mirror of https://github.com/GOSTSec/sgminer
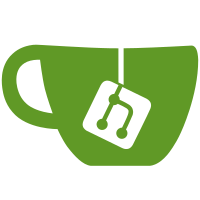
7 changed files with 398 additions and 3 deletions
@ -0,0 +1,232 @@ |
|||||||
|
/*-
|
||||||
|
* Copyright 2009 Colin Percival, 2014 savale |
||||||
|
* All rights reserved. |
||||||
|
* |
||||||
|
* Redistribution and use in source and binary forms, with or without |
||||||
|
* modification, are permitted provided that the following conditions |
||||||
|
* are met: |
||||||
|
* 1. Redistributions of source code must retain the above copyright |
||||||
|
* notice, this list of conditions and the following disclaimer. |
||||||
|
* 2. Redistributions in binary form must reproduce the above copyright |
||||||
|
* notice, this list of conditions and the following disclaimer in the |
||||||
|
* documentation and/or other materials provided with the distribution. |
||||||
|
* |
||||||
|
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR AND CONTRIBUTORS ``AS IS'' AND |
||||||
|
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE |
||||||
|
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE |
||||||
|
* ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS BE LIABLE |
||||||
|
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL |
||||||
|
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS |
||||||
|
* OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) |
||||||
|
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT |
||||||
|
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY |
||||||
|
* OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF |
||||||
|
* SUCH DAMAGE. |
||||||
|
* |
||||||
|
* This file was originally written by Colin Percival as part of the Tarsnap |
||||||
|
* online backup system. |
||||||
|
*/ |
||||||
|
|
||||||
|
#include "config.h" |
||||||
|
#include "miner.h" |
||||||
|
|
||||||
|
#include <stdlib.h> |
||||||
|
#include <stdint.h> |
||||||
|
#include <string.h> |
||||||
|
|
||||||
|
// Cyclic right rotation.
|
||||||
|
|
||||||
|
#ifndef ROTR64 |
||||||
|
#define ROTR64(x, y) (((x) >> (y)) ^ ((x) << (64 - (y)))) |
||||||
|
#endif |
||||||
|
|
||||||
|
// Little-endian byte access.
|
||||||
|
|
||||||
|
#define B2B_GET64(p) \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[0]) ^ \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[1]) << 8) ^ \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[2]) << 16) ^ \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[3]) << 24) ^ \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[4]) << 32) ^ \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[5]) << 40) ^ \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[6]) << 48) ^ \ |
||||||
|
(((uint64_t) ((uint8_t *) (p))[7]) << 56)) |
||||||
|
|
||||||
|
// G Mixing function.
|
||||||
|
|
||||||
|
#define B2B_G(a, b, c, d, x, y) { \ |
||||||
|
v[a] = v[a] + v[b] + x; \ |
||||||
|
v[d] = ROTR64(v[d] ^ v[a], 32); \ |
||||||
|
v[c] = v[c] + v[d]; \ |
||||||
|
v[b] = ROTR64(v[b] ^ v[c], 24); \ |
||||||
|
v[a] = v[a] + v[b] + y; \ |
||||||
|
v[d] = ROTR64(v[d] ^ v[a], 16); \ |
||||||
|
v[c] = v[c] + v[d]; \ |
||||||
|
v[b] = ROTR64(v[b] ^ v[c], 63); } |
||||||
|
|
||||||
|
// Initialization Vector.
|
||||||
|
|
||||||
|
static const uint64_t blake2b_iv[8] = { |
||||||
|
0x6A09E667F3BCC908, 0xBB67AE8584CAA73B, |
||||||
|
0x3C6EF372FE94F82B, 0xA54FF53A5F1D36F1, |
||||||
|
0x510E527FADE682D1, 0x9B05688C2B3E6C1F, |
||||||
|
0x1F83D9ABFB41BD6B, 0x5BE0CD19137E2179 |
||||||
|
}; |
||||||
|
|
||||||
|
// state context
|
||||||
|
typedef struct { |
||||||
|
uint8_t b[128]; // input buffer
|
||||||
|
uint64_t h[8]; // chained state
|
||||||
|
uint64_t t[2]; // total number of bytes
|
||||||
|
size_t c; // pointer for b[]
|
||||||
|
size_t outlen; // digest size
|
||||||
|
} blake2b_ctx; |
||||||
|
|
||||||
|
void blake2b_update(blake2b_ctx *ctx, // context
|
||||||
|
const void *in, size_t inlen); // data to be hashed
|
||||||
|
|
||||||
|
// Compression function. "last" flag indicates last block.
|
||||||
|
|
||||||
|
static void blake2b_compress(blake2b_ctx *ctx, int last) |
||||||
|
{ |
||||||
|
const uint8_t sigma[12][16] = { |
||||||
|
{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15 }, |
||||||
|
{ 14, 10, 4, 8, 9, 15, 13, 6, 1, 12, 0, 2, 11, 7, 5, 3 }, |
||||||
|
{ 11, 8, 12, 0, 5, 2, 15, 13, 10, 14, 3, 6, 7, 1, 9, 4 }, |
||||||
|
{ 7, 9, 3, 1, 13, 12, 11, 14, 2, 6, 5, 10, 4, 0, 15, 8 }, |
||||||
|
{ 9, 0, 5, 7, 2, 4, 10, 15, 14, 1, 11, 12, 6, 8, 3, 13 }, |
||||||
|
{ 2, 12, 6, 10, 0, 11, 8, 3, 4, 13, 7, 5, 15, 14, 1, 9 }, |
||||||
|
{ 12, 5, 1, 15, 14, 13, 4, 10, 0, 7, 6, 3, 9, 2, 8, 11 }, |
||||||
|
{ 13, 11, 7, 14, 12, 1, 3, 9, 5, 0, 15, 4, 8, 6, 2, 10 }, |
||||||
|
{ 6, 15, 14, 9, 11, 3, 0, 8, 12, 2, 13, 7, 1, 4, 10, 5 }, |
||||||
|
{ 10, 2, 8, 4, 7, 6, 1, 5, 15, 11, 9, 14, 3, 12, 13, 0 }, |
||||||
|
{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15 }, |
||||||
|
{ 14, 10, 4, 8, 9, 15, 13, 6, 1, 12, 0, 2, 11, 7, 5, 3 } |
||||||
|
}; |
||||||
|
int i; |
||||||
|
uint64_t v[16], m[16]; |
||||||
|
|
||||||
|
for (i = 0; i < 8; i++) { // init work variables
|
||||||
|
v[i] = ctx->h[i]; |
||||||
|
v[i + 8] = blake2b_iv[i]; |
||||||
|
} |
||||||
|
|
||||||
|
v[12] ^= ctx->t[0]; // low 64 bits of offset
|
||||||
|
v[13] ^= ctx->t[1]; // high 64 bits
|
||||||
|
if (last) // last block flag set ?
|
||||||
|
v[14] = ~v[14]; |
||||||
|
|
||||||
|
for (i = 0; i < 16; i++) // get little-endian words
|
||||||
|
m[i] = B2B_GET64(&ctx->b[8 * i]); |
||||||
|
|
||||||
|
for (i = 0; i < 12; i++) { // twelve rounds
|
||||||
|
B2B_G( 0, 4, 8, 12, m[sigma[i][ 0]], m[sigma[i][ 1]]); |
||||||
|
B2B_G( 1, 5, 9, 13, m[sigma[i][ 2]], m[sigma[i][ 3]]); |
||||||
|
B2B_G( 2, 6, 10, 14, m[sigma[i][ 4]], m[sigma[i][ 5]]); |
||||||
|
B2B_G( 3, 7, 11, 15, m[sigma[i][ 6]], m[sigma[i][ 7]]); |
||||||
|
B2B_G( 0, 5, 10, 15, m[sigma[i][ 8]], m[sigma[i][ 9]]); |
||||||
|
B2B_G( 1, 6, 11, 12, m[sigma[i][10]], m[sigma[i][11]]); |
||||||
|
B2B_G( 2, 7, 8, 13, m[sigma[i][12]], m[sigma[i][13]]); |
||||||
|
B2B_G( 3, 4, 9, 14, m[sigma[i][14]], m[sigma[i][15]]); |
||||||
|
} |
||||||
|
|
||||||
|
for( i = 0; i < 8; ++i ) |
||||||
|
ctx->h[i] ^= v[i] ^ v[i + 8]; |
||||||
|
} |
||||||
|
|
||||||
|
// Initialize the hashing context "ctx" with optional key "key".
|
||||||
|
// 1 <= outlen <= 64 gives the digest size in bytes.
|
||||||
|
// Secret key (also <= 64 bytes) is optional (keylen = 0).
|
||||||
|
|
||||||
|
int blake2b_init(blake2b_ctx *ctx, size_t outlen, |
||||||
|
const void *key, size_t keylen) // (keylen=0: no key)
|
||||||
|
{ |
||||||
|
size_t i; |
||||||
|
|
||||||
|
if (outlen == 0 || outlen > 64 || keylen > 64) |
||||||
|
return -1; // illegal parameters
|
||||||
|
|
||||||
|
for (i = 0; i < 8; i++) // state, "param block"
|
||||||
|
ctx->h[i] = blake2b_iv[i]; |
||||||
|
ctx->h[0] ^= 0x01010000 ^ (keylen << 8) ^ outlen; |
||||||
|
|
||||||
|
ctx->t[0] = 0; // input count low word
|
||||||
|
ctx->t[1] = 0; // input count high word
|
||||||
|
ctx->c = 0; // pointer within buffer
|
||||||
|
ctx->outlen = outlen; |
||||||
|
|
||||||
|
for (i = keylen; i < 128; i++) // zero input block
|
||||||
|
ctx->b[i] = 0; |
||||||
|
if (keylen > 0) { |
||||||
|
blake2b_update(ctx, key, keylen); |
||||||
|
ctx->c = 128; // at the end
|
||||||
|
} |
||||||
|
|
||||||
|
return 0; |
||||||
|
} |
||||||
|
|
||||||
|
// Add "inlen" bytes from "in" into the hash.
|
||||||
|
|
||||||
|
void blake2b_update(blake2b_ctx *ctx, |
||||||
|
const void *in, size_t inlen) // data bytes
|
||||||
|
{ |
||||||
|
size_t i; |
||||||
|
|
||||||
|
for (i = 0; i < inlen; i++) { |
||||||
|
if (ctx->c == 128) { // buffer full ?
|
||||||
|
ctx->t[0] += ctx->c; // add counters
|
||||||
|
if (ctx->t[0] < ctx->c) // carry overflow ?
|
||||||
|
ctx->t[1]++; // high word
|
||||||
|
blake2b_compress(ctx, 0); // compress (not last)
|
||||||
|
ctx->c = 0; // counter to zero
|
||||||
|
} |
||||||
|
ctx->b[ctx->c++] = ((const uint8_t *) in)[i]; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
// Generate the message digest (size given in init).
|
||||||
|
// Result placed in "out".
|
||||||
|
|
||||||
|
void blake2b_final(blake2b_ctx *ctx, void *out) |
||||||
|
{ |
||||||
|
size_t i; |
||||||
|
|
||||||
|
ctx->t[0] += ctx->c; // mark last block offset
|
||||||
|
if (ctx->t[0] < ctx->c) // carry overflow
|
||||||
|
ctx->t[1]++; // high word
|
||||||
|
|
||||||
|
while (ctx->c < 128) // fill up with zeros
|
||||||
|
ctx->b[ctx->c++] = 0; |
||||||
|
blake2b_compress(ctx, 1); // final block flag = 1
|
||||||
|
|
||||||
|
// little endian convert and store
|
||||||
|
for (i = 0; i < ctx->outlen; i++) { |
||||||
|
((uint8_t *) out)[i] = |
||||||
|
(ctx->h[i >> 3] >> (8 * (i & 7))) & 0xFF; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
#ifdef __APPLE_CC__ |
||||||
|
static |
||||||
|
#endif |
||||||
|
void siaHash(void *state, const void *input) |
||||||
|
{ |
||||||
|
blake2b_ctx ctx; |
||||||
|
blake2b_init(&ctx, 32, NULL, 0); |
||||||
|
blake2b_update(&ctx, input, 80); |
||||||
|
blake2b_final(&ctx, state); |
||||||
|
} |
||||||
|
|
||||||
|
void sia_regenhash(struct work *work) |
||||||
|
{ |
||||||
|
uint32_t data[20]; |
||||||
|
uint32_t hash[16]; |
||||||
|
char *scratchbuf; |
||||||
|
uint32_t *nonce = (uint32_t *)(work->data + 32); |
||||||
|
uint32_t *ohash = (uint32_t *)(work->hash); |
||||||
|
|
||||||
|
be32enc_vect(data, (const uint32_t *)work->data, 20); |
||||||
|
data[8] = htobe32(*nonce); |
||||||
|
siaHash(hash, data); |
||||||
|
swab256(ohash, hash); |
||||||
|
} |
@ -0,0 +1,8 @@ |
|||||||
|
#ifndef SIAH_H |
||||||
|
#define SIAH_H |
||||||
|
|
||||||
|
#include "miner.h" |
||||||
|
|
||||||
|
extern void sia_regenhash(struct work *work); |
||||||
|
|
||||||
|
#endif /* FRESHH_H */ |
@ -0,0 +1,120 @@ |
|||||||
|
|
||||||
|
#if __ENDIAN_LITTLE__ |
||||||
|
#define SPH_LITTLE_ENDIAN 1 |
||||||
|
#else |
||||||
|
#define SPH_BIG_ENDIAN 1 |
||||||
|
#endif |
||||||
|
|
||||||
|
#define SPH_UPTR sph_u64 |
||||||
|
|
||||||
|
typedef unsigned int sph_u32; |
||||||
|
typedef int sph_s32; |
||||||
|
#ifndef __OPENCL_VERSION__ |
||||||
|
typedef unsigned long long sph_u64; |
||||||
|
typedef long long sph_s64; |
||||||
|
#else |
||||||
|
typedef unsigned long sph_u64; |
||||||
|
typedef long sph_s64; |
||||||
|
#endif |
||||||
|
|
||||||
|
#define SPH_64 1 |
||||||
|
#define SPH_64_TRUE 1 |
||||||
|
|
||||||
|
#define SWAP4(x) as_uint(as_uchar4(x).wzyx) |
||||||
|
#define SWAP8(x) as_ulong(as_uchar8(x).s76543210) |
||||||
|
|
||||||
|
#if SPH_BIG_ENDIAN |
||||||
|
#define DEC64E(x) (x) |
||||||
|
#define DEC64BE(x) (*(const __global sph_u64 *) (x)); |
||||||
|
#define DEC32LE(x) SWAP4(*(const __global sph_u32 *) (x)); |
||||||
|
#else |
||||||
|
#define DEC64E(x) SWAP8(x) |
||||||
|
#define DEC64BE(x) SWAP8(*(const __global sph_u64 *) (x)); |
||||||
|
#define DEC64LE(x) (*(const __global sph_u64 *) (x)); |
||||||
|
#define DEC32LE(x) (*(const __global sph_u32 *) (x)); |
||||||
|
#endif |
||||||
|
|
||||||
|
inline static uint2 ror64(const uint2 x, const uint y) |
||||||
|
{ |
||||||
|
return (uint2)(((x).x>>y)^((x).y<<(32-y)),((x).y>>y)^((x).x<<(32-y))); |
||||||
|
} |
||||||
|
inline static uint2 ror64_2(const uint2 x, const uint y) |
||||||
|
{ |
||||||
|
return (uint2)(((x).y>>(y-32))^((x).x<<(64-y)),((x).x>>(y-32))^((x).y<<(64-y))); |
||||||
|
} |
||||||
|
__constant static const uchar blake2b_sigma[12][16] = { |
||||||
|
{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15 } , |
||||||
|
{ 14, 10, 4, 8, 9, 15, 13, 6, 1, 12, 0, 2, 11, 7, 5, 3 } , |
||||||
|
{ 11, 8, 12, 0, 5, 2, 15, 13, 10, 14, 3, 6, 7, 1, 9, 4 } , |
||||||
|
{ 7, 9, 3, 1, 13, 12, 11, 14, 2, 6, 5, 10, 4, 0, 15, 8 } , |
||||||
|
{ 9, 0, 5, 7, 2, 4, 10, 15, 14, 1, 11, 12, 6, 8, 3, 13 } , |
||||||
|
{ 2, 12, 6, 10, 0, 11, 8, 3, 4, 13, 7, 5, 15, 14, 1, 9 } , |
||||||
|
{ 12, 5, 1, 15, 14, 13, 4, 10, 0, 7, 6, 3, 9, 2, 8, 11 } , |
||||||
|
{ 13, 11, 7, 14, 12, 1, 3, 9, 5, 0, 15, 4, 8, 6, 2, 10 } , |
||||||
|
{ 6, 15, 14, 9, 11, 3, 0, 8, 12, 2, 13, 7, 1, 4, 10, 5 } , |
||||||
|
{ 10, 2, 8, 4, 7, 6, 1, 5, 15, 11, 9, 14, 3, 12, 13, 0 } , |
||||||
|
{ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15 } , |
||||||
|
{ 14, 10, 4, 8, 9, 15, 13, 6, 1, 12, 0, 2, 11, 7, 5, 3 } }; |
||||||
|
|
||||||
|
__kernel void search(__global unsigned char* block, volatile __global uint* output, const ulong target) { |
||||||
|
sph_u32 gid = get_global_id(0); |
||||||
|
|
||||||
|
ulong m[16]; |
||||||
|
m[0] = DEC64LE(block + 0); |
||||||
|
m[1] = DEC64LE(block + 8); |
||||||
|
m[2] = DEC64LE(block + 16); |
||||||
|
m[3] = DEC64LE(block + 24); |
||||||
|
m[4] = DEC64LE(block + 32); |
||||||
|
m[4] &= 0xFFFFFFFF00000000; |
||||||
|
m[4] ^= (gid); |
||||||
|
m[5] = DEC64LE(block + 40); |
||||||
|
m[6] = DEC64LE(block + 48); |
||||||
|
m[7] = DEC64LE(block + 56); |
||||||
|
m[8] = DEC64LE(block + 64); |
||||||
|
m[9] = DEC64LE(block + 72); |
||||||
|
m[10] = m[11] = m[12] = m[13] = m[14] = m[15] = 0; |
||||||
|
|
||||||
|
ulong v[16] = { 0x6a09e667f2bdc928, 0xbb67ae8584caa73b, 0x3c6ef372fe94f82b, 0xa54ff53a5f1d36f1, |
||||||
|
0x510e527fade682d1, 0x9b05688c2b3e6c1f, 0x1f83d9abfb41bd6b, 0x5be0cd19137e2179, |
||||||
|
0x6a09e667f3bcc908, 0xbb67ae8584caa73b, 0x3c6ef372fe94f82b, 0xa54ff53a5f1d36f1, |
||||||
|
0x510e527fade68281, 0x9b05688c2b3e6c1f, 0xe07c265404be4294, 0x5be0cd19137e2179 }; |
||||||
|
|
||||||
|
#define G(r,i,a,b,c,d) \ |
||||||
|
a = a + b + m[ blake2b_sigma[r][2*i] ]; \ |
||||||
|
((uint2*)&d)[0] = ((uint2*)&d)[0].yx ^ ((uint2*)&a)[0].yx; \ |
||||||
|
c = c + d; \ |
||||||
|
((uint2*)&b)[0] = ror64( ((uint2*)&b)[0] ^ ((uint2*)&c)[0], 24U); \ |
||||||
|
a = a + b + m[ blake2b_sigma[r][2*i+1] ]; \ |
||||||
|
((uint2*)&d)[0] = ror64( ((uint2*)&d)[0] ^ ((uint2*)&a)[0], 16U); \ |
||||||
|
c = c + d; \ |
||||||
|
((uint2*)&b)[0] = ror64_2( ((uint2*)&b)[0] ^ ((uint2*)&c)[0], 63U); |
||||||
|
|
||||||
|
#define ROUND(r) \ |
||||||
|
G(r,0,v[ 0],v[ 4],v[ 8],v[12]); \ |
||||||
|
G(r,1,v[ 1],v[ 5],v[ 9],v[13]); \ |
||||||
|
G(r,2,v[ 2],v[ 6],v[10],v[14]); \ |
||||||
|
G(r,3,v[ 3],v[ 7],v[11],v[15]); \ |
||||||
|
G(r,4,v[ 0],v[ 5],v[10],v[15]); \ |
||||||
|
G(r,5,v[ 1],v[ 6],v[11],v[12]); \ |
||||||
|
G(r,6,v[ 2],v[ 7],v[ 8],v[13]); \ |
||||||
|
G(r,7,v[ 3],v[ 4],v[ 9],v[14]); |
||||||
|
ROUND( 0 ); |
||||||
|
ROUND( 1 ); |
||||||
|
ROUND( 2 ); |
||||||
|
ROUND( 3 ); |
||||||
|
ROUND( 4 ); |
||||||
|
ROUND( 5 ); |
||||||
|
ROUND( 6 ); |
||||||
|
ROUND( 7 ); |
||||||
|
ROUND( 8 ); |
||||||
|
ROUND( 9 ); |
||||||
|
ROUND( 10 ); |
||||||
|
ROUND( 11 ); |
||||||
|
|
||||||
|
#undef G |
||||||
|
#undef ROUND |
||||||
|
|
||||||
|
bool result = (SWAP8(0x6a09e667f2bdc928 ^ v[0] ^ v[8]) <= target); |
||||||
|
if (result) |
||||||
|
output[output[0xFF]++] = SWAP4(gid); |
||||||
|
} |
Loading…
Reference in new issue