mirror of https://github.com/GOSTSec/sgminer
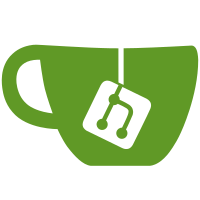
9 changed files with 158 additions and 263 deletions
@ -1,162 +0,0 @@
@@ -1,162 +0,0 @@
|
||||
#! /bin/sh |
||||
# mkinstalldirs --- make directory hierarchy |
||||
|
||||
scriptversion=2009-04-28.21; # UTC |
||||
|
||||
# Original author: Noah Friedman <friedman@prep.ai.mit.edu> |
||||
# Created: 1993-05-16 |
||||
# Public domain. |
||||
# |
||||
# This file is maintained in Automake, please report |
||||
# bugs to <bug-automake@gnu.org> or send patches to |
||||
# <automake-patches@gnu.org>. |
||||
|
||||
nl=' |
||||
' |
||||
IFS=" "" $nl" |
||||
errstatus=0 |
||||
dirmode= |
||||
|
||||
usage="\ |
||||
Usage: mkinstalldirs [-h] [--help] [--version] [-m MODE] DIR ... |
||||
|
||||
Create each directory DIR (with mode MODE, if specified), including all |
||||
leading file name components. |
||||
|
||||
Report bugs to <bug-automake@gnu.org>." |
||||
|
||||
# process command line arguments |
||||
while test $# -gt 0 ; do |
||||
case $1 in |
||||
-h | --help | --h*) # -h for help |
||||
echo "$usage" |
||||
exit $? |
||||
;; |
||||
-m) # -m PERM arg |
||||
shift |
||||
test $# -eq 0 && { echo "$usage" 1>&2; exit 1; } |
||||
dirmode=$1 |
||||
shift |
||||
;; |
||||
--version) |
||||
echo "$0 $scriptversion" |
||||
exit $? |
||||
;; |
||||
--) # stop option processing |
||||
shift |
||||
break |
||||
;; |
||||
-*) # unknown option |
||||
echo "$usage" 1>&2 |
||||
exit 1 |
||||
;; |
||||
*) # first non-opt arg |
||||
break |
||||
;; |
||||
esac |
||||
done |
||||
|
||||
for file |
||||
do |
||||
if test -d "$file"; then |
||||
shift |
||||
else |
||||
break |
||||
fi |
||||
done |
||||
|
||||
case $# in |
||||
0) exit 0 ;; |
||||
esac |
||||
|
||||
# Solaris 8's mkdir -p isn't thread-safe. If you mkdir -p a/b and |
||||
# mkdir -p a/c at the same time, both will detect that a is missing, |
||||
# one will create a, then the other will try to create a and die with |
||||
# a "File exists" error. This is a problem when calling mkinstalldirs |
||||
# from a parallel make. We use --version in the probe to restrict |
||||
# ourselves to GNU mkdir, which is thread-safe. |
||||
case $dirmode in |
||||
'') |
||||
if mkdir -p --version . >/dev/null 2>&1 && test ! -d ./--version; then |
||||
echo "mkdir -p -- $*" |
||||
exec mkdir -p -- "$@" |
||||
else |
||||
# On NextStep and OpenStep, the 'mkdir' command does not |
||||
# recognize any option. It will interpret all options as |
||||
# directories to create, and then abort because '.' already |
||||
# exists. |
||||
test -d ./-p && rmdir ./-p |
||||
test -d ./--version && rmdir ./--version |
||||
fi |
||||
;; |
||||
*) |
||||
if mkdir -m "$dirmode" -p --version . >/dev/null 2>&1 && |
||||
test ! -d ./--version; then |
||||
echo "mkdir -m $dirmode -p -- $*" |
||||
exec mkdir -m "$dirmode" -p -- "$@" |
||||
else |
||||
# Clean up after NextStep and OpenStep mkdir. |
||||
for d in ./-m ./-p ./--version "./$dirmode"; |
||||
do |
||||
test -d $d && rmdir $d |
||||
done |
||||
fi |
||||
;; |
||||
esac |
||||
|
||||
for file |
||||
do |
||||
case $file in |
||||
/*) pathcomp=/ ;; |
||||
*) pathcomp= ;; |
||||
esac |
||||
oIFS=$IFS |
||||
IFS=/ |
||||
set fnord $file |
||||
shift |
||||
IFS=$oIFS |
||||
|
||||
for d |
||||
do |
||||
test "x$d" = x && continue |
||||
|
||||
pathcomp=$pathcomp$d |
||||
case $pathcomp in |
||||
-*) pathcomp=./$pathcomp ;; |
||||
esac |
||||
|
||||
if test ! -d "$pathcomp"; then |
||||
echo "mkdir $pathcomp" |
||||
|
||||
mkdir "$pathcomp" || lasterr=$? |
||||
|
||||
if test ! -d "$pathcomp"; then |
||||
errstatus=$lasterr |
||||
else |
||||
if test ! -z "$dirmode"; then |
||||
echo "chmod $dirmode $pathcomp" |
||||
lasterr= |
||||
chmod "$dirmode" "$pathcomp" || lasterr=$? |
||||
|
||||
if test ! -z "$lasterr"; then |
||||
errstatus=$lasterr |
||||
fi |
||||
fi |
||||
fi |
||||
fi |
||||
|
||||
pathcomp=$pathcomp/ |
||||
done |
||||
done |
||||
|
||||
exit $errstatus |
||||
|
||||
# Local Variables: |
||||
# mode: shell-script |
||||
# sh-indentation: 2 |
||||
# eval: (add-hook 'write-file-hooks 'time-stamp) |
||||
# time-stamp-start: "scriptversion=" |
||||
# time-stamp-format: "%:y-%02m-%02d.%02H" |
||||
# time-stamp-time-zone: "UTC" |
||||
# time-stamp-end: "; # UTC" |
||||
# End: |
Loading…
Reference in new issue