mirror of https://github.com/GOSTSec/sgminer
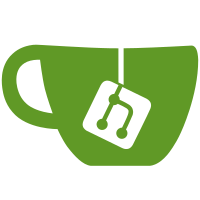
15 changed files with 5794 additions and 1269 deletions
@ -0,0 +1,398 @@ |
|||||||
|
#ifndef API_H |
||||||
|
#define API_H |
||||||
|
|
||||||
|
#include "config.h" |
||||||
|
|
||||||
|
#include "miner.h" |
||||||
|
|
||||||
|
// BUFSIZ varies on Windows and Linux
|
||||||
|
#define TMPBUFSIZ 8192 |
||||||
|
|
||||||
|
// Number of requests to queue - normally would be small
|
||||||
|
#define QUEUE 100 |
||||||
|
|
||||||
|
#ifdef WIN32 |
||||||
|
struct WSAERRORS { |
||||||
|
int id; |
||||||
|
char *code; |
||||||
|
} WSAErrors[] = { |
||||||
|
{ 0, "No error" }, |
||||||
|
{ WSAEINTR, "Interrupted system call" }, |
||||||
|
{ WSAEBADF, "Bad file number" }, |
||||||
|
{ WSAEACCES, "Permission denied" }, |
||||||
|
{ WSAEFAULT, "Bad address" }, |
||||||
|
{ WSAEINVAL, "Invalid argument" }, |
||||||
|
{ WSAEMFILE, "Too many open sockets" }, |
||||||
|
{ WSAEWOULDBLOCK, "Operation would block" }, |
||||||
|
{ WSAEINPROGRESS, "Operation now in progress" }, |
||||||
|
{ WSAEALREADY, "Operation already in progress" }, |
||||||
|
{ WSAENOTSOCK, "Socket operation on non-socket" }, |
||||||
|
{ WSAEDESTADDRREQ, "Destination address required" }, |
||||||
|
{ WSAEMSGSIZE, "Message too long" }, |
||||||
|
{ WSAEPROTOTYPE, "Protocol wrong type for socket" }, |
||||||
|
{ WSAENOPROTOOPT, "Bad protocol option" }, |
||||||
|
{ WSAEPROTONOSUPPORT, "Protocol not supported" }, |
||||||
|
{ WSAESOCKTNOSUPPORT, "Socket type not supported" }, |
||||||
|
{ WSAEOPNOTSUPP, "Operation not supported on socket" }, |
||||||
|
{ WSAEPFNOSUPPORT, "Protocol family not supported" }, |
||||||
|
{ WSAEAFNOSUPPORT, "Address family not supported" }, |
||||||
|
{ WSAEADDRINUSE, "Address already in use" }, |
||||||
|
{ WSAEADDRNOTAVAIL, "Can't assign requested address" }, |
||||||
|
{ WSAENETDOWN, "Network is down" }, |
||||||
|
{ WSAENETUNREACH, "Network is unreachable" }, |
||||||
|
{ WSAENETRESET, "Net connection reset" }, |
||||||
|
{ WSAECONNABORTED, "Software caused connection abort" }, |
||||||
|
{ WSAECONNRESET, "Connection reset by peer" }, |
||||||
|
{ WSAENOBUFS, "No buffer space available" }, |
||||||
|
{ WSAEISCONN, "Socket is already connected" }, |
||||||
|
{ WSAENOTCONN, "Socket is not connected" }, |
||||||
|
{ WSAESHUTDOWN, "Can't send after socket shutdown" }, |
||||||
|
{ WSAETOOMANYREFS, "Too many references, can't splice" }, |
||||||
|
{ WSAETIMEDOUT, "Connection timed out" }, |
||||||
|
{ WSAECONNREFUSED, "Connection refused" }, |
||||||
|
{ WSAELOOP, "Too many levels of symbolic links" }, |
||||||
|
{ WSAENAMETOOLONG, "File name too long" }, |
||||||
|
{ WSAEHOSTDOWN, "Host is down" }, |
||||||
|
{ WSAEHOSTUNREACH, "No route to host" }, |
||||||
|
{ WSAENOTEMPTY, "Directory not empty" }, |
||||||
|
{ WSAEPROCLIM, "Too many processes" }, |
||||||
|
{ WSAEUSERS, "Too many users" }, |
||||||
|
{ WSAEDQUOT, "Disc quota exceeded" }, |
||||||
|
{ WSAESTALE, "Stale NFS file handle" }, |
||||||
|
{ WSAEREMOTE, "Too many levels of remote in path" }, |
||||||
|
{ WSASYSNOTREADY, "Network system is unavailable" }, |
||||||
|
{ WSAVERNOTSUPPORTED, "Winsock version out of range" }, |
||||||
|
{ WSANOTINITIALISED, "WSAStartup not yet called" }, |
||||||
|
{ WSAEDISCON, "Graceful shutdown in progress" }, |
||||||
|
{ WSAHOST_NOT_FOUND, "Host not found" }, |
||||||
|
{ WSANO_DATA, "No host data of that type was found" }, |
||||||
|
{ -1, "Unknown error code" } |
||||||
|
}; |
||||||
|
#endif |
||||||
|
|
||||||
|
#define COMSTR "," |
||||||
|
#define SEPSTR "|" |
||||||
|
|
||||||
|
#define CMDJOIN '+' |
||||||
|
#define JOIN_CMD "CMD=" |
||||||
|
#define BETWEEN_JOIN SEPSTR |
||||||
|
#define _DYNAMIC "D" |
||||||
|
|
||||||
|
#define _DEVS "DEVS" |
||||||
|
#define _POOLS "POOLS" |
||||||
|
#define _PROFILES "PROFILES" |
||||||
|
#define _SUMMARY "SUMMARY" |
||||||
|
#define _STATUS "STATUS" |
||||||
|
#define _VERSION "VERSION" |
||||||
|
#define _MINECONFIG "CONFIG" |
||||||
|
#define _GPU "GPU" |
||||||
|
|
||||||
|
#define _GPUS "GPUS" |
||||||
|
#define _NOTIFY "NOTIFY" |
||||||
|
#define _DEVDETAILS "DEVDETAILS" |
||||||
|
#define _BYE "BYE" |
||||||
|
#define _RESTART "RESTART" |
||||||
|
#define _MINESTATS "STATS" |
||||||
|
#define _CHECK "CHECK" |
||||||
|
#define _MINECOIN "COIN" |
||||||
|
#define _DEBUGSET "DEBUG" |
||||||
|
#define _SETCONFIG "SETCONFIG" |
||||||
|
|
||||||
|
#define JSON0 "{" |
||||||
|
#define JSON1 "\"" |
||||||
|
#define JSON2 "\":[" |
||||||
|
#define JSON3 "]" |
||||||
|
#define JSON4 ",\"id\":1" |
||||||
|
// If anyone cares, id=0 for truncated output
|
||||||
|
#define JSON4_TRUNCATED ",\"id\":0" |
||||||
|
#define JSON5 "}" |
||||||
|
|
||||||
|
#define JSON_START JSON0 |
||||||
|
#define JSON_DEVS JSON1 _DEVS JSON2 |
||||||
|
#define JSON_POOLS JSON1 _POOLS JSON2 |
||||||
|
#define JSON_PROFILES JSON1 _POOLS JSON2 |
||||||
|
#define JSON_SUMMARY JSON1 _SUMMARY JSON2 |
||||||
|
#define JSON_STATUS JSON1 _STATUS JSON2 |
||||||
|
#define JSON_VERSION JSON1 _VERSION JSON2 |
||||||
|
#define JSON_MINECONFIG JSON1 _MINECONFIG JSON2 |
||||||
|
#define JSON_GPU JSON1 _GPU JSON2 |
||||||
|
|
||||||
|
#define JSON_GPUS JSON1 _GPUS JSON2 |
||||||
|
#define JSON_NOTIFY JSON1 _NOTIFY JSON2 |
||||||
|
#define JSON_DEVDETAILS JSON1 _DEVDETAILS JSON2 |
||||||
|
#define JSON_CLOSE JSON3 |
||||||
|
#define JSON_MINESTATS JSON1 _MINESTATS JSON2 |
||||||
|
#define JSON_CHECK JSON1 _CHECK JSON2 |
||||||
|
#define JSON_MINECOIN JSON1 _MINECOIN JSON2 |
||||||
|
#define JSON_DEBUGSET JSON1 _DEBUGSET JSON2 |
||||||
|
#define JSON_SETCONFIG JSON1 _SETCONFIG JSON2 |
||||||
|
|
||||||
|
#define JSON_END JSON4 JSON5 |
||||||
|
#define JSON_END_TRUNCATED JSON4_TRUNCATED JSON5 |
||||||
|
#define JSON_BETWEEN_JOIN "," |
||||||
|
|
||||||
|
#define MSG_INVGPU 1 |
||||||
|
#define MSG_ALRENA 2 |
||||||
|
#define MSG_ALRDIS 3 |
||||||
|
#define MSG_GPUMRE 4 |
||||||
|
#define MSG_GPUREN 5 |
||||||
|
#define MSG_GPUNON 6 |
||||||
|
#define MSG_POOL 7 |
||||||
|
#define MSG_NOPOOL 8 |
||||||
|
#define MSG_DEVS 9 |
||||||
|
#define MSG_NODEVS 10 |
||||||
|
#define MSG_SUMM 11 |
||||||
|
#define MSG_GPUDIS 12 |
||||||
|
#define MSG_GPUREI 13 |
||||||
|
#define MSG_INVCMD 14 |
||||||
|
#define MSG_MISID 15 |
||||||
|
#define MSG_GPUDEV 17 |
||||||
|
|
||||||
|
#define MSG_NUMGPU 20 |
||||||
|
|
||||||
|
#define MSG_VERSION 22 |
||||||
|
#define MSG_INVJSON 23 |
||||||
|
#define MSG_MISCMD 24 |
||||||
|
#define MSG_MISPID 25 |
||||||
|
#define MSG_INVPID 26 |
||||||
|
#define MSG_SWITCHP 27 |
||||||
|
#define MSG_MISVAL 28 |
||||||
|
#define MSG_NOADL 29 |
||||||
|
#define MSG_NOGPUADL 30 |
||||||
|
#define MSG_INVINT 31 |
||||||
|
#define MSG_GPUINT 32 |
||||||
|
#define MSG_MINECONFIG 33 |
||||||
|
#define MSG_GPUMERR 34 |
||||||
|
#define MSG_GPUMEM 35 |
||||||
|
#define MSG_GPUEERR 36 |
||||||
|
#define MSG_GPUENG 37 |
||||||
|
#define MSG_GPUVERR 38 |
||||||
|
#define MSG_GPUVDDC 39 |
||||||
|
#define MSG_GPUFERR 40 |
||||||
|
#define MSG_GPUFAN 41 |
||||||
|
#define MSG_MISFN 42 |
||||||
|
#define MSG_BADFN 43 |
||||||
|
#define MSG_SAVED 44 |
||||||
|
#define MSG_ACCDENY 45 |
||||||
|
#define MSG_ACCOK 46 |
||||||
|
#define MSG_ENAPOOL 47 |
||||||
|
#define MSG_DISPOOL 48 |
||||||
|
#define MSG_ALRENAP 49 |
||||||
|
#define MSG_ALRDISP 50 |
||||||
|
#define MSG_MISPDP 52 |
||||||
|
#define MSG_INVPDP 53 |
||||||
|
#define MSG_TOOMANYP 54 |
||||||
|
#define MSG_ADDPOOL 55 |
||||||
|
|
||||||
|
#define MSG_NOTIFY 60 |
||||||
|
|
||||||
|
#define MSG_REMLASTP 66 |
||||||
|
#define MSG_ACTPOOL 67 |
||||||
|
#define MSG_REMPOOL 68 |
||||||
|
#define MSG_DEVDETAILS 69 |
||||||
|
#define MSG_MINESTATS 70 |
||||||
|
#define MSG_MISCHK 71 |
||||||
|
#define MSG_CHECK 72 |
||||||
|
#define MSG_POOLPRIO 73 |
||||||
|
#define MSG_DUPPID 74 |
||||||
|
#define MSG_MISBOOL 75 |
||||||
|
#define MSG_INVBOOL 76 |
||||||
|
#define MSG_FOO 77 |
||||||
|
#define MSG_MINECOIN 78 |
||||||
|
#define MSG_DEBUGSET 79 |
||||||
|
#define MSG_SETCONFIG 82 |
||||||
|
#define MSG_UNKCON 83 |
||||||
|
#define MSG_INVNUM 84 |
||||||
|
#define MSG_CONPAR 85 |
||||||
|
#define MSG_CONVAL 86 |
||||||
|
|
||||||
|
#define MSG_NOUSTA 88 |
||||||
|
|
||||||
|
#define MSG_ZERMIS 94 |
||||||
|
#define MSG_ZERINV 95 |
||||||
|
#define MSG_ZERSUM 96 |
||||||
|
#define MSG_ZERNOSUM 97 |
||||||
|
|
||||||
|
#define MSG_BYE 0x101 |
||||||
|
|
||||||
|
#define MSG_INVNEG 121 |
||||||
|
#define MSG_SETQUOTA 122 |
||||||
|
#define MSG_LOCKOK 123 |
||||||
|
#define MSG_LOCKDIS 124 |
||||||
|
|
||||||
|
#define MSG_CHSTRAT 125 |
||||||
|
#define MSG_MISSTRAT 126 |
||||||
|
#define MSG_INVSTRAT 127 |
||||||
|
#define MSG_MISSTRATINT 128 |
||||||
|
|
||||||
|
#define MSG_PROFILE 129 |
||||||
|
#define MSG_NOPROFILE 130 |
||||||
|
|
||||||
|
#define MSG_PROFILEEXIST 131 |
||||||
|
#define MSG_MISPRD 132 |
||||||
|
#define MSG_ADDPROFILE 133 |
||||||
|
|
||||||
|
#define MSG_MISPRID 134 |
||||||
|
#define MSG_PRNOEXIST 135 |
||||||
|
#define MSG_PRISDEFAULT 136 |
||||||
|
#define MSG_PRINUSE 137 |
||||||
|
#define MSG_REMPROFILE 138 |
||||||
|
|
||||||
|
#define MSG_CHPOOLPR 139 |
||||||
|
|
||||||
|
enum code_severity { |
||||||
|
SEVERITY_ERR, |
||||||
|
SEVERITY_WARN, |
||||||
|
SEVERITY_INFO, |
||||||
|
SEVERITY_SUCC, |
||||||
|
SEVERITY_FAIL |
||||||
|
}; |
||||||
|
|
||||||
|
enum code_parameters { |
||||||
|
PARAM_GPU, |
||||||
|
PARAM_PID, |
||||||
|
PARAM_GPUMAX, |
||||||
|
PARAM_PMAX, |
||||||
|
PARAM_PRMAX, |
||||||
|
PARAM_POOLMAX, |
||||||
|
|
||||||
|
// Single generic case: have the code resolve it - see below
|
||||||
|
PARAM_DMAX, |
||||||
|
|
||||||
|
PARAM_CMD, |
||||||
|
PARAM_POOL, |
||||||
|
PARAM_STR, |
||||||
|
PARAM_BOTH, |
||||||
|
PARAM_BOOL, |
||||||
|
PARAM_SET, |
||||||
|
PARAM_INT, |
||||||
|
PARAM_NONE |
||||||
|
}; |
||||||
|
|
||||||
|
struct CODES { |
||||||
|
const enum code_severity severity; |
||||||
|
const int code; |
||||||
|
const enum code_parameters params; |
||||||
|
const char *description; |
||||||
|
}; |
||||||
|
|
||||||
|
extern struct CODES codes[]; |
||||||
|
|
||||||
|
struct IP4ACCESS { |
||||||
|
in_addr_t ip; |
||||||
|
in_addr_t mask; |
||||||
|
char group; |
||||||
|
}; |
||||||
|
|
||||||
|
#define GROUP(g) (toupper(g)) |
||||||
|
#define PRIVGROUP GROUP('W') |
||||||
|
#define NOPRIVGROUP GROUP('R') |
||||||
|
#define ISPRIVGROUP(g) (GROUP(g) == PRIVGROUP) |
||||||
|
#define GROUPOFFSET(g) (GROUP(g) - GROUP('A')) |
||||||
|
#define VALIDGROUP(g) (GROUP(g) >= GROUP('A') && GROUP(g) <= GROUP('Z')) |
||||||
|
#define COMMANDS(g) (apigroups[GROUPOFFSET(g)].commands) |
||||||
|
#define DEFINEDGROUP(g) (ISPRIVGROUP(g) || COMMANDS(g) != NULL) |
||||||
|
|
||||||
|
struct APIGROUPS { |
||||||
|
// This becomes a string like: "|cmd1|cmd2|cmd3|" so it's quick to search
|
||||||
|
char *commands; |
||||||
|
} apigroups['Z' - 'A' + 1]; // only A=0 to Z=25 (R: noprivs, W: allprivs)
|
||||||
|
|
||||||
|
struct io_data { |
||||||
|
size_t siz; |
||||||
|
char *ptr; |
||||||
|
char *cur; |
||||||
|
bool sock; |
||||||
|
bool close; |
||||||
|
}; |
||||||
|
|
||||||
|
struct io_list { |
||||||
|
struct io_data *io_data; |
||||||
|
struct io_list *prev; |
||||||
|
struct io_list *next; |
||||||
|
}; |
||||||
|
|
||||||
|
extern void message(struct io_data *io_data, int messageid, int paramid, char *param2, bool isjson); |
||||||
|
extern bool io_add(struct io_data *io_data, char *buf); |
||||||
|
extern void io_close(struct io_data *io_data); |
||||||
|
extern void io_free(); |
||||||
|
|
||||||
|
extern struct api_data *api_add_escape(struct api_data *root, char *name, char *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_string(struct api_data *root, char *name, char *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_const(struct api_data *root, char *name, const char *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_uint8(struct api_data *root, char *name, uint8_t *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_uint16(struct api_data *root, char *name, uint16_t *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_int(struct api_data *root, char *name, int *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_uint(struct api_data *root, char *name, unsigned int *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_uint32(struct api_data *root, char *name, uint32_t *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_hex32(struct api_data *root, char *name, uint32_t *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_uint64(struct api_data *root, char *name, uint64_t *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_double(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_elapsed(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_bool(struct api_data *root, char *name, bool *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_timeval(struct api_data *root, char *name, struct timeval *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_time(struct api_data *root, char *name, time_t *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_mhs(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_khs(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_mhtotal(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_temp(struct api_data *root, char *name, float *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_utility(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_freq(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_volts(struct api_data *root, char *name, float *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_hs(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_diff(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_percent(struct api_data *root, char *name, double *data, bool copy_data); |
||||||
|
extern struct api_data *api_add_avg(struct api_data *root, char *name, float *data, bool copy_data); |
||||||
|
extern struct api_data *print_data(struct api_data *root, char *buf, bool isjson, bool precom); |
||||||
|
|
||||||
|
#define SOCKBUFALLOCSIZ 65536 |
||||||
|
|
||||||
|
#define io_new(init) _io_new(init, false) |
||||||
|
#define sock_io_new() _io_new(SOCKBUFALLOCSIZ, true) |
||||||
|
|
||||||
|
#if LOCK_TRACKING |
||||||
|
|
||||||
|
#define LOCK_FMT_FFL " - called from %s %s():%d" |
||||||
|
#define LOCKMSG(fmt, ...) fprintf(stderr, "APILOCK: " fmt "\n", ##__VA_ARGS__) |
||||||
|
#define LOCKMSGMORE(fmt, ...) fprintf(stderr, " " fmt "\n", ##__VA_ARGS__) |
||||||
|
#define LOCKMSGFFL(fmt, ...) fprintf(stderr, "APILOCK: " fmt LOCK_FMT_FFL "\n", ##__VA_ARGS__, file, func, linenum) |
||||||
|
#define LOCKMSGFLUSH() fflush(stderr) |
||||||
|
|
||||||
|
typedef struct lockstat { |
||||||
|
uint64_t lock_id; |
||||||
|
const char *file; |
||||||
|
const char *func; |
||||||
|
int linenum; |
||||||
|
struct timeval tv; |
||||||
|
} LOCKSTAT; |
||||||
|
|
||||||
|
typedef struct lockline { |
||||||
|
struct lockline *prev; |
||||||
|
struct lockstat *stat; |
||||||
|
struct lockline *next; |
||||||
|
} LOCKLINE; |
||||||
|
|
||||||
|
typedef struct lockinfo { |
||||||
|
void *lock; |
||||||
|
enum cglock_typ typ; |
||||||
|
const char *file; |
||||||
|
const char *func; |
||||||
|
int linenum; |
||||||
|
uint64_t gets; |
||||||
|
uint64_t gots; |
||||||
|
uint64_t tries; |
||||||
|
uint64_t dids; |
||||||
|
uint64_t didnts; // should be tries - dids
|
||||||
|
uint64_t unlocks; |
||||||
|
LOCKSTAT lastgot; |
||||||
|
LOCKLINE *lockgets; |
||||||
|
LOCKLINE *locktries; |
||||||
|
} LOCKINFO; |
||||||
|
|
||||||
|
typedef struct locklist { |
||||||
|
LOCKINFO *info; |
||||||
|
struct locklist *next; |
||||||
|
} LOCKLIST; |
||||||
|
#endif |
||||||
|
|
||||||
|
#endif /* API_H */ |
@ -0,0 +1,122 @@ |
|||||||
|
#ifndef CONFIG_PARSER_H |
||||||
|
#define CONFIG_PARSER_H |
||||||
|
|
||||||
|
#include "config.h" |
||||||
|
|
||||||
|
#include "miner.h" |
||||||
|
#include "api.h" |
||||||
|
#include "algorithm.h" |
||||||
|
|
||||||
|
//helper to check for empty or NULL strings
|
||||||
|
#ifndef empty_string |
||||||
|
#define empty_string(str) ((str && str[0] != '\0')?0:1) |
||||||
|
#endif |
||||||
|
#ifndef safe_cmp |
||||||
|
#define safe_cmp(val1, val2) (((val1 && strcmp(val1, val2) != 0) || empty_string(val1))?1:0) |
||||||
|
#endif |
||||||
|
#ifndef isnull |
||||||
|
#define isnull(str, default_str) ((str == NULL)?default_str:str) |
||||||
|
#endif |
||||||
|
|
||||||
|
//helper function to get a gpu option value
|
||||||
|
#ifndef gpu_opt |
||||||
|
#define gpu_opt(i,optname) gpus[i].optname |
||||||
|
#endif |
||||||
|
|
||||||
|
//profile structure
|
||||||
|
struct profile { |
||||||
|
int profile_no; |
||||||
|
char *name; |
||||||
|
bool removed; |
||||||
|
|
||||||
|
algorithm_t algorithm; |
||||||
|
const char *devices; |
||||||
|
const char *intensity; |
||||||
|
const char *xintensity; |
||||||
|
const char *rawintensity; |
||||||
|
const char *lookup_gap; |
||||||
|
const char *gpu_engine; |
||||||
|
const char *gpu_memclock; |
||||||
|
const char *gpu_threads; |
||||||
|
const char *gpu_fan; |
||||||
|
const char *gpu_powertune; |
||||||
|
const char *gpu_vddc; |
||||||
|
const char *shaders; |
||||||
|
const char *thread_concurrency; |
||||||
|
const char *worksize; |
||||||
|
}; |
||||||
|
|
||||||
|
/* globals needed outside */ |
||||||
|
extern char *cnfbuf; |
||||||
|
extern int fileconf_load; |
||||||
|
extern const char def_conf[]; |
||||||
|
extern char *default_config; |
||||||
|
extern bool config_loaded; |
||||||
|
|
||||||
|
extern int json_array_index; |
||||||
|
|
||||||
|
extern struct profile default_profile; |
||||||
|
extern struct profile **profiles; |
||||||
|
extern int total_profiles; |
||||||
|
|
||||||
|
/* option parser functions */ |
||||||
|
extern char *set_default_devices(const char *arg); |
||||||
|
extern char *set_default_lookup_gap(const char *arg); |
||||||
|
extern char *set_default_intensity(const char *arg); |
||||||
|
extern char *set_default_xintensity(const char *arg); |
||||||
|
extern char *set_default_rawintensity(const char *arg); |
||||||
|
extern char *set_default_thread_concurrency(const char *arg); |
||||||
|
#ifdef HAVE_ADL |
||||||
|
extern char *set_default_gpu_engine(const char *arg); |
||||||
|
extern char *set_default_gpu_memclock(const char *arg); |
||||||
|
extern char *set_default_gpu_threads(const char *arg); |
||||||
|
extern char *set_default_gpu_fan(const char *arg); |
||||||
|
extern char *set_default_gpu_powertune(const char *arg); |
||||||
|
extern char *set_default_gpu_vddc(const char *arg); |
||||||
|
#endif |
||||||
|
extern char *set_default_profile(char *arg); |
||||||
|
extern char *set_default_shaders(const char *arg); |
||||||
|
extern char *set_default_worksize(const char *arg); |
||||||
|
|
||||||
|
extern char *set_profile_name(const char *arg); |
||||||
|
extern char *set_profile_algorithm(const char *arg); |
||||||
|
extern char *set_profile_devices(const char *arg); |
||||||
|
extern char *set_profile_lookup_gap(const char *arg); |
||||||
|
extern char *set_profile_intensity(const char *arg); |
||||||
|
extern char *set_profile_xintensity(const char *arg); |
||||||
|
extern char *set_profile_rawintensity(const char *arg); |
||||||
|
extern char *set_profile_thread_concurrency(const char *arg); |
||||||
|
#ifdef HAVE_ADL |
||||||
|
extern char *set_profile_gpu_engine(const char *arg); |
||||||
|
extern char *set_profile_gpu_memclock(const char *arg); |
||||||
|
extern char *set_profile_gpu_threads(const char *arg); |
||||||
|
extern char *set_profile_gpu_fan(const char *arg); |
||||||
|
extern char *set_profile_gpu_powertune(const char *arg); |
||||||
|
extern char *set_profile_gpu_vddc(const char *arg); |
||||||
|
#endif |
||||||
|
extern char *set_profile_nfactor(const char *arg); |
||||||
|
extern char *set_profile_shaders(const char *arg); |
||||||
|
extern char *set_profile_worksize(const char *arg); |
||||||
|
|
||||||
|
/* config parser functions */ |
||||||
|
extern char *parse_config(json_t *val, const char *key, const char *parentkey, bool fileconf, int parent_iteration); |
||||||
|
extern char *load_config(const char *arg, const char *parentkey, void __maybe_unused *unused); |
||||||
|
extern char *set_default_config(const char *arg); |
||||||
|
extern void load_default_config(void); |
||||||
|
|
||||||
|
/* startup functions */ |
||||||
|
extern void load_default_profile(); |
||||||
|
extern void apply_defaults(); |
||||||
|
extern void apply_pool_profiles(); |
||||||
|
extern void apply_pool_profile(struct pool *pool); |
||||||
|
|
||||||
|
/* config writer */ |
||||||
|
extern void write_config(const char *filename); |
||||||
|
|
||||||
|
/* API functions */ |
||||||
|
extern void api_profile_list(struct io_data *io_data, __maybe_unused SOCKETTYPE c, __maybe_unused char *param, bool isjson, __maybe_unused char group); |
||||||
|
extern void api_profile_add(struct io_data *io_data, __maybe_unused SOCKETTYPE c, char *param, bool isjson, __maybe_unused char group); |
||||||
|
extern void api_profile_remove(struct io_data *io_data, __maybe_unused SOCKETTYPE c, char *param, bool isjson, __maybe_unused char group); |
||||||
|
extern void api_pool_profile(struct io_data *io_data, __maybe_unused SOCKETTYPE c, char *param, bool isjson, __maybe_unused char group); |
||||||
|
|
||||||
|
#endif // CONFIG_PARSER_H
|
@ -1,71 +1,94 @@ |
|||||||
{ |
{ |
||||||
"pools" : [ |
"pools": [ |
||||||
{ |
{ |
||||||
"name" : "Preferred Pool", |
"name":"Testpool X11", |
||||||
"url" : "http://url1:8332", |
"url": "stratum+tcp://url1:4440", |
||||||
"user" : "user1", |
"user": "user", |
||||||
"pass" : "pass1" |
"pass": "x", |
||||||
}, |
"priority": "1", |
||||||
{ |
"profile": "x11" |
||||||
"name" : "Failover Pool", |
}, |
||||||
"url" : "http://url2:8344", |
{ |
||||||
"user" : "user2", |
"name":"Testpool Scrypt", |
||||||
"pass" : "pass2", |
"url": "stratum+tcp://url2:3339", |
||||||
"state" : "enabled" |
"user": "user", |
||||||
}, |
"pass": "x", |
||||||
{ |
"priority": "2", |
||||||
"name" : "Failover Pool", |
"profile": "scrypt" |
||||||
"url" : "http://url3:8344", |
}, |
||||||
"user" : "user3", |
{ |
||||||
"pass" : "pass3", |
"name":"Testpool X11 with other profile", |
||||||
"algorithm" : "adaptive-n-factor", |
"url": "stratum+tcp://url3:4440", |
||||||
"state" : "disabled" |
"user": "user", |
||||||
}, |
"pass": "x", |
||||||
{ |
"priority": "0", |
||||||
"name" : "Testing Pool", |
"profile": "x11test" |
||||||
"url" : "http://url4:8332", |
}, |
||||||
"user" : "user4", |
{ |
||||||
"pass" : "pass4", |
"name":"Testpool Using default profile", |
||||||
"state" : "hidden" |
"url": "stratum+tcp://url4:3333", |
||||||
} |
"user": "user", |
||||||
], |
"pass": "x" |
||||||
|
} |
||||||
"failover-only" : true, |
], |
||||||
"failover-switch-delay" : "60", |
"profiles": [ |
||||||
"no-pool-disable" : true, |
{ |
||||||
|
"name": "x11", |
||||||
"kernel-path" : "/usr/local/bin", |
"algorithm": "darkcoin-mod", |
||||||
"kernel" : "alexkarold,ckolivas,zuikkis", |
"intensity": "19", |
||||||
|
"thread-concurrency": "10696,8192", |
||||||
"algorithm" : "scrypt", |
"worksize": "128", |
||||||
|
"gpu-engine": "1100", |
||||||
"intensity" : "d,9,9,9", |
"gpu-threads": "2", |
||||||
"xintensity" : "0,0,0", |
"gpu-fan": "75" |
||||||
"rawintensity" : "0,0,0", |
}, |
||||||
"lookup-gap" : "2,2,2", |
{ |
||||||
|
"name": "scrypt", |
||||||
"thread-concurrency" : "15508,15508,15508", |
"algorithm": "ckolivas", |
||||||
"shaders" : "0,0,0", |
"lookup-gap": "2", |
||||||
|
"intensity": "19,13", |
||||||
"gpu-threads" : "2", |
"thread-concurrency": "19656,8192", |
||||||
"gpu-engine" : "0-985,0-950,0-1000", |
"worksize": "256", |
||||||
"gpu-memclock" : "860,825,875", |
"gpu-engine": "1000,1065", |
||||||
"gpu-powertune" : "20,20,20", |
"gpu-memclock": "1500", |
||||||
|
"gpu-threads": "1,2", |
||||||
"gpu-vddc" : "0.000,0.000,0.000", |
"gpu-fan": "85", |
||||||
"gpu-fan" : "0-85,0-85,0-85", |
"gpu-powertune": "20" |
||||||
|
}, |
||||||
"auto-gpu" : true, |
{ |
||||||
"auto-fan" : true, |
"name": "x11test", |
||||||
|
"algorithm": "darkcoin-mod", |
||||||
"temp-target" : "75,75,75", |
"device": "1", |
||||||
"temp-overheat" : "80,85,90", |
"intensity": "19", |
||||||
"temp-cutoff" : "95", |
"thread-concurrency": "10696,8192", |
||||||
"temp-hysteresis" : "3", |
"worksize": "512", |
||||||
|
"gpu-engine": "1100", |
||||||
"queue" : "1", |
"gpu-threads": "2", |
||||||
"scan-time" : "7", |
"gpu-fan": "75" |
||||||
"expiry" : "28", |
} |
||||||
|
], |
||||||
"log" : "5" |
"failover-only": true, |
||||||
} |
"default-profile": "scrypt", |
||||||
|
"temp-cutoff": "95,95", |
||||||
|
"temp-overheat": "90,90", |
||||||
|
"temp-target": "80,80", |
||||||
|
"gpu-memdiff": "0,0", |
||||||
|
"shares": "0", |
||||||
|
"kernel-path": "/usr/local/bin", |
||||||
|
"api-allow": "W:127.0.0.1", |
||||||
|
"api-listen": true, |
||||||
|
"api-mcast-port": "4028", |
||||||
|
"api-port": "4028", |
||||||
|
"expiry": "1", |
||||||
|
"failover-switch-delay": "60", |
||||||
|
"gpu-dyninterval": "7", |
||||||
|
"gpu-platform": "-1", |
||||||
|
"hamsi-expand-big": "4", |
||||||
|
"log": "5", |
||||||
|
"no-pool-disable": true, |
||||||
|
"no-client-reconnect": true, |
||||||
|
"queue": "0", |
||||||
|
"scan-time": "1", |
||||||
|
"tcp-keepalive": "30", |
||||||
|
"temp-hysteresis": "3" |
||||||
|
} |
Loading…
Reference in new issue