mirror of https://github.com/GOSTSec/poolserver
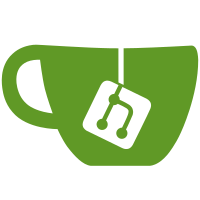
16 changed files with 278 additions and 58 deletions
@ -0,0 +1,65 @@
@@ -0,0 +1,65 @@
|
||||
#ifndef STRATUM_CLIENT_H_ |
||||
#define STRATUM_CLIENT_H_ |
||||
|
||||
#include "Common.h" |
||||
#include "Log.h" |
||||
#include "JSON.h" |
||||
|
||||
#include <boost/asio.hpp> |
||||
#include <boost/bind.hpp> |
||||
|
||||
#define MAX_PACKET 2048 |
||||
|
||||
using namespace boost; |
||||
using namespace boost::asio::ip; |
||||
|
||||
namespace Stratum |
||||
{ |
||||
class Client |
||||
{ |
||||
public: |
||||
Client(asio::io_service& io_service) : _socket(io_service) |
||||
{ |
||||
} |
||||
|
||||
tcp::socket& GetSocket() |
||||
{ |
||||
return _socket; |
||||
} |
||||
|
||||
void Start() |
||||
{ |
||||
boost::asio::async_read_until( |
||||
_socket, |
||||
_recvBuffer, |
||||
'\n', |
||||
boost::bind(&Client::_OnReceive, this, asio::placeholders::error, asio::placeholders::bytes_transferred)); |
||||
} |
||||
|
||||
void SendMessage(JSON msg) |
||||
{ |
||||
std::string data = msg.ToString(); |
||||
_socket.send(boost::asio::buffer(data.c_str(), data.length())); |
||||
} |
||||
|
||||
void OnMessage(JSON msg) |
||||
{ |
||||
std::string method = msg.Get<std::string>("method"); |
||||
sLog.Debug(LOG_SERVER, "Method: %s", method.c_str()); |
||||
} |
||||
public: |
||||
void _OnReceive(const boost::system::error_code& error, size_t bytes_transferred) |
||||
{ |
||||
std::istream is(&_recvBuffer); |
||||
std::stringstream iss; |
||||
iss << is.rdbuf(); |
||||
sLog.Debug(LOG_SERVER, "Received: %s", iss.str().c_str()); |
||||
OnMessage(JSON::FromString(iss.str())); |
||||
} |
||||
private: |
||||
asio::streambuf _recvBuffer; |
||||
tcp::socket _socket; |
||||
}; |
||||
} |
||||
|
||||
#endif |
@ -0,0 +1,122 @@
@@ -0,0 +1,122 @@
|
||||
#ifndef STRATUM_SERVER_H_ |
||||
#define STRATUM_SERVER_H_ |
||||
|
||||
#include "Common.h" |
||||
#include "Client.h" |
||||
#include "Config.h" |
||||
#include "Log.h" |
||||
#include "JSON.h" |
||||
#include "JSONRPC.h" |
||||
|
||||
#include <boost/asio.hpp> |
||||
#include <boost/bind.hpp> |
||||
#include <list> |
||||
|
||||
using namespace boost; |
||||
using namespace boost::asio::ip; |
||||
|
||||
namespace Stratum |
||||
{ |
||||
struct ListenInfo |
||||
{ |
||||
std::string Host; |
||||
uint16 Port; |
||||
}; |
||||
|
||||
class Server |
||||
{ |
||||
public: |
||||
Server(asio::io_service& io_service) : _io_service(io_service), _acceptor(io_service), _blockCheckTimer(io_service), _blockHeight(0) |
||||
{ |
||||
} |
||||
|
||||
~Server() |
||||
{ |
||||
std::list<Client*>::iterator it; |
||||
for (it = _clients.begin(); it != _clients.end(); ++it) |
||||
delete *it; |
||||
} |
||||
|
||||
void Start(tcp::endpoint endpoint) |
||||
{ |
||||
_CheckBlocks(); |
||||
|
||||
_acceptor.open(endpoint.protocol()); |
||||
_acceptor.set_option(tcp::acceptor::reuse_address(true)); |
||||
_acceptor.bind(endpoint); |
||||
_acceptor.listen(); |
||||
|
||||
_StartAccept(); |
||||
|
||||
sLog.Debug(LOG_STRATUM, "Stratum server started"); |
||||
} |
||||
|
||||
void SetBitcoinRPC(JSONRPC* bitcoinrpc) |
||||
{ |
||||
_bitcoinrpc = bitcoinrpc; |
||||
} |
||||
|
||||
void SendToAll(JSON msg) |
||||
{ |
||||
std::list<Client*>::iterator it; |
||||
for (it = _clients.begin(); it != _clients.end(); ++it) |
||||
(*it)->SendMessage(msg); |
||||
} |
||||
|
||||
private: |
||||
void _StartAccept() |
||||
{ |
||||
Client* client = new Client(_io_service); |
||||
|
||||
_acceptor.async_accept(client->GetSocket(), boost::bind(&Server::_OnAccept, this, client, asio::placeholders::error)); |
||||
} |
||||
|
||||
void _OnAccept(Client* client, const boost::system::error_code& error) |
||||
{ |
||||
if (!error) { |
||||
sLog.Debug(LOG_STRATUM, "New stratum client accepted"); |
||||
client->Start(); |
||||
_clients.push_back(client); |
||||
} else { |
||||
sLog.Debug(LOG_STRATUM, "Failed to accept stratum client"); |
||||
delete client; |
||||
} |
||||
|
||||
_StartAccept(); |
||||
} |
||||
|
||||
void _CheckBlocks() |
||||
{ |
||||
sLog.Debug(LOG_STRATUM, "Checking for new blocks..."); |
||||
|
||||
JSON response = _bitcoinrpc->Query("getinfo"); |
||||
uint32 curBlock = response.Get<uint32>("blocks"); |
||||
|
||||
// Initializing server
|
||||
if (_blockHeight == 0) { |
||||
_blockHeight = curBlock; |
||||
} else if (curBlock > _blockHeight) { |
||||
sLog.Debug(LOG_STRATUM, "New block on network! Height: %u", curBlock); |
||||
_blockHeight = curBlock; |
||||
// do some crazy stuff
|
||||
} |
||||
|
||||
_blockCheckTimer.expires_from_now(boost::posix_time::milliseconds(sConfig.Get<uint32>("StratumBlockCheckTime"))); |
||||
_blockCheckTimer.async_wait(boost::bind(&Server::_CheckBlocks, this)); |
||||
} |
||||
private: |
||||
// Network
|
||||
std::list<Client*> _clients; |
||||
asio::io_service& _io_service; |
||||
tcp::acceptor _acceptor; |
||||
|
||||
// RPC
|
||||
JSONRPC* _bitcoinrpc; |
||||
|
||||
// Bitcoin info
|
||||
asio::deadline_timer _blockCheckTimer; |
||||
uint32 _blockHeight; |
||||
}; |
||||
} |
||||
|
||||
#endif |
@ -1,11 +0,0 @@
@@ -1,11 +0,0 @@
|
||||
#ifndef BITCOINRPC_H_ |
||||
#define BITCOINRPC_H_ |
||||
|
||||
#include "JSONRPC.h" |
||||
|
||||
class BitCoinRPC: public JSONRPC |
||||
{ |
||||
|
||||
}; |
||||
|
||||
#endif |
@ -0,0 +1,40 @@
@@ -0,0 +1,40 @@
|
||||
#ifndef BITCOIN_H_ |
||||
#define BITCOIN_H_ |
||||
|
||||
namespace Bitcoin |
||||
{ |
||||
class TxIn |
||||
{ |
||||
}; |
||||
|
||||
class TxOut |
||||
{ |
||||
}; |
||||
|
||||
class Transaction |
||||
{ |
||||
uint32 Version; |
||||
std::vector<TxIn> in; |
||||
std::vector<TxOut> out; |
||||
uint32 LockTime; |
||||
}; |
||||
|
||||
class BlockHeader |
||||
{ |
||||
uint32 Version; |
||||
std::array<char, 32> PrevBlockHash; |
||||
std::array<char, 32> MerkleRootHash; |
||||
uint32 Time; |
||||
uint32 Bits; |
||||
uint32 Nonce; |
||||
}; |
||||
|
||||
class Block : public BlockHeader |
||||
{ |
||||
std::vector<Transaction> tx; |
||||
}; |
||||
|
||||
class BlockTemplate |
||||
} |
||||
|
||||
#endif |
@ -1,6 +0,0 @@
@@ -1,6 +0,0 @@
|
||||
#include "Server.h" |
||||
|
||||
Stratum::Server::Server(std::string ip, uint32_t port) |
||||
{ |
||||
|
||||
} |
@ -1,20 +0,0 @@
@@ -1,20 +0,0 @@
|
||||
#ifndef STRATUM_SERVER_H_ |
||||
#define STRATUM_SERVER_H_ |
||||
|
||||
#include <boost/cstdint.hpp> |
||||
#include <string> |
||||
|
||||
namespace Stratum |
||||
{ |
||||
class Server |
||||
{ |
||||
public: |
||||
Server(std::string ip, uint32_t port); |
||||
~Server(); |
||||
|
||||
private: |
||||
|
||||
}; |
||||
} |
||||
|
||||
#endif |
Loading…
Reference in new issue