS'' AND ANY EXPRESS |
||||
* OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED |
||||
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE |
||||
* ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHORS OR CONTRIBUTORS BE |
||||
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR |
||||
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF |
||||
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR |
||||
* BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, |
||||
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE |
||||
* OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, |
||||
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
||||
*/ |
||||
#include <stdio.h> |
||||
#include <stdlib.h> |
||||
#include <string.h> |
||||
#include <time.h> |
||||
#include "lyra2v2.h" |
||||
#include "spongev2.h" |
||||
|
||||
/**
|
||||
* Executes Lyra2 based on the G function from Blake2b. This version supports salts and passwords |
||||
* whose combined length is smaller than the size of the memory matrix, (i.e., (nRows x nCols x b) bits, |
||||
* where "b" is the underlying sponge's bitrate). In this implementation, the "basil" is composed by all |
||||
* integer parameters (treated as type "unsigned int") in the order they are provided, plus the value |
||||
* of nCols, (i.e., basil = kLen || pwdlen || saltlen || timeCost || nRows || nCols). |
||||
* |
||||
* @param K The derived key to be output by the algorithm |
||||
* @param kLen Desired key length |
||||
* @param pwd User password |
||||
* @param pwdlen Password length |
||||
* @param salt Salt |
||||
* @param saltlen Salt length |
||||
* @param timeCost Parameter to determine the processing time (T) |
||||
* @param nRows Number or rows of the memory matrix (R) |
||||
* @param nCols Number of columns of the memory matrix (C) |
||||
* |
||||
* @return 0 if the key is generated correctly; -1 if there is an error (usually due to lack of memory for allocation) |
||||
*/ |
||||
int LYRA2V2(void *K, uint64_t kLen, const void *pwd, uint64_t pwdlen, const void *salt, uint64_t saltlen, uint64_t timeCost, uint64_t nRows, uint64_t nCols) { |
||||
|
||||
//============================= Basic variables ============================//
|
||||
int64_t row = 2; //index of row to be processed
|
||||
int64_t prev = 1; //index of prev (last row ever computed/modified)
|
||||
int64_t rowa = 0; //index of row* (a previous row, deterministically picked during Setup and randomly picked while Wandering)
|
||||
int64_t tau; //Time Loop iterator
|
||||
int64_t step = 1; //Visitation step (used during Setup and Wandering phases)
|
||||
int64_t window = 2; //Visitation window (used to define which rows can be revisited during Setup)
|
||||
int64_t gap = 1; //Modifier to the step, assuming the values 1 or -1
|
||||
int64_t i; //auxiliary iteration counter
|
||||
//==========================================================================/
|
||||
|
||||
//========== Initializing the Memory Matrix and pointers to it =============//
|
||||
//Tries to allocate enough space for the whole memory matrix
|
||||
|
||||
|
||||
const int64_t ROW_LEN_INT64 = BLOCK_LEN_INT64 * nCols; |
||||
const int64_t ROW_LEN_BYTES = ROW_LEN_INT64 * 8; |
||||
|
||||
i = (int64_t) ((int64_t) nRows * (int64_t) ROW_LEN_BYTES); |
||||
uint64_t *wholeMatrix = (uint64_t*) malloc(i); |
||||
if (wholeMatrix == NULL) { |
||||
return -1; |
||||
} |
||||
memset(wholeMatrix, 0, i); |
||||
|
||||
//Allocates pointers to each row of the matrix
|
||||
uint64_t **memMatrix = (uint64_t**) malloc(nRows * sizeof(uint64_t*)); |
||||
if (memMatrix == NULL) { |
||||
return -1; |
||||
} |
||||
//Places the pointers in the correct positions
|
||||
uint64_t *ptrWord = wholeMatrix; |
||||
for (i = 0; i < nRows; i++) { |
||||
memMatrix[i] = ptrWord; |
||||
ptrWord += ROW_LEN_INT64; |
||||
} |
||||
//==========================================================================/
|
||||
|
||||
//============= Getting the password + salt + basil padded with 10*1 ===============//
|
||||
//OBS.:The memory matrix will temporarily hold the password: not for saving memory,
|
||||
//but this ensures that the password copied locally will be overwritten as soon as possible
|
||||
|
||||
//First, we clean enough blocks for the password, salt, basil and padding
|
||||
uint64_t nBlocksInput = ((saltlen + pwdlen + 6 * sizeof (uint64_t)) / BLOCK_LEN_BLAKE2_SAFE_BYTES) + 1; |
||||
byte *ptrByte = (byte*) wholeMatrix; |
||||
memset(ptrByte, 0, nBlocksInput * BLOCK_LEN_BLAKE2_SAFE_BYTES); |
||||
|
||||
//Prepends the password
|
||||
memcpy(ptrByte, pwd, pwdlen); |
||||
ptrByte += pwdlen; |
||||
|
||||
//Concatenates the salt
|
||||
memcpy(ptrByte, salt, saltlen); |
||||
ptrByte += saltlen; |
||||
|
||||
//Concatenates the basil: every integer passed as parameter, in the order they are provided by the interface
|
||||
memcpy(ptrByte, &kLen, sizeof (uint64_t)); |
||||
ptrByte += sizeof (uint64_t); |
||||
memcpy(ptrByte, &pwdlen, sizeof (uint64_t)); |
||||
ptrByte += sizeof (uint64_t); |
||||
memcpy(ptrByte, &saltlen, sizeof (uint64_t)); |
||||
ptrByte += sizeof (uint64_t); |
||||
memcpy(ptrByte, &timeCost, sizeof (uint64_t)); |
||||
ptrByte += sizeof (uint64_t); |
||||
memcpy(ptrByte, &nRows, sizeof (uint64_t)); |
||||
ptrByte += sizeof (uint64_t); |
||||
memcpy(ptrByte, &nCols, sizeof (uint64_t)); |
||||
ptrByte += sizeof (uint64_t); |
||||
|
||||
//Now comes the padding
|
||||
*ptrByte = 0x80; //first byte of padding: right after the password
|
||||
ptrByte = (byte*) wholeMatrix; //resets the pointer to the start of the memory matrix
|
||||
ptrByte += nBlocksInput * BLOCK_LEN_BLAKE2_SAFE_BYTES - 1; //sets the pointer to the correct position: end of incomplete block
|
||||
*ptrByte ^= 0x01; //last byte of padding: at the end of the last incomplete block
|
||||
//==========================================================================/
|
||||
|
||||
//======================= Initializing the Sponge State ====================//
|
||||
//Sponge state: 16 uint64_t, BLOCK_LEN_INT64 words of them for the bitrate (b) and the remainder for the capacity (c)
|
||||
uint64_t *state = (uint64_t*) malloc(16 * sizeof(uint64_t)); |
||||
if (state == NULL) { |
||||
return -1; |
||||
} |
||||
initStatev2(state); |
||||
//==========================================================================/
|
||||
|
||||
//================================ Setup Phase =============================//
|
||||
//Absorbing salt, password and basil: this is the only place in which the block length is hard-coded to 512 bits
|
||||
ptrWord = wholeMatrix; |
||||
for (i = 0; i < nBlocksInput; i++) { |
||||
absorbBlockBlake2Safev2(state, ptrWord); //absorbs each block of pad(pwd || salt || basil)
|
||||
ptrWord += BLOCK_LEN_BLAKE2_SAFE_INT64; //goes to next block of pad(pwd || salt || basil)
|
||||
} |
||||
|
||||
//Initializes M[0] and M[1]
|
||||
reducedSqueezeRow0v2(state, memMatrix[0], nCols); //The locally copied password is most likely overwritten here
|
||||
reducedDuplexRow1v2(state, memMatrix[0], memMatrix[1], nCols); |
||||
|
||||
do { |
||||
//M[row] = rand; //M[row*] = M[row*] XOR rotW(rand)
|
||||
reducedDuplexRowSetupv2(state, memMatrix[prev], memMatrix[rowa], memMatrix[row], nCols); |
||||
|
||||
|
||||
//updates the value of row* (deterministically picked during Setup))
|
||||
rowa = (rowa + step) & (window - 1); |
||||
//update prev: it now points to the last row ever computed
|
||||
prev = row; |
||||
//updates row: goes to the next row to be computed
|
||||
row++; |
||||
|
||||
//Checks if all rows in the window where visited.
|
||||
if (rowa == 0) { |
||||
step = window + gap; //changes the step: approximately doubles its value
|
||||
window *= 2; //doubles the size of the re-visitation window
|
||||
gap = -gap; //inverts the modifier to the step
|
||||
} |
||||
|
||||
} while (row < nRows); |
||||
//==========================================================================/
|
||||
|
||||
//============================ Wandering Phase =============================//
|
||||
row = 0; //Resets the visitation to the first row of the memory matrix
|
||||
for (tau = 1; tau <= timeCost; tau++) { |
||||
//Step is approximately half the number of all rows of the memory matrix for an odd tau; otherwise, it is -1
|
||||
step = (tau % 2 == 0) ? -1 : nRows / 2 - 1; |
||||
do { |
||||
//Selects a pseudorandom index row*
|
||||
//------------------------------------------------------------------------------------------
|
||||
//rowa = ((unsigned int)state[0]) & (nRows-1); //(USE THIS IF nRows IS A POWER OF 2)
|
||||
rowa = ((uint64_t) (state[0])) % nRows; //(USE THIS FOR THE "GENERIC" CASE)
|
||||
//------------------------------------------------------------------------------------------
|
||||
|
||||
//Performs a reduced-round duplexing operation over M[row*] XOR M[prev], updating both M[row*] and M[row]
|
||||
reducedDuplexRowv2(state, memMatrix[prev], memMatrix[rowa], memMatrix[row], nCols); |
||||
|
||||
//update prev: it now points to the last row ever computed
|
||||
prev = row; |
||||
|
||||
//updates row: goes to the next row to be computed
|
||||
//------------------------------------------------------------------------------------------
|
||||
//row = (row + step) & (nRows-1); //(USE THIS IF nRows IS A POWER OF 2)
|
||||
row = (row + step) % nRows; //(USE THIS FOR THE "GENERIC" CASE)
|
||||
//------------------------------------------------------------------------------------------
|
||||
|
||||
} while (row != 0); |
||||
} |
||||
//==========================================================================/
|
||||
|
||||
//============================ Wrap-up Phase ===============================//
|
||||
//Absorbs the last block of the memory matrix
|
||||
absorbBlockv2(state, memMatrix[rowa]); |
||||
|
||||
//Squeezes the key
|
||||
squeezev2(state, (unsigned char*)K, kLen); |
||||
//==========================================================================/
|
||||
|
||||
//========================= Freeing the memory =============================//
|
||||
free(memMatrix); |
||||
free(wholeMatrix); |
||||
|
||||
//Wiping out the sponge's internal state before freeing it
|
||||
memset(state, 0, 16 * sizeof (uint64_t)); |
||||
free(state); |
||||
//==========================================================================/
|
||||
|
||||
return 0; |
||||
} |
@ -0,0 +1,42 @@
@@ -0,0 +1,42 @@
|
||||
/**
|
||||
* Header file for the Lyra2 Password Hashing Scheme (PHS). |
||||
* |
||||
* Author: The Lyra PHC team (http://www.lyra-kdf.net/) -- 2014.
|
||||
* |
||||
* This software is hereby placed in the public domain. |
||||
* |
||||
* THIS SOFTWARE IS PROVIDED BY THE AUTHORS ''AS IS'' AND ANY EXPRESS |
||||
* OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED |
||||
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE |
||||
* ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHORS OR CONTRIBUTORS BE |
||||
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR |
||||
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF |
||||
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR |
||||
* BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, |
||||
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE |
||||
* OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, |
||||
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
||||
*/ |
||||
#ifndef LYRA2V2_H_ |
||||
#define LYRA2V2_H_ |
||||
|
||||
#include <stdint.h> |
||||
|
||||
typedef unsigned char byte; |
||||
|
||||
//Block length required so Blake2's Initialization Vector (IV) is not overwritten (THIS SHOULD NOT BE MODIFIED)
|
||||
#define BLOCK_LEN_BLAKE2_SAFE_INT64 8 //512 bits (=64 bytes, =8 uint64_t)
|
||||
#define BLOCK_LEN_BLAKE2_SAFE_BYTES (BLOCK_LEN_BLAKE2_SAFE_INT64 * 8) //same as above, in bytes
|
||||
|
||||
|
||||
#ifdef BLOCK_LEN_BITS |
||||
#define BLOCK_LEN_INT64 (BLOCK_LEN_BITS/64) //Block length: 768 bits (=96 bytes, =12 uint64_t)
|
||||
#define BLOCK_LEN_BYTES (BLOCK_LEN_BITS/8) //Block length, in bytes
|
||||
#else //default block lenght: 768 bits
|
||||
#define BLOCK_LEN_INT64 12 //Block length: 768 bits (=96 bytes, =12 uint64_t)
|
||||
#define BLOCK_LEN_BYTES (BLOCK_LEN_INT64 * 8) //Block length, in bytes
|
||||
#endif |
||||
|
||||
int LYRA2V2(void *K, uint64_t kLen, const void *pwd, uint64_t pwdlen, const void *salt, uint64_t saltlen, uint64_t timeCost, uint64_t nRows, uint64_t nCols); |
||||
|
||||
#endif /* LYRA2_H_ */ |
@ -0,0 +1,745 @@
@@ -0,0 +1,745 @@
|
||||
/**
|
||||
* A simple implementation of Blake2b's internal permutation |
||||
* in the form of a sponge. |
||||
* |
||||
* Author: The Lyra PHC team (http://www.lyra-kdf.net/) -- 2014.
|
||||
* |
||||
* This software is hereby placed in the public domain. |
||||
* |
||||
* THIS SOFTWARE IS PROVIDED BY THE AUTHORS ''AS IS'' AND ANY EXPRESS |
||||
* OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED |
||||
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE |
||||
* ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHORS OR CONTRIBUTORS BE |
||||
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR |
||||
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF |
||||
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR |
||||
* BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, |
||||
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE |
||||
* OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, |
||||
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
||||
*/ |
||||
#include <string.h> |
||||
#include <stdio.h> |
||||
#include <time.h> |
||||
#include "spongev2.h" |
||||
#include "lyra2v2.h" |
||||
|
||||
|
||||
|
||||
/**
|
||||
* Initializes the Sponge State. The first 512 bits are set to zeros and the remainder |
||||
* receive Blake2b's IV as per Blake2b's specification. <b>Note:</b> Even though sponges |
||||
* typically have their internal state initialized with zeros, Blake2b's G function |
||||
* has a fixed point: if the internal state and message are both filled with zeros. the |
||||
* resulting permutation will always be a block filled with zeros; this happens because |
||||
* Blake2b does not use the constants originally employed in Blake2 inside its G function, |
||||
* relying on the IV for avoiding possible fixed points. |
||||
* |
||||
* @param state The 1024-bit array to be initialized |
||||
*/ |
||||
inline void initStatev2(uint64_t state[/*16*/]) { |
||||
//First 512 bis are zeros
|
||||
memset(state, 0, 64); |
||||
//Remainder BLOCK_LEN_BLAKE2_SAFE_BYTES are reserved to the IV
|
||||
state[8] = blake2b_IV[0]; |
||||
state[9] = blake2b_IV[1]; |
||||
state[10] = blake2b_IV[2]; |
||||
state[11] = blake2b_IV[3]; |
||||
state[12] = blake2b_IV[4]; |
||||
state[13] = blake2b_IV[5]; |
||||
state[14] = blake2b_IV[6]; |
||||
state[15] = blake2b_IV[7]; |
||||
} |
||||
|
||||
/**
|
||||
* Execute Blake2b's G function, with all 12 rounds. |
||||
* |
||||
* @param v A 1024-bit (16 uint64_t) array to be processed by Blake2b's G function |
||||
*/ |
||||
inline static void blake2bLyra(uint64_t *v) { |
||||
ROUND_LYRA(0); |
||||
ROUND_LYRA(1); |
||||
ROUND_LYRA(2); |
||||
ROUND_LYRA(3); |
||||
ROUND_LYRA(4); |
||||
ROUND_LYRA(5); |
||||
ROUND_LYRA(6); |
||||
ROUND_LYRA(7); |
||||
ROUND_LYRA(8); |
||||
ROUND_LYRA(9); |
||||
ROUND_LYRA(10); |
||||
ROUND_LYRA(11); |
||||
} |
||||
|
||||
/**
|
||||
* Executes a reduced version of Blake2b's G function with only one round |
||||
* @param v A 1024-bit (16 uint64_t) array to be processed by Blake2b's G function |
||||
*/ |
||||
inline static void reducedBlake2bLyra(uint64_t *v) { |
||||
ROUND_LYRA(0); |
||||
} |
||||
|
||||
/**
|
||||
* Performs a squeeze operation, using Blake2b's G function as the |
||||
* internal permutation |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param out Array that will receive the data squeezed |
||||
* @param len The number of bytes to be squeezed into the "out" array |
||||
*/ |
||||
inline void squeezev2(uint64_t *state, byte *out, unsigned int len) { |
||||
int fullBlocks = len / BLOCK_LEN_BYTES; |
||||
byte *ptr = out; |
||||
int i; |
||||
//Squeezes full blocks
|
||||
for (i = 0; i < fullBlocks; i++) { |
||||
memcpy(ptr, state, BLOCK_LEN_BYTES); |
||||
blake2bLyra(state); |
||||
ptr += BLOCK_LEN_BYTES; |
||||
} |
||||
|
||||
//Squeezes remaining bytes
|
||||
memcpy(ptr, state, (len % BLOCK_LEN_BYTES)); |
||||
} |
||||
|
||||
/**
|
||||
* Performs an absorb operation for a single block (BLOCK_LEN_INT64 words |
||||
* of type uint64_t), using Blake2b's G function as the internal permutation |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param in The block to be absorbed (BLOCK_LEN_INT64 words) |
||||
*/ |
||||
inline void absorbBlockv2(uint64_t *state, const uint64_t *in) { |
||||
//XORs the first BLOCK_LEN_INT64 words of "in" with the current state
|
||||
state[0] ^= in[0]; |
||||
state[1] ^= in[1]; |
||||
state[2] ^= in[2]; |
||||
state[3] ^= in[3]; |
||||
state[4] ^= in[4]; |
||||
state[5] ^= in[5]; |
||||
state[6] ^= in[6]; |
||||
state[7] ^= in[7]; |
||||
state[8] ^= in[8]; |
||||
state[9] ^= in[9]; |
||||
state[10] ^= in[10]; |
||||
state[11] ^= in[11]; |
||||
|
||||
//Applies the transformation f to the sponge's state
|
||||
blake2bLyra(state); |
||||
} |
||||
|
||||
/**
|
||||
* Performs an absorb operation for a single block (BLOCK_LEN_BLAKE2_SAFE_INT64 |
||||
* words of type uint64_t), using Blake2b's G function as the internal permutation |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param in The block to be absorbed (BLOCK_LEN_BLAKE2_SAFE_INT64 words) |
||||
*/ |
||||
inline void absorbBlockBlake2Safev2(uint64_t *state, const uint64_t *in) { |
||||
//XORs the first BLOCK_LEN_BLAKE2_SAFE_INT64 words of "in" with the current state
|
||||
|
||||
state[0] ^= in[0]; |
||||
state[1] ^= in[1]; |
||||
state[2] ^= in[2]; |
||||
state[3] ^= in[3]; |
||||
state[4] ^= in[4]; |
||||
state[5] ^= in[5]; |
||||
state[6] ^= in[6]; |
||||
state[7] ^= in[7]; |
||||
|
||||
|
||||
//Applies the transformation f to the sponge's state
|
||||
blake2bLyra(state); |
||||
|
||||
} |
||||
|
||||
/**
|
||||
* Performs a reduced squeeze operation for a single row, from the highest to |
||||
* the lowest index, using the reduced-round Blake2b's G function as the |
||||
* internal permutation |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowOut Row to receive the data squeezed |
||||
*/ |
||||
inline void reducedSqueezeRow0v2(uint64_t* state, uint64_t* rowOut, uint64_t nCols) { |
||||
uint64_t* ptrWord = rowOut + (nCols-1)*BLOCK_LEN_INT64; //In Lyra2: pointer to M[0][C-1]
|
||||
int i; |
||||
//M[row][C-1-col] = H.reduced_squeeze()
|
||||
for (i = 0; i < nCols; i++) { |
||||
ptrWord[0] = state[0]; |
||||
ptrWord[1] = state[1]; |
||||
ptrWord[2] = state[2]; |
||||
ptrWord[3] = state[3]; |
||||
ptrWord[4] = state[4]; |
||||
ptrWord[5] = state[5]; |
||||
ptrWord[6] = state[6]; |
||||
ptrWord[7] = state[7]; |
||||
ptrWord[8] = state[8]; |
||||
ptrWord[9] = state[9]; |
||||
ptrWord[10] = state[10]; |
||||
ptrWord[11] = state[11]; |
||||
|
||||
//Goes to next block (column) that will receive the squeezed data
|
||||
ptrWord -= BLOCK_LEN_INT64; |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
} |
||||
} |
||||
|
||||
/**
|
||||
* Performs a reduced duplex operation for a single row, from the highest to |
||||
* the lowest index, using the reduced-round Blake2b's G function as the |
||||
* internal permutation |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowIn Row to feed the sponge |
||||
* @param rowOut Row to receive the sponge's output |
||||
*/ |
||||
inline void reducedDuplexRow1v2(uint64_t *state, uint64_t *rowIn, uint64_t *rowOut, uint64_t nCols) { |
||||
uint64_t* ptrWordIn = rowIn; //In Lyra2: pointer to prev
|
||||
uint64_t* ptrWordOut = rowOut + (nCols-1)*BLOCK_LEN_INT64; //In Lyra2: pointer to row
|
||||
int i; |
||||
|
||||
for (i = 0; i < nCols; i++) { |
||||
|
||||
//Absorbing "M[prev][col]"
|
||||
state[0] ^= (ptrWordIn[0]); |
||||
state[1] ^= (ptrWordIn[1]); |
||||
state[2] ^= (ptrWordIn[2]); |
||||
state[3] ^= (ptrWordIn[3]); |
||||
state[4] ^= (ptrWordIn[4]); |
||||
state[5] ^= (ptrWordIn[5]); |
||||
state[6] ^= (ptrWordIn[6]); |
||||
state[7] ^= (ptrWordIn[7]); |
||||
state[8] ^= (ptrWordIn[8]); |
||||
state[9] ^= (ptrWordIn[9]); |
||||
state[10] ^= (ptrWordIn[10]); |
||||
state[11] ^= (ptrWordIn[11]); |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
//M[row][C-1-col] = M[prev][col] XOR rand
|
||||
ptrWordOut[0] = ptrWordIn[0] ^ state[0]; |
||||
ptrWordOut[1] = ptrWordIn[1] ^ state[1]; |
||||
ptrWordOut[2] = ptrWordIn[2] ^ state[2]; |
||||
ptrWordOut[3] = ptrWordIn[3] ^ state[3]; |
||||
ptrWordOut[4] = ptrWordIn[4] ^ state[4]; |
||||
ptrWordOut[5] = ptrWordIn[5] ^ state[5]; |
||||
ptrWordOut[6] = ptrWordIn[6] ^ state[6]; |
||||
ptrWordOut[7] = ptrWordIn[7] ^ state[7]; |
||||
ptrWordOut[8] = ptrWordIn[8] ^ state[8]; |
||||
ptrWordOut[9] = ptrWordIn[9] ^ state[9]; |
||||
ptrWordOut[10] = ptrWordIn[10] ^ state[10]; |
||||
ptrWordOut[11] = ptrWordIn[11] ^ state[11]; |
||||
|
||||
|
||||
//Input: next column (i.e., next block in sequence)
|
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
//Output: goes to previous column
|
||||
ptrWordOut -= BLOCK_LEN_INT64; |
||||
} |
||||
} |
||||
|
||||
/**
|
||||
* Performs a duplexing operation over "M[rowInOut][col] [+] M[rowIn][col]" (i.e., |
||||
* the wordwise addition of two columns, ignoring carries between words). The |
||||
* output of this operation, "rand", is then used to make |
||||
* "M[rowOut][(N_COLS-1)-col] = M[rowIn][col] XOR rand" and |
||||
* "M[rowInOut][col] = M[rowInOut][col] XOR rotW(rand)", where rotW is a 64-bit |
||||
* rotation to the left and N_COLS is a system parameter. |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowIn Row used only as input |
||||
* @param rowInOut Row used as input and to receive output after rotation |
||||
* @param rowOut Row receiving the output |
||||
* |
||||
*/ |
||||
inline void reducedDuplexRowSetupv2(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut, uint64_t nCols) { |
||||
uint64_t* ptrWordIn = rowIn; //In Lyra2: pointer to prev
|
||||
uint64_t* ptrWordInOut = rowInOut; //In Lyra2: pointer to row*
|
||||
uint64_t* ptrWordOut = rowOut + (nCols-1)*BLOCK_LEN_INT64; //In Lyra2: pointer to row
|
||||
int i; |
||||
|
||||
for (i = 0; i < nCols; i++) { |
||||
//Absorbing "M[prev] [+] M[row*]"
|
||||
state[0] ^= (ptrWordIn[0] + ptrWordInOut[0]); |
||||
state[1] ^= (ptrWordIn[1] + ptrWordInOut[1]); |
||||
state[2] ^= (ptrWordIn[2] + ptrWordInOut[2]); |
||||
state[3] ^= (ptrWordIn[3] + ptrWordInOut[3]); |
||||
state[4] ^= (ptrWordIn[4] + ptrWordInOut[4]); |
||||
state[5] ^= (ptrWordIn[5] + ptrWordInOut[5]); |
||||
state[6] ^= (ptrWordIn[6] + ptrWordInOut[6]); |
||||
state[7] ^= (ptrWordIn[7] + ptrWordInOut[7]); |
||||
state[8] ^= (ptrWordIn[8] + ptrWordInOut[8]); |
||||
state[9] ^= (ptrWordIn[9] + ptrWordInOut[9]); |
||||
state[10] ^= (ptrWordIn[10] + ptrWordInOut[10]); |
||||
state[11] ^= (ptrWordIn[11] + ptrWordInOut[11]); |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
//M[row][col] = M[prev][col] XOR rand
|
||||
ptrWordOut[0] = ptrWordIn[0] ^ state[0]; |
||||
ptrWordOut[1] = ptrWordIn[1] ^ state[1]; |
||||
ptrWordOut[2] = ptrWordIn[2] ^ state[2]; |
||||
ptrWordOut[3] = ptrWordIn[3] ^ state[3]; |
||||
ptrWordOut[4] = ptrWordIn[4] ^ state[4]; |
||||
ptrWordOut[5] = ptrWordIn[5] ^ state[5]; |
||||
ptrWordOut[6] = ptrWordIn[6] ^ state[6]; |
||||
ptrWordOut[7] = ptrWordIn[7] ^ state[7]; |
||||
ptrWordOut[8] = ptrWordIn[8] ^ state[8]; |
||||
ptrWordOut[9] = ptrWordIn[9] ^ state[9]; |
||||
ptrWordOut[10] = ptrWordIn[10] ^ state[10]; |
||||
ptrWordOut[11] = ptrWordIn[11] ^ state[11]; |
||||
|
||||
//M[row*][col] = M[row*][col] XOR rotW(rand)
|
||||
ptrWordInOut[0] ^= state[11]; |
||||
ptrWordInOut[1] ^= state[0]; |
||||
ptrWordInOut[2] ^= state[1]; |
||||
ptrWordInOut[3] ^= state[2]; |
||||
ptrWordInOut[4] ^= state[3]; |
||||
ptrWordInOut[5] ^= state[4]; |
||||
ptrWordInOut[6] ^= state[5]; |
||||
ptrWordInOut[7] ^= state[6]; |
||||
ptrWordInOut[8] ^= state[7]; |
||||
ptrWordInOut[9] ^= state[8]; |
||||
ptrWordInOut[10] ^= state[9]; |
||||
ptrWordInOut[11] ^= state[10]; |
||||
|
||||
//Inputs: next column (i.e., next block in sequence)
|
||||
ptrWordInOut += BLOCK_LEN_INT64; |
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
//Output: goes to previous column
|
||||
ptrWordOut -= BLOCK_LEN_INT64; |
||||
} |
||||
} |
||||
|
||||
/**
|
||||
* Performs a duplexing operation over "M[rowInOut][col] [+] M[rowIn][col]" (i.e., |
||||
* the wordwise addition of two columns, ignoring carries between words). The |
||||
* output of this operation, "rand", is then used to make |
||||
* "M[rowOut][col] = M[rowOut][col] XOR rand" and |
||||
* "M[rowInOut][col] = M[rowInOut][col] XOR rotW(rand)", where rotW is a 64-bit |
||||
* rotation to the left. |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowIn Row used only as input |
||||
* @param rowInOut Row used as input and to receive output after rotation |
||||
* @param rowOut Row receiving the output |
||||
* |
||||
*/ |
||||
inline void reducedDuplexRowv2(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut, uint64_t nCols) { |
||||
uint64_t* ptrWordInOut = rowInOut; //In Lyra2: pointer to row*
|
||||
uint64_t* ptrWordIn = rowIn; //In Lyra2: pointer to prev
|
||||
uint64_t* ptrWordOut = rowOut; //In Lyra2: pointer to row
|
||||
int i; |
||||
|
||||
for (i = 0; i < nCols; i++) { |
||||
|
||||
//Absorbing "M[prev] [+] M[row*]"
|
||||
state[0] ^= (ptrWordIn[0] + ptrWordInOut[0]); |
||||
state[1] ^= (ptrWordIn[1] + ptrWordInOut[1]); |
||||
state[2] ^= (ptrWordIn[2] + ptrWordInOut[2]); |
||||
state[3] ^= (ptrWordIn[3] + ptrWordInOut[3]); |
||||
state[4] ^= (ptrWordIn[4] + ptrWordInOut[4]); |
||||
state[5] ^= (ptrWordIn[5] + ptrWordInOut[5]); |
||||
state[6] ^= (ptrWordIn[6] + ptrWordInOut[6]); |
||||
state[7] ^= (ptrWordIn[7] + ptrWordInOut[7]); |
||||
state[8] ^= (ptrWordIn[8] + ptrWordInOut[8]); |
||||
state[9] ^= (ptrWordIn[9] + ptrWordInOut[9]); |
||||
state[10] ^= (ptrWordIn[10] + ptrWordInOut[10]); |
||||
state[11] ^= (ptrWordIn[11] + ptrWordInOut[11]); |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
//M[rowOut][col] = M[rowOut][col] XOR rand
|
||||
ptrWordOut[0] ^= state[0]; |
||||
ptrWordOut[1] ^= state[1]; |
||||
ptrWordOut[2] ^= state[2]; |
||||
ptrWordOut[3] ^= state[3]; |
||||
ptrWordOut[4] ^= state[4]; |
||||
ptrWordOut[5] ^= state[5]; |
||||
ptrWordOut[6] ^= state[6]; |
||||
ptrWordOut[7] ^= state[7]; |
||||
ptrWordOut[8] ^= state[8]; |
||||
ptrWordOut[9] ^= state[9]; |
||||
ptrWordOut[10] ^= state[10]; |
||||
ptrWordOut[11] ^= state[11]; |
||||
|
||||
//M[rowInOut][col] = M[rowInOut][col] XOR rotW(rand)
|
||||
ptrWordInOut[0] ^= state[11]; |
||||
ptrWordInOut[1] ^= state[0]; |
||||
ptrWordInOut[2] ^= state[1]; |
||||
ptrWordInOut[3] ^= state[2]; |
||||
ptrWordInOut[4] ^= state[3]; |
||||
ptrWordInOut[5] ^= state[4]; |
||||
ptrWordInOut[6] ^= state[5]; |
||||
ptrWordInOut[7] ^= state[6]; |
||||
ptrWordInOut[8] ^= state[7]; |
||||
ptrWordInOut[9] ^= state[8]; |
||||
ptrWordInOut[10] ^= state[9]; |
||||
ptrWordInOut[11] ^= state[10]; |
||||
|
||||
//Goes to next block
|
||||
ptrWordOut += BLOCK_LEN_INT64; |
||||
ptrWordInOut += BLOCK_LEN_INT64; |
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
} |
||||
} |
||||
|
||||
|
||||
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
|
||||
|
||||
/**
|
||||
* Performs a duplex operation over "M[rowInOut] [+] M[rowIn]", writing the output "rand" |
||||
* on M[rowOut] and making "M[rowInOut] = M[rowInOut] XOR rotW(rand)", where rotW is a 64-bit |
||||
* rotation to the left. |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowIn Row used only as input |
||||
* @param rowInOut Row used as input and to receive output after rotation |
||||
* @param rowOut Row receiving the output |
||||
* |
||||
*/ |
||||
/*
|
||||
inline void reducedDuplexRowSetupOLD(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut) { |
||||
uint64_t* ptrWordIn = rowIn; //In Lyra2: pointer to prev
|
||||
uint64_t* ptrWordInOut = rowInOut; //In Lyra2: pointer to row*
|
||||
uint64_t* ptrWordOut = rowOut; //In Lyra2: pointer to row
|
||||
int i; |
||||
for (i = 0; i < N_COLS; i++) { |
||||
|
||||
//Absorbing "M[rowInOut] XOR M[rowIn]"
|
||||
state[0] ^= ptrWordInOut[0] ^ ptrWordIn[0]; |
||||
state[1] ^= ptrWordInOut[1] ^ ptrWordIn[1]; |
||||
state[2] ^= ptrWordInOut[2] ^ ptrWordIn[2]; |
||||
state[3] ^= ptrWordInOut[3] ^ ptrWordIn[3]; |
||||
state[4] ^= ptrWordInOut[4] ^ ptrWordIn[4]; |
||||
state[5] ^= ptrWordInOut[5] ^ ptrWordIn[5]; |
||||
state[6] ^= ptrWordInOut[6] ^ ptrWordIn[6]; |
||||
state[7] ^= ptrWordInOut[7] ^ ptrWordIn[7]; |
||||
state[8] ^= ptrWordInOut[8] ^ ptrWordIn[8]; |
||||
state[9] ^= ptrWordInOut[9] ^ ptrWordIn[9]; |
||||
state[10] ^= ptrWordInOut[10] ^ ptrWordIn[10]; |
||||
state[11] ^= ptrWordInOut[11] ^ ptrWordIn[11]; |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
//M[row][col] = rand
|
||||
ptrWordOut[0] = state[0]; |
||||
ptrWordOut[1] = state[1]; |
||||
ptrWordOut[2] = state[2]; |
||||
ptrWordOut[3] = state[3]; |
||||
ptrWordOut[4] = state[4]; |
||||
ptrWordOut[5] = state[5]; |
||||
ptrWordOut[6] = state[6]; |
||||
ptrWordOut[7] = state[7]; |
||||
ptrWordOut[8] = state[8]; |
||||
ptrWordOut[9] = state[9]; |
||||
ptrWordOut[10] = state[10]; |
||||
ptrWordOut[11] = state[11]; |
||||
|
||||
|
||||
//M[row*][col] = M[row*][col] XOR rotW(rand)
|
||||
ptrWordInOut[0] ^= state[10]; |
||||
ptrWordInOut[1] ^= state[11]; |
||||
ptrWordInOut[2] ^= state[0]; |
||||
ptrWordInOut[3] ^= state[1]; |
||||
ptrWordInOut[4] ^= state[2]; |
||||
ptrWordInOut[5] ^= state[3]; |
||||
ptrWordInOut[6] ^= state[4]; |
||||
ptrWordInOut[7] ^= state[5]; |
||||
ptrWordInOut[8] ^= state[6]; |
||||
ptrWordInOut[9] ^= state[7]; |
||||
ptrWordInOut[10] ^= state[8]; |
||||
ptrWordInOut[11] ^= state[9]; |
||||
|
||||
//Goes to next column (i.e., next block in sequence)
|
||||
ptrWordInOut += BLOCK_LEN_INT64; |
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
ptrWordOut += BLOCK_LEN_INT64; |
||||
} |
||||
} |
||||
*/ |
||||
|
||||
/**
|
||||
* Performs a duplex operation over "M[rowInOut] XOR M[rowIn]", writing the output "rand" |
||||
* on M[rowOut] and making "M[rowInOut] = M[rowInOut] XOR rotW(rand)", where rotW is a 64-bit |
||||
* rotation to the left. |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowIn Row used only as input |
||||
* @param rowInOut Row used as input and to receive output after rotation |
||||
* @param rowOut Row receiving the output |
||||
* |
||||
*/ |
||||
/*
|
||||
inline void reducedDuplexRowSetupv5(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut) { |
||||
uint64_t* ptrWordIn = rowIn; //In Lyra2: pointer to prev
|
||||
uint64_t* ptrWordInOut = rowInOut; //In Lyra2: pointer to row*
|
||||
uint64_t* ptrWordOut = rowOut; //In Lyra2: pointer to row
|
||||
int i; |
||||
for (i = 0; i < N_COLS; i++) { |
||||
|
||||
//Absorbing "M[rowInOut] XOR M[rowIn]"
|
||||
state[0] ^= ptrWordInOut[0] + ptrWordIn[0]; |
||||
state[1] ^= ptrWordInOut[1] + ptrWordIn[1]; |
||||
state[2] ^= ptrWordInOut[2] + ptrWordIn[2]; |
||||
state[3] ^= ptrWordInOut[3] + ptrWordIn[3]; |
||||
state[4] ^= ptrWordInOut[4] + ptrWordIn[4]; |
||||
state[5] ^= ptrWordInOut[5] + ptrWordIn[5]; |
||||
state[6] ^= ptrWordInOut[6] + ptrWordIn[6]; |
||||
state[7] ^= ptrWordInOut[7] + ptrWordIn[7]; |
||||
state[8] ^= ptrWordInOut[8] + ptrWordIn[8]; |
||||
state[9] ^= ptrWordInOut[9] + ptrWordIn[9]; |
||||
state[10] ^= ptrWordInOut[10] + ptrWordIn[10]; |
||||
state[11] ^= ptrWordInOut[11] + ptrWordIn[11]; |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
|
||||
//M[row*][col] = M[row*][col] XOR rotW(rand)
|
||||
ptrWordInOut[0] ^= state[10]; |
||||
ptrWordInOut[1] ^= state[11]; |
||||
ptrWordInOut[2] ^= state[0]; |
||||
ptrWordInOut[3] ^= state[1]; |
||||
ptrWordInOut[4] ^= state[2]; |
||||
ptrWordInOut[5] ^= state[3]; |
||||
ptrWordInOut[6] ^= state[4]; |
||||
ptrWordInOut[7] ^= state[5]; |
||||
ptrWordInOut[8] ^= state[6]; |
||||
ptrWordInOut[9] ^= state[7]; |
||||
ptrWordInOut[10] ^= state[8]; |
||||
ptrWordInOut[11] ^= state[9]; |
||||
|
||||
|
||||
//M[row][col] = rand
|
||||
ptrWordOut[0] = state[0] ^ ptrWordIn[0]; |
||||
ptrWordOut[1] = state[1] ^ ptrWordIn[1]; |
||||
ptrWordOut[2] = state[2] ^ ptrWordIn[2]; |
||||
ptrWordOut[3] = state[3] ^ ptrWordIn[3]; |
||||
ptrWordOut[4] = state[4] ^ ptrWordIn[4]; |
||||
ptrWordOut[5] = state[5] ^ ptrWordIn[5]; |
||||
ptrWordOut[6] = state[6] ^ ptrWordIn[6]; |
||||
ptrWordOut[7] = state[7] ^ ptrWordIn[7]; |
||||
ptrWordOut[8] = state[8] ^ ptrWordIn[8]; |
||||
ptrWordOut[9] = state[9] ^ ptrWordIn[9]; |
||||
ptrWordOut[10] = state[10] ^ ptrWordIn[10]; |
||||
ptrWordOut[11] = state[11] ^ ptrWordIn[11]; |
||||
|
||||
//Goes to next column (i.e., next block in sequence)
|
||||
ptrWordInOut += BLOCK_LEN_INT64; |
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
ptrWordOut += BLOCK_LEN_INT64; |
||||
} |
||||
} |
||||
*/ |
||||
|
||||
/**
|
||||
* Performs a duplex operation over "M[rowInOut] XOR M[rowIn]", writing the output "rand" |
||||
* on M[rowOut] and making "M[rowInOut] = M[rowInOut] XOR rotW(rand)", where rotW is a 64-bit |
||||
* rotation to the left. |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowIn Row used only as input |
||||
* @param rowInOut Row used as input and to receive output after rotation |
||||
* @param rowOut Row receiving the output |
||||
* |
||||
*/ |
||||
/*
|
||||
inline void reducedDuplexRowSetupv5c(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut) { |
||||
uint64_t* ptrWordIn = rowIn; //In Lyra2: pointer to prev
|
||||
uint64_t* ptrWordInOut = rowInOut; //In Lyra2: pointer to row*
|
||||
uint64_t* ptrWordOut = rowOut; |
||||
int i; |
||||
|
||||
for (i = 0; i < N_COLS / 2; i++) { |
||||
//Absorbing "M[rowInOut] XOR M[rowIn]"
|
||||
state[0] ^= ptrWordInOut[0] + ptrWordIn[0]; |
||||
state[1] ^= ptrWordInOut[1] + ptrWordIn[1]; |
||||
state[2] ^= ptrWordInOut[2] + ptrWordIn[2]; |
||||
state[3] ^= ptrWordInOut[3] + ptrWordIn[3]; |
||||
state[4] ^= ptrWordInOut[4] + ptrWordIn[4]; |
||||
state[5] ^= ptrWordInOut[5] + ptrWordIn[5]; |
||||
state[6] ^= ptrWordInOut[6] + ptrWordIn[6]; |
||||
state[7] ^= ptrWordInOut[7] + ptrWordIn[7]; |
||||
state[8] ^= ptrWordInOut[8] + ptrWordIn[8]; |
||||
state[9] ^= ptrWordInOut[9] + ptrWordIn[9]; |
||||
state[10] ^= ptrWordInOut[10] + ptrWordIn[10]; |
||||
state[11] ^= ptrWordInOut[11] + ptrWordIn[11]; |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
|
||||
//M[row*][col] = M[row*][col] XOR rotW(rand)
|
||||
ptrWordInOut[0] ^= state[10]; |
||||
ptrWordInOut[1] ^= state[11]; |
||||
ptrWordInOut[2] ^= state[0]; |
||||
ptrWordInOut[3] ^= state[1]; |
||||
ptrWordInOut[4] ^= state[2]; |
||||
ptrWordInOut[5] ^= state[3]; |
||||
ptrWordInOut[6] ^= state[4]; |
||||
ptrWordInOut[7] ^= state[5]; |
||||
ptrWordInOut[8] ^= state[6]; |
||||
ptrWordInOut[9] ^= state[7]; |
||||
ptrWordInOut[10] ^= state[8]; |
||||
ptrWordInOut[11] ^= state[9]; |
||||
|
||||
|
||||
//M[row][col] = rand
|
||||
ptrWordOut[0] = state[0] ^ ptrWordIn[0]; |
||||
ptrWordOut[1] = state[1] ^ ptrWordIn[1]; |
||||
ptrWordOut[2] = state[2] ^ ptrWordIn[2]; |
||||
ptrWordOut[3] = state[3] ^ ptrWordIn[3]; |
||||
ptrWordOut[4] = state[4] ^ ptrWordIn[4]; |
||||
ptrWordOut[5] = state[5] ^ ptrWordIn[5]; |
||||
ptrWordOut[6] = state[6] ^ ptrWordIn[6]; |
||||
ptrWordOut[7] = state[7] ^ ptrWordIn[7]; |
||||
ptrWordOut[8] = state[8] ^ ptrWordIn[8]; |
||||
ptrWordOut[9] = state[9] ^ ptrWordIn[9]; |
||||
ptrWordOut[10] = state[10] ^ ptrWordIn[10]; |
||||
ptrWordOut[11] = state[11] ^ ptrWordIn[11]; |
||||
|
||||
//Goes to next column (i.e., next block in sequence)
|
||||
ptrWordInOut += BLOCK_LEN_INT64; |
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
ptrWordOut += 2 * BLOCK_LEN_INT64; |
||||
} |
||||
|
||||
ptrWordOut = rowOut + BLOCK_LEN_INT64; |
||||
for (i = 0; i < N_COLS / 2; i++) { |
||||
//Absorbing "M[rowInOut] XOR M[rowIn]"
|
||||
state[0] ^= ptrWordInOut[0] + ptrWordIn[0]; |
||||
state[1] ^= ptrWordInOut[1] + ptrWordIn[1]; |
||||
state[2] ^= ptrWordInOut[2] + ptrWordIn[2]; |
||||
state[3] ^= ptrWordInOut[3] + ptrWordIn[3]; |
||||
state[4] ^= ptrWordInOut[4] + ptrWordIn[4]; |
||||
state[5] ^= ptrWordInOut[5] + ptrWordIn[5]; |
||||
state[6] ^= ptrWordInOut[6] + ptrWordIn[6]; |
||||
state[7] ^= ptrWordInOut[7] + ptrWordIn[7]; |
||||
state[8] ^= ptrWordInOut[8] + ptrWordIn[8]; |
||||
state[9] ^= ptrWordInOut[9] + ptrWordIn[9]; |
||||
state[10] ^= ptrWordInOut[10] + ptrWordIn[10]; |
||||
state[11] ^= ptrWordInOut[11] + ptrWordIn[11]; |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
|
||||
//M[row*][col] = M[row*][col] XOR rotW(rand)
|
||||
ptrWordInOut[0] ^= state[10]; |
||||
ptrWordInOut[1] ^= state[11]; |
||||
ptrWordInOut[2] ^= state[0]; |
||||
ptrWordInOut[3] ^= state[1]; |
||||
ptrWordInOut[4] ^= state[2]; |
||||
ptrWordInOut[5] ^= state[3]; |
||||
ptrWordInOut[6] ^= state[4]; |
||||
ptrWordInOut[7] ^= state[5]; |
||||
ptrWordInOut[8] ^= state[6]; |
||||
ptrWordInOut[9] ^= state[7]; |
||||
ptrWordInOut[10] ^= state[8]; |
||||
ptrWordInOut[11] ^= state[9]; |
||||
|
||||
|
||||
//M[row][col] = rand
|
||||
ptrWordOut[0] = state[0] ^ ptrWordIn[0]; |
||||
ptrWordOut[1] = state[1] ^ ptrWordIn[1]; |
||||
ptrWordOut[2] = state[2] ^ ptrWordIn[2]; |
||||
ptrWordOut[3] = state[3] ^ ptrWordIn[3]; |
||||
ptrWordOut[4] = state[4] ^ ptrWordIn[4]; |
||||
ptrWordOut[5] = state[5] ^ ptrWordIn[5]; |
||||
ptrWordOut[6] = state[6] ^ ptrWordIn[6]; |
||||
ptrWordOut[7] = state[7] ^ ptrWordIn[7]; |
||||
ptrWordOut[8] = state[8] ^ ptrWordIn[8]; |
||||
ptrWordOut[9] = state[9] ^ ptrWordIn[9]; |
||||
ptrWordOut[10] = state[10] ^ ptrWordIn[10]; |
||||
ptrWordOut[11] = state[11] ^ ptrWordIn[11]; |
||||
|
||||
//Goes to next column (i.e., next block in sequence)
|
||||
ptrWordInOut += BLOCK_LEN_INT64; |
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
ptrWordOut += 2 * BLOCK_LEN_INT64; |
||||
} |
||||
} |
||||
*/ |
||||
|
||||
/**
|
||||
* Performs a duplex operation over "M[rowInOut] XOR M[rowIn]", using the output "rand" |
||||
* to make "M[rowOut][col] = M[rowOut][col] XOR rand" and "M[rowInOut] = M[rowInOut] XOR rotW(rand)", |
||||
* where rotW is a 64-bit rotation to the left. |
||||
* |
||||
* @param state The current state of the sponge |
||||
* @param rowIn Row used only as input |
||||
* @param rowInOut Row used as input and to receive output after rotation |
||||
* @param rowOut Row receiving the output |
||||
* |
||||
*/ |
||||
/*
|
||||
inline void reducedDuplexRowd(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut) { |
||||
uint64_t* ptrWordInOut = rowInOut; //In Lyra2: pointer to row*
|
||||
uint64_t* ptrWordIn = rowIn; //In Lyra2: pointer to prev
|
||||
uint64_t* ptrWordOut = rowOut; //In Lyra2: pointer to row
|
||||
int i; |
||||
for (i = 0; i < N_COLS; i++) { |
||||
|
||||
//Absorbing "M[rowInOut] XOR M[rowIn]"
|
||||
state[0] ^= ptrWordInOut[0] + ptrWordIn[0]; |
||||
state[1] ^= ptrWordInOut[1] + ptrWordIn[1]; |
||||
state[2] ^= ptrWordInOut[2] + ptrWordIn[2]; |
||||
state[3] ^= ptrWordInOut[3] + ptrWordIn[3]; |
||||
state[4] ^= ptrWordInOut[4] + ptrWordIn[4]; |
||||
state[5] ^= ptrWordInOut[5] + ptrWordIn[5]; |
||||
state[6] ^= ptrWordInOut[6] + ptrWordIn[6]; |
||||
state[7] ^= ptrWordInOut[7] + ptrWordIn[7]; |
||||
state[8] ^= ptrWordInOut[8] + ptrWordIn[8]; |
||||
state[9] ^= ptrWordInOut[9] + ptrWordIn[9]; |
||||
state[10] ^= ptrWordInOut[10] + ptrWordIn[10]; |
||||
state[11] ^= ptrWordInOut[11] + ptrWordIn[11]; |
||||
|
||||
//Applies the reduced-round transformation f to the sponge's state
|
||||
reducedBlake2bLyra(state); |
||||
|
||||
//M[rowOut][col] = M[rowOut][col] XOR rand
|
||||
ptrWordOut[0] ^= state[0]; |
||||
ptrWordOut[1] ^= state[1]; |
||||
ptrWordOut[2] ^= state[2]; |
||||
ptrWordOut[3] ^= state[3]; |
||||
ptrWordOut[4] ^= state[4]; |
||||
ptrWordOut[5] ^= state[5]; |
||||
ptrWordOut[6] ^= state[6]; |
||||
ptrWordOut[7] ^= state[7]; |
||||
ptrWordOut[8] ^= state[8]; |
||||
ptrWordOut[9] ^= state[9]; |
||||
ptrWordOut[10] ^= state[10]; |
||||
ptrWordOut[11] ^= state[11]; |
||||
|
||||
//M[rowInOut][col] = M[rowInOut][col] XOR rotW(rand)
|
||||
|
||||
|
||||
//Goes to next block
|
||||
ptrWordOut += BLOCK_LEN_INT64; |
||||
ptrWordInOut += BLOCK_LEN_INT64; |
||||
ptrWordIn += BLOCK_LEN_INT64; |
||||
} |
||||
} |
||||
*/ |
||||
|
||||
/**
|
||||
Prints an array of unsigned chars |
||||
*/ |
||||
void printArrayv2(unsigned char *array, unsigned int size, char *name) { |
||||
int i; |
||||
printf("%s: ", name); |
||||
for (i = 0; i < size; i++) { |
||||
printf("%2x|", array[i]); |
||||
} |
||||
printf("\n"); |
||||
} |
||||
|
||||
////////////////////////////////////////////////////////////////////////////////////////////////
|
@ -0,0 +1,108 @@
@@ -0,0 +1,108 @@
|
||||
/**
|
||||
* Header file for Blake2b's internal permutation in the form of a sponge. |
||||
* This code is based on the original Blake2b's implementation provided by |
||||
* Samuel Neves (https://blake2.net/)
|
||||
* |
||||
* Author: The Lyra PHC team (http://www.lyra-kdf.net/) -- 2014.
|
||||
* |
||||
* This software is hereby placed in the public domain. |
||||
* |
||||
* THIS SOFTWARE IS PROVIDED BY THE AUTHORS ''AS IS'' AND ANY EXPRESS |
||||
* OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED |
||||
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE |
||||
* ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHORS OR CONTRIBUTORS BE |
||||
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR |
||||
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF |
||||
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR |
||||
* BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, |
||||
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE |
||||
* OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, |
||||
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
||||
*/ |
||||
#ifndef SPONGE_H_ |
||||
#define SPONGE_H_ |
||||
|
||||
#include <stdint.h> |
||||
|
||||
#if defined(__GNUC__) |
||||
#define ALIGN __attribute__ ((aligned(32))) |
||||
#elif defined(_MSC_VER) |
||||
#define ALIGN __declspec(align(32)) |
||||
#else |
||||
#define ALIGN |
||||
#endif |
||||
|
||||
|
||||
/*Blake2b IV Array*/ |
||||
static const uint64_t blake2b_IV[8] = |
||||
{ |
||||
0x6a09e667f3bcc908ULL, 0xbb67ae8584caa73bULL, |
||||
0x3c6ef372fe94f82bULL, 0xa54ff53a5f1d36f1ULL, |
||||
0x510e527fade682d1ULL, 0x9b05688c2b3e6c1fULL, |
||||
0x1f83d9abfb41bd6bULL, 0x5be0cd19137e2179ULL |
||||
}; |
||||
|
||||
/*Blake2b's rotation*/ |
||||
static inline uint64_t rotr64( const uint64_t w, const unsigned c ){ |
||||
return ( w >> c ) | ( w << ( 64 - c ) ); |
||||
} |
||||
|
||||
/*Blake2b's G function*/ |
||||
#define G(r,i,a,b,c,d) \ |
||||
do { \ |
||||
a = a + b; \ |
||||
d = rotr64(d ^ a, 32); \ |
||||
c = c + d; \ |
||||
b = rotr64(b ^ c, 24); \ |
||||
a = a + b; \ |
||||
d = rotr64(d ^ a, 16); \ |
||||
c = c + d; \ |
||||
b = rotr64(b ^ c, 63); \ |
||||
} while(0) |
||||
|
||||
|
||||
/*One Round of the Blake2b's compression function*/ |
||||
#define ROUND_LYRA(r) \ |
||||
G(r,0,v[ 0],v[ 4],v[ 8],v[12]); \ |
||||
G(r,1,v[ 1],v[ 5],v[ 9],v[13]); \ |
||||
G(r,2,v[ 2],v[ 6],v[10],v[14]); \ |
||||
G(r,3,v[ 3],v[ 7],v[11],v[15]); \ |
||||
G(r,4,v[ 0],v[ 5],v[10],v[15]); \ |
||||
G(r,5,v[ 1],v[ 6],v[11],v[12]); \ |
||||
G(r,6,v[ 2],v[ 7],v[ 8],v[13]); \ |
||||
G(r,7,v[ 3],v[ 4],v[ 9],v[14]); |
||||
|
||||
|
||||
//---- Housekeeping
|
||||
extern void initStatev2(uint64_t state[/*16*/]); |
||||
|
||||
//---- Squeezes
|
||||
extern void squeezev2(uint64_t *state, unsigned char *out, unsigned int len); |
||||
extern void reducedSqueezeRow0v2(uint64_t* state, uint64_t* row, uint64_t nCols); |
||||
|
||||
//---- Absorbs
|
||||
extern void absorbBlockv2(uint64_t *state, const uint64_t *in); |
||||
extern void absorbBlockBlake2Safev2(uint64_t *state, const uint64_t *in); |
||||
|
||||
//---- Duplexes
|
||||
extern void reducedDuplexRow1v2(uint64_t *state, uint64_t *rowIn, uint64_t *rowOut, uint64_t nCols); |
||||
extern void reducedDuplexRowSetupv2(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut, uint64_t nCols); |
||||
extern void reducedDuplexRowv2(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut, uint64_t nCols); |
||||
|
||||
//---- Misc
|
||||
void printArrayv2(unsigned char *array, unsigned int size, char *name); |
||||
|
||||
////////////////////////////////////////////////////////////////////////////////////////////////
|
||||
|
||||
|
||||
////TESTS////
|
||||
//void reducedDuplexRowc(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut);
|
||||
//void reducedDuplexRowd(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut);
|
||||
//void reducedDuplexRowSetupv4(uint64_t *state, uint64_t *rowIn1, uint64_t *rowIn2, uint64_t *rowOut1, uint64_t *rowOut2);
|
||||
//void reducedDuplexRowSetupv5(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut);
|
||||
//void reducedDuplexRowSetupv5c(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut);
|
||||
//void reducedDuplexRowSetupv5d(uint64_t *state, uint64_t *rowIn, uint64_t *rowInOut, uint64_t *rowOut);
|
||||
/////////////
|
||||
|
||||
|
||||
#endif /* SPONGE_H_ */ |
@ -1,58 +1,10 @@
@@ -1,58 +1,10 @@
|
||||
#ifndef WHIRLPOOLX_H |
||||
#define WHIRLPOOLX_H |
||||
|
||||
#include <stdint.h> |
||||
#include "miner.h" |
||||
|
||||
// The combined effect of gamma (SubBytes) and theta (MixRows)
|
||||
static uint64_t MAGIC_TABLE[256] = { |
||||
UINT64_C(0xD83078C018601818), UINT64_C(0x2646AF05238C2323), UINT64_C(0xB891F97EC63FC6C6), UINT64_C(0xFBCD6F13E887E8E8), UINT64_C(0xCB13A14C87268787), UINT64_C(0x116D62A9B8DAB8B8), UINT64_C(0x0902050801040101), UINT64_C(0x0D9E6E424F214F4F), |
||||
UINT64_C(0x9B6CEEAD36D83636), UINT64_C(0xFF510459A6A2A6A6), UINT64_C(0x0CB9BDDED26FD2D2), UINT64_C(0x0EF706FBF5F3F5F5), UINT64_C(0x96F280EF79F97979), UINT64_C(0x30DECE5F6FA16F6F), UINT64_C(0x6D3FEFFC917E9191), UINT64_C(0xF8A407AA52555252), |
||||
UINT64_C(0x47C0FD27609D6060), UINT64_C(0x35657689BCCABCBC), UINT64_C(0x372BCDAC9B569B9B), UINT64_C(0x8A018C048E028E8E), UINT64_C(0xD25B1571A3B6A3A3), UINT64_C(0x6C183C600C300C0C), UINT64_C(0x84F68AFF7BF17B7B), UINT64_C(0x806AE1B535D43535), |
||||
UINT64_C(0xF53A69E81D741D1D), UINT64_C(0xB3DD4753E0A7E0E0), UINT64_C(0x21B3ACF6D77BD7D7), UINT64_C(0x9C99ED5EC22FC2C2), UINT64_C(0x435C966D2EB82E2E), UINT64_C(0x29967A624B314B4B), UINT64_C(0x5DE121A3FEDFFEFE), UINT64_C(0xD5AE168257415757), |
||||
UINT64_C(0xBD2A41A815541515), UINT64_C(0xE8EEB69F77C17777), UINT64_C(0x926EEBA537DC3737), UINT64_C(0x9ED7567BE5B3E5E5), UINT64_C(0x1323D98C9F469F9F), UINT64_C(0x23FD17D3F0E7F0F0), UINT64_C(0x20947F6A4A354A4A), UINT64_C(0x44A9959EDA4FDADA), |
||||
UINT64_C(0xA2B025FA587D5858), UINT64_C(0xCF8FCA06C903C9C9), UINT64_C(0x7C528D5529A42929), UINT64_C(0x5A1422500A280A0A), UINT64_C(0x507F4FE1B1FEB1B1), UINT64_C(0xC95D1A69A0BAA0A0), UINT64_C(0x14D6DA7F6BB16B6B), UINT64_C(0xD917AB5C852E8585), |
||||
UINT64_C(0x3C677381BDCEBDBD), UINT64_C(0x8FBA34D25D695D5D), UINT64_C(0x9020508010401010), UINT64_C(0x07F503F3F4F7F4F4), UINT64_C(0xDD8BC016CB0BCBCB), UINT64_C(0xD37CC6ED3EF83E3E), UINT64_C(0x2D0A112805140505), UINT64_C(0x78CEE61F67816767), |
||||
UINT64_C(0x97D55373E4B7E4E4), UINT64_C(0x024EBB25279C2727), UINT64_C(0x7382583241194141), UINT64_C(0xA70B9D2C8B168B8B), UINT64_C(0xF6530151A7A6A7A7), UINT64_C(0xB2FA94CF7DE97D7D), UINT64_C(0x4937FBDC956E9595), UINT64_C(0x56AD9F8ED847D8D8), |
||||
UINT64_C(0x70EB308BFBCBFBFB), UINT64_C(0xCDC17123EE9FEEEE), UINT64_C(0xBBF891C77CED7C7C), UINT64_C(0x71CCE31766856666), UINT64_C(0x7BA78EA6DD53DDDD), UINT64_C(0xAF2E4BB8175C1717), UINT64_C(0x458E460247014747), UINT64_C(0x1A21DC849E429E9E), |
||||
UINT64_C(0xD489C51ECA0FCACA), UINT64_C(0x585A99752DB42D2D), UINT64_C(0x2E637991BFC6BFBF), UINT64_C(0x3F0E1B38071C0707), UINT64_C(0xAC472301AD8EADAD), UINT64_C(0xB0B42FEA5A755A5A), UINT64_C(0xEF1BB56C83368383), UINT64_C(0xB666FF8533CC3333), |
||||
UINT64_C(0x5CC6F23F63916363), UINT64_C(0x12040A1002080202), UINT64_C(0x93493839AA92AAAA), UINT64_C(0xDEE2A8AF71D97171), UINT64_C(0xC68DCF0EC807C8C8), UINT64_C(0xD1327DC819641919), UINT64_C(0x3B92707249394949), UINT64_C(0x5FAF9A86D943D9D9), |
||||
UINT64_C(0x31F91DC3F2EFF2F2), UINT64_C(0xA8DB484BE3ABE3E3), UINT64_C(0xB9B62AE25B715B5B), UINT64_C(0xBC0D9234881A8888), UINT64_C(0x3E29C8A49A529A9A), UINT64_C(0x0B4CBE2D26982626), UINT64_C(0xBF64FA8D32C83232), UINT64_C(0x597D4AE9B0FAB0B0), |
||||
UINT64_C(0xF2CF6A1BE983E9E9), UINT64_C(0x771E33780F3C0F0F), UINT64_C(0x33B7A6E6D573D5D5), UINT64_C(0xF41DBA74803A8080), UINT64_C(0x27617C99BEC2BEBE), UINT64_C(0xEB87DE26CD13CDCD), UINT64_C(0x8968E4BD34D03434), UINT64_C(0x3290757A483D4848), |
||||
UINT64_C(0x54E324ABFFDBFFFF), UINT64_C(0x8DF48FF77AF57A7A), UINT64_C(0x643DEAF4907A9090), UINT64_C(0x9DBE3EC25F615F5F), UINT64_C(0x3D40A01D20802020), UINT64_C(0x0FD0D56768BD6868), UINT64_C(0xCA3472D01A681A1A), UINT64_C(0xB7412C19AE82AEAE), |
||||
UINT64_C(0x7D755EC9B4EAB4B4), UINT64_C(0xCEA8199A544D5454), UINT64_C(0x7F3BE5EC93769393), UINT64_C(0x2F44AA0D22882222), UINT64_C(0x63C8E907648D6464), UINT64_C(0x2AFF12DBF1E3F1F1), UINT64_C(0xCCE6A2BF73D17373), UINT64_C(0x82245A9012481212), |
||||
UINT64_C(0x7A805D3A401D4040), UINT64_C(0x4810284008200808), UINT64_C(0x959BE856C32BC3C3), UINT64_C(0xDFC57B33EC97ECEC), UINT64_C(0x4DAB9096DB4BDBDB), UINT64_C(0xC05F1F61A1BEA1A1), UINT64_C(0x9107831C8D0E8D8D), UINT64_C(0xC87AC9F53DF43D3D), |
||||
UINT64_C(0x5B33F1CC97669797), UINT64_C(0x0000000000000000), UINT64_C(0xF983D436CF1BCFCF), UINT64_C(0x6E5687452BAC2B2B), UINT64_C(0xE1ECB39776C57676), UINT64_C(0xE619B06482328282), UINT64_C(0x28B1A9FED67FD6D6), UINT64_C(0xC33677D81B6C1B1B), |
||||
UINT64_C(0x74775BC1B5EEB5B5), UINT64_C(0xBE432911AF86AFAF), UINT64_C(0x1DD4DF776AB56A6A), UINT64_C(0xEAA00DBA505D5050), UINT64_C(0x578A4C1245094545), UINT64_C(0x38FB18CBF3EBF3F3), UINT64_C(0xAD60F09D30C03030), UINT64_C(0xC4C3742BEF9BEFEF), |
||||
UINT64_C(0xDA7EC3E53FFC3F3F), UINT64_C(0xC7AA1C9255495555), UINT64_C(0xDB591079A2B2A2A2), UINT64_C(0xE9C96503EA8FEAEA), UINT64_C(0x6ACAEC0F65896565), UINT64_C(0x036968B9BAD2BABA), UINT64_C(0x4A5E93652FBC2F2F), UINT64_C(0x8E9DE74EC027C0C0), |
||||
UINT64_C(0x60A181BEDE5FDEDE), UINT64_C(0xFC386CE01C701C1C), UINT64_C(0x46E72EBBFDD3FDFD), UINT64_C(0x1F9A64524D294D4D), UINT64_C(0x7639E0E492729292), UINT64_C(0xFAEABC8F75C97575), UINT64_C(0x360C1E3006180606), UINT64_C(0xAE0998248A128A8A), |
||||
UINT64_C(0x4B7940F9B2F2B2B2), UINT64_C(0x85D15963E6BFE6E6), UINT64_C(0x7E1C36700E380E0E), UINT64_C(0xE73E63F81F7C1F1F), UINT64_C(0x55C4F73762956262), UINT64_C(0x3AB5A3EED477D4D4), UINT64_C(0x814D3229A89AA8A8), UINT64_C(0x5231F4C496629696), |
||||
UINT64_C(0x62EF3A9BF9C3F9F9), UINT64_C(0xA397F666C533C5C5), UINT64_C(0x104AB13525942525), UINT64_C(0xABB220F259795959), UINT64_C(0xD015AE54842A8484), UINT64_C(0xC5E4A7B772D57272), UINT64_C(0xEC72DDD539E43939), UINT64_C(0x1698615A4C2D4C4C), |
||||
UINT64_C(0x94BC3BCA5E655E5E), UINT64_C(0x9FF085E778FD7878), UINT64_C(0xE570D8DD38E03838), UINT64_C(0x980586148C0A8C8C), UINT64_C(0x17BFB2C6D163D1D1), UINT64_C(0xE4570B41A5AEA5A5), UINT64_C(0xA1D94D43E2AFE2E2), UINT64_C(0x4EC2F82F61996161), |
||||
UINT64_C(0x427B45F1B3F6B3B3), UINT64_C(0x3442A51521842121), UINT64_C(0x0825D6949C4A9C9C), UINT64_C(0xEE3C66F01E781E1E), UINT64_C(0x6186522243114343), UINT64_C(0xB193FC76C73BC7C7), UINT64_C(0x4FE52BB3FCD7FCFC), UINT64_C(0x2408142004100404), |
||||
UINT64_C(0xE3A208B251595151), UINT64_C(0x252FC7BC995E9999), UINT64_C(0x22DAC44F6DA96D6D), UINT64_C(0x651A39680D340D0D), UINT64_C(0x79E93583FACFFAFA), UINT64_C(0x69A384B6DF5BDFDF), UINT64_C(0xA9FC9BD77EE57E7E), UINT64_C(0x1948B43D24902424), |
||||
UINT64_C(0xFE76D7C53BEC3B3B), UINT64_C(0x9A4B3D31AB96ABAB), UINT64_C(0xF081D13ECE1FCECE), UINT64_C(0x9922558811441111), UINT64_C(0x8303890C8F068F8F), UINT64_C(0x049C6B4A4E254E4E), UINT64_C(0x667351D1B7E6B7B7), UINT64_C(0xE0CB600BEB8BEBEB), |
||||
UINT64_C(0xC178CCFD3CF03C3C), UINT64_C(0xFD1FBF7C813E8181), UINT64_C(0x4035FED4946A9494), UINT64_C(0x1CF30CEBF7FBF7F7), UINT64_C(0x186F67A1B9DEB9B9), UINT64_C(0x8B265F98134C1313), UINT64_C(0x51589C7D2CB02C2C), UINT64_C(0x05BBB8D6D36BD3D3), |
||||
UINT64_C(0x8CD35C6BE7BBE7E7), UINT64_C(0x39DCCB576EA56E6E), UINT64_C(0xAA95F36EC437C4C4), UINT64_C(0x1B060F18030C0303), UINT64_C(0xDCAC138A56455656), UINT64_C(0x5E88491A440D4444), UINT64_C(0xA0FE9EDF7FE17F7F), UINT64_C(0x884F3721A99EA9A9), |
||||
UINT64_C(0x6754824D2AA82A2A), UINT64_C(0x0A6B6DB1BBD6BBBB), UINT64_C(0x879FE246C123C1C1), UINT64_C(0xF1A602A253515353), UINT64_C(0x72A58BAEDC57DCDC), UINT64_C(0x531627580B2C0B0B), UINT64_C(0x0127D39C9D4E9D9D), UINT64_C(0x2BD8C1476CAD6C6C), |
||||
UINT64_C(0xA462F59531C43131), UINT64_C(0xF3E8B98774CD7474), UINT64_C(0x15F109E3F6FFF6F6), UINT64_C(0x4C8C430A46054646), UINT64_C(0xA5452609AC8AACAC), UINT64_C(0xB50F973C891E8989), UINT64_C(0xB42844A014501414), UINT64_C(0xBADF425BE1A3E1E1), |
||||
UINT64_C(0xA62C4EB016581616), UINT64_C(0xF774D2CD3AE83A3A), UINT64_C(0x06D2D06F69B96969), UINT64_C(0x41122D4809240909), UINT64_C(0xD7E0ADA770DD7070), UINT64_C(0x6F7154D9B6E2B6B6), UINT64_C(0x1EBDB7CED067D0D0), UINT64_C(0xD6C77E3BED93EDED), |
||||
UINT64_C(0xE285DB2ECC17CCCC), UINT64_C(0x6884572A42154242), UINT64_C(0x2C2DC2B4985A9898), UINT64_C(0xED550E49A4AAA4A4), UINT64_C(0x7550885D28A02828), UINT64_C(0x86B831DA5C6D5C5C), UINT64_C(0x6BED3F93F8C7F8F8), UINT64_C(0xC211A44486228686), |
||||
}; |
||||
|
||||
static uint64_t WHIRLPOOL_ROUND_CONSTANTS[32] = { |
||||
UINT64_C(0x4F01B887E8C62318), UINT64_C(0x52916F79F5D2A636), UINT64_C(0x357B0CA38E9BBC60), UINT64_C(0x57FE4B2EC2D7E01D), |
||||
UINT64_C(0xDA4AF09FE5377715), UINT64_C(0x856BA0B10A29C958), UINT64_C(0x67053ECBF4105DBD), UINT64_C(0xD8957DA78B4127E4), |
||||
UINT64_C(0x9E4717DD667CEEFB), UINT64_C(0x33835AAD07BF2DCA), UINT64_C(0xD94919C871AA0263), UINT64_C(0xB032269A885BE3F2), |
||||
UINT64_C(0x4834CDBE80D50FE9), UINT64_C(0xAE1A68205F907AFF), UINT64_C(0x1273F164229354B4), UINT64_C(0x3D8DA1DBECC30840), |
||||
UINT64_C(0x1BD682762BCF0097), UINT64_C(0xEF30F345506AAFB5), UINT64_C(0xC02FBA65EAA2553F), UINT64_C(0x8A0675924DFD1CDE), |
||||
UINT64_C(0x96A8D4621F0EE6B2), UINT64_C(0x4C3972845925C5F9), UINT64_C(0x61E2A5D18C38785E), UINT64_C(0x04FCC7431E9C21B3), |
||||
UINT64_C(0x247EDFFA0D6D9951), UINT64_C(0xEBB74E8F11CEAB3B), UINT64_C(0xD32C13B9F794813C), UINT64_C(0xA97F445603C46EE7), |
||||
UINT64_C(0x6C9D0BDC53C1BB2A), UINT64_C(0xE11489AC46F67431), UINT64_C(0xEDD0B67009693A16), UINT64_C(0x86F85C28A49842CC), |
||||
}; |
||||
|
||||
extern int whirlpoolx_test(unsigned char *pdata, const unsigned char *ptarget, uint32_t nonce); |
||||
extern void whirlpoolx_regenhash(struct work *work); |
||||
extern void whirlpool_round(uint64_t block[8], const uint64_t key[8]); |
||||
|
||||
#endif /* WHIRLPOOLX_H */ |
Loading…
Reference in new issue